In the world of programming, writing clean, efficient, and error-free code is paramount. Python, known for its simplicity and readability, is a popular choice among developers. However, even experienced developers can make mistakes, and that’s where Python syntax checkers come into play. Syntax checkers help identify and correct errors in your code, ensuring it adheres to Python’s syntax rules. This article will explore how to use Python syntax checkers effectively to improve your code quality and avoid common pitfalls.
How to find error in python code
To find errors in Python code, you can use the following methods:
- Syntax Checkers (Linters): Tools like Pylint, Flake8, and Pyright analyze your code for syntax errors, style issues, and potential bugs.
- Debugging: Use the built-in Python debugger (
pdb
) or IDE-integrated debuggers to step through code, inspect variables, and identify errors. - Error Messages: Carefully read Python’s error messages and tracebacks to locate the source of the issue.
- Unit Tests: Write unit tests to validate code functionality and identify logical errors.
- Code Reviews: Peer reviews can help catch errors and improve code quality.
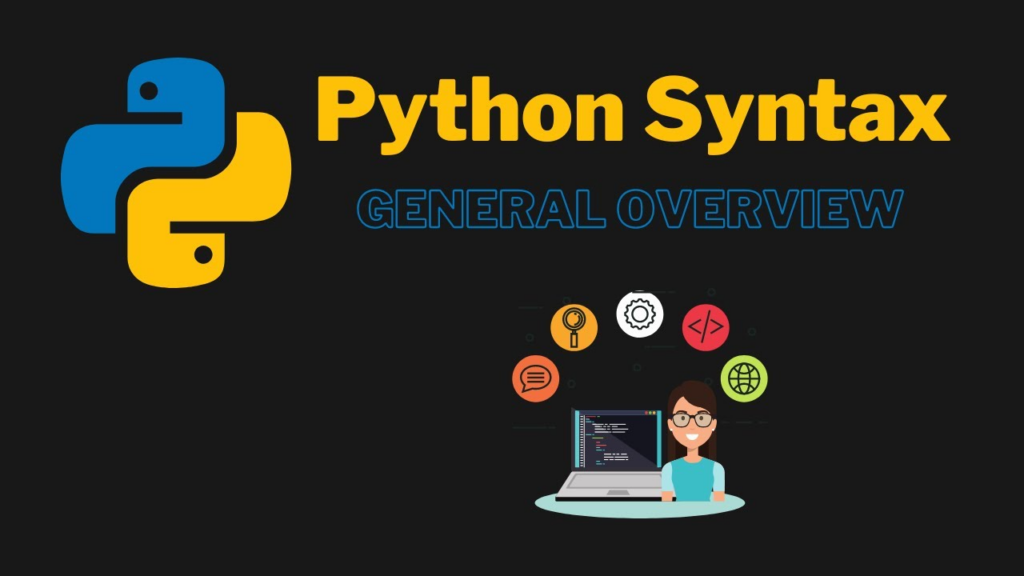
How to Fix Python Code: A Step-by-Step Guide
Debugging Python code efficiently requires a structured approach. Below is an in-depth breakdown of the key steps to fix issues in your Python code.
1. Review Error Messages
One of the most important steps in fixing Python code is carefully reading the error messages and tracebacks provided by the interpreter. These messages often indicate:
- The type of error (e.g.,
SyntaxError
,TypeError
,NameError
) - The specific file and line number where the issue occurs
- A brief description of what went wrong
🔹 Example:
pythonprint("Hello, world"
🛑 Error Output:
plaintextSyntaxError: unexpected EOF while parsing
💡 Fix: Missing closing parenthesis. Corrected version:
pythonCopyEditprint("Hello, world")
2. Debugging Techniques
Debugging tools help inspect variable values, track code execution, and identify logical errors.
✅ Using print()
Statements:
Insert print()
statements to check variable values and execution flow.
pythonx = 5
y = 0
print("Before division")
print(x / y) # This will cause a ZeroDivisionError
Fix: Ensure y
is not zero before performing division.
✅ Using pdb
(Python Debugger):
The built-in Python Debugger (pdb
) allows stepping through code interactively.
pythonimport pdb
def add(a, b):
pdb.set_trace() # Debugging starts here
return a + b
print(add(5, 10))
Commands inside pdb
:
n
(next step)s
(step into function)c
(continue execution)q
(quit debugging)
✅ Using IDE Debuggers:
Most modern IDEs (e.g., PyCharm, VS Code) have built-in debuggers that provide breakpoints, variable inspection, and execution control.
3. Syntax Checkers
Python has various linters and formatters that help detect syntax and style issues.
✅ Using flake8
:
bashpip install flake8
flake8 script.py
✅ Using pylint
:
bashpip install pylint
pylint script.py
✅ Using black
(Auto Formatter):
bashCopyEditpip install black
black script.py
These tools enforce PEP 8 standards and improve code readability.
4. Refactor Code
Refactoring involves restructuring code to improve clarity, efficiency, and maintainability.
✅ Break Down Complex Functions:
Instead of one long function, divide tasks into smaller, reusable functions.
❌ Bad Code:
pythondef calculate_area(shape, width, height):
if shape == "rectangle":
return width * height
elif shape == "triangle":
return 0.5 * width * height
else:
return None
✔ Refactored Code:
pythondef rectangle_area(width, height):
return width * height
def triangle_area(width, height):
return 0.5 * width * height
def calculate_area(shape, width, height):
shapes = {"rectangle": rectangle_area, "triangle": triangle_area}
return shapes.get(shape, lambda w, h: None)(width, height)
✅ Use Meaningful Variable Names:
Instead of vague variable names like a
, b
, use descriptive names:
python# Bad variable names
x = 5
y = 10
z = x * y
# Good variable names
width = 5
height = 10
area = width * height
5. Consult Documentation
Always refer to official Python documentation or external library documentation when unsure about functions and their usage.
6. Testing Code
Testing ensures that fixes work as expected and prevents future issues.
✅ Using assert
Statements for Quick Testing:
pythondef add(a, b):
return a + b
assert add(2, 3) == 5 # Test passes
assert add(-1, 1) == 0 # Test passes
✅ Writing Unit Tests (unittest
):
pythonimport unittest
def multiply(a, b):
return a * b
class TestMathOperations(unittest.TestCase):
def test_multiply(self):
self.assertEqual(multiply(3, 4), 12)
self.assertEqual(multiply(0, 5), 0)
if __name__ == "__main__":
unittest.main()
✅ Using pytest
for More Flexibility:
bashpip install pytest
pytest test_script.py
1. Understanding Python Syntax Checkers
Python syntax checkers, also known as linters, are tools that analyze your code for syntax errors, style issues, and potential bugs. They provide real-time feedback, helping developers catch mistakes early in the development process. These tools can be integrated into various development environments, making them accessible and easy to use.
2. Popular Python Syntax Checkers
Several Python syntax checkers are available, each with its unique features and capabilities. Here are some of the most popular ones:
- Pylint: Pylint is a widely used static code analysis tool that checks for errors, enforces coding standards, and suggests code refactoring. It provides detailed reports on your code’s quality and helps you follow best practices.
- Flake8: Flake8 is a lightweight tool that combines several linters, including PyFlakes, pycodestyle, and McCabe. It checks for syntax errors, style violations, and complexity issues, making it a versatile choice for Python Developers.
- Pyright: Pyright is a static type checker and linter for Python, developed by Microsoft. It offers type checking, error detection, and code suggestions, making it ideal for projects that require type annotations.
- Bandit: Bandit is a security-focused linter that scans your code for common security issues. It’s particularly useful for identifying vulnerabilities in Python applications.
3. Setting Up Python Syntax Checkers
Using syntax checkers helps ensure your Python code is clean, readable, and adheres to best practices. Two popular tools for this are Pylint and Flake8. Below is a step-by-step guide to setting up and using them effectively in your development environment.
3.1. Installing and Configuring Pylint
What is Pylint?
Pylint is a static code analysis tool that checks for errors, enforces coding standards (PEP 8), and suggests improvements in Python code.
Installing Pylint
To install Pylint, use pip, Python’s package manager. Run the following command in your terminal or command prompt:
bashpip install pylint
Running Pylint
To check a Python script with Pylint, navigate to the directory where your file is located and execute:
bashpylint filename.py
Pylint will analyze the file and generate a report that includes warnings, errors, and suggestions for improvement. The report typically consists of:
- Convention (C): Coding standard violations (e.g., variable naming issues)
- Refactor (R): Code that can be improved for better readability or efficiency
- Warning (W): Potential issues that may cause errors
- Error (E/F): Definite errors that prevent the script from running correctly
Configuring Pylint
Pylint allows customization using a configuration file (.pylintrc
). This is useful for tailoring checks according to your project’s requirements.
To generate a default configuration file, run:
bashpylint --generate-rcfile > .pylintrc
This will create a .pylintrc
file in your current directory. You can open and modify this file to:
- Disable specific warnings or errors
- Adjust thresholds for certain checks
- Customize the formatting of output reports
Example of disabling a warning (modify .pylintrc
):
ini[MESSAGES CONTROL]
disable=C0114 # Disables the warning for missing module docstrings
3.2. Installing and Configuring Flake8
What is Flake8?
Flake8 is another powerful tool that combines PyFlakes, pycodestyle, and McCabe to detect:
- Style violations based on PEP 8
- Logical errors in the code
- Complexity issues
Installing Flake8
You can install Flake8 using pip:
bashCopyEditpip install flake8
Running Flake8
To check a Python file for errors and style violations, navigate to its directory and run:
bashflake8 filename.py
Flake8 will provide a concise output highlighting the line number, error code, and description of the issue.
Example output:
csharpfilename.py:3:10: E225 missing whitespace around operator
filename.py:8:5: F401 'os' imported but unused
Configuring Flake8
Flake8 supports customization through a configuration file (.flake8
). You can create this file in your project’s root directory and specify your preferences.
Example .flake8
file:
ini[flake8]
max-line-length = 100 # Set line length limit (default is 79)
ignore = E203, E266, E501 # Ignore specific error codes
exclude = venv/, migrations/ # Exclude directories
This configuration:
- Sets a maximum line length of 100 instead of the default 79.
- Ignores specific error codes such as E203, E266, E501.
- Excludes certain directories (venv/ and migrations/) from checks.
Using Flake8 with Plugins
Flake8 supports additional plugins for more functionality. Some useful plugins include:
- flake8-docstrings: Checks for missing or improper docstrings.
- flake8-bugbear: Detects common programming mistakes.
- flake8-import-order: Ensures imports follow best practices.
To install a plugin, use:
bashpip install flake8-docstrings
Then, enable it in .flake8
:
ini[flake8]
enable-extensions = D
3.3. Integrating Pylint and Flake8 with Your IDE
To make syntax checking more convenient, integrate these tools with your code editor:
VS Code
- Install the Python extension from the VS Code marketplace.
- Open settings (
Ctrl + ,
) and search for linting. - Enable Pylint and Flake8 under
"python.linting.enabled": true
. - Add configurations in
settings.json
:
jsonCopyEdit"python.linting.pylintEnabled": true,
"python.linting.flake8Enabled": true,
"python.linting.lintOnSave": true
PyCharm
- Go to Settings > Tools > External Tools.
- Add a new tool and configure it to run
pylint
orflake8
on save.
3.4. Choosing Between Pylint and Flake8
Both Pylint and Flake8 serve different purposes:
Feature | Pylint | Flake8 |
---|---|---|
Checks for syntax errors | ✅ | ✅ |
Follows PEP 8 style guide | ✅ | ✅ |
Provides coding suggestions | ✅ | ❌ |
Detects code complexity issues | ✅ | ✅ |
More customizable | ✅ | ❌ |
Faster execution | ❌ | ✅ |
- Use Pylint if you need detailed code analysis, error detection, and suggestions.
- Use Flake8 if you prefer a lightweight and fast tool focused on style and syntax errors.
For best results, you can use both together!
4. Using Python Syntax Checkers in Integrated Development Environments (IDEs)
Modern Integrated Development Environments (IDEs) offer built-in support for Python syntax checkers, helping developers catch errors, enforce coding standards, and maintain code quality. These tools provide real-time feedback, allowing you to identify and resolve syntax issues as you write your code. Below is a guide on how to integrate syntax checkers into some of the most popular Python IDEs.
4.1. Visual Studio Code (VS Code)
Visual Studio Code (VS Code) is a widely used, lightweight, yet powerful code editor with excellent Python support. It includes features like IntelliSense, debugging, and extensions that enhance coding productivity. To enable syntax checking in VS Code, follow these steps:
Step 1: Install the Python Extension
To get Python-specific features, install the Python extension from the VS Code Marketplace:
- Open VS Code.
- Go to Extensions (Ctrl+Shift+X).
- Search for “Python” and install the official extension by Microsoft.
Step 2: Enable and Configure Linting
Linting helps enforce code quality by flagging errors, warnings, and stylistic issues. To enable and configure a linter:
- Open the Command Palette (Ctrl+Shift+P).
- Search for “Python: Select Linter” and choose your preferred linter:
- Pylint (default, recommended)
- Flake8 (lighter, used for PEP8 compliance)
- mypy (for static type checking)
- Once selected, VS Code will automatically start linting your Python files.
Step 3: Real-Time Feedback
With linting enabled, VS Code will highlight syntax errors, style issues, and warnings in real time. Errors appear as red squiggly lines, while warnings appear in yellow. You can hover over them for details and suggestions.
Additional Customization (Optional)
For more customization, modify the settings.json file:
- Open Settings (Ctrl+,).
- Search for “Python Linting” and tweak options like enabling/disabling specific linters or adjusting rules.
4.2. PyCharm
PyCharm, developed by JetBrains, is a feature-rich IDE tailored for Python development. It provides powerful tools such as code inspections, refactoring, and built-in support for linters.
Step 1: Enable Code Inspections
PyCharm includes code inspections, which help detect syntax errors, warnings, and improvement suggestions. To enable them:
- Navigate to File > Settings > Editor > Inspections.
- Expand the Python section.
- Enable inspections for syntax errors, PEP8 compliance, and other coding issues.
Step 2: Configure a Linter (Pylint, Flake8, or mypy)
PyCharm supports multiple linters, including Pylint, Flake8, and mypy. To configure a linter:
- Open File > Settings > Tools > External Tools.
- Click Add, then specify the linter command (e.g.,
pylint <file>
). - Adjust arguments as needed to customize linter behavior.
Alternatively, you can install and configure linters via Preferences > Project: <Your Project> > Python Interpreter, where you can install Pylint or Flake8 directly into your project environment.
Step 3: Run Code Analysis
To inspect your code and view syntax suggestions:
- Select Code > Inspect Code from the menu.
- Choose the desired scope (file, directory, or project).
- Click OK, and PyCharm will generate a report highlighting errors and recommendations.
Step 4: Enable On-the-Fly Code Inspection
For real-time syntax checking, ensure “On-the-fly code analysis” is enabled under File > Settings > Editor > General > Code Completion. This will display syntax warnings and errors as you type.
4.3. Other Popular IDEs with Syntax Checking Support
Jupyter Notebook
- Jupyter Notebooks support real-time syntax checking through the
pyflakes
andflake8
packages.
- You can install them using:
bashpip install pyflakes flake8
- Use
%load_ext pycodestyle_magic
to enable inline linting within Jupyter cells.
Eclipse (with PyDev Plugin)
- PyDev for Eclipse offers built-in syntax checking.
- To enable it:
- Go to Window > Preferences > PyDev > Editor > Code Analysis.
- Enable Pylint and configure error highlighting.
Spyder
- Spyder includes Pylint for syntax checking.
- To enable it:
- Open Tools > Preferences > Editor > Code Introspection/Linting.
- Enable Pylint for real-time syntax feedback.
5. Best Practices for Using Python Syntax Checkers
To maximize the benefits of Python syntax checkers, consider the following best practices:
- Consistent Usage: Regularly run syntax checkers on your codebase to catch errors early and maintain code quality.
- Understand Warnings: Not all warnings require immediate fixes. Understand the context and prioritize critical issues.
- Follow Coding Standards: Adhere to coding standards like PEP 8 to ensure consistency and readability in your code.
- Automate Checks: Integrate syntax checkers into your Continuous Integration (CI) pipeline to automate code quality checks.
- Review Reports: Regularly review the reports generated by syntax checkers and address the issues identified.
6. Common Issues and How to Resolve Them
While using Python syntax checkers, you may encounter common issues. Here are a few examples and how to resolve them:
- False Positives: Sometimes, syntax checkers may flag valid code as an issue. Review the warning and suppress it if necessary.
- Performance Impact: Running syntax checkers on large codebases can be time-consuming. Use caching or configure checks to run selectively.
- Configuration Conflicts: Different projects may have varying coding standards. Ensure your linter configuration aligns with the project’s requirements.
Conclusion
Python syntax checkers are invaluable tools for improving code quality and ensuring adherence to best practices. By using these tools consistently and understanding their feedback, you can catch errors early, write cleaner code, and maintain high standards in your projects. Whether you’re a seasoned developer or just starting with Python, integrating syntax checkers into your workflow is a step toward becoming a more efficient and effective programmer. If you’re looking to deepen your understanding of Python, Python Online Training with Certification from H2K Infosys can help you master the language and best practices, giving you the skills you need to take your programming to the next level.