What is Log4j?
Log4j stands for Log for Java. It’s a straightforward, dependable, fast, and adaptable logging framework developed in Java. Log4j libraries are notable for their high configurability through external configuration files during runtime. Configuration for Log4j can be done using various types of files, such as XML, properties, JSON, etc. Additionally, Log4j has been adapted to languages beyond Java, including C, C++, Perl, Python, and C#.
Why Opt for Log4j?
- Open-Source: Log4j is an open-source tool, making it accessible and customizable.
- Suitable for Projects of All Sizes: It finds utility in both small and large-scale projects.
- Structured Flow Documentation: Log4j enables the storage of project flow details in files or databases.
- Enhanced Status Insight: Instead of using regular output statements (SOPL), log statements within the code provide a clear picture of project status during execution. This aids in effective debugging and monitoring.
Main Components of Log4j:
Loggers: Loggers are employed to record information. Implementing loggers in your project involves several steps:
- Create an instance of the logger class: The Logger class is a Java utility with pre-implemented generic methods for log4j usage.
- Specify the Log4j level: There are five available log levels:
- All: Captures everything at this level.
- DEBUG: Logs debugging information, useful during development.
- INFO: Logs informational messages to track application progress.
- WARN: Logs problematic information and unexpected system behavior.
- ERROR: Logs error messages to aid in troubleshooting while allowing the system to continue.
- FATAL: Logs critical system information causing application crashes.
- OFF: Disables logging.
Appenders: Appenders deliver logging information to specified destinations such as databases, files, consoles, etc. Various types of appenders include:
- ConsoleAppender: Logs to standard output.
- File Appender: Prints logs to a designated file.
- Rolling File Appender: Appends to a file with a maximum size.
- Note: Appenders can be called by any name in the log4j properties.
- Layouts: Layouts are used to format logging information in diverse styles.
To manage logging activities, the Logger class offers various methods. It provides two static methods to obtain a Logger object.
Configuring Log4j:
To set up log4j, follow these steps:
- Decide on the Appender: Determine which appender to use for configuration. Adjust the appender’s parameters accordingly.
- Implement DEBUG Level and RollingFileAppender: Choose the DEBUG log level and configure the RollingFileAppender parameters.
- Set Up Two Logs:
- Root Logger: This logger records all system-generated logs and writes them to a file named “Testing.logs.”
- Application Logger: This logger captures information generated by manual commands in the code and logs them to a file named “Manual.logs.”
- Utilize the PatternLayout: Apply the PatternLayout for formatting.
For the root logger, the configuration might look like this:
log4j.rootLogger=DEBUG,files log4j.appender.files=org.apache.log4j.RollingFileAppender log4j.appender.files.File=E:\\Testing\\src\\Testing.logs log4j.appender.files.maxFileSize=800KB log4j.appender.files.maxBackupIndex=6 log4j.appender.files.layout=org.apache.log4j.PatternLayout log4j.appender.files.layout.ConversionPattern=%d{ABSOLUTE} %5p %c<strong>{1}</strong>:%L - %m%n log4j.appender.files.Append=false
Application Logs
log4j.logger.devpinoyLogger=DEBUG, dest2 log4j.appender.dest2=org.apache.log4j.RollingFileAppender log4j.appender.dest2.maxFileSize=800KB log4j.appender.dest2.maxBackupIndex=6 log4j.appender.dest2.layout=org.apache.log4j.PatternLayout log4j.appender.dest2.layout.ConversionPattern=%d{dd/MM/yyyy HH:mm:ss} %c %m%n log4j.appender.dest2.File=E:\\Testing\\src\\ManualTest.logs log4j.appender.dest2.Append=false
In the provided example, we’ve configured log4j to save logs in two distinct files: “Testing.logs” and “ManualTest.logs.”
In the configuration, “File” is utilized to specify the file name where the logs will be stored. Additionally, we have “dest2” and other identifiers.
The “maxFileSize” parameter configures the maximum size of each log file. When the file size reaches this limit, a new file is created with the same name, and the old file is indexed.
“maxBackupIndex” determines the maximum number of backup files to retain.
The “layout” parameter sets the format for the log file.
Setting “Append” to false will create new files for each logging instance rather than appending to existing files.
How to Use Log4j in Your Script:
In the code snippet below, we’ve employed “log” as a reference variable linked to the getLogger method of the Logger Class.
import org.apache.log4j.Logger;
public class ExampleClass {
private static final Logger log = Logger.getLogger(ExampleClass.class);
public static void main(String[] args) {
log.debug("This is a debug message.");
log.info("This is an info message.");
log.warn("This is a warning message.");
log.error("This is an error message.");
log.fatal("This is a fatal message.");
}
}
Using Log4j with Selenium:
Step 1: Open Eclipse and create a new project named “log4j_test.”
Step 2: Right-click on the “src” folder, then select “Build Path” and choose “Configure Build Path.”
Step 3: Navigate to the “Libraries” tab and incorporate Log4j libraries. You can obtain the Log4j libraries by downloading them from the following URL: https://logging.apache.org/log4j/1.2/download.html
Step 4: Extract the downloaded Log4j file and integrate the Log4j libraries into your project.
Step 5: Generate a new file:
- Right-click on the “src” folder.
- Choose “New,” then select “Other.”
- Under “General,” choose “File.”
- Input “log4j.properties” as the file name.
- Click on the “Finish” button.
Create two additional files with the names “Testing.logs” and “ManualTest.logs.” These files will contain all the logs generated by the system and manually logged statements, respectively.
Step 6: Copy the configuration in log4j.properties
#Root logger log4j.rootLogger=DEBUG,files log4j.appender.files=org.apache.log4j.RollingFileAppender log4j.appender.files.File=D:\\selenium_log4j\\log4jDemo\\src\\Testing.logs log4j.appender.files.maxFileSize=800KB log4j.appender.files.maxBackupIndex=6 log4j.appender.files.layout=org.apache.log4j.PatternLayout log4j.appender.files.layout.ConversionPattern=%d{ABSOLUTE} %5p %c<strong>{1}</strong>:%L - %m%n log4j.appender.files.Append=false #Application Logs log4j.logger.devpinoyLogger=DEBUG, dest1 log4j.appender.dest1=org.apache.log4j.RollingFileAppender log4j.appender.dest1.maxFileSize=800KB log4j.appender.dest1.maxBackupIndex=6 log4j.appender.dest1.layout=org.apache.log4j.PatternLayout log4j.appender.dest1.layout.ConversionPattern=%d{dd/MM/yyyy HH:mm:ss} %c %m%n log4j.appender.dest1.File=D:\\selenium_log4j\\log4jDemo\\src\\ManualTest.logs log4j.appender.dest1.Append=false
Step 7: Generate a Main Class
- Right-click on the “src” directory.
- Choose “New,” then select “Class.”
- Enter the class name as “Log4jDemo.”
- Click on the “Finish” button.
package log4j_test;
import java.util.concurrent.TimeUnit;
import org.apache.log4j.Logger;
import org.apache.log4j.PropertyConfigurator;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
public class Log4jDemo {
public static void main(String[] args) {
System.setProperty("webdriver.gecko.driver","F:\\drivers\\geckodriver.exe");Â Â
    WebDriver driver = new FirefoxDriver();
        Logger log = Logger.getLogger("devpinoyLogger");
       Â
        driver.get("https://www.google.co.in");
log.debug("opening webiste");
        driver.manage().timeouts().implicitlyWait(20, TimeUnit.SECONDS);
       driver.findElement(By.name("q")).sendKeys("Selenium");
       log.debug("Entered Selenium Keyword");
}
}
We establish system-level logs using the command: Logger.getLogger("devpinoyLogger")
.
To store data in the “Manual.log” file, we utilize the log.debug
method.
Step 8: Execute the script and navigate to the locations of “ManualTest” and “Testing” logs to inspect the logged data.
XML File Configuration with Log4j:
Log4j’s logging system can also be configured using an XML configuration file. Below are the steps to set up log4j libraries using XML:
- The attributes enclosed between
<appender>
and</appender>
define the properties of the appender component. - The attribute “name” with the values “fileAppender” and “Rolling file appender” signifies the utilization of the RollingFileAppender as the output type.

3. The “name” attribute with the value “File” indicates that it is a file-based appender, and the “file” attribute points to the location where the output should be stored.

4. The <layout>
tag specifies the type of layout desired in the output, in this case, a pattern layout.

5. The <priority>
tag defines the priority level.

Integration of XML with Log4j
1. Create a Java class and create an object for logger class using getLogger() method from Logger class.

2. Load the xml file using configure method from the DOMConfigurator class

3. Log a message to the file

Complete program for XML integration with log4j
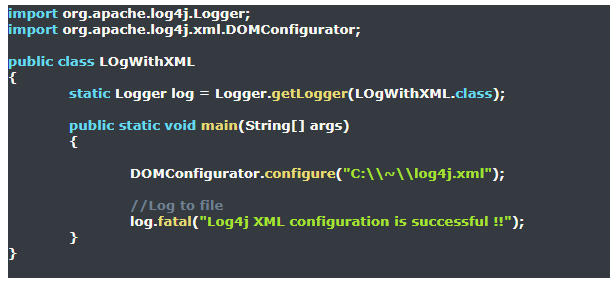
Conclusion:
- Log4j is a simple, reliable, fast, and flexible login framework which is written in java language.