Introduction
In today’s competitive IT landscape, automation testing has become a cornerstone of efficient software delivery. Selenium C# WebDriver NUnit is one of the most robust combinations for automation testing, enabling testers to ensure software quality while meeting tight deadlines. If you’re eager to learn how to master this stack, our Selenium course online at H2K Infosys can help you build expertise.
Why Choose Selenium C# WebDriver NUnit?
Automation testing has become an essential practice in modern software development, ensuring faster releases, higher accuracy, and improved software quality. Among the many tools and frameworks available, Selenium continues to dominate as the most preferred automation testing tool in the industry. When paired with C# and NUnit, it becomes a powerful framework for creating maintainable, scalable, and efficient test scripts. This trio is especially advantageous for teams working on .NET-based applications. Below, we explore the reasons why this combination of Selenium C# Webdriver Nunit is a game-changer for automation testing.
Selenium: The Gold Standard for Automation Testing
Selenium has earned its place as the go-to tool for web application automation due to its flexibility, cross-browser compatibility, and community support. It supports multiple programming languages, including C#, making it suitable for diverse development environments. Selenium WebDriver allows direct interaction with browsers, enabling robust test case execution with real-world scenarios. Its ability to handle dynamic elements and execute parallel testing makes it indispensable for testers.
C#: A Powerful and Versatile Programming Language
C# is a robust, type-safe, and object-oriented programming language developed by Microsoft. It is a favorite among developers working in .NET ecosystems due to its rich features, ease of use, and excellent integration capabilities. Writing Selenium scripts in C# is straightforward and provides seamless access to the .NET libraries, enabling testers to create clean, reusable, and modular code.
NUnit: A Reliable Testing Framework
NUnit is an open-source unit testing framework that complements C# beautifully. It offers features like annotations, test runners, and assertions, which simplify writing and managing test cases. Its support for parallel testing and integration with Continuous Integration (CI) tools like Jenkins makes it an excellent choice for enterprise-level applications. NUnit’s compatibility with Selenium further enhances its appeal, making it easy to structure test suites and achieve comprehensive test coverage.
Ideal for .NET-Based Applications
The Selenium C# WebDriver NUnit combination is particularly well-suited for testing .NET-based web applications. Since C# and NUnit are integral parts of the .NET ecosystem, developers and testers can leverage their familiarity with these tools to build efficient and reliable automation scripts. This reduces the learning curve and boosts productivity, enabling quicker adoption within teams.
Maintainable and Scalable Test Frameworks
Automation scripts are only as effective as their ability to adapt to changes in the application under test. By using Selenium WebDriver, C#, and NUnit together, testers can create highly maintainable and scalable test frameworks. The object-oriented nature of C# allows for the creation of reusable components, while NUnit’s modular test execution makes it easy to update or add new tests without disrupting existing workflows.
Cross-Browser and Platform Compatibility
Selenium WebDriver supports cross-browser testing, allowing teams to validate their applications on multiple browsers like Chrome, Firefox, Edge, and Safari. Combined with the power of NUnit and C#, testers can write platform-agnostic scripts that ensure consistent performance across devices and operating systems, which is crucial in today’s diverse digital landscape.
Strong Community and Resource Availability
Both Selenium and NUnit boast active communities and extensive online resources. This means that developers and testers can access comprehensive documentation, tutorials, and forums to troubleshoot issues and stay updated with the latest advancements. The vibrant community around C# in the .NET ecosystem further enhances the overall support available for this combination.
Seamless Integration with CI/CD Pipelines
Automation testing is an integral part of DevOps workflows, and the Selenium C# WebDriver NUnit setup integrates seamlessly with CI/CD pipelines. Tools like Jenkins, Azure DevOps, and GitHub Actions can easily execute NUnit test suites, ensuring continuous testing and rapid feedback in the software delivery lifecycle.
Cost-Effective and Open Source
All three components Selenium, C#, and NUnit are open source or free to use, making this combination a cost-effective solution for small to large-scale testing projects. Organizations can build sophisticated test frameworks without incurring additional licensing costs, which is particularly beneficial for startups and growing businesses.
Enhanced Test Script Debugging and Reporting
Debugging is a critical aspect of test script development, and using C# with NUnit provides robust debugging tools within Visual Studio. Additionally, NUnit’s reporting features enable teams to gain actionable insights into test results, facilitating informed decision-making and continuous improvement.
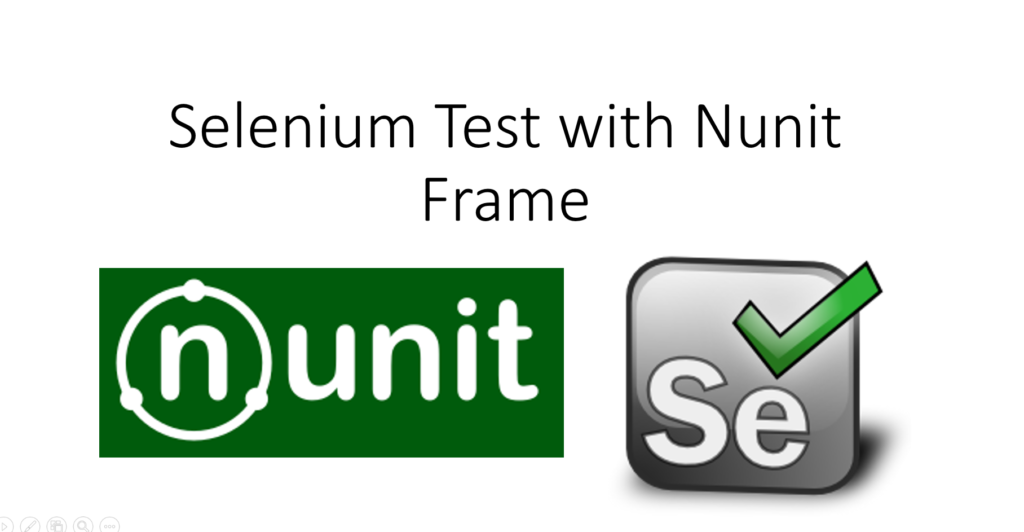
At H2K Infosys, our Selenium certification course equips you with the knowledge and hands-on experience to master these tools, enabling you to advance in your career and deliver reliable software.
Understanding the Components
Selenium WebDriver
Selenium WebDriver is an open-source tool that automates web browsers. It allows testers to simulate user interactions with a website, making it possible to validate the functionality of web applications efficiently.
Key Features of Selenium WebDriver:
- Cross-browser support (e.g., Chrome, Firefox, Edge)
- Platform independence
- Integration with programming languages like C#, Java, Python, and more
- Flexibility in designing custom test frameworks
C#: The Programming Powerhouse
C# is a modern, object-oriented programming language developed by Microsoft. Its strong typing, readability, and compatibility with .NET frameworks make it a natural choice for building test scripts.
Why C# for Selenium?
- Seamless integration with Visual Studio
- Rich library support
- High performance in handling large test cases
- Familiarity with .NET developers
NUnit: The Test Runner Framework
NUnit is a widely used testing framework for .NET languages, including C#. It enables developers to write and execute unit tests, making it a key component in automation testing.
Features of NUnit:
- Data-driven testing support with attributes like
[TestCase]
and[TestFixture]
- Easy assertion handling
- Parallel test execution
- Strong community support
Setting Up Selenium C# WebDriver NUnit
Setting up Selenium C# WebDriver NUnit is a crucial step in building a robust test automation framework. This Selenium C# Webdriver Nunit process ensures that your environment is fully equipped to write, execute, and manage automated test cases efficiently.
The setup involves configuring your development tools, downloading necessary packages, and creating a seamless integration between Selenium WebDriver, the C# programming language, and the NUnit framework. With the right setup, you can unlock the full potential of this powerful combination, enabling effective automation testing for web applications. Let’s walk through the step-by-step process of Selenium C# Webdriver Nunit to ensure you’re ready to start your automation journey.
Prerequisites
To get started with Selenium C# WebDriver NUnit, you’ll need:
- Visual Studio IDE (Community edition works well)
- NuGet Package Manager
- Latest Selenium WebDriver
- NUnit and NUnit3TestAdapter packages
Installation Steps
- Install Visual Studio: Download and install Visual Studio Community Edition.
- Create a New Project: Open Visual Studio and select “Create a new project.” Choose a Console App (.NET Framework) template.
- Add Required Packages: Use the NuGet Package Manager to install Selenium WebDriver and NUnit packages. Run the following commands in the Package Manager Console:bashCopyEdit
Install-Package Selenium.WebDriver Install-Package NUnit Install-Package NUnit3TestAdapter
- Set Up WebDriver: Download the required browser driver (e.g., ChromeDriver) and configure it in your project directory.
Writing Your First Selenium C# WebDriver NUnit Test
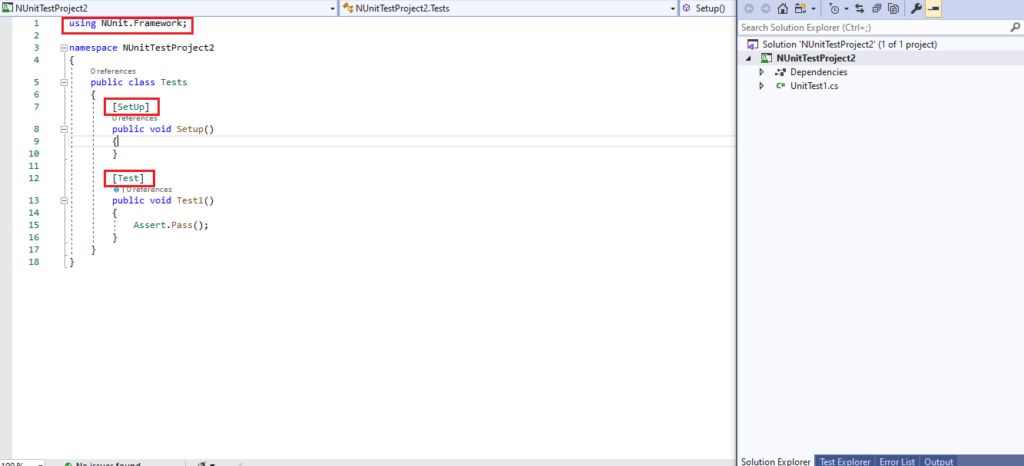
Here’s an example of a Selenium C# Webdriver Nunit simple test case that navigates to Google and verifies the title:
csharpEditusing NUnit.Framework;
using OpenQA.Selenium;
using OpenQA.Selenium.Chrome;
namespace SeleniumTests
{
[TestFixture]
public class GoogleTests
{
IWebDriver driver;
[SetUp]
public void StartBrowser()
{
driver = new ChromeDriver();
driver.Manage().Window.Maximize();
}
[Test]
public void VerifyGoogleTitle()
{
driver.Navigate().GoToUrl("https://www.google.com");
string title = driver.Title;
Assert.AreEqual("Google", title, "Title does not match!");
}
[TearDown]
public void CloseBrowser()
{
driver.Quit();
}
}
}
Explanation:
- [SetUp]: Initializes the WebDriver before each test.
- [Test]: Defines a test method to verify Google’s title.
- [TearDown]: Closes the browser after test execution.
Best Practices for Selenium C# WebDriver NUnit
To maximize the efficiency of your automation testing with Selenium C# WebDriver NUnit, it’s essential to follow best practices. Use Page Object Model (POM) to create reusable and maintainable test scripts. Leverage NUnit attributes like [SetUp]
and [TearDown]
for organizing test cases.
Incorporate parallel test execution to save time and ensure thorough test coverage. Additionally, handle dynamic elements effectively and use meaningful assertions to validate test results. By adopting these strategies, your Selenium C# WebDriver NUnit framework can achieve scalability, reliability, and faster testing cycles, ensuring superior software quality.
Use Page Object Model (POM)
The Page Object Model is a design pattern that enhances code maintainability. It separates the UI logic from test logic, making your test scripts easier to update.
Implement Explicit Waits
Instead of relying on implicit waits, use WebDriverWait for better control over element loading:
csharpCopyEditWebDriverWait wait = new WebDriverWait(driver, TimeSpan.FromSeconds(10));
IWebElement element = wait.Until(SeleniumExtras.WaitHelpers.ExpectedConditions.ElementIsVisible(By.Id("example")));
Parameterize Test Data
Use NUnit’s [TestCase]
attribute to execute tests with multiple input values:
csharpCopyEdit[TestCase("https://www.google.com", "Google")]
[TestCase("https://www.bing.com", "Bing")]
public void VerifyTitle(string url, string expectedTitle)
{
driver.Navigate().GoToUrl(url);
Assert.AreEqual(expectedTitle, driver.Title, "Title does not match!");
}
Why Choose H2K Infosys for Selenium Automation Testing?
At H2K Infosys, we offer comprehensive Selenium course online that combines theoretical knowledge with hands-on experience. Our courses are designed to cater to beginners as well as experienced professionals.
Key Highlights:
- Expert-Led Training: Learn from seasoned industry experts with real-world experience.
- Hands-On Projects: Work on real-time projects to build confidence in handling complex scenarios.
- Flexible Learning: Access our courses anytime, anywhere with lifetime access to materials.
- Career Support: Get resume assistance, interview prep, and job placement guidance.
By enrolling in our Selenium certification course, you’ll gain the skills needed to build robust test frameworks using Selenium C# WebDriver NUnit.
Applications of Selenium C# WebDriver NUnit
Cross-Browser Testing
Easily validate the compatibility of your web applications across multiple browsers.
Regression Testing
Automate repetitive test cases to save time and ensure consistent software quality.
Continuous Integration/Continuous Deployment (CI/CD)
Integrate your test scripts with CI/CD tools like Jenkins for seamless software delivery.
Common Challenges and How to Overcome Them
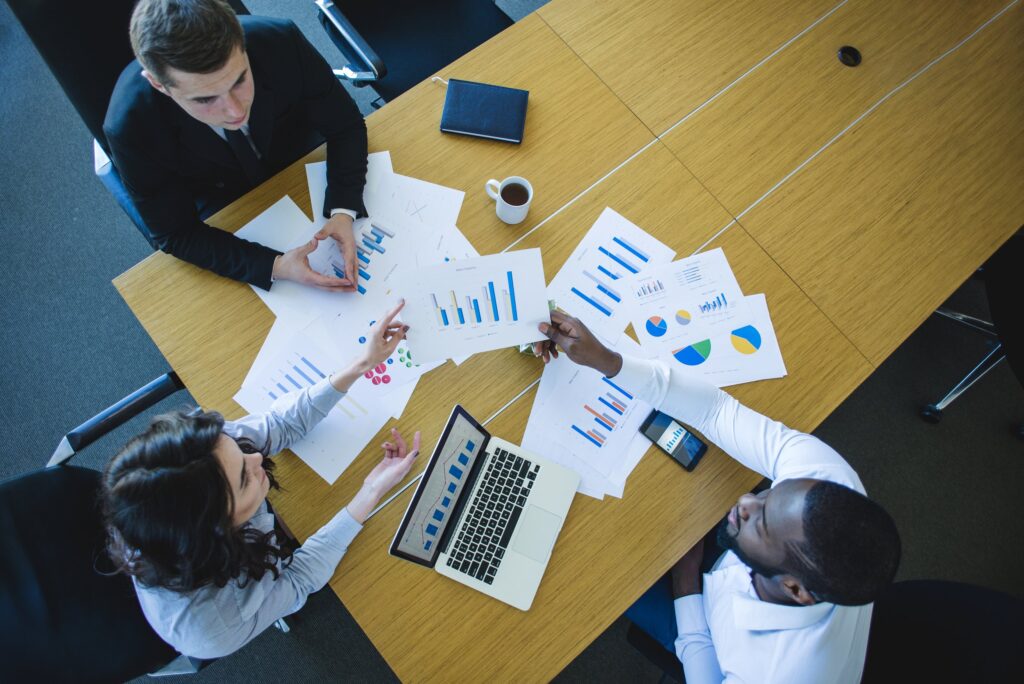
Handling Dynamic Elements
Dynamic elements can cause test failures. Use robust locators like XPath or CSS Selectors and implement explicit waits.
Browser Compatibility Issues
Ensure you have the latest browser drivers and test on different versions to avoid compatibility issues.
Debugging Failures
Leverage NUnit’s detailed test reports to quickly identify and resolve issues.
Conclusion
Mastering Selenium C# WebDriver NUnit opens doors to exciting opportunities in the field of automation testing. With its versatility and power, this stack is ideal for building scalable and maintainable test frameworks.
Enroll in H2K Infosys’s Selenium certification course to gain hands-on experience, industry insights, and expert guidance. Whether you’re a beginner or a seasoned professional, our courses will help you excel in automation testing.