Selenium WebDriver’s interaction with HTTP cookies adds significant flexibility to web automation testing by enabling the manipulation of user-specific data stored on the client side. Cookies serve as identifiers containing information about a user’s preferences, browsing session, and often authentication details. Stored in a key-value pair format, these cookies provide a seamless way to access, add, modify, or delete specific pieces of data relevant to a user’s session on a website.
This functionality proves invaluable when running automated tests that require a particular state or context, such as tests that need to simulate a returning user’s experience, carry session-specific preferences, or maintain login credentials across multiple pages.
With Selenium WebDriver, testers can not only view cookies associated with a browser session but can also create custom cookies, update values, and clear cookies as needed to reset the session state. This capability ensures that test scripts are not limited to generic use cases but can be customized to simulate a variety of user interactions, maintaining continuity across page navigations.
For instance, a tester might add a cookie to bypass a login page or simulate a user returning to a specific section of a website. Such stateful testing allows for a more comprehensive assessment of a website’s behavior under different user conditions, making it possible to validate personalized elements, conditional content, or session-related functionalities.
By enabling precise cookie management, Selenium WebDriver empowers testers to conduct in-depth testing and ensures that applications behave consistently across various scenarios that mimic real-world user journeys.
What are an HTTP Cookie and its working?
HTTP cookies, also known as browser cookies, web cookies, or Internet cookies, are small text files stored in the web browser of the client machine, containing valuable information about a user’s activities and preferences. These cookies play a crucial role in customizing the user experience, as they store data such as login details, user settings, and session identifiers on the client side. When a user revisits a website, the browser automatically sends these cookies back to the server, allowing the server to recognize the user and tailor the content based on their previous interactions.
In Selenium software testing, the ability to handle and manipulate cookies is essential for replicating real user scenarios. By accessing and controlling these cookies, testers can simulate various states, track user journeys, and ensure that web applications respond accurately to returning users or specific session settings, enhancing the reliability and thoroughness of test cases.
Need for Handling Cookies in Automation
During automation, we need to handle cookies for the following reasons-
- There will be scenarios that are specific to cookies which are needed to be automated and requires entries in cookie files
- During automation, we can create and load cookie files, in such situations, we can skip some scenarios for each test case like login to the application as the same gets Handling cookies. For example in an application like Gmail, we need to login only once, after that the login information gets stored in cookies and each time we open the Gmail link the login details get loaded. This saves the test execution time.
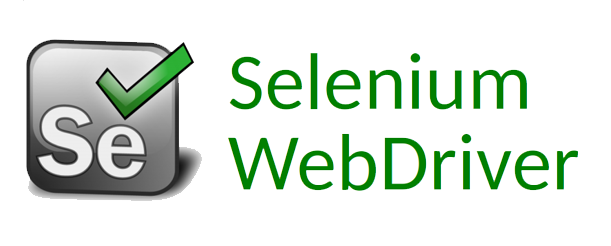
Selenium WebDriver Query Commands For Cookies
With Selenium WebDriver’s built-in methods, interacting with browser cookies becomes straightforward and efficient, allowing testers to add, retrieve, and delete cookies during test sessions. Using methods such as addCookie()
and deleteCookieNamed()
, specific cookies can be manipulated by simply referencing their names.
This enables testers to control individual cookies precisely, making it possible to establish or reset particular states within a session without impacting others.
For instance, by using the addCookie()
method, testers can programmatically add a cookie to simulate a logged-in state, while deleteCookieNamed()
can remove a specific cookie to emulate a session timeout or logout.
These capabilities are instrumental in selenium software testing for managing test cases that depend on stateful interactions, ensuring a more tailored and accurate testing environment that mimics real-world user behaviors.
Get Cookies: This statement is used to get all the cookies for the current domains that are stored in the web browser.
Method Name: getCookies()
Syntax: driver.manage().getCookies();
Returns: It returns a set of cookies for the current domain.
Get the Cookie by Specific Name: This statement is used to get the specific cookie with a given name.
Method Name: getCookieNamed(java.lang.String name)
Syntax: driver.manage().getCookieNamed(arg0);
Parameters: name – It specifies the name of the cookie
This statement will return the specific cookie value for the name specified, or null if no cookie is found with the given name.
Add Cookie: This statement is used to add and create the cookie into cookies.
Method Name: addCookie(Cookie cookie)
Syntax:driver.manage().addCookie(arg0);
Parameters: cookie – It specifies the name of the cookie to add.
Example: Adding a Cookie
public void addCookie() { System.setProperty("webdriver.chrome.driver", "F:\\drivers\\chromedriver.exe"); //create chrome instance driver = new ChromeDriver(); String URL="http://flipkart.com/"; driver.navigate().to(URL); //For cookie we should pass name and value as parameters // Here we pass, name=cookie and value=987654321 Cookie cname = new Cookie("cookie", "987654321"); driver.manage().addCookie(cname); // After adding the cookie we will check all the cookies by displaying them. Set<Cookie> cookiesList = driver.manage().getCookies(); for(Cookie getcookies :cookiesList) { System.out.println(getcookies ); } }
Delete Cookie: This statement is used to delete a cookie from the browsers “cookie jar”.
Method Name: deleteCookie(Cookie cookie)
Syntax: driver.manage().deleteCookie(arg0);
Parameter: Cookie
Delete All Cookies: This statement will delete all the cookies for the current domain.
Method Name: deleteAllCookies()
Syntax: driver.manage().deleteAllCookies();
Parameters: N/A
Delete Cookie with Name: This statement is used to delete a named cookie from the current domain.
Method Name: deleteCookieNamed(java.lang.String name)
Syntax: driver.manage().deleteCookieNamed(arg0);
Parameters: name – The name of the cookie to delete according to its name.Example: Consider the below example for deleting the specific cookie with cookie name as “–utmb”
public void deleteSpecificCookieName() { System.setProperty("webdriver.chrome.driver", "F:\\drivers\\chromedriver.exe"); //create chrome instance driver = new ChromeDriver(); String URL="http://www.flipkart.com"; driver.navigate().to(URL); driver.manage().deleteCookieNamed("__utmb"); }
Deleting all the cookies of the domain
public void deleteAllCookies() { System.setProperty("webdriver.chrome.driver", "F:\\drivers\\chromedriver.exe"); //create a chrome instance driver = new ChromeDriver(); String URL="http://www.flipkart.com"; driver.navigate().to(URL); driver.manage().deleteAllCookies(); }
Demo: Cookie handling in Selenium.
We will use “facebook.com for our demo purpose.
Step 1: Login into the application and store the authentication cookie.
Step 2: Use the stored cookie to login into the application without using a username and password.
Step 1: Storing cookie information.
Here, we load a website for storing the username into cookies. Using Selenium Webdriver, we will load the below website and enter the username, password, and click on the submit button.
package CookieExample; import java.io.BufferedWriter; import java.io.File; import java.io.FileWriter; import java.util.Set; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.Cookie; public class StoreCookie{ public static void main(String[] args) { WebDriver driver; System.setProperty("webdriver.chrome.driver", "F:\\drivers\\chromedriver.exe"); //create a chrome instance driver = new ChromeDriver(); driver.get("http://www.facebook.com/"); // If you are already registered just input the Email id and Password driver.findElement(By.name("username")).sendKeys("abc123"); driver.findElement(By.name("password")).sendKeys("123xyz"); driver.findElement(By.name("submit")).click(); // To store the Login Information create file named Cookies File file = new File("Cookie.data"); try { // Delete if old file exists file.delete(); file.createNewFile(); FileWriter fileWrite = new FileWriter(file); BufferedWriter Bwrite = new BufferedWriter(fileWrite); for(Cookie ck : driver.manage().getCookies()) String writeup = cook.getName()+";"+cook.getValue()+";"+cook.getDomain()+";"+cook.getPath()+"" + ";"+cook.getExpiry()+";"+cook.isSecure(); bufferwrite.write(writeup); System.out.println(writeup); bufferwrite.newLine(); } bufferwrite.flush();bufferwrite.close();fileWriter.close(); }catch(Exception exp){ exp.printStackTrace(); } } }
Output
When you run the above test it displays the encrypted cookie details stored in a web browser in the console.
Step 2: Using the stored cookie in step 1 to login to the application.
Below test script will retrieve the details from the cookies which is stored in a file ‘Cookie.data’. Here, we retrieve the tokens with the help of a semicolon as a separator. After this, we create a Cookie in the Chrome browser, and with the help of ‘addCookie’, we add these details to the cookie.
package CookieExample; import java.io.BufferedReader; import java.io.File; import java.io.FileReader; import java.util.Date; import java.util.StringTokenizer; import org.openqa.selenium.Cookie; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; public class CookieRetriev { WebDriver driver; public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "F:\\drivers\\chromedriver.exe"); //create chrome instance driver = new ChromeDriver(); try{ File file = new File("Cookie.data"); FileReader fileReader = new FileReader(file); BufferedReader Buffreader = new BufferedReader(fileReader); String strline; while((strline=Buffreader.readLine())!=null){ StringTokenizer token = new StringTokenizer(strline,";"); while(token.hasMoreTokens()){ String name = token.nextToken();String value = token.nextToken(); String domain = token.nextToken();String path = token.nextToken(); Date expiry = null; String val; if(!(val=token.nextToken()).equals("null")){ expiry = new Date(val); } Boolean isSecure = new Boolean(token.nextToken()).booleanValue(); Cookie ck = new Cookie(name,value,domain,path,expiry,isSecure); driver.manage().addCookie(ck); } } }catch(Exception ex){ ex.printStackTrace(); } driver.get("http://www.facebook.com/"); } }
Output:
When you execute the above code you will directly take to the login success screen without entering the user name and password.
Conclusion
In conclusion, Selenium WebDriver offers significant advantages for testing efficiency by allowing automation scripts to handle authentication seamlessly through cookie management. By storing and reusing session cookies, testers can bypass repetitive login steps, thus eliminating the need to enter usernames and passwords with every test run.
This not only streamlines the testing process but also reduces the chance of errors associated with manual entry, making the automation workflow smoother and faster.
Moreover, the ability to manage cookies in Selenium WebDriver facilitates stateful testing scenarios, where maintaining a specific user session or context across multiple tests is essential. This feature is particularly useful for testing applications that rely heavily on user authentication and personalization.
By leveraging Selenium WebDriver’s cookie-handling methods, testers can replicate real-world user conditions more accurately, achieving comprehensive test coverage while saving valuable time in regression and end-to-end testing cycles.
Call to Action
Enhance your Selenium WebDriver testing skills with Cookies Handling in Selenium WebDriver at H2K Infosys! Our training offers hands-on experience in managing cookies, enabling you to bypass repetitive login steps, control session data, and create accurate, stateful tests. With expert guidance, you’ll learn to add, retrieve, and delete cookies, making your test scenarios more efficient and realistic.
Join H2K Infosys to unlock the power of effective cookie management in Selenium WebDriver. Perfect for both beginners and advanced testers, this course saves you time and boosts accuracy in your testing workflows. Enroll now to elevate your skills and bring added precision to your automated testing projects!