In the world of programming, writing clean, efficient, and error-free code is paramount. Python, known for its simplicity and readability, is a popular choice among developers. However, even experienced developers can make mistakes, and that’s where Python syntax checkers come into play. Syntax checkers help identify and correct errors in your code, ensuring it adheres to Python’s syntax rules. This article will explore how to use Python syntax checkers effectively to improve your code quality and avoid common pitfalls.
How to find error in python code
To find errors in Python code, you can use the following methods:
- Syntax Checkers (Linters): Tools like Pylint, Flake8, and Pyright analyze your code for syntax errors, style issues, and potential bugs.
- Debugging: Use the built-in Python debugger (
pdb
) or IDE-integrated debuggers to step through code, inspect variables, and identify errors. - Error Messages: Carefully read Python’s error messages and tracebacks to locate the source of the issue.
- Unit Tests: Write unit tests to validate code functionality and identify logical errors.
- Code Reviews: Peer reviews can help catch errors and improve code quality.
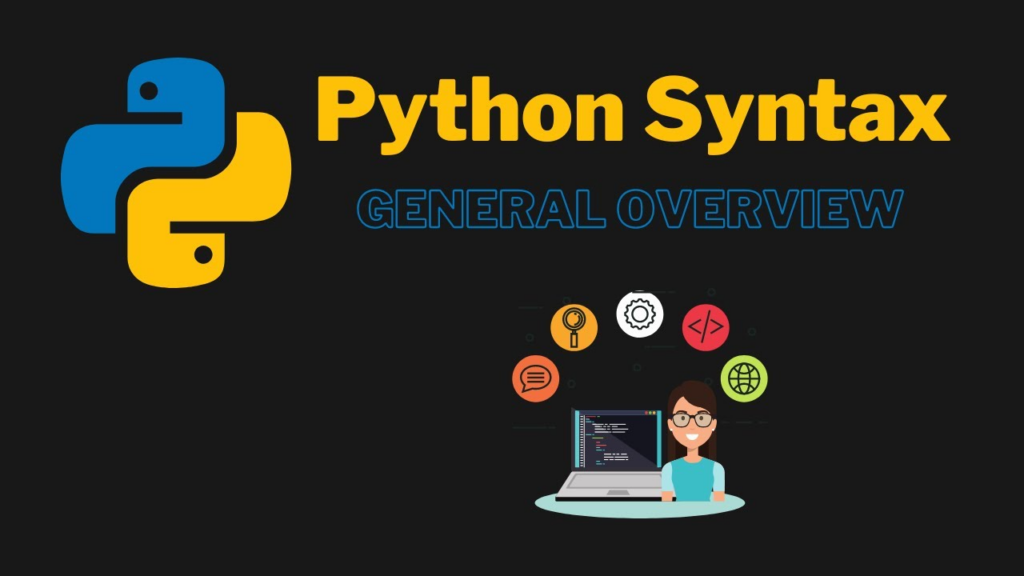
How to fix my code python ?
Review Error Messages: Carefully read Python’s error messages and tracebacks to identify the source of the issue.
Debugging: Use debugging tools like pdb
or your IDE’s debugger to step through the code and inspect variable’s
Syntax Checkers: Run linters like Pylint or Flake8 to catch syntax and style errors.
Refactor Code: Simplify complex code, ensure proper variable names, and adhere to coding standards.
Consult Documentation: Refer to Python documentation and relevant libraries for proper usage.
Testing: Write unit tests to verify your fixes and prevent future issues.
1. Understanding Python Syntax Checkers
Python syntax checkers, also known as linters, are tools that analyze your code for syntax errors, style issues, and potential bugs. They provide real-time feedback, helping developers catch mistakes early in the development process. These tools can be integrated into various development environments, making them accessible and easy to use.
2. Popular Python Syntax Checkers
Several Python syntax checkers are available, each with its unique features and capabilities. Here are some of the most popular ones:
- Pylint: Pylint is a widely used static code analysis tool that checks for errors, enforces coding standards, and suggests code refactoring. It provides detailed reports on your code’s quality and helps you follow best practices.
- Flake8: Flake8 is a lightweight tool that combines several linters, including PyFlakes, pycodestyle, and McCabe. It checks for syntax errors, style violations, and complexity issues, making it a versatile choice for Python Developers.
- Pyright: Pyright is a static type checker and linter for Python, developed by Microsoft. It offers type checking, error detection, and code suggestions, making it ideal for projects that require type annotations.
- Bandit: Bandit is a security-focused linter that scans your code for common security issues. It’s particularly useful for identifying vulnerabilities in Python applications.
3. Setting Up Python Syntax Checkers
To use Python syntax checkers effectively, you need to set them up in your development environment. Here’s a step-by-step guide to getting started with Pylint and Flake8:
3.1. Installing Pylint
- Install Pylint: You can install Pylint using pip, Python’s package manager. Run the following command in your terminal:bashCopy code
pip install pylint
- Running Pylint: To check a Python file, navigate to its directory in the terminal and run:bashCopy code
pylint filename.py
Pylint will analyze the file and provide a report with warnings, errors, and suggestions for improvement. - Configuring Pylint: Pylint can be customized using a configuration file (
.pylintrc
). You can generate a default configuration file using the command:bashCopy codepylint --generate-rcfile > .pylintrc
Modify this file to tailor Pylint’s checks to your project’s requirements.
3.2. Installing Flake8
- Install Flake8: Similar to Pylint, install Flake8 using pip:bashCopy code
pip install flake8
- Running Flake8: To check a file, navigate to its directory and run:bashCopy code
flake8 filename.py
Flake8 will display a list of issues found in the file, including style violations and syntax errors. - Configuring Flake8: You can customize Flake8 using a configuration file (
.flake8
). Create this file in your project’s root directory and specify your preferences.
4. Using Python Syntax Checkers in Integrated Development Environments (IDEs)
Many modern IDEs support Python syntax checkers, providing real-time feedback as you write code. Here’s how to integrate syntax checkers into popular IDEs:
4.1. Visual Studio Code
Visual Studio Code (VS Code) is a popular, lightweight code editor with excellent support for Python. To integrate syntax checkers:
- Install Python Extension: Install the Python extension from the VS Code marketplace.
- Configure Linting: In VS Code, open the Command Palette (Ctrl+Shift+P), search for “Python: Select Linter,” and choose your preferred linter (e.g., Pylint, Flake8).
- Real-Time Feedback: The linter will provide real-time feedback as you write code, highlighting errors and suggestions.
4.2. PyCharm
PyCharm is a powerful IDE for Python development. It has built-in support for various linters and provides a comprehensive development environment.
- Enable Code Inspections: In PyCharm, navigate to
File > Settings > Editor > Inspections
and enable the desired code inspections. - Configure Linter: Under
File > Settings > Tools > External Tools
, you can add Pylint or other linters and configure their settings. - Analyze Code: Use
Code > Inspect Code
to run inspections and view the results.
5. Best Practices for Using Python Syntax Checkers
To maximize the benefits of Python syntax checkers, consider the following best practices:
- Consistent Usage: Regularly run syntax checkers on your codebase to catch errors early and maintain code quality.
- Understand Warnings: Not all warnings require immediate fixes. Understand the context and prioritize critical issues.
- Follow Coding Standards: Adhere to coding standards like PEP 8 to ensure consistency and readability in your code.
- Automate Checks: Integrate syntax checkers into your Continuous Integration (CI) pipeline to automate code quality checks.
- Review Reports: Regularly review the reports generated by syntax checkers and address the issues identified.
6. Common Issues and How to Resolve Them
While using Python syntax checkers, you may encounter common issues. Here are a few examples and how to resolve them:
- False Positives: Sometimes, syntax checkers may flag valid code as an issue. Review the warning and suppress it if necessary.
- Performance Impact: Running syntax checkers on large codebases can be time-consuming. Use caching or configure checks to run selectively.
- Configuration Conflicts: Different projects may have varying coding standards. Ensure your linter configuration aligns with the project’s requirements.
Conclusion
Python syntax checkers are invaluable tools for improving code quality and ensuring adherence to best practices. By using these tools consistently and understanding their feedback, you can catch errors early, write cleaner code, and maintain high standards in your projects. Whether you’re a seasoned developer or just starting with Python, integrating syntax checkers into your workflow is a step toward becoming a more efficient and effective programmer. If you’re looking to deepen your understanding of Python, Python Online Training with Certification from H2K Infosys can help you master the language and best practices, giving you the skills you need to take your programming to the next level.