In this article, we’ll explore some of the most commonly used Python Methods and Functions that are essential for any programmer. Python, known for its simplicity and versatility, offers a wide array of built-in functions and methods to streamline programming tasks. Whether you are pursuing a Python Certification or enrolled in a Python Online Course, mastering these functions will greatly enhance your coding efficiency.
We’ll cover topics such as finding elements, manipulating lists, and handling strings, providing examples and explanations for each. Understanding these core concepts is crucial for anyone aiming for a Python Online Course Certification.
Mastering Python methods and functions is crucial for any programmer. These built-in tools enhance code efficiency and readability. From manipulating lists to handling strings and finding elements, understanding Python methods and functions is essential, especially for those pursuing a Python Certification or enrolled in a Python Online Course
1. Calculating the Average in Python
To calculate the average of a list of numbers in Python, you can use the sum()
and len()
functions. Here’s a simple example:
numbers = [10, 20, 30, 40, 50]
average = sum(numbers) / len(numbers)
print("Average:", average)
2. Finding Elements in Python
.find() in Python
The find()
method is used to locate the position of a substring within a string. If the substring is found, it returns the index; otherwise, it returns -1.
text = "Hello, welcome to Python programming."
position = text.find("Python")
print("Position of 'Python':", position)
3. Flattening a List in Python
To flatten a nested list in Python, you can use list comprehensions or the itertools.chain
method. Here’s an example using list comprehensions:
nested_list = [[1, 2, 3], [4, 5], [6, 7, 8]]
flattened_list = [item for sublist in nested_list for item in sublist]
print("Flattened List:", flattened_list)
4. Index Function in Python
.index() Function in Python
The index()
method is used to find the first occurrence of a specified value in a list. It raises a ValueError
if the value is not found.
fruits = ["apple", "banana", "cherry"]
index = fruits.index("banana")
print("Index of 'banana':", index)
5. Join Method in Python
.join() Method in Python
The join()
method is used to concatenate elements of a list into a single string with a specified separator.
words = ["Hello", "world", "Python", "is", "great"]
sentence = " ".join(words)
print("Joined Sentence:", sentence)
6. Length Function in Python
.len() Function in Python
The len()
function returns the number of items in an object. This can be a list, string, tuple, dictionary, etc.
names = ["Alice", "Bob", "Charlie"]
print("Length of list:", len(names))
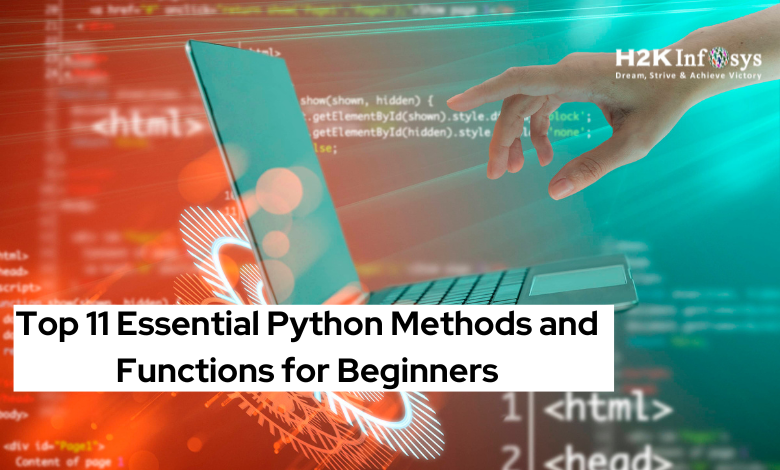
7. Lower Function in Python
.lower() Function in Python
The lower()
method converts all uppercase characters in a string to lowercase.
greeting = "HELLO WORLD"
print("Lowercase:", greeting.lower())
8. Map Function in Python
.map() Function in Python
The map()
function applies a given function to all items in an input list.
numbers = [1, 2, 3, 4, 5]
squared_numbers = list(map(lambda x: x**2, numbers))
print("Squared Numbers:", squared_numbers)
9. Readline Function in Python
.readline() Function in Python
The readline()
method reads a single line from a file.
with open('example.txt', 'r') as file:
first_line = file.readline()
print("First Line:", first_line)
10. Remove List Element in Python
.remove() List Element in Python
The remove()
method removes the first occurrence of a specified value from a list.
colors = ["red", "green", "blue", "green"]
colors.remove("green")
print("Colors after removal:", colors)
11. Replace in Python
.replace() Method in Python
The replace()
method replaces a specified phrase with another specified phrase in a string.
message = "Hello world"
new_message = message.replace("world", "Python")
print("Replaced Message:", new_message)
Conclusion
Understanding and effectively utilizing these common Python functions and methods can significantly enhance your programming skills. Whether you’re manipulating lists, handling strings, or performing arithmetic operations, these tools are essential for writing clean and efficient code. Keep practicing and exploring more functions to become a proficient Python programmer. Happy coding!