Salesforce is one of the most popular customer relationship management (CRM) platforms that businesses rely on for sales, service, and marketing operations. Python, known for its simplicity and versatility, is a widely used programming language that offers an efficient way to integrate and interact with Salesforce data. With the growing demand for professionals who can automate processes and manage data, learning Salesforce integration using Python is highly beneficial, especially if you’re pursuing a Salesforce certification or looking to become a Salesforce Administrator.
In this blog, we will take you through a step-by-step guide on how to integrate Salesforce using Python. Whether you are already familiar with Python or a newcomer seeking a Salesforce certification like SFDC Salesforce certification, this guide will help you enhance your integration skills.
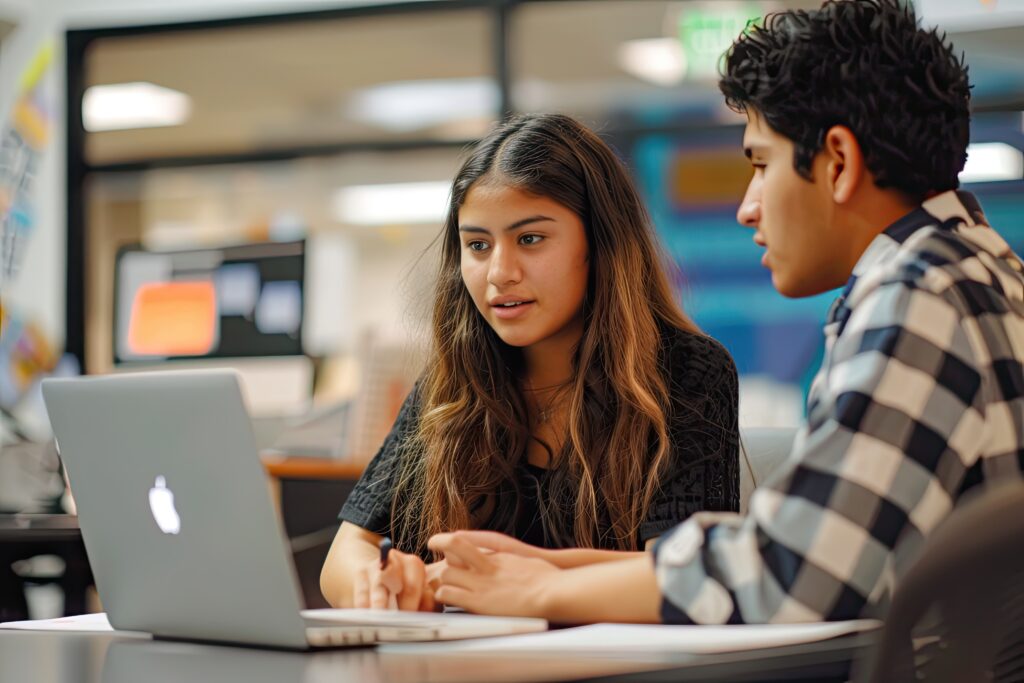
Why Integrate Salesforce Using Python?
Before diving into the steps, let’s understand the benefits of using Python for Salesforce integration:
- Automation of Tasks: Integrating Salesforce with Python helps automate repetitive tasks like data updates, queries, and record creation.
- Custom API Interactions: Python allows easy communication with Salesforce’s API, enabling custom functionalities tailored to your organization’s needs.
- Data Handling Efficiency: With Python’s ability to process and manipulate data efficiently, integrating Salesforce helps manage bulk data operations, updates, and analytics.
These capabilities can significantly boost productivity and are key aspects of learning during your Salesforce certification training, especially if you’re preparing for roles like Salesforce Administrator.
Step 1: Set Up Your Salesforce Developer Account
To get started with Salesforce integration, you’ll need access to a Salesforce Developer Account. This account allows you to experiment and test integration without impacting production data. Follow these steps to create an account:
- Sign up for a free Salesforce developer account.
- Verify your email and log into the Salesforce dashboard.
- Navigate through the platform to familiarize yourself with key Salesforce objects such as Leads, Accounts, and Opportunities.
This setup is crucial for hands-on experience if you are pursuing any Salesforce certification, as practical knowledge of Salesforce APIs and objects is often tested.
Step 2: Install Python and Required Libraries
Next, you’ll need to install Python and the necessary libraries for Salesforce integration. If you don’t already have Python installed, you can download and set it up. Once Python is installed, follow these steps:
- Install Python Libraries: To connect and interact with Salesforce, you’ll require some Python libraries. The two most important ones are:
simple_salesforce
: A library that simplifies interaction with Salesforce APIs.requests
: A library to handle HTTP requests.
To install these, use the following commands:
bash
pip install simple_salesforce
pip install requests
These libraries will help streamline the process of connecting to Salesforce, making API calls, and handling responses. Mastery of these tools is essential for those working towards Salesforce certifications like SFDC Salesforce certification.
Step 3: Generate Salesforce API Credentials
To enable Python to communicate with Salesforce, you’ll need to generate API credentials. Here are the steps:
- Generate Security Token: In Salesforce, go to your settings and look for the option to reset your Security Token. This token will be sent to your registered email.
- Create a Connected App: In Salesforce, navigate to Setup, search for App Manager, and create a new connected app. This will give you the credentials (Client ID and Client Secret) needed for authentication.
- Enable OAuth settings and select required scopes (such as
api
andfull access
). - Once created, take note of the Client ID and Client Secret.
- Enable OAuth settings and select required scopes (such as
These credentials are necessary to access Salesforce APIs and automate tasks using Python.
Step 4: Authenticating with Salesforce via Python
Now that you have your Salesforce API credentials and Python set up, it’s time to authenticate Salesforce with Python. Authentication is the first step to making API requests to Salesforce.
Here’s a basic Python script for authentication:
python
from simple_salesforce import Salesforce
# Salesforce login credentials
username = '[email protected]'
password = 'your_password'
security_token = 'your_security_token'
# Connect to Salesforce
sf = Salesforce(username=username, password=password, security_token=security_token)
print(sf)
This script connects to Salesforce and prints the session details upon successful authentication. As you prepare for a Salesforce certification or work towards a Salesforce administrator role, mastering Salesforce authentication using Python is a crucial skill.
Step 5: Querying Salesforce Data with Python
Once authenticated, you can start querying Salesforce data using Python. Salesforce uses SOQL (Salesforce Object Query Language) to retrieve data from its objects. Here’s how you can query data:
python
# Query Salesforce to get a list of Account records
query = "SELECT Id, Name FROM Account LIMIT 10"
accounts = sf.query(query)
# Display the fetched records
for account in accounts['records']:
print(account['Id'], account['Name'])
This query retrieves the first 10 Account records from Salesforce and prints their IDs and Names. Querying data is fundamental in managing Salesforce information, and understanding how to construct SOQL queries is part of any Salesforce administrator training.
Step 6: Creating and Updating Salesforce Records
With Python, you can not only retrieve data but also create and update Salesforce records. Let’s see how you can automate these processes.
Creating a Salesforce Record
Here’s an example of how to create a new Account record in Salesforce:
python
# Create a new Account in Salesforce
new_account = sf.Account.create({
'Name': 'Python Integration Corp'
})
print(f"New Account Created with ID: {new_account['id']}")
This script creates a new account named “Python Integration Corp” and prints the ID of the newly created record.
Updating a Salesforce Record
To update an existing Salesforce record, you need the record’s ID. Here’s how you can update a record:
python
# Update an existing Account
sf.Account.update('record_id', {
'Name': 'Updated Python Corp'
})
With this functionality, you can automate updates for Salesforce records, such as Leads, Contacts, or Opportunities.
Step 7: Error Handling in Salesforce API Calls
Error handling is an important part of API integration. If something goes wrong—such as an authentication error or incorrect API call—it’s important to handle these situations gracefully.
Here’s an example of how to handle errors when making Salesforce API calls:
python
try:
# Attempt to create a new Account
new_account = sf.Account.create({
'Name': 'New Business Account'
})
except Exception as e:
print(f"An error occurred: {e}")
This script ensures that any errors encountered during the API call are caught and printed, allowing for easier debugging and troubleshooting. Learning how to handle API errors is crucial for anyone pursuing Salesforce certification and can be a game-changer for automating processes as a Salesforce Administrator.
Step 8: Automating Salesforce Tasks with Python
One of the most powerful features of integrating Salesforce with Python is the ability to automate tasks. By using Python scripts, you can:
- Automate Reports: Query Salesforce data and generate automated reports.
- Bulk Data Uploads: Upload bulk records such as leads or opportunities directly into Salesforce.
- Scheduled Tasks: Automate processes like sending email alerts when certain conditions in Salesforce are met.
Automation using Python will streamline repetitive tasks, increase productivity, and significantly reduce manual data handling, which is highly relevant for anyone studying for SFDC Salesforce certification.
Step 9: Testing and Deploying the Integration
Once you’ve successfully created and tested your integration in a developer environment, you can deploy your Python scripts into production. For production environments, ensure that:
- API Limits: Salesforce has API usage limits, so be mindful of the number of requests made.
- Security: Keep your credentials safe and secure, and avoid hardcoding sensitive information in your scripts.
- Error Logging: Implement robust error logging mechanisms to monitor and debug issues in production.
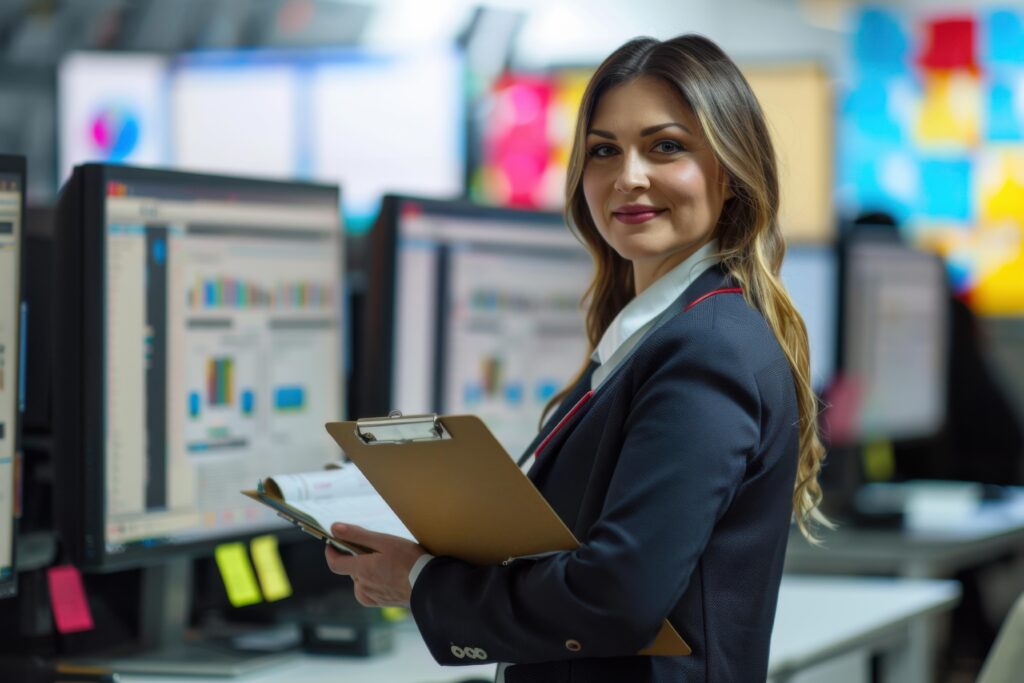
Conclusion
Integrating Salesforce with Python is an essential skill for automating workflows, managing data, and streamlining business processes. From authenticating with Salesforce to querying data and automating tasks, Python makes it easier to interact with Salesforce’s API. For professionals seeking a Salesforce certification or aiming to become a Salesforce administrator, mastering Python integration opens up a wide array of possibilities for automation and data management.
Incorporating this knowledge into your skillset can significantly improve your ability to handle Salesforce’s vast capabilities. Whether you are preparing for an SFDC Salesforce certification or planning to advance your career as a Salesforce Administrator, these steps will provide the foundation for successful Salesforce integration using Python.
One Response