In Python programming, especially for data analysis and scientific computing, NumPy is an essential library that helps in managing large datasets with ease. Two of the most frequently used functionalities in NumPy are NumPy Reshape and NumPy Flatten. These functions enable users to manipulate the shape of arrays, making it easier to handle data in different structures. Whether you are working with multi-dimensional data for machine learning or analyzing large datasets, understanding these functions is crucial.
In this comprehensive guide, we will cover NumPy Reshape and NumPy Flatten in Python, explaining how they work, their practical applications, and real-world use cases. This knowledge is invaluable for professionals and learners looking to gain expertise through a Python online training course or simply looking to enhance their data manipulation skills.
A vital property of NumPy arrays is their shape. The shape of an array can be determined by using the numpy reshape and numpy flatten attribute. But what about if you want to change the shape of an array? The numpy. Reshape () and numpy.flatten() functions are used to change the shape of an array. In this tutorial, we will discuss how to implement them in your code.
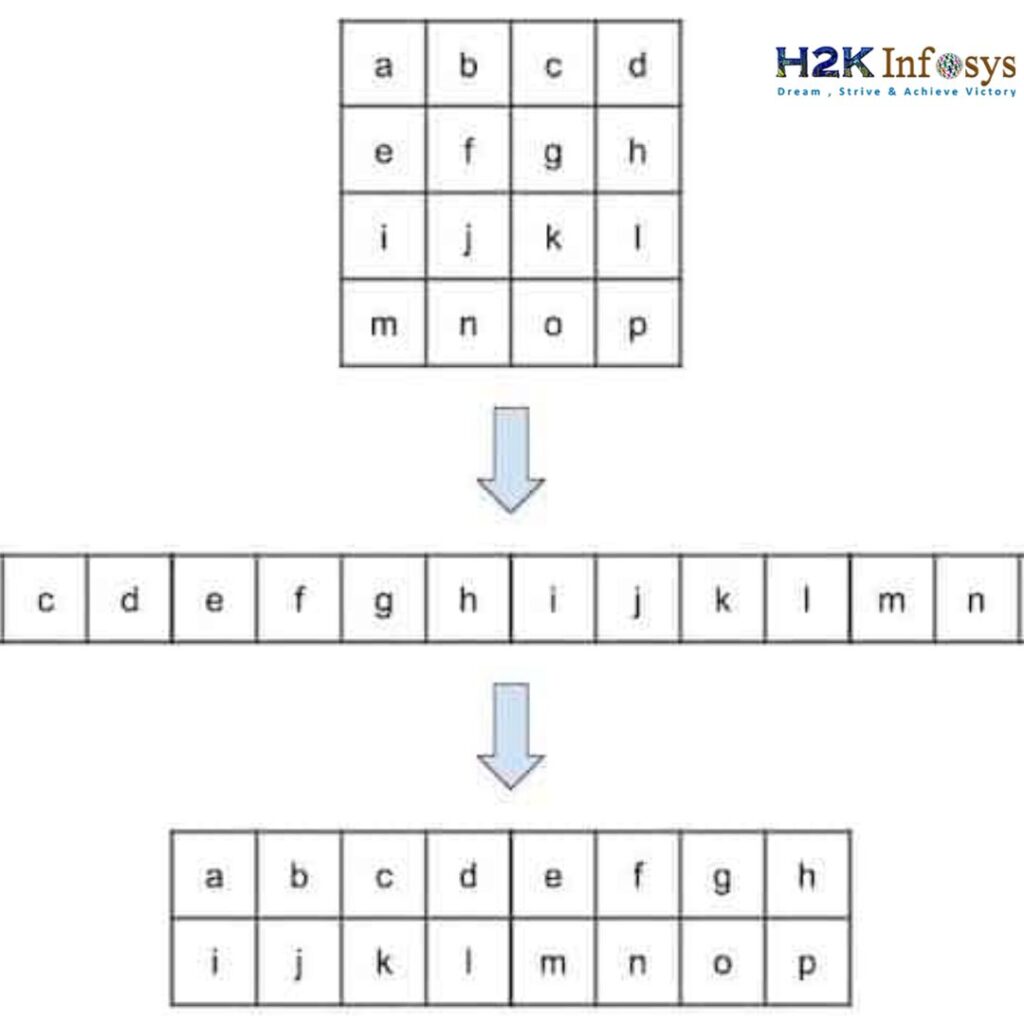
Working with Array Shapes in NumPy Reshape and NumPy Flatten
In data science and machine learning, arrays are fundamental structures used to represent data. The ability to manipulate the shape of arrays is crucial for tasks like matrix operations, reshaping datasets, or preparing input data for models. NumPy, a powerful library in Python, offers two important methods for shape manipulation: numpy.reshape()
and numpy.flatten()
. Both serve different purposes but are often used together in various tasks.
In this guide, we will explore:
- How to determine the shape of an array.
- How to change the shape of an array.
- When to use
reshape()
vs.flatten()
.
Determining the Shape of an Array
Before diving into reshaping or flattening, it’s essential to understand how to determine the shape of an array.
You can check the shape of a NumPy array using the .shape
attribute. This tells you the number of elements along each dimension.
Example:
python
import numpy as np
# Create a 3x2 array
arr = np.array([[1, 2], [3, 4], [5, 6]])
# Check the shape of the array
print(arr.shape) # Output: (3, 2)
Here, the array has 3 rows and 2 columns, so the shape is (3, 2)
.
Changing the Shape of an Array: numpy.reshape()
The numpy.reshape()
function is used to change the shape of an array without altering its data. This is particularly useful when you need to transform data between different formats (e.g., converting a 2D array into a 1D array, or a 1D array into a 3D array).
Syntax:
python
numpy.reshape(arr, new_shape, order='C')
arr
: The array to reshape.new_shape
: The desired shape as a tuple. You can specify-1
for one of the dimensions, and NumPy will automatically infer the correct size based on the number of elements.order
: This determines how the array is read and reshaped.'C'
reads row-wise (C-style, default),'F'
reads column-wise (Fortran-style).
Example 1: Reshape a 1D array to a 2D array
python
# Create a 1D array
arr = np.array([1, 2, 3, 4, 5, 6])
# Reshape it to 2D (3 rows, 2 columns)
reshaped_arr = np.reshape(arr, (3, 2))
print(reshaped_arr)
Output:
lua
[[1 2]
[3 4]
[5 6]]
Example 2: Use -1
to let NumPy infer the shape
python
# Reshape 1D array to 3 rows, with columns inferred automatically
reshaped_arr = np.reshape(arr, (3, -1))
print(reshaped_arr)
Output:
lua
[[1 2]
[3 4]
[5 6]]
Here, specifying -1
tells NumPy to compute the number of columns automatically based on the array’s size.
Using the reshape() method
The reshape()
method is commonly used in programming, particularly in Python with libraries like NumPy, to change the shape of an array without altering its data. This method is crucial in data manipulation, machine learning, and scientific computing, where you often need to adjust data structures to fit specific algorithms or visualization requirements.
Understanding the reshape()
Method in NumPy
The reshape()
method in NumPy allows you to change the shape of an array. It returns a new array with the specified shape, but the total number of elements must remain the same.
Syntax:
python
numpy.reshape(array, new_shape)
array
: The input array you want to reshape.new_shape
: The desired shape of the array, specified as a tuple (e.g.,(rows, columns)
). The product of the dimensions in the new shape must match the number of elements in the original array.
Key Points:
- The
reshape()
method does not change the data itself; it only changes the arrangement of elements. - The new shape must be compatible with the total number of elements. For example, an array with 12 elements can be reshaped to
(3, 4)
,(4, 3)
,(2, 6)
, etc., but not(5, 3)
.
Examples of Using reshape()
in NumPy
Here are some examples demonstrating the use of the reshape()
method:
Example 1: Basic Reshaping
python
import numpy as np
# Create a 1D array with 12 elements
arr = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12])
# Reshape to a 2D array with 3 rows and 4 columns
reshaped_arr = np.reshape(arr, (3, 4))
print(reshaped_arr)
Output:
lua
[[ 1 2 3 4]
[ 5 6 7 8]
[ 9 10 11 12]]
Example 2: Reshape to a 3D Array
python
# Reshape the same array to a 3D array with shape (2, 3, 2)
reshaped_arr_3d = np.reshape(arr, (2, 3, 2))
print(reshaped_arr_3d)
Output:
lua
[[[ 1 2]
[ 3 4]
[ 5 6]]
[[ 7 8]
[ 9 10]
[11 12]]]
Example 3: Using -1
to Automatically Calculate Dimension
The -1
parameter can be used in the reshape to let NumPy automatically calculate the size of that dimension.
python
# Let NumPy decide the number of rows by specifying -1
reshaped_arr_auto = np.reshape(arr, (-1, 6))
print(reshaped_arr_auto)
Output:
luaCopy code[[ 1 2 3 4 5 6]
[ 7 8 9 10 11 12]]
In this example, the -1
tells NumPy to figure out the correct number of rows, which results in two rows because 12 elements need to be split across 6 columns.
Common Use Cases of reshape()
- Data Preparation: Reshaping arrays to feed into machine learning models, especially when working with image data or neural networks that require specific input shapes.
- Matrix Operations: Changing the arrangement of data for matrix operations, ensuring compatibility with other matrices in multiplication, addition, etc.
- Data Visualization: Adjusting the shape of data to fit visualization tools like heatmaps, 3D plots, etc.
Error Handling
If you try to reshape an array to a shape that doesn’t match the number of elements, you will get a ValueError
.
python
# Incorrect reshaping due to incompatible shape
try:
incorrect_reshape = np.reshape(arr, (5, 3))
except ValueError as e:
print(f"Error: {e}")
Output:
sqlCopy codeError: cannot reshape array of size 12 into shape (5,3)
The reshape() method is especially useful when building convolutional neural networks as most times, you will need to reshape the image shape from a 2-dimensional to a 3-dimensional array.
Using the numpy.flatten() method
The numpy.flatten()
method in NumPy is used to return a copy of the array collapsed into one dimension (i.e., it flattens the array into a 1D array). It works on arrays of any dimensionality, and the resulting array will contain all the elements from the original array in a single row.
Here’s a breakdown of the syntax:
Syntax
python
numpy.ndarray.flatten(order='C')
Parameters:
- order: Specifies the order in which the elements are read:
'C'
(default): Row-major order (C-style), which means elements are read row-wise.'F'
: Column-major order (Fortran-style), which means elements are read column-wise.'A'
: ‘F’ if the array is Fortran contiguous, ‘C’ otherwise.'K'
: As close to the memory layout as possible.
Example:
python
import numpy as np
# Creating a 2D array
arr = np.array([[1, 2], [3, 4]])
# Flattening the array
flat_arr = arr.flatten()
print(flat_arr)
Output:
csharp
[1 2 3 4]
You can also specify the order
parameter:
pythonCopy code# Flattening the array using Fortran-style order
flat_arr_f = arr.flatten(order='F')
print(flat_arr_f)
Output:
csharp
[1 3 2 4]
Key points:
flatten()
returns a copy of the original array.- If you want a flattened view instead of a copy, consider using
ravel()
which is more memory efficient when possible.
If you have any questions, feel free to leave them in the comment section and I’ll do my best to answer them.
Conclusion:
Understanding how to use NumPy Reshape and NumPy Flatten is essential for anyone working in data science, machine learning, or scientific computing. These powerful functions allow you to manipulate data structures, making it easier to prepare datasets for analysis, train machine learning models, and process multi-dimensional data more efficiently. Whether you’re reshaping a 1D array into 2D for easier analysis or flattening multi-dimensional arrays to feed into algorithms, these operations can significantly optimize your workflow.
For professionals and learners alike, mastering these functions opens up a wealth of opportunities to work with complex datasets across various fields. Enrolling in H2K’s Python online training course will give you hands-on experience in using NumPy Reshape, NumPy Flatten, and other advanced Python libraries, ensuring you’re prepared to handle real world data challenges with ease.
Don’t miss out on the opportunity to enhance your skills join H2K’s Python online training course today and gain the expertise to become proficient in data manipulation and more
2 Responses
Thanks!
You’re welcome!