Create Hello World React App
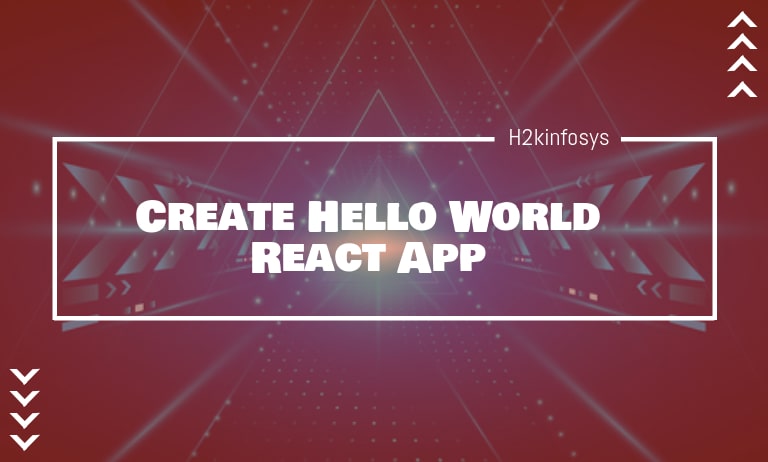
The create-react-app is an excellent tool that allows you to create and run React projects very quickly. This tool is wrapping with all of the required dependencies like Webpack, Babel for React project itself; you need to focus on writing React code only. This tool itself sets up the development environment, provides an excellent developer experience, and optimizes the production app.
The Create React App can work on any platform such as macOS, Windows, Linux, etc. Before creating a React Project using create-react-app, the following things need to be installed in your system.
- Node version >= 8.10
- NPM version >= 5.6
To check the Node version run the following command in the command prompt:
$ node -v
To check the NPM version run the following command in the command prompt:
$ npm -v
To install the Create React app run the below commands, and it will create a directory named react-app:
$ npm install -g create-react-app // or $ yarn global add create-react-app $ create-react-app react-app
After the Create React app finishes its job safely, a brief description of what it has done, and the npm commands that you can use inside the directory will be shown:
The above messages show that the React project is created successfully in our system. Now, you can start the server to access the application on the browser. Type the below command in the terminal window:
$ cd Desktop
$ npm start
NPM is a package manager that starts the server and accesses the application at the default server http://localhost:3000. Now, you will get the following screen.
Let us open /src/App.js to write the code. The file is written in a HTML/XML-like language, which is called JSX. It is always recommended to use JSX when working with React, because it performs faster and describes the UI better than the plain JavaScript. The only drawback is that JSX is not browser-friendly, so it needs to be transpiled with Babel.
import React, { Component } from 'react'; import logo from './logo.svg'; import './App.css'; class App extends Component { render() { return ( <div classname="App"> <div classname="App-header"> <img src="{logo}" classname="App-logo" alt="logo"> <h2>Welcome to React</h2> </div> <p classname="App-intro"> To get started, edit <code>src/App.js</code> and save to reload. </p> </div> ); } }
export default App;
/src/App.js contains a React component named App. A component is an instruction written to tell React what you want it to display on the screen. There are a few different built-in methods that can be used in a component, or you can make your own methods, but the most commonly used method is render. You can use render method to return a React element: a description of how it should look on the screen.
import React, { Component } from 'react'; import './App.css'; class App extends Component { render() { return ( <div classname="container"> <h1>Hello World!!!</h1> </div> ); } } export default App;
The src/index.js file is used for the delivery of App component to the final destination. It imports App. We export it at the end of the App.js file, and displays it into a container in public/index.html, using ReactDOM.
import React from 'react'; import ReactDOM from 'react-dom'; import './index.css'; import App from './App'; import registerServiceWorker from './registerServiceWorker'; ReactDOM.render(<app>, document.getElementById('root')); registerServiceWorker();</app>
ReactDOM.render needs two arguments: a React element and a DOM node to display it. Here, what we need to pass as an element is actually what the App component returns.
If you keep localhost:3000 open in your browser, you might have noticed your screen is already showing you the updated page. However, when your app gets complicated and more significant, what you have right now will not be ideal for deployment.
Running build npm run will create a directory named build and produce optimized builds of the app that are ready to be served with the best performance.
To serve it on your localhost, you can run the below command:
$ npm install -g serve
$ serve -s build // serving contents of the build directory
One Comment