What is JavaScript?
JavaScript is the primary language used within web browsers to enable interaction with HTML content. This means that browsers come equipped with a JavaScript engine that can interpret and execute JavaScript code. As a result, JavaScript commands can dynamically manipulate HTML elements, respond to user inputs, and update web pages without needing a full reload.
In a Selenium WebDriver course, you’ll learn how to leverage JavaScript execution within Selenium to handle these dynamic changes, enabling you to interact with web elements more efficiently and create robust automated tests for responsive web applications.
Developers can create interactive, responsive, and user-friendly web applications by embedding JavaScript directly in HTML or linking it through external files. JavaScript’s seamless integration with HTML and CSS makes it a cornerstone of modern web development, allowing for a rich, interactive user experience across various devices and platforms.
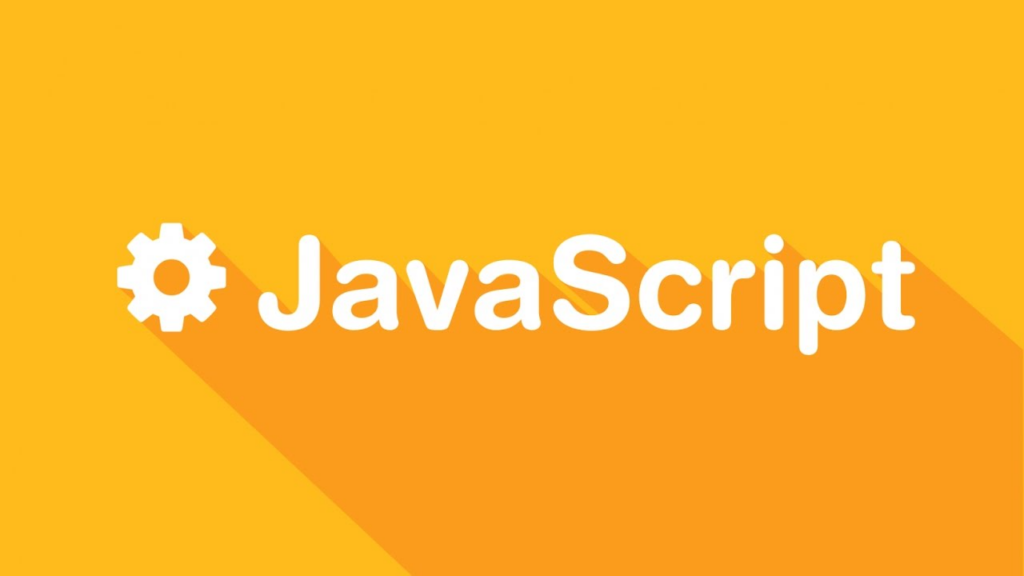
What is JavaScriptExecutor?
JavaScriptExecutor
is an interface in Selenium WebDriver that provides a way to execute JavaScript directly within the browser context from a test script. This interface is particularly useful when regular WebDriver methods fall short in interacting with certain elements or performing complex tasks. For example, JavaScriptExecutor
can manipulate the DOM, retrieve values, or handle elements hidden behind layers, helping tests to interact with dynamic web content more effectively.
JavaScriptExecutor
offers two primary methods: executeScript
and executeAsyncScript
. The executeScript
method runs JavaScript synchronously on the page, making it suitable for quick actions like modifying element properties or scrolling.
In contrast, executeAsyncScript
is used for asynchronous operations, pausing the WebDriver until the script completes. This is especially helpful for handling AJAX calls or other background processes. Together, these methods expand Selenium’s capabilities, allowing tests to interact deeply with modern, dynamic web application.
Why do we need to perform operations using JavaScriptExecutor?
Using Selenium Webdriver you can perform any kind of operations like sending the text, click on a button, and click on a radio button, select the drop-downs. So, in that case, why we need to perform operations using JavaScript is because in some applications on some web elements the actual selenium webdriver commands will not work even though we write the code correctly. In those scenarios, to perform the operations we take the help of JavaScript.
By using the JavaScriptExecutor interface you can perform those operations. For example, if we want to handle any dropdown then we use a select class provided by selenium.Similarly, for handling JavaScript operations selenium webdriver provides JavaScriptExecutor as an interface. There is no need to add for an extra plugin or add-on. To use JavaScriptExecutor you just need to import (org.openqa.selenium.JavascriptExecutor) in the script.
JavaScriptExecutor Methods
- executeAsyncScript
The executeAsyncScript
command in Selenium allows JavaScript to be executed as an asynchronous snippet within the context of the currently selected window or frame. This is particularly useful for handling scenarios where elements load dynamically or involve delayed actions, such as AJAX calls.
The JavaScript snippet is executed as the body of an anonymous function, ensuring that the code runs in isolation without affecting the rest of the page’s scripts.
To capture the result of the script, the return
keyword is used, allowing the returned value to be stored in a designated variable. This method is ideal for situations where a test must wait for specific processes to complete, like loading data or responding to user interactions, enhancing test reliability by allowing WebDriver to pause until the JavaScript function signals it has finished.
- executeScript
This method allows JavaScript to execute within the context of the currently selected window or frame in Selenium, giving direct access to elements and properties on the page that may otherwise be challenging to interact with. When using this method, the JavaScript code runs within the body of an anonymous function, creating a secure and isolated execution environment.
This setup enables the function to execute commands without interference and allows complex arguments to be passed into the function seamlessly.
By doing so, it supports the execution of intricate tasks like handling elements embedded in iframes, controlling animations, and managing AJAX-driven updates, which might not be possible with standard WebDriver commands. This method is a powerful tool for automating sophisticated interactions in dynamic web applications.
The script will return the values and the data types returned are
- Long
- String
- List
- WebElement.
- Boolean
The basic syntax for JavascriptExecutor:
Package:
import org.openqa.selenium.JavascriptExecutor;
Syntax:
JavascriptExecutor js = (JavascriptExecutor) driver;
js.executeScript(Script,Arguments);
Script – To execute the JavaScript
Arguments –The arguments we pass to the script(Optional). May be empty.
Returns –One of Boolean, String, Long, List, WebElement, or null.
Let’s look at some scenarios we could handle using this JavaScript Interface:
- To click on a Button using JavaScript in Selenium WebDriver
js.executeScript("document.getElementById('enter element id').click();");
//or
js.executeScript("arguments[0].click();", okButton);
- To generate Alert Pop window in Selenium WebDriver
js.executeScript("alert('Welcome To SeleniumTesting');");
- Typing Text without using sendKeys() method in Selenium WebDriver
js.executeScript("document.getElementById(id').value='someValue';");
js.executeScript("document.getElementById('Email').value='SeleniumTesting.com';");
- To handle the Checkbox in Selenium WebDriver
js.executeScript("document.getElementById('enter element id').checked=false;");
- To print the Title of a webpage
String titleText = js.executeScript("return document.title;").toString();
System.out.println(titleText);
- Refreshing the browser window using Javascript
((JavascriptExecutor)driver).executeScript(“history.go(0)”);
- To get entire webpage innertext in Selenium WebDriver
String innerText = js.executeScript(" return document.documentElement.innerText;").toString();
System.out.println(innerText);
- To make the checkbox has checked
((JavaScriptExecutor)driver.ExecuteScript(“document.querySelectorAll(‘input[value = read]’)[0].click()”);
- To get the Domain of a web page
((JavascriptExecutor)driver).executeScript(“return document.domain;”).toString();
- To get the URL of the web Page
((JavascriptExecutor)driver).executeScript(“return document.URL;”).toString();
- To Scroll the Page
To scroll the page vertically for 600px we use the following code
((JavascriptExecutor)driver).executeScript(“window.scrollBy(0,600)”);
To scroll the page vertically till the end we use the following code
((JavascriptExecutor)driver).executeScript(“window.scrollBy(0,document.body.scrollHeight)”);
- To navigate to the other Page
((JavascriptExecutor)driver).executeScript(“window.location=’http://SeleniumTesting.com’”);
- To get the Height and Width of a web page
((JavascriptExecutor)driver).executeScript(“return window.innerHeight;”).toString();((JavascriptExecutor)driver).executeScript(“return window.innerWidth;”).toString();
Example 1: Performing a sleep operation in the browser.
Scenario:
- Launch the browser.
- Open site “http://www.facebook.com”
- To perform further action the Application waits for 5 sec.
package SamplePackage;
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.annotations.Test;
public class TestJava {
@Test
public void Login()
{
System.setProperty("webdriver.chrome.driver","F:\\drivers\\chromedriver.exe");
//create chrome instance
driver = new ChromeDriver();
JavascriptExecutor js = (JavascriptExecutor)driver;
//Launching the Site.
driver.get("http://www.facebook.com");
//Maximize window
driver.manage().window().maximize();
//Set the Script Timeout to 10 seconds
driver.manage().timeouts().setScriptTimeout(10, TimeUnit.SECONDS);
//Declare and set the start time
long start_time = System.currentTimeMillis();
//Calling executeAsyncScript() method and wait for 5 seconds
js.executeAsyncScript("window.setTimeout(arguments[arguments.length - 1], 5000);");
//Get the difference (currentTime - startTime) of times.
System.out.println("Passed time: " + (System.currentTimeMillis() - start_time));
}
}
Example 2: Below program will handle some of the scenarios we use in while writing scripts.
package seleniumTesting;
import org.openqa.selenium.By;
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.annotations.Test;
public class JavaScriptExecutorClass {
static WebDriver driver;
@Test
public static void javaScriptExeMethod(){
System.setProperty("webdriver.chrome.driver","F:\\drivers\\chromedriver.ex
//create chrome instance
driver = new ChromeDriver();
driver.get("https://www.gmail.com");
WebElement loginButton = driver.findElement(By.xpath("//*[@id='next']"));
JavascriptExecutor js = (JavascriptExecutor)driver;
*//Typing the text in Selenium WebDriver without using sendKeys() method
js.executeScript("document.getElementById('some id').value='someValue';");
js.executeScript("document.getElementById('Email').value='SeleniumTesting.com';");*/
/*//To click a button using JavaScript
//js.executeScript("arguments[0].click();", okButton);
//or
js.executeScript("document.getElementById('enter element id').click();");
js.executeScript("document.getElementById(ok).click();");*/
/*//to handle checkbox
js.executeScript("document.getElementById('enter element id').checked=false;");
/*//to generate Alert Pop window in selenium
js.executeScript("alert('Java world);");*/
/*//to refresh browser window using Javascript
((JavascriptExecutor)driver).executeScript(“history.go(0)”);
*/
/*// to get the innertext of the webpage in Selenium
String innerText = js.executeScript(" return document.documentElement.innerText;").toString();
System.out.println(innerText);
/*//to get the Title of our webpage
String titleText = js.executeScript("return document.title;").toString();
System.out.println(titleText);
/*//to get the domain
String dText = js.executeScript("return document.domain;").toString();
System.out.println(dText);*/
/*//to get the URL of our webpage
String urlText = js.executeScript("return document.URL;").toString();
System.out.println(urlText);*/
/*//to perform Scroll on application using Selenium
//Vertical scroll - down by 600 pixels
((JavascriptExecutor)driver).executeScript(“window.scrollBy(0,600)”);
// we use the code for scrolling till the bottom of the page
//js.executeScript("window.scrollBy(0,document.body.scrollHeight)");*/
/*//to navigate to different page using Javascript
//Navigate to new Page
js.executeScript("window.location = 'https://www.seleniumtesting.com");*/
}
}
Example 3: Scroll Down using JavaScriptExecutor.
Execute the below selenium script.
- Launch the site
- Scroll down by 500 pixel
package SamplePackage;
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.annotations.Test;
public class ScrollDown_Test {
@Test
public void Login()
{
System.setProperty("webdriver.chrome.driver","F:\\drivers\\chromedriver.exe");
//create chrome instance
WebDriver driver = new ChromeDriver();
//Creating the JavascriptExecutor interface object by Typecasting
JavascriptExecutor js = (JavascriptExecutor)driver;
//Launching the Site.
driver.get("http://www.facebook.com/");
//Maximize window
driver.manage().window().maximize();
//Vertical scroll down by 500 pixels
js.executeScript("window.scrollBy(0,500)");
}
}
Conclusion:
JavaScriptExecutor is often employed in Selenium WebDriver tests when standard WebDriver methods like click()
fail to interact with certain web elements due to various issues. These issues can arise from elements being hidden behind layers, not fully loaded, or obscured by dynamic content on the page. JavaScriptExecutor bypasses these limitations by allowing direct interaction with the element through JavaScript, ensuring that the element is clicked regardless of its visibility state or overlapping layers.
This approach can be particularly effective for complex or highly dynamic websites where traditional WebDriver methods struggle with locating or clicking elements, thus enhancing the reliability of automated test scripts by handling interactions that would otherwise cause test failures.
- To handle, JavaScriptExecutor offers two methods “executeAsyncScript” and “executescript”.
- JavaScriptExecutor will execute the JavaScript using Selenium Webdriver.
- Fetched URL, the title of the page, and domain name using JavaScriptExecutor.
- Generate the ‘Alert’ window by using JavaScriptExecutor in Selenium Webdriver.
- Scroll the window to down using JavaScriptExecutor.
- Will navigate to the different pages using JavaScriptExecutor.
Call to Action
Unlock the full potential of Selenium WebDriver with our detailed guide on JavaScriptExecutor in Selenium WebDriver with Example at H2K Infosys! Learn how to leverage JavaScript commands to interact seamlessly with web elements, handle dynamic content, and boost your automation skills.
Dive into hands-on examples with our expert-led tutorials and take your testing expertise to the next level. Join H2K Infosys today and master the art of effective test automation with JavaScriptExecutor in Selenium!