Python is one of the most widely used programming languages today, celebrated for its ease of use and flexibility. Whether you’re a beginner looking to learn programming or an experienced developer wanting to enhance your skills, understanding Python Tutorial is essential. This python tutorial will cover the basics of Python, its applications, and how to get started with online courses, including Python certification options.
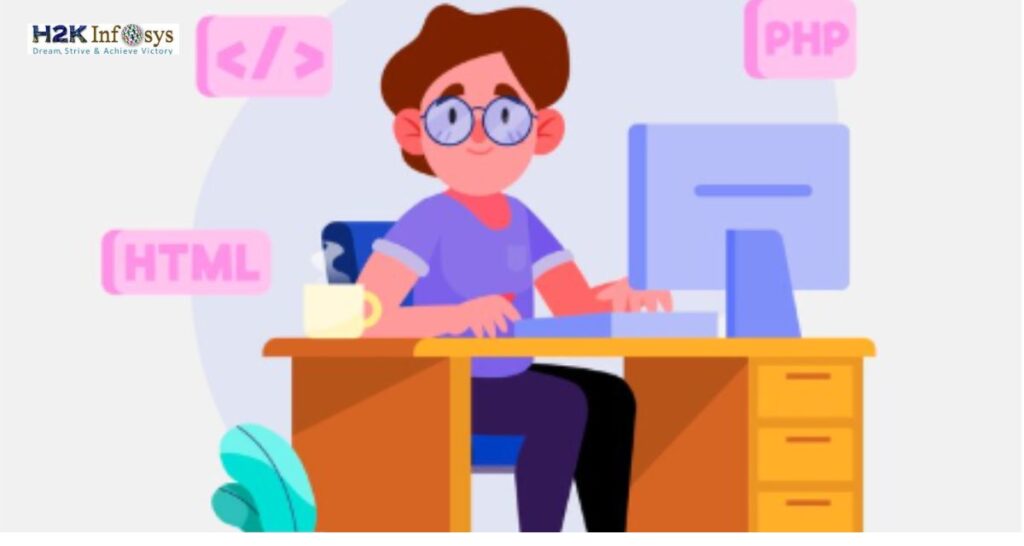
Why need to take a Python Tutorial?
Python’s popularity stems from its readability and ease of use. It is widely used in various fields such as web development, data analysis, artificial intelligence, machine learning, and more. Learning Python can open up numerous career opportunities, making it a valuable skill in today’s job market.
Key Features of Python Tutorial for Beginners
Some key features of a Python tutorial tailored for beginners:
- Easy to Read and Write: It uses simple syntax that resembles English, making it accessible for beginners.
- Versatile: it can be used for various applications, from web development to scientific computing.
- Large Community: With a vast community of developers, finding resources, libraries, and support is easier than ever.
- Rich Ecosystem: Python tutorial offers a plethora of libraries and frameworks that simplify complex tasks.
Getting Started with Python
Installation
To begin coding in Python tutorial, you must first download and install it on your device.
- Download Python: Visit the official Python website and download the latest version.
- Install Python: Install Python: Run the installer and follow the instructions. Make sure to check the box that says “Add Python to PATH.
- Verify Installation: Open your command prompt (Windows) or terminal (Mac/Linux) and type
python --version
to check if Python is installed correctly.
Writing Your First Program
Once you have installed Python, you can write your first program:
Open a text editor (like Notepad or VSCode).
Write the following code:
print("Hello, World!")
Save the file as hello.py
.
Run the program by typing python hello.py
in your command prompt or terminal.
Basic Concepts of Python
Variables and Data Types
In this , variables are used to store data values. You don’t need to declare the variable type explicitly; Python infers it automatically.
# Example of variables
name = "Alice" # String
age = 30 # Integer
height = 5.5 # Float
is_student = True # Boolean
Control Flow
Control flow statements allow you to dictate the flow of your program based on conditions.
# Example of an if statement
if age > 18:
print("You are an adult.")
else:
print("You are a minor.")
Loops
Loops allow you to execute a block of code multiple times.
# Example of a for loop
for i in range(5):
print(i)
Functions
Functions are reusable pieces of code that perform a specific task.
def greet(name):
return f"Hello, {name}!"
print(greet("Alice"))
Practical Applications of Python tutorial
1. Web Development
Python is widely used for building web applications, thanks to its simple syntax and powerful frameworks. It allows developers to create complex web applications quickly and efficiently.
- Django: A high-level framework that encourages rapid development and clean, pragmatic design. It is well-suited for large-scale applications like e-commerce websites, content management systems, and social media platforms.
- Flask: A micro-framework that is lightweight and flexible. It’s ideal for smaller projects or applications where you need more control over the components you use.
Example: You could build a blog platform or an online marketplace using Django, or a simple API server with Flask.
2. Data Analysis
Python has become the go-to language for data science, with powerful libraries such as Pandas, NumPy, and Matplotlib that make data manipulation, analysis, and visualization seamless.
- Pandas: Provides data structures and functions needed to manipulate structured data (e.g., CSV, Excel, SQL). It’s essential for cleaning, filtering, and analyzing large datasets.
- NumPy: A library for numerical computing that offers powerful array structures and functions for performing complex mathematical operations.
- Matplotlib: A library for creating static, animated, and interactive visualizations in Python. It’s commonly used to generate graphs and charts to help understand data.
Example: You could analyze financial data, create visualizations of market trends, or process large datasets from social media or scientific research.
3. Machine Learning
Python has become a dominant language in machine learning and artificial intelligence (AI), thanks to its libraries such as TensorFlow, Keras, Scikit-learn, and PyTorch.
- Scikit-learn: A machine learning library that includes simple tools for data mining and data analysis. It supports various algorithms for classification, regression, clustering, and dimensionality reduction.
- TensorFlow and Keras: These are deep learning libraries that provide high-level APIs for building neural networks and complex machine learning models.
- PyTorch: A deep learning library developed by Facebook that is popular for research and production models, particularly in natural language processing (NLP) and computer vision.Example: You could create a predictive model for customer behavior, build a recommendation system, or train a deep learning model for image recognition or sentiment analysis.
4. Automation
Python is widely used for automating repetitive tasks, from simple file handling to web scraping and even automating entire workflows. Popular libraries for automation include Selenium, Beautiful Soup, PyAutoGUI, and APScheduler.
- Selenium: A tool for automating web browsers. It’s often used for web scraping and automating interactions with websites.
- Beautiful Soup: A Python library for parsing HTML and XML documents, commonly used for web scraping.
- PyAutoGUI: A library that provides functions for automating GUI operations such as mouse movements, clicks, and keyboard presses.
- APScheduler: A Python library for scheduling tasks to run at specified intervals (e.g., daily, weekly).Example: You could automate the process of downloading reports from websites, interact with applications to fill forms or process files, or scrape data from web pages for research purposes.
5. Scientific and Numeric Computing
Python is widely used in scientific research, engineering, and finance due to libraries that enable complex computations, simulations, and numerical analysis.
- SciPy: A library built on NumPy for scientific and technical computing. It includes modules for optimization, integration, interpolation, eigenvalue problems, and more.
- SymPy: A symbolic mathematics library used for algebraic manipulations, calculus, and equation solving.
- Matplotlib and Seaborn: For visualizing data in scientific plots and statistical graphs.Example: You could simulate physical processes, perform statistical analysis on experimental data, or build mathematical models for engineering systems.
6. Game Development
Python is used in game development, particularly for scripting and creating simple games. The Pygame library is widely used for creating 2D games.
- Pygame: A set of Python modules designed for writing video games. It includes computer graphics and sound libraries, making it easy to create interactive games.
Example: You could create a simple 2D game like a puzzle game, or develop the logic for more complex games using Python.
7. Networking
Python can be used for network programming, including creating server-client applications, handling protocols, and automating network tasks.
- Socket Programming: Python’s
socket
library is used to create server-client applications, enabling communication between machines over a network. - Twisted: A popular event-driven networking engine that allows the development of high-performance network applications.
- Paramiko: A library for SSH2 protocol, commonly used for automating remote server interactions.Example: You could write a simple chat application, automate server monitoring tasks, or build a file-sharing service.
8. Cybersecurity
Python is also widely used in the cybersecurity domain, both for building security tools and for penetration testing and vulnerability assessment.
- Scapy: A library used for packet manipulation, building and dissecting network packets, and performing security tests.
- Requests: A library for making HTTP requests, often used in web scraping, security testing, and automating HTTP-based interactions.
- Paramiko: Used to automate secure communication between computers via SSH.Example: You could build a tool for scanning open ports on networks, creating a password manager, or perform security audits on web applications.
9. Internet of Things (IoT)
Python is used for programming embedded systems and IoT devices due to its simplicity and powerful libraries. MicroPython and CircuitPython are two specialized versions of Python that work on microcontrollers.
- MicroPython: A lean implementation of Python designed for microcontrollers. It allows you to write Python code that runs directly on hardware devices like Raspberry Pi or Arduino.
- CircuitPython: Similar to MicroPython, it is optimized for beginners and used on devices like Adafruit’s microcontrollers.
Example: You could use Python to control sensors, build smart home devices, or create connected devices that gather and transmit data.
10. Desktop Application Development
Python is used for building cross-platform desktop applications. Frameworks like Tkinter, PyQt, and Kivy provide tools for creating graphical user interfaces (GUIs).
Kivy: A library for building multi-touch applications, supporting a wide range of devices (e.g., phones, tablets, desktops).Example: You could build a desktop calculator, a file manager, or a photo editing application using Python.
Tkinter: A standard GUI library that comes with Python, useful for creating basic desktop applications with windows, buttons, and other GUI components.
PyQt: A set of Python bindings for the Qt application framework, offering tools for creating more complex and professional-looking desktop applications.
Learning Resources
To master Python effectively, consider enrolling in an online course. Here are some excellent options:
Best Python Certification Courses
Python Certification Course by H2K Infosys
H2K Infosys offers a comprehensive Python Certification Course that provides 100% job-oriented training. The course includes real-time project work, ensuring that you gain hands-on experience with Python programming. This course is designed for learners of all levels, from beginners to experienced professionals, making it one of the best options available.
Online Python Course with Job Guarantee
This course focuses on practical skills that are in high demand in the job market. It includes real-world projects and offers job placement assistance upon completion, making it an excellent choice for those looking to secure a position in the tech industry.
Google IT Automation with Python Professional Certificate
This beginner-level program consists of six courses designed to help you learn Python Tutorial and apply it to automate tasks. It is ideal for IT professionals looking to enhance their automation skills and comes with a certification upon completion.
Conclusion
Python Tutorial opens up numerous possibilities in various fields such as web development, data science, automation, and more. With its straightforward syntax and vast community support, it’s an excellent choice for both beginners and experienced developers alike.By enrolling in a Python Online Course, you can enhance your skills while earning valuable certifications that will boost your career prospects. Whether you choose a course with job guarantees or one focused on certification, investing time in learning Python will yield significant benefits in your professional journey.
Key Takeaways
- Start Small: Begin with basic concepts before moving on to advanced topics.
- Build Projects: Apply what you’ve learned by working on real-world projects.
- Consider Certification: A Python Certification can enhance your resume significantly.
Ready to dive into the world of programming? Start your journey today by enrolling in a Python training course!
2 Responses