What is Selenium Framework?
Selenium Automation Frameworks are a code structure that helps maintain the code in an organized and efficient manner. Without frameworks, we would place the developed code and the provided data in the same location, making it neither re-usable nor readable. Selenium Automation Frameworks offer several benefits, such as increased re-usage of code, higher portability, reduced maintenance costs, and improved code readability. These advantages make frameworks essential for optimizing automation testing processes.
Selenium WebDriver created three type of frameworks to automate manual test cases
- Keyword Driven Framework
- Data Driven Framework
- Hybrid Framework
Data Driven Framework
In data driven framework all our test data is generated from external files like CSV, XML, Excel or some database table.
If we want to read or write in an Excel, Apache provides a very famous library called POI. This library will read and write both XLS and XLSX file format of Excel.
An HSSF implementation is provided by POI library to read XLS files. Implementation of POI library will be the choice to read XLSX, XSSF.
Keyword Driven Test Framework:
In Excel worksheet we will write all the operations and instructions in Keyword driven framework.
Components of Keyword Driven Framework
The list of components are involved in Keyword Driven Framework.
- Function Library
- Excel Sheet to store Keywords
- Design Test Case Template
- Object Repository for Elements/Locators
- Test Scripts or Driver Script
Function Library:
This is a Java class file where we define keywords.
For Example:
Let us perform the following actions in one or more test cases:
- Enter the URL.
- Click on an element.
- Type in a textbox.
Then, the library file is created by defining individual methods for all the actions
Here, we will create a user-defined method for the action by āEntering the URLā.
Keyword is the name provided for the user-defined method.
So, here āenter_URLā is the Keyword
public void enter_URL(WebDriver driver,String TestData) throws IOException
{
driver.get(TestData);
}
Parameters:
Driver: In the Main Class the driver is initialized and is passed in here.
TestData: It is read from the external source by the Main Class and passed it in here.
Function:
Here, driver.get(): is the function that performs the action āenter URLā.
Keywords.java
package Keywords;
import java.io.File;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
public class Keywords {
String path = System.getProperty("user.dir");
WebDriver driver;
public void enter_URL(WebDriver driver,String TestData) throws IOException
{
driver.get(TestData);
}
public void type(WebDriver driver, String typeLocator, String testdata, String ObjectName)
{
driver.findElement(this.getObject(ObjectName,typeLocator)).sendKeys(testdata);
}
public void click(WebDriver driver,String ObjectName, String typeLocator)
{
driver.findElement(this.getObject(ObjectName,typeLocator)).click();
}
}
Excel Sheet to Store Keywords
All the user-defined methods along with its functionality details are maintained in the excel sheet so that the user can easily understand what Keyword the library file holds.
package Keywords;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.util.Properties;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class Keywords {
String path = System.getProperty("user.dir");
WebDriver driver;
public void enter_URL(WebDriver driver,String TestData) throws IOException{
driver.get(TestData);
}
public void type(WebDriver driver, String typeLocator, String testdata, String ObjectName) throws IOException{
driver.findElement(this.getObject(ObjectName,typeLocator)).sendKeys(testdata);
//driver.findElement(By.xpath("//")).sendKeys(testdata);
}
public void wait(WebDriver driver,String ObjectName, String typeLocator) throws IOException{
WebDriverWait wait = new WebDriverWait(driver, 60);
wait.until(ExpectedConditions.visibilityOf(driver.findElement(this.getObject(ObjectName,typeLocator))));
}
public void click(WebDriver driver,String ObjectName, String typeLocator) throws IOException{
driver.findElement(this.getObject(ObjectName,typeLocator)).click();
}
public String get_currentURL(WebDriver driver){
String URL = driver.getCurrentUrl();
System.out.println("print URL "+URL);
return URL;
}
By getObject(String ObjectName, String typeLocator) throws IOException{
File file = new File(path+"\\Externals\\Object Repository.properties");
FileInputStream fileInput = new FileInputStream(file);
Properties prop = new Properties();
//find by the xpath
if(typeLocator.equalsIgnoreCase("XPATH")){
return By.xpath(prop.getProperty(ObjectName));
}
//find by the class
else if(typeLocator.equalsIgnoreCase("CLASSNAME")){
return By.className(prop.getProperty(ObjectName));
}
//find by name locator
else if(typeLocator.equalsIgnoreCase("NAME")){
return By.name(prop.getProperty(ObjectName));
}
//Find by css lcatoor
else if(typeLocator.equalsIgnoreCase("CSS")){
return By.cssSelector(prop.getProperty(ObjectName));
}
//find by the link
else if(typeLocator.equalsIgnoreCase("LINK")){
return By.linkText(prop.getProperty(ObjectName));
}
//find by the partial link
else if(typeLocator.equalsIgnoreCase("PARTIALLINK")){
return By.partialLinkText(prop.getProperty(ObjectName));
}
return null;
}
}
Design Test Case Template
Test Case Template can be created as per our project convenience. The externalization can be only for Keywords, or even for Test Data and UI Elements are also externalized.
A sample test case template is created:
In the given example, the template is created as follows:
- Each sheet corresponds to a Test Case and the last sheet holds the āKeywords listā.
- Each row corresponds to the test steps of a TC.
- Each Column is the parameters that are necessary for each action.
Object Repository for Locators
UI locator can be identified and its value can be mentioned in the test case template or maintained in a separate Object Repository.
In the below example, the element identification properties
- Locator Type ā The identification used is id, Xpath, ClassName, etc.
- Locator Value ā Value of that attribute ā For Example: If its Id attribute, then the value of id and so on.
Test Scripts or Driver Script
package KeywordFramework;
import java.io.FileInputStream;
import java.io.IOException;
import java.util.LinkedList;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.xssf.usermodel.XSSFSheet;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.testng.annotations.Test;
import org.testng.asserts.Assertion;
import Keywords.Assertions;
import Keywords.Keywords;
public class Irctc {
WebDriver driver;
String path = System.getProperty("user.dir");
Keywords keyword = new Keywords();
Assertions assertion = new Assertions();
@Test
public void readExcelandexecute() throws IOException, InterruptedException{
String excelFilePath = path+"\\Externals\\Test Cases.xlsx";
FileInputStream fileInputStream = new FileInputStream(excelFilePath);
XSSFWorkbook workbook = new XSSFWorkbook(fileInputStream);
int testcasescount = workbook.getNumberOfSheets()-1;
System.out.println("Total no of test cases :"+testcasescount);
for (int testcase=0;testcase<testcasescount;testcase++){
System.setProperty("webdriver.gecko.driver","F:\\drivers\\geckodriver.exe");
driver = new FirefoxDriver();
XSSFSheet worksheet = workbook.getSheetAt(testcase);
System.out.println("worksheet Number "+testcase+":"+worksheet.getSheetName());
int row = worksheet.getLastRowNum();
int column = worksheet.getRow(1).getLastCellNum();
for(int i=1;i<=row;i++){
LinkedList<String> Testexecution = new LinkedList<>();
System.out.println("Row value :"+i+"First cell value as : "+worksheet.getRow(i).getCell(0));
for(int j=0;j<column-1;j++){
System.out.println("Column index :"+j);
Cell Criteria = worksheet.getRow(i).getCell(j);
String CriteriaText;
if(Criteria==null){
CriteriaText = null;
}else{
CriteriaText = Criteria.getStringCellValue();
}
Testexecution.add(CriteriaText);
}
System.out.println("List :"+Testexecution);
String TestStep = Testexecution.get(0);
String ObjectName = Testexecution.get(1);
String TypeLocator = Testexecution.get(2);
String Testdata = Testexecution.get(3);
String AssertionType = Testexecution.get(4);
String ExpectedValue = Testexecution.get(5);
String ActualValue = Testexecution.get(6);
perform(TestStep,ObjectName,TypeLocator,Testdata,AssertionType,ExpectedValue,ActualValue);
System.out.println("Row"+i+" is read and action performed");
}
driver.close();
System.out.println("****TEST CASE "+worksheet.getSheetName()+" is executed*****");
}
}
public void perform(String operation, String objectName, String typeLocator, String testdata,
String assertionType, String expectedValue, String actualValue) throws IOException, InterruptedException {
switch (operation) {
case "enter_URL":
//Perform click action
keyword.enter_URL(driver,testdata);
break;
case "get_currentURL":
keyword.get_currentURL(driver);
break;
case "type":
keyword.type(driver, objectName, typeLocator, testdata);
case "click":
keyword.click(driver, objectName, typeLocator);
case "implicitWait":
Thread.sleep(8000);
default:
break;
}
if(operation.contains("AssertURL")){
switch(assertionType){
case "contains":
assertion.AssertURLContains(driver, keyword.get_currentURL(driver), expectedValue);
case "equals":
assertion.AssertURLEquals(driver, keyword.get_currentURL(driver), expectedValue);
}
}
if(operation.contains("AssertElement"){
assertion.AssertElement(driver, assertionType, objectName, typeLocator);
}
}
}
Hybrid Test Framework
Hybrid Driven Framework is a combination of both the Data-Driven and Keyword-Driven framework. Here, the keywords, the test data, are externalized. Keywords are maintained in a separate Java class file and test data can be maintained either in properties file or excel file or can use the data provider of a TestNG framework.
Components of Hybrid Driven Framework
Components of the Hybrid Framework are similar as Keyword Driven Framework wherein every Test Data, Keywords, are externalized making the script in a more generalized form
- Function Library
- Excel Sheet to store Keywords
- Design Test Case Template
- Object Repository for Elements/Locators
- Test Scripts or Driver Script
Function Library
User-defined methods are created for each user action. In other words, Keywords are created in the library file.
For Example: Let us automate the below test cases.
Test Case No | Description | Test Steps | Expected Result |
1 | Verify Amazon logo | 1. Enter URL – https://www.amazon.com | Amazon logo should be displayed in home page |
2 | Verify valid signIn | 1. Enter URL – https://www.amazon.com 2. Click on ‘SignIn’ link 3. Enter valid Email 4. Click on continue 5. Enter Valid Password 6. Click on SignInButton | User Icon should be present in Homepage |
3 | Invalid login | 1. Enter URL – https://www.amazon.com 2. Click on ‘SignIn’ link 3. Enter invalid Email 4. Click on continue | This error message should contain ‘cannot find an account’ |
First, the test cases are analyzed and note down its actions.
In TC 01: Verify Amazon logo which is present- the user actions will be: Enter the URL
In TC 02: Verify Valid SignIn- the user actions are Enter URL, Click, TypeIn
In TC03: Verify Invalid Login- the user actions are Enter URL, Click, TypeIn
Now, for each action the library file will be created with Keywords defined as below:
Keywords.java
Excel Sheet to store Keywords
Keywords that we created in the library file are stored in an excel sheet with description to understand the framework easily for anyone who uses it.
Test Case Design Template
For the framework we need to create the Test Case template. Both Test Data and Keywords should be externalized in Hybrid Framework, So we need to create the template accordingly. For example:
For Test Case 2 ā Verify valid SignIn
Test Steps | Locator Type | Locator Value | TestData | AssertionType | Expected Value |
Enter_URL | www.amazon.com | ||||
Click | Xpath | //div[contains(@id,’SignIn’)] | |||
typeIn | Xpath | //div[contains(@id,’SignIn’)] | [email protected] | ||
Click | Xpath | //div[contains(@id,’continue’)] | |||
typeIn | Id | Password | Password@123 | ||
Click | Id | SignIn | |||
AssertElement | Xpath | //div[contains(@id,’usericon’)] | Displayed |
In the same way we create test steps for each test case in a separate sheet.
Object Repository for Elements
For all elements on the webpage we maintain a separate Repository. Each WebElement is referred with a name followed with its value. Test Case template will hold the Object Name and from the repository its value is taken as shown below:
Test Steps | Locator Type | Locator Value | TestData | AssertionType | Expected Value |
Enter_URL | www.amazon.com | ||||
Click | Xpath | LoginLink | |||
typeIn | Xpath | EmailTextbox | [email protected] | ||
Click | Xpath | ContinueButton | |||
typeIn | Id | PasswordTextbox | Password@123 | ||
Click | Id | SignInButton | |||
AssertElement | Xpath | UserIcon | Displayed |
Here LoginLink is the name of the object and from OR.properties we read its value as ā//div[contains(@id,āSignInā)]
Accordingly from ObjectRepository we read the value from the code and will be included in the script Keywords.java
For Example:
Library file: In Keywords.java consider the āclickā action
package Keywords;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.util.Properties;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class Keywords {
public void click(WebDriver driver, String ObjectName, String typeLocator)
throws IOException{
driver.findElement(this.getObject(ObjectName, typeLocator)).click();
}
By getObject(String ObjectName, String typeLocator) throws
IOException{
//Object Repository is opened
File file = new File(path+"\\Externals\\Object Repository.properties");
FileInputStream fileInput = new FileInputStream(file);
//Properties file is read
Properties prop = new Properties();
if(typeLocator.equalsIgnoreCase("XPATH")){
return By.xpath(prop.getProperty(ObjectName));
// ObjectName is read and its value is returned
}
else if(typeLocator.equalsIgnoreCase("ID")){
return By.id(prop.getProperty(ObjectName));
// ObjectName is read and its value is returned
}
else if(typeLocator.equalsIgnoreCase("NAME")){
return By.name(prop.getProperty(ObjectName));
// ObjectName is read and its value is returned
}
return null;
}
}
Object Repository for Test Data in Test Cases
- Externalizing Test Data from Test case Template:
Test Steps | Locator Type | Locator Value | TestData | AssertionType | Expected Value |
Enter_URL | URL | ||||
Click | Xpath | LoginLink | |||
typeIn | Xpath | EmailTextbox | |||
Click | Xpath | ContinueButton | |||
typeIn | Id | PasswordTextbox | password | ||
Click | Id | SignInButton | |||
AssertElement | Xpath | UserIcon | Displayed |
Similarly the test data is also read from the properties file.
Object Repository for test data
General data like Browser name, executable driver location, test case filename, etc can also be externalized in a separate repository.
In the above example, the browser parameter is externalized in a properties file ā Basic.properties.
- Passing Test Data from TestNG Suite:
TestData can be also be passed from a suite file of TestNG to the method.
We use a <parameter> tag in the TestNG.xml file above the class.
Syntax: <parameter name = āany_nameā value=āvalue_to_be_passedā/>
Once the testing suite is specified with the parameter name and its value, annotations are used in the script to specify which method uses the value. This is specified using the @Parameters annotation.
Syntax: @Parameters({āvalue_to_be_passedā})
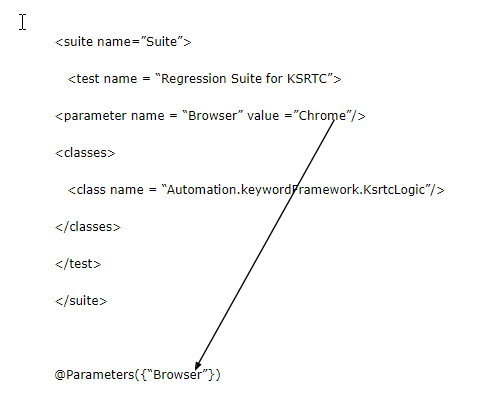
Driver Script
This contains the main logic from the test case template excel sheet and it will read all the test cases and performs the corresponding action by reading from the library file. The script is designed based on the test case template created.
package HybridFramework;
import java.lang.reflect.Method;
public class DriverScript
{
public static Actions actionKeywords;
public static String sActions;
//This is reflection class object and declared as 'public static'
//and it can be used outside the main[] method
public static Method method[];
public static void main(String[] args) throws Exception
{
//Declaring the path of the Excel file with the name of the Excel file
String sPath = "C:\\Users\\qwerty\\workspace\\Selenium Frameworks \\data.xlsx";
//Here we are passing the path of the excel file and SheetName to connect with the Excel file
ReadExcelData.setExcelFile(sPath, "Sheet1");
for (int iRow=1;iRow<=9;iRow++)
{
sActions = ReadExcelData.getCellData(iRow, 1);
execute_Actions();
}
}
private static void execute_Actions() throws Exception
{
//Here we are instantiating a new object of class 'Actions'
actionKeywords = new Actions();
//It will load all the methods of the class 'Actions' in it.
method = actionKeywords.getClass().getMethods();
//It will run for the number of actions in the Action Keyword class
for(int i = 0;i<method.length;i++)
{
if(method[i].getName().equals(sActions))
{
method[i].invoke(actionKeywords);
break;
}
}
}
}
Example: Test Case creation in Framework using POM
Step1: Create a class BrowserFactory
package com.automation.utility;
import org.openqa.selenium.WebDriver;
public class BrowserFactory {
public static WebDriver startApplication(WebDriver driver,String browserName, String appURL)
{
if(browserName.equals(āchromeā))
{
System.setProperty(āwebDriver.chrome.driverā,ā./Drivers/chromedriver.exeā);
driver = new ChromeDriver();
driver.manage().timeouts().pageLoadTimeout(20, TimeUnit.SECONDS);
}
else if(browserName.equals(āFirefoxā))
{
System.setProperty(āwebDriver.gecko.driverā,ā./ Drivers/geckodriver.exeā);
driver = new FirefoxDriver();
}
else if(browserName.equals(āIEā))
{
System.setProperty(āwebDriver.ie.driverā,ā./ Drivers/IEDriverServer.exeā);
driver = new InternetExplorerDriver();
}
else
{
System.out.println(āWe do not support this browserā);
}
driver.manage().timeouts().pageLoadTimeout(20, TimeUnit.SECONDS);
driver.manage().window().maximize();
driver.get(appURL);
driver.manage().timeouts().implicitlyWait(30,TimeUnit.SECONDS);
return driver;
}
public static void quitBrowser(WebDriver driver)
{
driver.quit();
}
}
Step2: Create a New Class LoginTestCRM
package com.automation.testcases;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.support.PageFactory;
import org.testng.annotations.Test;
import com.automation.pages.LoginPage;
import com.automation.utility.BrowserFactory;
public class LoginTestCRM extends BaseClass {
@Test
Public void loginApp()
{
LoginPage loginpage = PageFactory.initElements(driver, LoginPage.class);
loginPage.loginToCRM(āSeleniumā, āAbcd@123456ā);
}
}
Step 3: Create a New Class LoginPage
package com.automation.pages;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.support.FindBy;
public class LoginPage {
WebDriver driver;
public LoginPage(WebDriver ldriver)
{
this.driver=ldriver;
}
@FindBy(name=āusernameā) WebElement uname;
@FindBy(name=āpasswordā) WebElement pass
@FindBy(xpath=ā//input[@value=āLoginā]ā) WebElement loginButton;
Public void loginToCRM(String usernameApplication, String passwordApplication)
{
uname.sendKeys(xxxzbc);
pass.sendKeys(xxxxx);
loginButton.click();
}
}
Step4: Create a class (BaseClass)
package com.automation.pages;
import org.openqa.selenium.WebDriver;
import org.testng.annotations.AfterClass;
import org.testng.annotations.BeforeClass;
import com.automation.utility.BrowserFactory;
public class BaseClass {
public WebDriver driver;
@BeforeClass
public void setup()
{
driver= BrowserFactory.startApplication(driver, āChromeā, āhttps://www.freecrm.com/index.htmlā);
}
@AfterClass
public void teardown()
{
BrowserFactory.quitBrowser(driver);
}
}
Right-click on LoginTestCRM class and Run the application.
Conclusion:
- In Selenium WebDriver we can create three types of frameworks.
- They are Data Driven, Keyword Driven, and Hybrid Driven framework.
- Using TestNG’s data provider we can achieve Data-driven framework
- Keywords are written in some external files like excel file and java code will call this excel file and execute the test cases in Keyword driven framework,.
- The hybrid framework is a combination of keyword driven and data driven framework.

In conclusion, mastering Data-Driven, Keyword-Driven, and Hybrid frameworks in Selenium empowers testers to create scalable and efficient automation solutions. These frameworks enhance flexibility, reusability, and ease of maintenance in test scripts, making them indispensable for modern testing needs. Enroll in Selenium for automation testing courses to gain expertise in building and implementing these advanced frameworks and elevate your testing career to new heights.
Call to Action
Master Advanced Selenium Automation Frameworks with H2K Infosys
Take your automation testing skills to the next level by mastering Data-Driven, Keyword-Driven, and Hybrid frameworks in Selenium. At H2K Infosys, we provide expert-led training that covers everything you need to know about these advanced frameworks, enabling you to build scalable, efficient, and reusable test scripts. With hands-on projects and real-world examples, you’ll gain the practical experience required to excel in automation testing.
Donāt miss the opportunity to enhance your testing expertise with comprehensive Selenium training. Learn to implement and optimize advanced frameworks that are in demand across industries. With industry-expert trainers, real-time project experience, and 24/7 support, H2K Infosys ensures you’re fully equipped to succeed. Enroll today and make your mark in the competitive world of automation testing!