Introduction
Selenium Python has become an essential tool in the world of automation testing. As software systems grow more complex and the demand for faster releases increases, testing processes need to be optimized for both speed and accuracy. Automation testing has become a critical part of the software development process, helping teams save time and reduce human error. Among the various tools available for automation testing, Selenium stands out as one of the most popular choices.
For those looking to deepen their understanding and skills in Selenium, enrolling in a Selenium course online can be a great way to gain hands-on experience, enhance expertise, and ensure efficient automation of web applications. This course provides structured learning on best practices, techniques, and the use of Selenium in real-world testing scenarios, making it an invaluable resource for anyone looking to excel in automation testing.
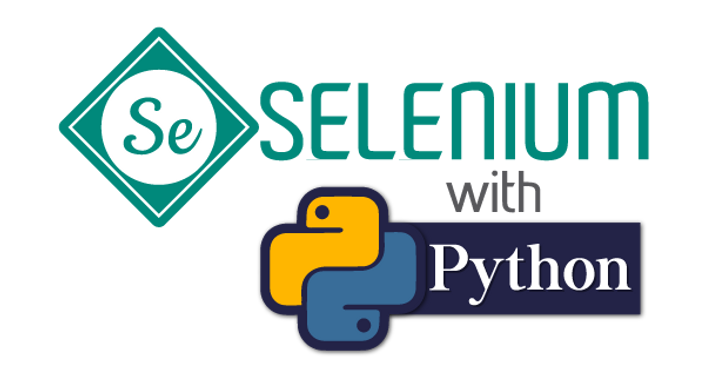
However, while Selenium is powerful, its performance can be significantly impacted by how the tests are written. If you are using Selenium with Python for automation testing, it is essential to optimize your scripts to ensure speed and efficiency, allowing you to execute tests more quickly without sacrificing quality.
In this article, we will explore various techniques and best practices to optimize the performance of your Selenium Python scripts. From choosing the right locators to leveraging parallel execution and optimizing browser settings, we’ll cover strategies to make your tests faster and more efficient.
Why Speed Optimization Matters in Selenium Automation Testing
Speed is crucial in automation testing, especially when integrated into continuous integration/continuous deployment (CI/CD) pipelines. The faster the tests are executed, the faster developers get feedback, enabling quicker fixes for any issues found.
Here are some reasons why optimizing Selenium Python scripts is important:
- Faster Feedback Loops: Developers can receive quicker feedback on issues, leading to faster bug fixes.
- More Efficient CI/CD Pipelines: Faster test execution ensures that the testing phase doesn’t become a bottleneck in your CI/CD process.
- Reduced Testing Costs: With shorter test execution times, you can save on resources and infrastructure costs.
- Faster Release Timelines: Optimized tests contribute to more efficient workflows and allow software to be released on time.
As per Capgemini’s report, 50% of software companies aim to improve test automation to meet release deadlines, highlighting the importance of optimizing test performance.
Common Reasons for Slow Selenium Python Scripts
Before diving into solutions, it’s important to understand the common causes of slow test execution in Selenium Python. Identifying the reasons can help you take the necessary steps to optimize your tests:
- Unoptimized Locators: Using inefficient locators, such as generic or overly complex XPath, can slow down the execution of your Selenium scripts.
- Excessive Wait Times: Using hard-coded
sleep
statements or over-relying on implicit waits can unnecessarily delay script execution. - Inefficient Browser Settings: Default browser configurations might not be optimized for testing, leading to slower test execution.
- Lack of Parallel Execution: Running tests sequentially instead of executing them in parallel can waste valuable time.
- Large DOM Size: Interacting with complex or large web pages without optimization can increase the time it takes for Selenium to execute the tests.
Easy Ways to Make Selenium Python Faster
Now that we understand why Selenium scripts can be slow, it’s essential to focus on actionable steps that can significantly boost the performance and efficiency of your Selenium Python scripts. By optimizing your test scripts, you can ensure quicker feedback loops, reduce the amount of time needed for each test cycle, and ultimately improve the productivity of your development and testing teams.
We’ll discuss in detail various best practices that can be adopted to improve the performance of your Selenium scripts, ranging from using efficient locators and reducing unnecessary interactions with the DOM, to leveraging parallel execution and running tests in headless mode. These optimizations are not just about writing code that runs faster; they also ensure that you can handle larger test suites, test across different browsers and platforms, and keep your test execution times within acceptable limits.
By carefully considering these techniques, you will be able to streamline your testing process and ensure a more efficient automation pipeline. Let’s explore each of these optimizations in greater depth to help you fine-tune your Selenium Python scripts and get the most out of your automation efforts.
Use Efficient Locators
One of the most significant factors in speeding up Selenium tests is choosing the right locators. Locators are how Selenium identifies elements on a webpage, and inefficient locators can drastically slow down your scripts.
Best Practices:
- ID Locators: The fastest and most reliable locators. Use IDs whenever possible.
- CSS Selectors: Faster than XPath and more efficient when identifying elements.
- Class Names: Use class names only when IDs are unavailable.
Example:
Inefficient Locator (XPath):
pythonCopyEditelement = driver.find_element_by_xpath("//div[@class='example']/span[1]")
Efficient Locator (CSS Selector):
pythonCopyEditelement = driver.find_element_by_css_selector("div.example > span:first-child")
By choosing more efficient locators like ID
and CSS Selectors
, you can significantly improve the execution speed of your Selenium scripts.
Use Explicit Waits Instead of Implicit Waits or Sleep
Using sleep
statements or implicit waits can unnecessarily delay the execution of your tests. Instead, explicit waits are a better approach as they allow Selenium to wait until a specific condition is met before continuing the script execution.
Best Practices:
- Avoid hard-coded
sleep
statements. - Use Selenium’s
WebDriverWait
class for more efficient waiting.
Example:
pythonCopyEditfrom selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
# Wait for an element to be present before continuing
wait = WebDriverWait(driver, 10)
element = wait.until(EC.presence_of_element_located((By.ID, "example-id")))
Explicit waits ensure that the script only waits for the necessary time, reducing unnecessary delays.
Optimize Browser Settings
By disabling unnecessary browser features, you can reduce the amount of time Selenium spends on each test. For instance, you can disable images, JavaScript, and extensions that are not needed for testing purposes.
Best Practices:
- Disable browser extensions.
- Disable GPU acceleration.
- Run tests in headless mode, which runs the browser without a graphical user interface (GUI) and consumes fewer resources.
Example:
pythonCopyEditfrom selenium.webdriver.chrome.options import Options
options = Options()
options.add_argument("--disable-extensions")
options.add_argument("--disable-gpu")
options.add_argument("--headless") # Run in headless mode
# Disable images
prefs = {"profile.managed_default_content_settings.images": 2}
options.add_experimental_option("prefs", prefs)
driver = webdriver.Chrome(options=options)
By optimizing browser settings, you can make your Selenium tests run faster.
Leverage Parallel Execution
Parallel execution allows you to run multiple tests simultaneously, significantly reducing the total time taken to execute a test suite. Selenium provides tools to run tests across multiple devices and browsers at the same time, helping you scale your testing efforts.
Best Practices:
- Use tools like
pytest-xdist
to run tests in parallel. - Split large test suites into smaller test cases that can be executed in parallel.
Example: Install pytest-xdist
:
bashCopyEditpip install pytest-xdist
Run tests with multiple threads:
bashCopyEditpytest -n 4 # Run tests in parallel with 4 threads
Parallel execution can drastically reduce the total time spent on testing, making it an essential strategy for optimizing Selenium test performance.
Reduce DOM Interaction
Minimizing interactions with the DOM (Document Object Model) can speed up the execution of your tests. Instead of fetching elements multiple times, try to store them in a variable and interact with the stored element.
Example:
Inefficient Code:
pythonCopyEditfor i in range(100):
driver.find_element_by_id("example-id").click()
Optimized Code:
pythonCopyEditelement = driver.find_element_by_id("example-id")
for i in range(100):
element.click()
Reducing redundant interactions with the DOM can make your scripts much more efficient.
Use Headless Browsing
Running tests in headless mode can help speed up execution by using fewer system resources. This is especially useful when you do not need to visualize the browser’s user interface during testing.
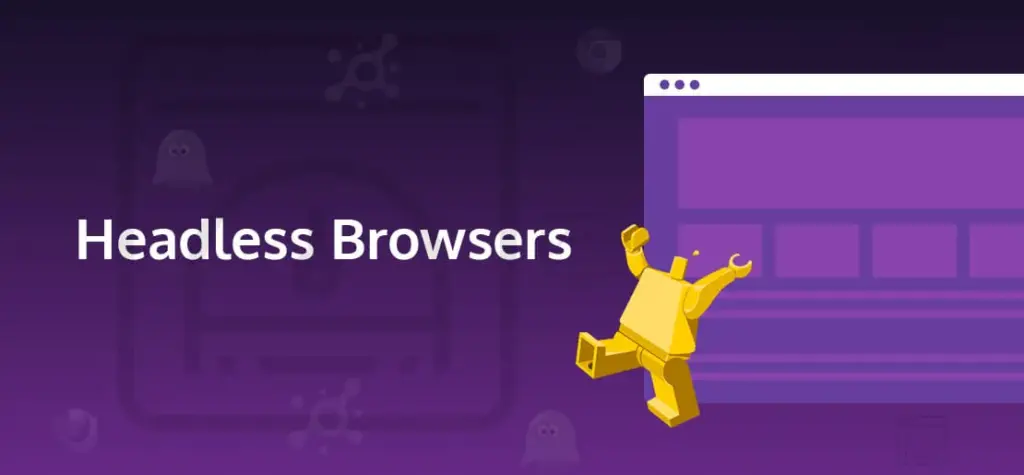
Best Practices:
- Use headless mode for faster tests when the GUI is not required.
Example:
pythonCopyEditoptions = webdriver.ChromeOptions()
options.add_argument('--headless')
options.add_argument('--disable-gpu')
# Initialize WebDriver
driver = webdriver.Chrome(options=options)
Headless browsing is a must for optimizing Selenium testing performance, especially for large test suites.
Optimize Test Data and Environment
Efficient test data management is also critical for speeding up test execution. Ensure your test data is lightweight and avoids unnecessary dependencies on backend systems.
Best Practices:
- Use mock APIs to simulate backend responses.
- Keep your test data sets small and relevant to the test scenario.
Example:
pythonCopyEditfrom unittest.mock import MagicMock
mock_api = MagicMock(return_value={"status": "success"})
response = mock_api()
print(response)
By optimizing your test environment and data, you reduce external factors that can slow down your test execution.
Key Takeaways
- Efficient Locators: Use
ID
orCSS Selectors
for faster element identification. - Explicit Waits: Replace hard-coded waits with
WebDriverWait
for better performance. - Browser Optimization: Disable unnecessary features to speed up test execution.
- Parallel Testing: Run tests in parallel to significantly reduce testing time.
- Headless Mode: Use headless browsing to save system resources.
- Minimized DOM Interaction: Avoid redundant interactions with web elements.
- Mock APIs: Use lightweight test data and mock external dependencies.
By implementing these strategies, you can significantly improve the performance of your Selenium Python scripts, allowing you to complete your tests faster while maintaining high accuracy and efficiency.
Conclusion
Optimizing the speed of your Selenium Python scripts is crucial for efficient automation testing. By following the best practices outlined in this article such as using efficient locators, optimizing browser settings, leveraging parallel execution, and minimizing unnecessary DOM interactions you can drastically improve the performance of your Selenium scripts.
Speed optimization not only leads to faster feedback and reduced costs but also contributes to better resource utilization and improved productivity. By mastering these techniques, you will be able to execute automation tests more quickly, making your testing process more efficient and enabling faster delivery timelines for your projects.
If you want to dive deeper into Selenium and master these optimization techniques, consider enrolling in H2K Infosys’ Selenium certification course. Gain hands-on experience and elevate your career in software testing!