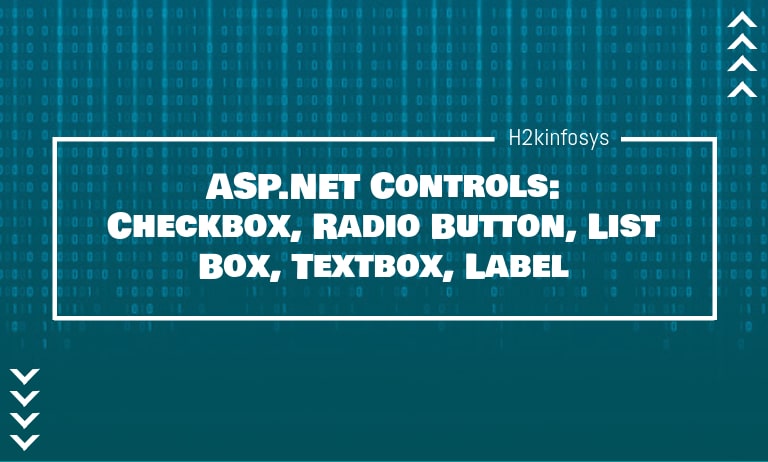
ASP.NET provides web forms controls, which are used to create HTML components. These controls are categories as server-based and client-based.
There are various server controls available for the web forms in ASP.NET:
- Label
- Checkbox
- Text Box
- Radio Button and many more.
Let us discuss all these controls one by one in detail.
Label:
Label control is used to display textual information or any message to the user on the web forms. It is mainly used to create captions for the other controls, such as a textbox. To create a label, you can either write code or use the drag and drop facility of the visual studio.
Syntax:
< asp:LabelID="Label1" runat="server" Text="Label" ></asp:Label>
Properties of Label Control:
- AccessKey: This is used to set keyboard shortcut for the label.
- BackColor: This is used to set the background color of the label.
- BorderColor: This is used to set the border color of the label.
- BorderWidth: This is used to set the width of the border of the label.
- Font: This is used to set the font for the label text.
- ForeColor: This is used to set the color of the label text.
- Text: This is used to set the text to be shown for the label.
- ToolTip: This displays the text when the mouse is over the label.
- Visible: This is used to set visibility of control on the form.
- Height: It is used to set the height of the control.
- Width: This sets the width of the control.
Example:
// WebControls.aspx <%@ Page Language="C#" AutoEventWireup="true" CodeBehind="WebControls.aspx.cs" Inherits="WebFormsControlls.WebControls" %> <!DOCTYPE html> <html xmlns="https://www.w3.org/1999/xhtml"> <head runat="server"> <title></title> <style type="text/css"> .auto-style1 { width: 100%; } .auto-style2 { margin-left: 0px; } .auto-style3 { width: 121px; } </style> </head> <body> <form id="form1" runat="server"> <div> <h4>Provide the Following Details:</h4> <table class="auto-style1"> <tr> <td class="auto-style3"> <asp:Label ID="lblName" runat="server" Text="User Name"></asp:Label></td> <td> <asp:TextBox ID="txtName" runat="server" CssClass="auto-style2"></asp:TextBox></td> </tr> <tr> <td class="auto-style3"> <asp:Label ID="lblFile" runat="server" Text="Upload a File"></asp:Label></td> <td> <asp:FileUpload ID="fileUploadId" runat="server" /></td> </tr> </table> </div> </form> </body> </html>
The property window of label control looks like:
Output:
Text Box:
It is an input control, which is used to take user input. To create a Text Box you can either write code or use the drag and drop facility web forms controls of visual studio IDE.
Syntax:
< asp:TextBoxID="TextBox1" runat="server" ></asp:TextBox>
Properties of Text Box Control:
- AccessKey: This is used to set keyboard shortcut for the control.
- BackColor: This is used to set background color of the control.
- BorderColor: This is used to set border color of the control.
- BorderWidth: This is used to set width of border of the control.
- Font: This is used to set font for the control text.
- ForeColor: This is used to set color of the control text.
- Text: This is used to set text to be shown for the control.
- ToolTip: This displays the text when mouse is over the control.
- Visible: This is used to set visibility of control on the form.
- Height: This is used to set height of the control.
- Width: This is used to set width of the control.
- MaxLength: This is used to set maximum number of characters that can be entered.
- Readonly: This is used to make control readonly.
Example
// WebControls.aspx <%@ Page Language="C#" AutoEventWireup="true" CodeBehind="WebControls.aspx.cs" Inherits="WebFormsControlls.WebControls" %> <!DOCTYPE html> <html xmlns="https://www.w3.org/1999/xhtml"> <head runat="server"> <title></title> </head> <body> <form id="form1" runat="server"> <div> <asp:Label ID="lblName" runat="server">User Name</asp:Label> <asp:TextBox ID="txtName" runat="server" ToolTip="Enter User Name"></asp:TextBox> </div> <p> <asp:Button ID="btnSubmit" runat="server" Text="Submit" OnClick="SubmitButton_Click" /> </p> <br /> </form> <asp:Label ID="lblInput" runat="server"></asp:Label> </body> </html>
Code Behind
// WebControls.aspx.cs
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; namespace WebFormsControlls { public partial class WebControls : System.Web.UI.Page { protected void SubmitButton_Click(object sender, EventArgs e) { lblInput.Text = lblName.Text; } } }
The property window of text box control looks like:
Output:
It displays the user input when the user submits the input to the server.
List Box: It allows the selection of single as well as multiple items in the list, unlike the dropdown control, which allows the selection of single item at a time.
Syntax:
<asp: ListBox id="ListBox1" Rows="6" Width="100px" SelectionMode="Single" runat="server"> </asp: ListBox>
Properties of List Box Control:
- Items.Count: It is used to return the total number of items in the list box.
- Items.Clear: It will clear all the selected items from the list box.
- SelectedItem.Text: It will return the text of the selected item.
- Items.Remove(name): It removes the item with text name.
- Items.RemoveAt(int index): It removes the item present at the given index.
- Items.Insert(int index, text): It inserts the text at the given index.
- SelectedItem: It returns the index of the selected item.
- SelectionMode: It specifies the selection mode single or multiple.
Example:
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="WebForm1.aspx.cs" Inherits="WebApplication1.WebForm1" %> <!DOCTYPE html> <html xmlns="https://www.w3.org/1999/xhtml"> <head runat="server"> <title> An example of ASP.Net ListBox</title> </head> <body> <form id="submitColor" runat="server"> <div> <h2>Choose a color:</h2> <asp: ListBox ID ='lstColor’ runat = 'server' AutoPostBack = 'true' Font-Size = 'X-Large' Rows = '5' ForeColors = 'Tomato' Width = '350' > <asp: ListItem> Dark Grey </asp: ListItem> <asp: ListItem> Red </asp: ListItem> <asp: ListItem> Green </asp: ListItem> <asp: ListItem> Blue </asp:ListItem> <asp: ListItem> Yellow </asp: ListItem> <asp: ListItem> Black </asp: ListItem> </asp: ListBox> </div> </form> </body> </html>
Output:
Radio Button: Radio Button control is an input control that is used to takes input from the user. It allows the user to select a choice from the group of choices.
Syntax:
< asp:RadioButtonID="RadioButton1" runat="server" Text="Male" GroupName="gender"/>
Properties of Radio Button Control:
- AccessKey: This is used to set keyboard shortcut for the control.
- BackColor: This is used to set the background color of the control.
- BorderColor: This is used to set the border color of the control.
- BorderWidth: This is used to set the width of the border of the control.
- Font: This is used to set the font for the control text.
- ForeColor: This is used to set the color of the control text.
- Text: This is used to set the text to be shown for the control.
- ToolTip: This displays the text when the mouse is over the control.
- Visible: This is used to set visibility of control on the form.
- Height: It is used to set the height of the control.
- Width: This sets the width of the control.
- GroupName: This is used to set the name of the radio button group.
Example:
// WebControls.aspx <%@ Page Language="C#" AutoEventWireup="true" CodeBehind="WebControls.aspx.cs" Inherits="WebFormsControlls.WebControls" %> <!DOCTYPE html> <html xmlns="https://www.w3.org/1999/xhtml"> <head runat="server"> <title></title> </head> <body> <form id="submitGender" runat="server"> <div> <asp:RadioButton ID="rdMale" runat="server" Text="Male" GroupName="gender" /> <asp:RadioButton ID="rdFemale" runat="server" Text="Female" GroupName="gender" /> </div> <p> <asp:Button ID="btnClick" runat="server" Text="Submit" OnClick="Button1_Click" style="width: 61px" /> </p> </form> <asp:Label runat="server" id="genderId"></asp:Label> </body> </html>
Code Behind
// WebControls.aspx.cs using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; namespace WebFormsControlls { public partial class WebControls : System.Web.UI.Page { protected void Button1_Click(object sender, EventArgs e) { genderId.Text = ""; if (rdMale.Checked) { genderId.Text = "Your gender is "+rdMale.Text; } else genderId.Text = "Your gender is "+rdFemale.Text; } } }
The property window of radio button control looks like:
Output:
When the user selects the gender.
Checkbox: Checkbox control is used to get multiple inputs from the user. It also allows the user to select choices from the set of choices. It takes the user input in yes or no format and is useful when we want multiple choices from the user.
Syntax:
< asp:CheckBox ID="CheckBox2" runat="server" Text="J2EE"/>
Properties of Checkbox Control:
- AccessKey: This is used to set keyboard shortcut for web forms controls
- BackColor: This is used to set the background color of the control.
- BorderColor: This is used to set the border color of the control.
- BorderWidth: This is used to set the width of the border of the control.
- Font: This is used to set the font for the control text.
- ForeColor: This is used to set the color of the control text.
- Text: This is used to set the text to be shown for the control.
- ToolTip: This displays the text when the mouse is over the control.
- Visible: This is used to set visibility of control on the form.
- Height: It is used to set the height of the control.
- Width: This sets the width of the control.
- Checked: This is used to set check state of the control, either true or false.
Example:
// WebControls.aspx <%@ Page Language="C#" AutoEventWireup="true" CodeBehind="WebControls.aspx.cs" Inherits="WebFormsControlls.WebControls" %> <!DOCTYPE html> <html xmlns="https://www.w3.org/1999/xhtml"> <head runat="server"> <title></title> </head> <body> <form id="submitCourse" runat="server"> <div> <h2>Select Courses</h2> <asp:CheckBox ID="chkJ2SE" runat="server" Text="J2SE" /> <asp:CheckBox ID="chkJ2EE" runat="server" Text="J2EE" /> <asp:CheckBox ID="chkSpring" runat="server" Text="Spring" /> </div> <p> <asp:Button ID="btnClick" runat="server" Text="Button" OnClick="Button1_Click" /> </p> </form> <p> Courses Selected: <asp:Label runat="server" ID="ShowCourses"></asp:Label> </p> </body> </html>
Code Behind
// WebControls.aspx.cs using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; namespace WebFormsControlls { public partial class WebControls : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { ShowCourses.Text = "None"; } protected void Button1_Click(object sender, EventArgs e) { var message = "" ; if (chkJ2SE.Checked) { message = chkJ2SE.Text+" "; } if (chkJ2EE.Checked) { message += chkJ2EE.Text + " "; } if (chkSpring.Checked) { message += chkSpring.Text; } ShowCourses.Text = message; } } }
Output:
After Selection
Button: Button control is used to perform events and is also used to submit client requests to the server.
Syntax:
< asp:ButtonID="Button1" runat="server" Text="Submit" BorderStyle="Solid" ToolTip="Submit"/>
Properties of Button Control:
- AccessKey: This is used to set keyboard shortcut for the control.
- BackColor: This is used to set the background color of the control.
- BorderColor: This is used to set the border color of the control.
- BorderWidth: This is used to set the width of the border of the control.
- Font: This is used to set the font for the control text.
- ForeColor: This is used to set the color of the control text.
- Text: This is used to set the text to be shown for the control.
- ToolTip: This displays the text when the mouse is over the control.
- Visible: This is used to set visibility of control on the form.
- Height: It is used to set the height of the control.
- Width: This sets the width of the control.
Example:
// WebControls.aspx <%@ Page Language="C#" AutoEventWireup="true" CodeBehind="WebControls.aspx.cs" Inherits="WebFormsControlls.WebControls" %> <!DOCTYPE html> <html xmlns="https://www.w3.org/1999/xhtml"> <head runat="server"> <title></title> </head> <body> <form id="submitButton" runat="server"> <div> <asp:Button ID="btnClick" runat="server" Text="Click here" OnClick="Button1_Click" /> </div> </form> <br /> <asp:Label ID="lblClick" runat="server"></asp:Label> </body> </html>
Code Behind
// WebControls.aspx.cs using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; namespace WebFormsControlls { public partial class WebControls : System.Web.UI.Page { protected void Button1_Click(object sender, EventArgs e) { lblClick.Text = "You Clicked The Button."; } } }
Output:
After clicking on the button
Event Handler in ASP.NET: ASP.NET provides an important feature for event handling to WebForms. While working with a web form, you can add events to controls. An event is something, which happens when an action is performed. The most common event is the clicking of a button on a form.
Example: When you enter the first value and the second value and click on the button Sum, then an event will happen, which will add the values and populate it in the text box Sum.
// EventHandling.aspx <%@ Page Language="C#" AutoEventWireup="true" CodeBehind="EnventHandling.aspx.cs" Inherits="asp.netexample.EnventHandling" %> <!DOCTYPE html> <html xmlns="https://www.w3.org/1999/xhtml"> <head runat="server"> <title></title> <style type="text/css"> .auto-style1 { width: 100%; } .auto-style2 { width: 108px; } </style> </head> <body> <form id="submitSum" runat="server"> <div> <table class="auto-style1"> <tr> <td class="auto-style2">First value</td> <td> <asp:TextBox ID="firstvalue" runat="server"></asp:TextBox> </td> </tr> <tr> <td class="auto-style2">Second value</td> <td> <asp:TextBox ID="secondvalue" runat="server"></asp:TextBox> </td> </tr> <tr> <td class="auto-style2">Sum</td> <td> <asp:TextBox ID="total" runat="server"></asp:TextBox> </td> </tr> <tr> <td class="auto-style2"> </td> <td> <br/> <asp:Button ID="Button1" runat="server" OnClick="Button1_Click"Text="Sum"/> </td> </tr> </table> </div> </form> </body> </html>
Code Behind
// EventHandling.aspx.cs using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; namespace asp.netexample { public partial class EnventHandling : System.Web.UI.Page { protected void Button1_Click(object sender, EventArgs e) { int a = Convert.ToInt32(firstvalue.Text); int b = Convert.ToInt32(secondvalue.Text); total.Text = (a + b).ToString(); } } }
Output:
One Comment