Introduction
Functional automation testing frameworks come in various flavours, including Keyword Driven Framework, Table-Driven Framework, and Action Word-based Framework. The Keyword Driven Framework’s fundamental operation is the division of the Test Case into four distinct components. Test Step is the first, followed by Test Step’s Object, Test Step’s Action, Test Object’s Data, and Test Object’s Action. You can check out our Selenium automation testing course Online to learn more.
With the aid of an Excel spreadsheet, the aforementioned categorization can be completed and maintained:
- Test Action: It is a brief explanation of the test step or the action that will be performed on the test object.
- Test Object: It’s the name of the object or element on the web page, similar to username and password.
- Action: This is the name of the procedure that will be carried out on any object, such as a click, an open browser window, an input, etc.
- Test Data: Data can be any data that the Object requires to carry out any activity, such as the value of the Username field.
With the keyword-driven approach to automation, the coding is kept distinct from the test case and test phase. This makes it much easier for a non-technical individual to comprehend automation. A manual tester can create automation scripts using this. While a hard-core technical coder is required for every keyword-driven project to build up the framework and work on ongoing changes and upgrades to the background automation code, this does not imply that an automation tester is not required. However, as an illustration, an automation team could consist of an automation coder and two manual testers.
What is Keyword Driven Testing?
Keyword Driven Testing (KDT) is a framework design pattern used to automate tests. It’s a methodology that allows testers to separate the test logic from the test data. This is achieved by defining keywords that represent actions to be performed on the application under test. These keywords are then written in an external data source, such as an Excel sheet or a database, which makes the tests highly readable and reusable.
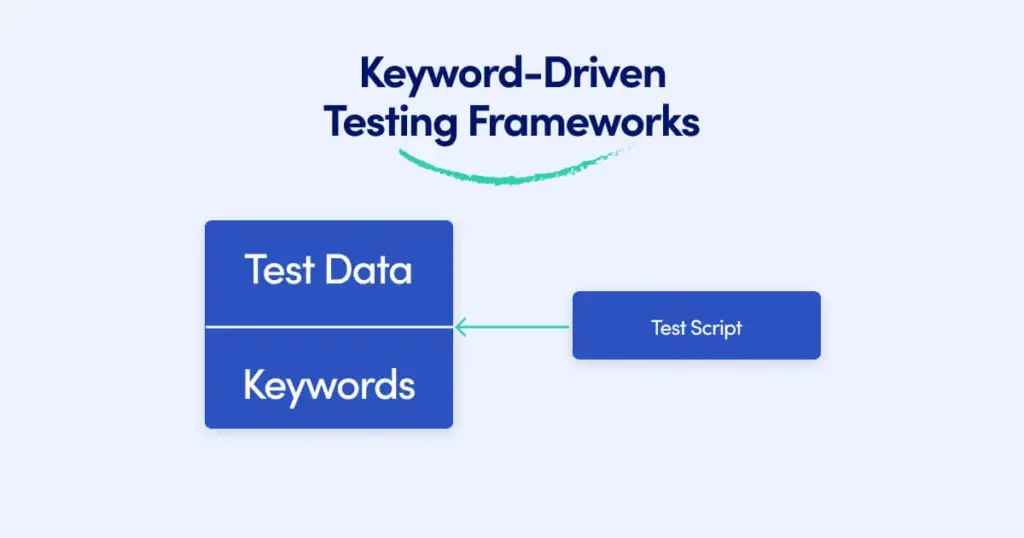
In simple terms, a Keyword Driven Framework allows testers to define a set of keywords or commands, such as “Click”, “Enter”, or “Verify”, that are used in the automation script to perform specific actions on the application. These keywords act as instructions for the automation tool to execute the tests.
Key Features of Keyword Driven Framework:
- Separation of Concerns: Test data and test logic are separated.
- Reusability: Keywords can be reused across multiple test cases.
- Readability: Test scripts are easier to read and maintain.
- Ease of Use: Testers with minimal programming knowledge can use the framework by interacting with the test data (typically in Excel).
Why Use the Keyword Driven Framework in Selenium?
The Keyword Driven Framework is particularly beneficial for Automation testing in Selenium for the following reasons:
Improved Test Maintenance:
Maintaining test scripts in traditional frameworks can be tedious and time-consuming. However, in a Keyword Driven Framework, test cases are data-driven and independent of the test scripts. This makes it much easier to modify test data without needing to rewrite the entire script.
Supports Test Automation for Non-Programmers:
One of the biggest advantages of the Keyword Driven Framework is that it enables non-programmers or business users to define and execute tests. By using simple keywords in an Excel sheet, even those without coding expertise can participate in writing and running test cases.
Enhanced Reusability:
The framework encourages reusability by defining keywords that can be reused across multiple test cases. For example, a “Login” keyword that tests the login functionality can be reused in all tests where the login step is required.
Scalability:
Keyword Driven Frameworks scale easily for large projects. Once you’ve created your initial set of keywords, you can quickly expand and add new test cases or functionalities by simply adding more data to the external files (Excel, CSV).
Easier to Understand and Implement:
With its tabular structure, the Keyword Driven Framework is easy to understand, especially for those new to automation software testing courses. Testers only need to focus on the test data, making it much simpler to implement automation.
How Does Keyword Driven Framework Work in Selenium?
Test Case Preparation:
In the Keyword Driven Framework, the test case is prepared using a set of keywords that describe each action to be performed. These actions are written in an external data source, such as an Excel file. For instance, consider the following data in an Excel file:
Step | Action | Element | Value |
---|---|---|---|
1 | Open | Browser | Chrome |
2 | Navigate | URL | www.example.com |
3 | Enter | username | testuser |
4 | Enter | password | password123 |
5 | Click | loginButton | |
6 | Verify | loginMessage | Welcome! |
Automation Script:
The automation script is written in a programming language (usually Java, Python, or C#). It includes code that interprets the keywords and performs the actions accordingly. Here is a simplified example of how the automation script could work in Java using Selenium:
javaCopy codepublic class KeywordDrivenTest {
WebDriver driver = new ChromeDriver();
public void executeTest(String action, String element, String value) {
switch (action.toLowerCase()) {
case "open":
driver.get(value);
break;
case "navigate":
driver.get(value);
break;
case "enter":
WebElement field = driver.findElement(By.name(element));
field.sendKeys(value);
break;
case "click":
WebElement button = driver.findElement(By.name(element));
button.click();
break;
case "verify":
WebElement message = driver.findElement(By.id(element));
assert message.getText().equals(value);
break;
default:
throw new IllegalArgumentException("Invalid action: " + action);
}
}
}
Execution:
The test execution involves reading each row from the external data source (Excel or CSV), interpreting the keywords, and performing the corresponding actions in the Selenium script.
Benefits of Keyword Driven Framework for Selenium Automation
Test Script Reusability:
Testers can reuse common keywords across multiple test cases, improving efficiency and reducing redundant code. For example, the “Enter” keyword can be used in various places where data entry is required.
Cross-Functional Collaboration:
With the Keyword Driven Framework, testers, developers, and Business analysts can collaborate more easily. Business analysts can write test data in the form of keywords without worrying about the underlying code, while developers focus on implementing the keywords in Selenium scripts.
Data-Driven Testing:
Since the test cases are data-driven, it’s easy to run the same test with different sets of data. This makes it highly effective for testing applications with varied inputs and user scenarios.
Reduced Test Script Complexity:
By using keywords, the complexity of test scripts is reduced. The script does not need to be aware of the specific actions and elements on the page. The external data (Excel, CSV) handles that, making the code cleaner and more focused on the execution logic.
Faster Test Execution:
Once the keywords are implemented and mapped to actions, the test cases can be executed faster. The only focus is on reading the data and performing the corresponding actions, making test execution efficient.
Practical Example: A Simple Keyword Driven Framework in Selenium
Let’s walk through a simple example of using the Keyword Driven Framework in Selenium. Assume that we want to test a login page with the following steps:
- Open the browser.
- Navigate to the URL.
- Enter the username and password.
- Click the login button.
- Verify that the login message is displayed.
We have a corresponding Excel file:
Step | Action | Element | Value |
---|---|---|---|
1 | Open | Browser | Chrome |
2 | Navigate | URL | www.example.com |
3 | Enter | username | testuser |
4 | Enter | password | password123 |
5 | Click | loginButton | |
6 | Verify | loginMessage | Welcome! |
Code Implementation:
javaCopy codepublic class KeywordDrivenFramework {
WebDriver driver;
String[][] testData;
public void readTestData() {
// Code to read data from Excel file (for simplicity, using a 2D array)
testData = new String[][] {
{"Open", "Browser", "Chrome"},
{"Navigate", "URL", "www.example.com"},
{"Enter", "username", "testuser"},
{"Enter", "password", "password123"},
{"Click", "loginButton", ""},
{"Verify", "loginMessage", "Welcome!"}
};
}
public void executeTest() {
for (String[] row : testData) {
String action = row[0];
String element = row[1];
String value = row[2];
executeAction(action, element, value);
}
}
public void executeAction(String action, String element, String value) {
switch (action.toLowerCase()) {
case "open":
driver.get("www.example.com");
break;
case "navigate":
driver.navigate().to(value);
break;
case "enter":
driver.findElement(By.name(element)).sendKeys(value);
break;
case "click":
driver.findElement(By.name(element)).click();
break;
case "verify":
assert driver.findElement(By.id(element)).getText().equals(value);
break;
}
}
public static void main(String[] args) {
KeywordDrivenFramework framework = new KeywordDrivenFramework();
framework.readTestData();
framework.executeTest();
}
}
Take into account that you need to automate the following steps in a flow:
- Launch a browser
- Go to the URL
- and select the “My Account” link.
- Input the Username
- Specify Password
- Press the Login button.
- Activate the Logout button.
- Close the browser
The following is a list of typical elements you will need to complete the task:
- Excel Worksheet: The majority of the keyword-driven data, including Test cases, Test Steps, Test Objects, and Test Actions, are kept on this sheet.
- Repository for objects: Property file to contain the web application’s HTML elements’ properties; this property file will be associated with the test objects.
- Term Function Database: Function files are crucial in the keyword-driven framework since they contain the workings of the actions, allowing each action to be called from this file.
- Data Sheet: An Excel file that contains the data values that an object needs to conduct an action on it.
- Execution Engine: The sole test script in the Keyword Framework is called Test, and it includes all the code necessary to run the test using an Excel sheet, a Function Lib, and a Properties file.
A Keyword Driven Framework’s general flow looks something like this:
- Execution Engine launches the test, connects to the collection of test cases, and begins running each one one at a time.
After selecting a test case, connected test steps are carried out consecutively.
- Additionally linked to page objects, actions, and test data are test steps.
- The execution Engine connects to the application and completes the test phase once it has all the information needed.
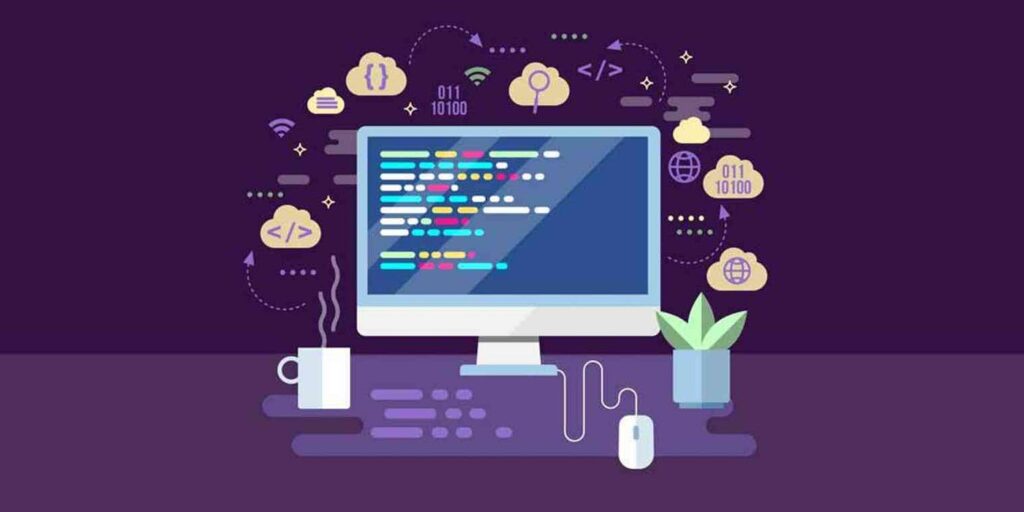
Advantages
- Less Technical Expertise: Manual testers and non-technical testers can quickly build test scripts for automation after the Framework is set up.
- Easy to understand: The test scripts are simple to read and comprehend because they are kept in Excel sheets and contain no exposed coding. Because keywords and actions match manual test cases so closely, they are simpler to create and manage.
- Early Start: Since an Object Repository may be readily set up later on, you can begin creating keyword-driven test cases before the application is delivered. Keyword data tables can be made using details acquired from Requirements or other documents, and they can be made to correlate to equivalent manual test processes.
Reusability of component: Reusability can be improved by adding modularization in Keyword Driven.
Reusability of code: The Keyword Driven Framework encourages extremely high code reuse because there is only one Execution Engine.
Conclusion
The Keyword Driven Framework in Selenium is an incredibly powerful tool that enhances the effectiveness, readability, and reusability of automation test scripts. By separating the test logic from the test data, it enables faster test creation, maintenance, and execution. Furthermore, it opens the door for non-programmers to engage with automation testing, empowering cross-functional teams to collaborate effectively.
Whether you’re a beginner or an experienced tester, incorporating Keyword Driven Testing into your Automation software testing course can significantly improve your testing skills and boost your career.
Key Takeaways:
- The Keyword Driven Framework simplifies test creation and execution.
- It improves collaboration across teams and enhances reusability.
- It’s ideal for both technical and non-technical users.
Ready to dive deeper into Selenium automation testing? Enroll in H2K Infosys’ hands-on automation software testing course today and enhance your career skills!