Introduction
Python is one of the most sought-after programming languages due to its simplicity, versatility, and broad applications, ranging from web development to data science, machine learning, automation, and more. If you’re pursuing a career in this development, it’s essential to have a solid understanding of core Python concepts and frameworks. To help you get prepared, we’ve compiled top 50 interview questions to test your skills.
Whether you’re looking for a Certification on Python programming or interested in online training on Python, mastering these essential topics will equip you for the job market. Let’s dive into the most important interview questions and answers you’ll face in 2025.
Interview Questions and Answers
1. What is Python?
Answer:
It is an interpreted, high-level programming language created by Guido van Rossum in 1991. It is known for its easy-to-read syntax, which makes it beginner-friendly and versatile for various applications, including web development, machine learning, and automation.
2. What are the key features of Python?
Answer:
- Easy-to-read syntax
- Extensive standard library
- Object-oriented
- Cross-platform compatibility
- Support for both procedural and functional programming
- Dynamic typing and automatic memory management
3. What is the difference between Python 2.x and Python 3.x?
Answer:
Python 3.x introduced several improvements, including better Unicode support, print as a function, and division of integers returning floats by default. Python 2.x, though deprecated, is still used in legacy systems. It’s crucial to use this 3 for all new projects.
4. What are Python decorators?
Answer:
Decorators are functions that allow you to modify or extend the behavior of other functions or methods without changing their code. They are commonly used in this language to add functionality to existing code in a clean, readable way.
5. Explain Python’s memory management.
Answer:
This uses automatic memory management, which includes reference counting and garbage collection. When an object is no longer referenced, the memory occupied by the object is released by the Garbage collector. The del
keyword can be used to delete objects explicitly.
6. What is a lambda function in Python?
Answer:
A lambda function is a small anonymous function defined using the lambda
keyword. It can have any number of arguments but only one expression. It is used for short-lived tasks where a function is required temporarily.
Example:
pythonCopy codeadd = lambda x, y: x + y
7. What are Python’s data types?
Answer:
It has several built-in data types, including:
- Numeric types:
int
,float
,complex
- Sequence types:
list
,tuple
,range
- Text type:
str
- Mapping type:
dict
- Set types:
set
,frozenset
- Boolean type:
bool
- Binary types:
bytes
,bytearray
8. What is a list comprehension in Python?
Answer:
List comprehension provides a concise way to create lists. It involves a single line of code, making it more efficient and readable than traditional for loops.
Example:
pythonCopy codesquares = [x**2 for x in range(10)]
9. What is the difference between deepcopy
and shallow copy
?
Answer:
- Shallow copy: Copies the reference of an object, meaning changes made to nested objects will reflect in both the original and copied objects.
- Deep copy: Creates a new object along with copies of nested objects, ensuring no reference is shared between the original and copied objects.
10. What is a tuple in Python?
Answer:
A tuple is an immutable, ordered collection of elements. Tuples are similar to lists but cannot be modified after their creation. They are typically used for heterogeneous data and ensure Data integrity.
Example:
pythonCopy codecoordinates = (3, 4)
11. Explain the concept of inheritance in Python.
Answer:
Inheritance is an object-oriented programming concept where a new class (child class) inherits properties and behaviors (methods) of an existing class (parent class). This promotes code reusability and modularity.
12. What is the purpose of self
in Python?
Answer:self
represents the instance of the class. It is used to access attributes and methods of the class. It differentiates instance variables from local variables in methods.
13. What are Python modules and packages?
Answer:
- Modules are files containing the code, including functions, variables, and classes.
- Packages are directories containing multiple modules. They are used to organize related modules in a structured manner.
14. What is the purpose of the __init__
method in Python?
Answer:__init__
is the constructor method in Python, which is automatically called when a new object is created from a class. It is used to initialize the object’s attributes.
15. What are the types of arguments in Python functions?
Answer:
The functions can accept:
- Positional arguments: Passed based on their position.
- Keyword arguments: Passed using key-value pairs.
- Default arguments: Assigned default values if not provided.
- Variable-length arguments:
*args
for non-keyword arguments and**kwargs
for keyword arguments.
16. What is a generator in Python?
Answer:
A generator is a type of iterable, like a list, but it doesn’t store its contents in memory. It yields items one at a time using the yield
keyword, making it memory efficient for large datasets.
17. How does exception handling work in Python?
Answer:
It handles exceptions using try
, except
, else
, and finally
blocks. The try
block is used for code that might raise an exception, except
catches the exception, else
executes if no exception occurs, and finally
runs regardless of the outcome.
18. What is the difference between ==
and is
operators in Python?
Answer:
==
checks if the values of two objects are equal.is
checks if two objects refer to the same memory location.
19. What is the use of pass
in Python?
Answer:
The pass
statement is a null operation used as a placeholder in code blocks where an action is required syntactically but no action is needed logically. It can be used in loops, functions, or classes.
20. How can you handle memory leaks in Python?
Answer:
Memory leaks in Python can be minimized by using it’s automatic garbage collection and reference counting. Tools like gc
module and memory profilers can be used to detect and manage memory usage.
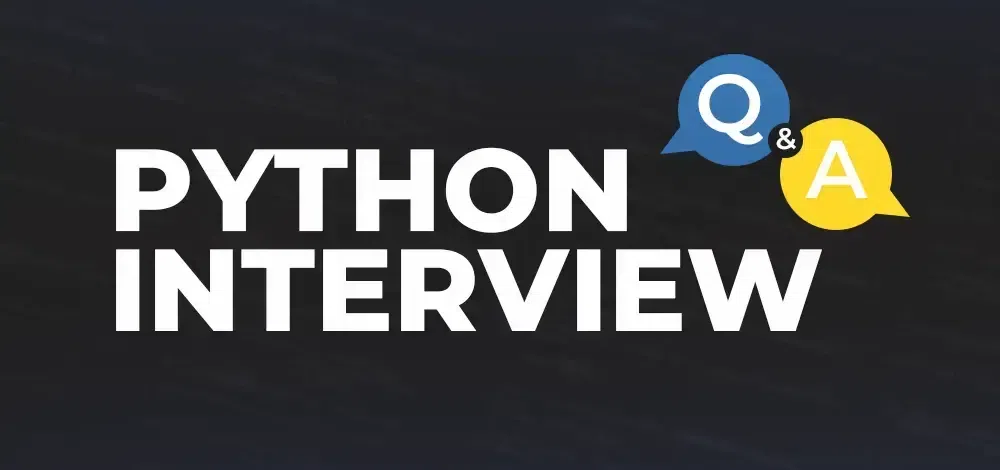
21. What is the difference between a function and a method in Python?
Answer:
- A function is a block of code that performs a specific task and can be called directly.
- A method is a function associated with an object, accessed using dot notation (e.g.,
object.method()
).
22. What are Python’s built-in data structures?
Answer:
It provides several built-in data structures, including:
- Lists: Ordered and mutable collections.
- Tuples: Ordered but immutable collections.
- Sets: Unordered collections of unique elements.
- Dictionaries: Unordered collections of key-value pairs.
23. What are Python’s built-in functions?
Answer:
It has numerous built-in functions such as len()
, range()
, sum()
, min()
, max()
, abs()
, and print()
. These functions help simplify many tasks.
24. What is a Python iterator?
Answer:
An iterator is an object that implements the __iter__()
and __next__()
methods. It allows you to traverse through all the elements in a collection (like lists, tuples) without exposing the underlying data structure.
25. What are Python lists and tuples?
Answer:
- Lists are ordered and mutable collections of elements, whereas
- Tuples are ordered and immutable collections.
26. What is the difference between range()
and xrange()
?
Answer:
range()
returns a list in Python 2.x and a generator in Python 3.x.xrange()
returns an iterator in Python 2.x and doesn’t exist in Python 3.x.
27. What is a Python class method?
Answer:
A class method is bound to the class, not the instance. It is defined with the @classmethod
decorator and takes cls
as its first argument.
28. How does Python handle memory management?
Answer:
It uses reference counting and garbage collection for memory management. Unused objects are automatically removed by the garbage collector.
29. What are Python’s built-in modules?
Answer:
This includes built-in modules like os
, sys
, math
, random
, datetime
, and json
that provide various functionalities for operating system interaction, mathematical computations, file handling, etc.
30. What is the purpose of the global
keyword in Python?
Answer:
The global
keyword is used to modify a variable outside the current function’s scope, allowing the function to change the value of a global variable.
31. What is a Python list slice?
Answer:
List slicing allows you to extract a portion of a list using a range of indices. Example: my_list[1:4]
returns elements from index 1 to 3.
32. What are Python’s super()
and __init__()
methods?
Answer:
super()
is used to call a method from a parent class in a subclass.__init__()
is the constructor method called when a class object is created, initializing instance variables.
33. What is the purpose of the yield
keyword in Python?
Answer:
The yield
keyword is used in a function to return an iterator. It helps generate a sequence of values lazily, which means values are produced only when needed.
34. What is Python’s __str__()
method?
Answer:
The __str__()
method in Python is used to return a string representation of an object, which is returned by the print()
function.
35. Explain the use of the try-except
block in Python.
Answer:
The try-except
block is used to handle exceptions in Python. The code in the try
block is executed, and if any exception occurs, it is handled in the except
block.
36. What are Python’s *args
and **kwargs
?
Answer:
*args
is used to pass a variable number of non-keyword arguments to a function.**kwargs
allows you to pass a variable number of keyword arguments to a function.
37. What is Python’s assert
statement?
Answer:
The assert
statement is used to test if a condition is true. If it evaluates to False
, it raises an AssertionError
.
38. How do you define a dictionary in Python?
Answer:
A dictionary is defined using curly braces {}
, with key-value pairs. Example:
pythonCopy codemy_dict = {"name": "Alice", "age": 25}
39. What are Python sets and how are they different from lists?
Answer:
A set is an unordered collection of unique elements, whereas a list is ordered and allows duplicates.
40. What are Python’s built-in data structures?
Answer:
Python has several built-in data structures such as lists, tuples, dictionaries, and sets, each serving different use cases for organizing and managing data.
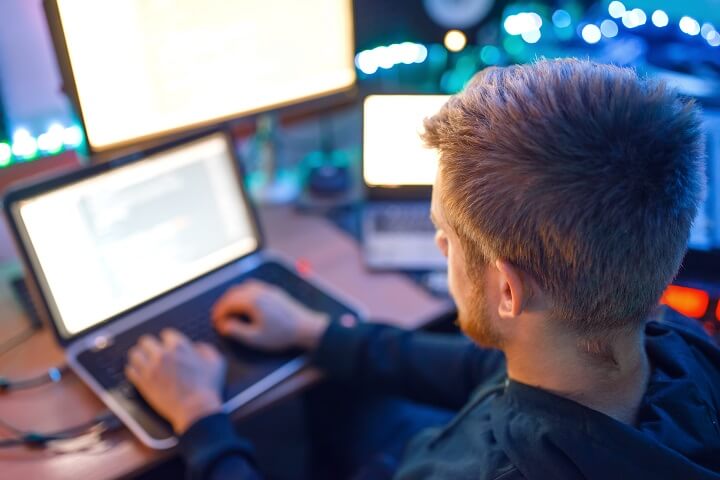
41. What is the difference between is
and ==
in Python?
Answer:
==
checks if two objects have the same value.is
checks if two objects reference the same memory location.
42. How can you reverse a list in Python?
Answer:
You can reverse a list in Python using list[::-1]
, or by using the reverse()
method on the list.
43. What are Python’s f-strings?
Answer:
F-strings are a way to embed expressions inside string literals. They are prefixed with an f
and use curly braces {}
to embed variables. Example:
pythonCopy codename = "Alice"
greeting = f"Hello, {name}!"
44. How do you handle exceptions in Python?
Answer:
Exceptions in Python are handled using the try-except
block. You can also use else
for code that runs if no exception occurs, and finally
for cleanup actions that run no matter what.
45. What is the difference between del
and remove()
in Python?
Answer:
del
is used to delete an object or element at a specified index or to delete an entire list.remove()
is used to remove the first occurrence of a value from a list.
46. How do you perform unit testing in Python?
Answer:
Unit testing in Python is done using the built-in unittest
module. You can create test cases by subclassing unittest.TestCase
and defining methods with assertions.
47. What is Python’s join()
method?
Answer:
The join()
method is used to join elements of an iterable (like a list or tuple) into a single string, with a specified separator between them.
Example:
pythonCopy codewords = ["Hello", "World"]
sentence = " ".join(words) # Output: "Hello World"
48. What is the purpose of os
module in Python?
Answer:
The os
module in Python provides functionalities for interacting with the operating system, such as reading or writing files, handling directories, and manipulating environment variables.
49. What is the difference between open()
and with open()
in Python?
Answer:
open()
opens a file for reading or writing but does not automatically close it.with open()
ensures that the file is properly closed after the block of code is executed, making it safer for file handling.
50. How do you check if a file exists in Python?
Answer:
You can check if a file exists using the os.path.exists()
or os.path.isfile()
method.
Example:
pythonCopy codeimport os
if os.path.exists('file.txt'):
print("File exists")
Conclusion
The future of Python is promising, and it remains one of the most valuable languages for developers. Understanding core concepts such as data types, classes, functions, and exception handling is essential for any developer looking to excel in their career.
Whether you are looking to advance your career with an Online training on Python, mastering these key areas will provide you with the skills necessary to succeed in the industry.
Start your journey by enrolling in one of the leading Python training courses today to unlock your potential and be prepared for the challenges ahead!
For instance, the open statement is a context manager in itself, which lets you open a file, keep it open as long as the execution is in the context of the with statement where you used it, and close it as soon as you leave the context, no matter whether you have left it because of an exception or during regular control flow.