Introduction
In the world of software development, XML (eXtensible Markup Language) plays a vital role in data exchange between applications, especially in large-scale systems. However, ensuring the correctness and structure of an XML document is equally crucial to maintain system integrity. This is where XML validation using XSD (XML Schema Definition) or DTD (Document Type Definition) comes into play. Understanding how to validate XML documents is a fundamental skill for aspiring developers, particularly those pursuing a Java Full Stack Developer Training program.
This blog will guide you step-by-step on how to validate XML documents using XSD and DTD, complete with practical examples and industry applications.
Introduction to XML Validation
XML serves as a widely accepted standard for storing and transferring data across different systems and applications due to its flexibility and platform independence. However, raw XML documents often encounter issues such as errors in syntax, missing mandatory fields, mismatched tags, or an overall incorrect structure.
These inconsistencies can lead to unexpected behavior, compatibility issues, and data loss when XML documents are processed by various systems. To address these challenges and ensure data reliability, validation mechanisms like XML Schema Definition (XSD) and Document Type Definition (DTD) are employed. These tools provide a structured framework that defines the rules and constraints an XML document must follow, such as permissible elements, attributes, data types, and their hierarchical relationships.
By validating XML documents against XSD or DTD, developers can guarantee data integrity, enhance interoperability between systems, and reduce the likelihood of errors during data exchange or processing. This ensures that XML remains a dependable choice for data representation in a wide array of applications.
For Java developers, validating XML is essential when working on backend systems, APIs, or configuration files in Full Stack Java Developer Training. The process ensures seamless data communication between systems.
What is XSD and DTD?
XSD (XML Schema Definition):
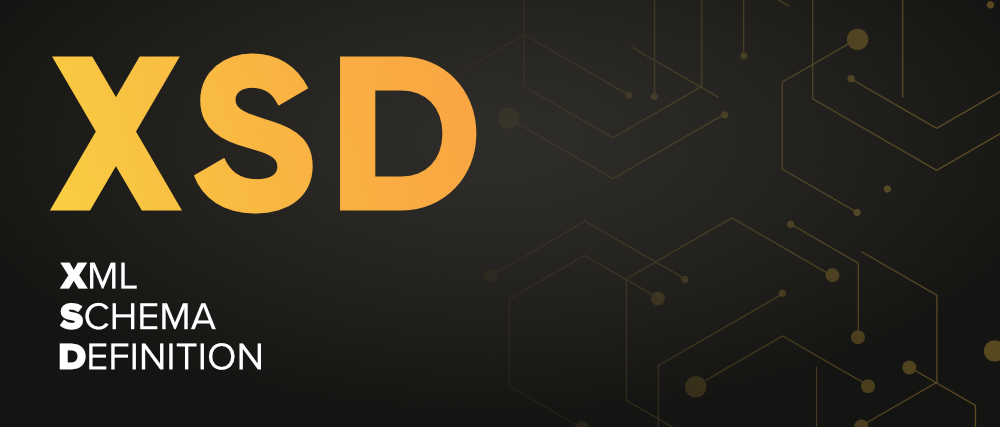
XSD (XML Schema Definition) is a highly versatile and powerful tool for defining the structure, content, and data types within an XML document. Unlike DTD, which has more limited functionality, XSD provides advanced capabilities to handle modern, complex data requirements. One of its key advantages is that it uses XML syntax itself, making it inherently extensible, readable, and compatible with existing XML tools and parsers.
This allows developers to specify detailed rules for elements and attributes, including data types, default values, and constraints like minimum and maximum occurrences. With XSD, you can also define namespaces to avoid naming conflicts in large or collaborative projects, ensuring seamless integration across different systems.
DTD (Document Type Definition):
DTD (Document Type Definition) is one of the earliest standards for defining the legal structure and elements of an XML document. It serves as a blueprint that outlines which elements, attributes, and relationships are permissible within a given XML file. Its simplicity is both a strength and a limitation. On one hand, DTD is straightforward to use and well-suited for basic document validation, making it an accessible choice for smaller or less complex XML files.
On the other hand, DTD lacks the sophistication required to handle the diverse and intricate data structures of modern applications. It does not support advanced data types, such as integers, dates, or booleans, which limits its utility in scenarios requiring strict data validation. Additionally, DTD is not written in XML itself, which makes it less extensible and more challenging to integrate with newer XML tools and technologies.
Key Differences Between XSD and DTD
No. | DTD | XSD |
1 | DTD refers to Document Type Definition. | XSD refers to XML Schema Definition. |
2 | These are derived from SGML syntax. | These are written in XML. |
3 | It does not support datatypes. | It supports datatypes. |
4 | It does not support namespaces. | It supports namespaces. |
5 | It does not define the order of child elements. | It defines the order of child elements. |
6 | It is not extensible. | It is extensible. |
7 | It is not simple and easy to learn. | It is simple and easy to learn. |
8 | It provides less control over the XML structure. | It provides more control over the XML structure. |
Why XML Validation Matters?
XML validation plays a crucial role in ensuring the reliability, consistency, and security of systems that rely on XML for data storage, transfer, or configuration. Whether you’re working with APIs, web services, or data exchange between applications, validating XML documents is essential to ensure they adhere to predefined rules and structures. Let’s delve deeper into why this is so critical:
Ensures Data Integrity
XML validation guarantees that the data contained within an XML document conforms to the structure and constraints defined in its schema (XSD) or DTD. This is particularly important when XML documents are exchanged between systems with different architectures, as validation ensures that the data remains accurate and reliable throughout the transfer process. For example, validating a financial transaction XML ensures that fields like account numbers, amounts, and dates are correctly formatted and populated.
Reduces Errors and Debugging Time
Errors in XML documents can cause applications to crash or produce incorrect results, which can be costly in production environments. Validation helps detect and prevent such issues early, reducing debugging time. For developers, this means fewer runtime surprises and smoother development workflows.
Maintains System Compatibility
Modern systems are often interconnected, relying on XML as a universal language for communication. Validation ensures that XML documents conform to shared standards, maintaining compatibility between different systems, services, and software components. This is particularly important in large-scale, enterprise-level Java projects where diverse technologies need to work seamlessly together.
Enhances Data Security
Malicious or malformed XML documents can pose security risks, such as injection attacks or system vulnerabilities. By validating XML, you can prevent the processing of documents that do not meet structural and content expectations, safeguarding your applications and data pipelines.
Facilitates Collaboration and Teamwork
In collaborative development environments, validation ensures that all team members and systems follow a consistent set of rules. This prevents misunderstandings or errors caused by inconsistent data formats, making it easier for teams to work together on large projects.
In a Java Full Stack Developer Training curriculum, learning to validate XML documents prepares developers for real-world challenges, such as working with APIs, databases, and configuration files.
Validating XML with XSD
Example XML Document (employees.xml):
xml
Copy code
<?xml version="1.0" encoding="UTF-8"?>
<employees xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="employees.xsd">
<employee>
<name>John Doe</name>
<age>30</age>
<position>Software Engineer</position>
</employee>
</employees>
Example XSD File (employees.xsd):
xml
Copy code
<?xml version="1.0" encoding="UTF-8"?>
<xs:schema xmlns:xs="http://www.w3.org/2001/XMLSchema">
<xs:element name="employees">
<xs:complexType>
<xs:sequence>
<xs:element name="employee" maxOccurs="unbounded">
<xs:complexType>
<xs:sequence>
<xs:element name="name" type="xs:string"/>
<xs:element name="age" type="xs:integer"/>
<xs:element name="position" type="xs:string"/>
</xs:sequence>
</xs:complexType>
</xs:element>
</xs:sequence>
</xs:complexType>
</xs:element>
</xs:schema>
Java Code for Validation:
java
Copy code
import java.io.File;
import javax.xml.XMLConstants;
import javax.xml.validation.Schema;
import javax.xml.validation.SchemaFactory;
import javax.xml.validation.Validator;
import org.xml.sax.SAXException;
public class XMLValidation {
public static void main(String[] args) {
try {
File xmlFile = new File("employees.xml");
File xsdFile = new File("employees.xsd");
SchemaFactory factory = SchemaFactory.newInstance(XMLConstants.W3C_XML_SCHEMA_NS_URI);
Schema schema = factory.newSchema(xsdFile);
Validator validator = schema.newValidator();
validator.validate(new javax.xml.transform.stream.StreamSource(xmlFile));
System.out.println("XML is valid!");
} catch (Exception e) {
System.out.println("XML is not valid: " + e.getMessage());
}
}
}
Explanation:
- SchemaFactory loads the XSD file.
- Validator checks the XML file against the schema.
- Errors are caught and displayed if the XML is invalid.
Validating XML with DTD
Example XML Document (products.xml):
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE products SYSTEM "products.dtd">
<products>
<product>
<name>Phone</name>
<price>699</price>
</product>
</products>
Example DTD File (products.dtd):
<!ELEMENT products (product+)>
<!ELEMENT product (name, price)>
<!ELEMENT name (#PCDATA)>
<!ELEMENT price (#PCDATA)>
Java Code for Validation:
import org.xml.sax.SAXException;
import javax.xml.parsers.SAXParser;
import javax.xml.parsers.SAXParserFactory;
import java.io.File;
public class DTDValidation {
public static void main(String[] args) {
try {
SAXParserFactory factory = SAXParserFactory.newInstance();
factory.setValidating(true); // Enable DTD validation
SAXParser parser = factory.newSAXParser();
parser.parse(new File("products.xml"), new org.xml.sax.helpers.DefaultHandler());
System.out.println("XML is valid with DTD!");
} catch (SAXException e) {
System.out.println("XML is not valid: " + e.getMessage());
} catch (Exception e) {
e.printStackTrace();
}
}
}
Explanation:
- The SAX parser is configured to validate using the DTD.
- The parser checks the XML structure against the DTD rules.
Common Errors in XML Validation
- Missing Schema Declaration: Forgetting to include the XSD or DTD declaration in the XML file.
- Invalid Data Types: Mismatch between data types in the XML and schema.
- Unclosed Tags: Syntax errors like missing closing tags in XML.
- Improper Nesting: Elements not following the hierarchical structure defined in the schema.
Applications of XML Validation in Java Full Stack Development
- Web Services: Ensuring API responses conform to the expected format.
- Configuration Files: Validating configuration files for servers or frameworks.
- Data Interchange: Validating data exchanged between microservices.
- Database Integration: Ensuring XML-based data imported/exported aligns with database schemas.
Best Practices for XML Validation
XML validation is a crucial step in ensuring the reliability and robustness of XML-based applications. Adopting best practices for XML validation can significantly improve the accuracy and efficiency of your applications, while minimizing errors and potential risks. Here’s a detailed look at some essential best practices every developer should follow:
Use Schema Definitions from the Start
Always define and use a proper XML Schema Definition (XSD) or Document Type Definition (DTD) when creating XML documents. These definitions serve as the blueprint for your XML structure, specifying the rules for data types, element hierarchy, and required attributes. This ensures consistency and avoids ambiguities in how the XML is structured and interpreted by different systems.
Validate XML Early and Often
Incorporate XML validation as an integral part of your Development lifecycle. Validating early during the creation of XML documents or data transmission ensures that issues are caught before they escalate. For applications that generate XML programmatically, integrate validation checks as part of the coding process to prevent invalid XML from reaching other parts of the system.
Leverage Validation Tools and Libraries
Use reliable XML parsers and validators like SAX, DOM, or StAX in Java, which support XML validation against XSDs or DTDs. Many modern IDEs and tools, such as Eclipse, IntelliJ IDEA, or online validators, provide built-in features for validating XML. These tools can simplify and automate validation tasks, making it easier to maintain accuracy.
Keep Schema Definitions Up to Date
As your application evolves, your XML schema or DTD may need updates to reflect changes in business logic or data requirements. Ensure that any modifications to schema definitions are promptly implemented and tested to avoid compatibility issues with older XML documents. Version control for schema files can help manage updates efficiently.
Avoid Overcomplicating Schema Designs
While it might be tempting to create a highly detailed and complex schema, simplicity is key to maintainability. Overcomplicated schemas can become difficult to understand and manage, leading to potential errors during validation. Keep your schema clear and concise, focusing on the essential elements and attributes needed for the application.
Conclusion
Validating XML documents using XSD and DTD is a critical skill for Java developers. It ensures that data exchanged between systems adheres to expected formats and structures, reducing errors and enhancing application stability.
Mastering XML validation is an integral part of Java Full Stack Developer Training, as it equips developers with the tools to build reliable and scalable applications.
Ready to take your Java skills to the next level? Enroll in H2K Infosys’ Java Full Stack Developer Training today and build expertise in XML validation, backend development, and full-stack application design!
One Response
is it possible to validate XML Document by DTD & XSD at the same time? what do i mean is the DTD & XSD validate code is inserted on the XML File. like this