What is Ajax?
Selenium supports Ajax, which stands for Asynchronous JavaScript and XML, so we can say that it is a combination of JavaScript and XML. It is a technique used for creating fast and dynamic web pages. It works with a group of technologies like JavaScript, HTTP requests, XML, HTML, CSS, etc. Ajax will send HTTP requests from the client to the server and receive data asynchronously without reloading the entire web page.
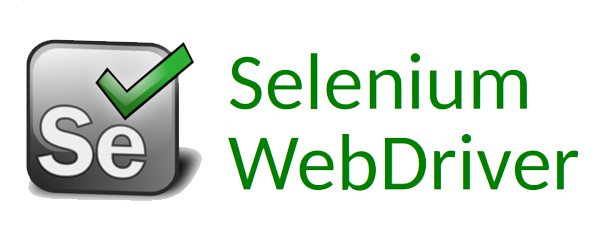
How does Ajax works?
For example, when you click on a submit button, JavaScript will make a request to the server, interpret the result and current screen will get the update without reloading the webpage. The wait command may not work to handle the AJAX controls. Itās just because the actual page is not going to refresh. Sometimes it may load in a second and sometimes take much longer.We have no control over loading time.
The best approach in Selenium software testing is to use dynamic waits, i.e., WebDriver Wait in combination with ExpectedCondition, to handle this kind of situation effectively. This approach ensures that the test scripts are robust and adaptable to varying loading times, improving the efficiency and reliability of automation tests.
Handling Ajax call Using Selenium Webdriver
Selenium sometimes struggles to interact with elements that appear dynamically after an AJAX call. This challenge arises because, in AJAX operations, the content is loaded asynchronously, and the exact wait time for the element to appear can never be guaranteed. Unlike traditional synchronous web applications where the entire page reloads, AJAX-driven pages update specific parts of the DOM without a complete reload.
This unpredictability introduces a layer of complexity, as waiting for a fixed amount of time often fails to capture the exact moment when the element becomes available. Consequently, the test script may proceed prematurely, leading to errors or failures when attempting to interact with the element.
The biggest hurdle in handling AJAX calls lies in accurately determining the webpage’s loading time for dynamic elements. Since AJAX calls depend on server responses and the complexity of the task being executed, they vary significantly in duration. Relying on static wait times, such as Thread.sleep
, is not a reliable solution, as it either results in unnecessary delays or insufficient waiting periods. Instead, using advanced dynamic waiting strategies, such as WebDriverWait
with ExpectedConditions
, provides a smarter and more adaptable approach to handling AJAX calls.
By ensuring the script pauses execution only until the element is actually present or visible, these techniques help overcome the uncertainty of AJAX timing, making your test scripts more robust and efficient.
Since the loaded web page will last only for a few seconds, it is difficult for the tester to test such applications through an automation tool. For this, the Selenium Webdriver has to use the wait command on Ajax Call. So by wait command execution, selenium will suspend the current Test Case execution and wait for the expected value. When the expected value appears, the Selenium Webdriver will execute suspended test cases.
The Following are the wait methods that Selenium Webdriver uses.
- Thread.Sleep(): It is not a current choice to use as it suspends the current thread for the specified amount of time and makes the current thread to be moved from the queue which is running to the waiting queue.
- Implicit Wait(): This method tells the Webdriver to wait for some time if the element is not available immediately, but this will wait for the entire time until the browser is open. So any elements on the web page could take the time the implicit wait is set for.
- Explicit Wait(): It is used to freeze the test execution time till a particular condition is met or maximum time lapses.
- Webdriver Wait(): It can be used for any type of condition. It can be achieved with WebDriverWait in combination with ExpectedCondition.
The problem with all the above waits is that you have to mention the time out of the unit. What happens if the element is still not present within the time? So we have one more wait called Fluent Wait.
- Fluent Wait: This interface has its timeout and polling interval. Each Fluent Wait determines the maximum amount of time we need to wait for a condition, as well as to check the frequency of the condition and also repeat the waiting process as per the defined interval.
Handling of AJAX calls using Implicit wait in Selenium Webdriver.
An implicit wait in Selenium is a mechanism that directs the WebDriver to poll the DOM for a specified amount of time while attempting to locate one or more elements until they become available. This type of wait is particularly useful in scenarios where elements take varying amounts of time to appear due to page load times, network delays, or asynchronous operations like AJAX calls. When an implicit wait is set, the WebDriver continuously checks the DOM for the presence or visibility of the target element during the defined period.
If the element is found within this timeframe, the script proceeds immediately without waiting for the full duration, thus optimizing execution time. Conversely, if the element fails to appear within the set interval, the WebDriver throws a NoSuchElementException
. While implicit waits can handle basic delays effectively, their applicability is limited in more dynamic scenarios involving complex or multi-stage asynchronous processes.
For such cases, explicit waits or fluent waits, which offer greater control and flexibility, are often better suited. However, implicit waits remain a foundational feature in Selenium for improving the resilience and reliability of test scripts by accommodating minor, predictable delays in element loading.
TheĀ default value timeout is 0.
The implicit wait is available for the lifetime of the Webdriver instance once you define it.
System.setProperty("webdriver.gecko.driver","F:\\drivers\\geckodriver.exe");Ā Ā
Ā
WebDriver driver = new FirefoxDriver();
browser.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS);
browser.get("//www.w3schools.com/");
WebElement ajaxControl = browser.findElement(By.id("DummyElement"));
Handling of AJAX calls using Explicit wait in Selenium Webdriver.
It is another built-in feature in Webdriver to handle AJAX calls. Whenever no other tricks work it will work just like Thread.sleep().
System.setProperty("webdriver.gecko.driver","F:\\drivers\\geckodriver.exe");
WebDriver driver = new FirefoxDriver();
browser.get("//www.w3schools.com/");
WebElement ajaxControl = (new WebDriverWait(browser, 15))
.until(ExpectedConditions.presenceOfElementLocated(By
.id("DummyElement")));
Check out more articles on our H2K Infosys blog.
Sample program of Implicit wait:
package SeleniumTester;
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.testng.annotations.Test;
public class AjaxDemoImplicit {
@Test
public void locatorDemo() throws Exception {
System.setProperty("webdriver.gecko.driver","F:\\drivers\\geckodriver.exe");Ā Ā
Ā Ā Ā Ā WebDriver driver = new FirefoxDriver();
//Implicit Wait
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
driver.get("https://www.facebook.com");
driver.close();
driver.quit();
}
}
Sample program of Explicit wait:
package SeleniumTester;
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
import org.testng.annotations.Test;
public class AjaxDemoExplicit {
@Test
public void locatorDemo() throws Exception {
System.setProperty("webdriver.gecko.driver","F:\\drivers\\geckodriver.exe");Ā Ā
Ā Ā Ā Ā WebDriver driver = new FirefoxDriver();
Ā Ā Ā Ā //Implicit Wait
Ā Ā Ā Ā driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
Ā Ā Ā Ā driver.get("https://www.facebook.com");
Ā Ā Ā Ā //Explicit Wait Declaration
Ā Ā Ā Ā WebDriverWait wait = new WebDriverWait(driver, 40);
Ā Ā Ā Ā wait.until(ExpectedConditions.titleContains("Facebook ā log in or sign up"));
Ā Ā Ā Ā System.out.println("Explicit wait result- " +wait);
Ā Ā Ā Ā driver.close();
Ā Ā Ā Ā driver.quit();
Ā Ā Ā Ā }
}
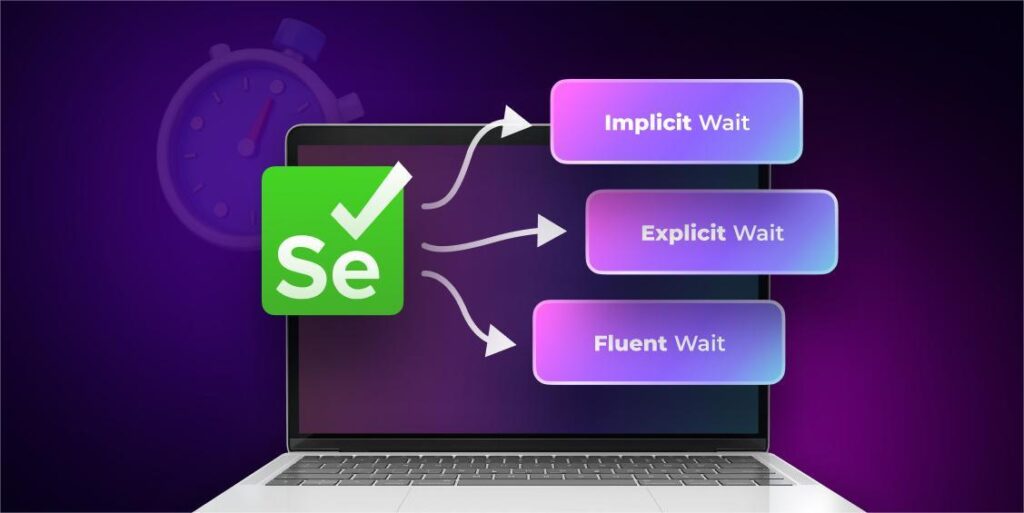
Conclusion
- AJAX allows us to retrieve small amounts of data from the Web page to the server without reloading the entire page.
- To test the Ajax application, we need to apply different wait methods
- ThreadSleep
- Implicit Wait
- Explicit Wait
- WebdriverWait
- Fluent Wait
- For testing tools creating automation test request may be difficult as AJAX application frequently use different encoding or serialization technique to submit the data.
Handling AJAX calls in Selenium WebDriver is a critical skill for creating reliable and efficient automated test scripts. AJAX (Asynchronous JavaScript and XML) allows web pages to update content dynamically without reloading the entire page, which presents unique challenges for automation testing. Since AJAX operations are asynchronous, traditional methods of waiting for page loads are insufficient. To address this, testers must utilize WebDriverās dynamic waiting strategies such as WebDriverWait
combined with ExpectedConditions
.
These methods enable the script to pause execution until specific conditions are met, such as the visibility of an element or the completion of background processes. This approach ensures the test scripts can handle unpredictable loading times caused by AJAX, minimizing the risk of flaky tests and enhancing test reliability.
Additionally, itās essential to incorporate robust strategies, such as validating data states and ensuring that AJAX requests are completed before asserting conditions or proceeding with further test steps. Leveraging JavaScript executors to monitor network activity or dynamically polling the DOM for changes can provide additional control and accuracy when testing AJAX-heavy applications. Mastering these techniques in Selenium WebDriver not only enhances the quality of your test automation suite but also contributes to more thorough and effective testing of dynamic web applications.
As web technologies continue to evolve, being adept at handling AJAX calls will remain a vital aspect of modern test automation.
Call to Action
Mastering the art of handling AJAX calls in Selenium WebDriver is a game-changer for any test automation professional. If youāre ready to elevate your skills and build robust, dynamic test scripts for modern web applications, itās time to invest in quality Selenium training with H2K Infosys. Learn how to implement advanced waiting strategies, use JavaScript executors, and monitor dynamic web elements seamlessly. With hands-on practice and expert guidance from H2K Infosys, you can gain the confidence to tackle complex challenges in web automation. Start your journey today and unlock the potential to become a sought-after Selenium automation expert.
Don’t let the complexities of AJAX handling slow down your testing progress. Join a comprehensive Selenium training program at H2K Infosys to gain the skills needed to handle asynchronous operations with precision. With the increasing demand for Selenium testers in the industry, this expertise can set you apart and fast-track your career in test automation.
Whether youāre a beginner or looking to sharpen your existing skills, H2K Infosys offers the right training program to make all the difference. Take the first step toward becoming a proficient Selenium WebDriver expert and transform your career in software testing today!