Introduction
Automation testing is an essential part of modern software development. Among the many tools available for test automation, Selenium WebDriver stands out due to its flexibility, ease of use, and compatibility with multiple browsers. However, one of the challenges in automated testing is handling complex UI elements that may not be easy to interact with using traditional Selenium commands. This is where Sikuli comes into play.
Sikuli is an open-source tool that uses image recognition to automate graphical user interface (GUI) interactions. When integrated with Selenium WebDriver, Sikuli can tackle UI elements that are otherwise difficult to manage with Selenium alone. In this article, we’ll explore the benefits of Sikuli integration with Selenium WebDriver, how to set it up, and its real-world applications for test automation.
If you are looking to deepen your understanding of Selenium automation testing and gain practical skills to excel in this domain, enrolling in a Selenium certification course or a Selenium course online will be the ideal next step.
What is Sikuli?
Before diving into the integration process, let’s take a moment to understand what Sikuli is and how it works.
Overview of Sikuli
Sikuli is a powerful tool that allows you to automate tasks by recognizing images or graphical elements on the screen. Unlike traditional methods of interacting with web elements (such as finding elements by ID, name, or XPath), Sikuli allows automation based on visual content. This makes it highly effective for applications where traditional HTML-based elements are not easily accessible, such as when testing flash elements, rich media, or custom widgets.
Sikuli uses the Java-based SikuliX engine to recognize and interact with images. By leveraging this image recognition technique, Sikuli can identify UI components and perform actions such as clicking, typing, and dragging-and-dropping without relying on the element’s properties in the code.
Benefits of Sikuli in Automation Testing
- UI-based Testing: Sikuli helps with testing applications where Selenium fails to recognize UI components due to the absence of HTML elements.
- Support for Rich Media: It is useful for automating testing of applications with complex visual interfaces such as Flash, Java applets, and custom controls.
- Cross-Platform Compatibility: Sikuli works across different platforms, including Windows, Linux, and macOS, making it suitable for a diverse range of automation projects.
- No Need for Locators: In traditional Selenium, you need to find elements through locators (ID, Name, XPath). Sikuli eliminates this by automating based on visual cues.
What is Selenium WebDriver?
Selenium WebDriver is one of the most popular and widely used tools for automating web applications. It provides a simple and efficient way to control a browser through programming languages like Java, Python, C#, and JavaScript, among others. As part of the Selenium Suite, WebDriver is a tool designed to support various browsers, allowing testers to automate tasks such as clicking buttons, filling out forms, and navigating web pages to validate the functionality of web applications.
WebDriver works by communicating directly with the browser’s native interface, making it faster and more efficient than other Selenium tools like Selenium RC. Unlike other tools that require a server to be up and running for browser communication, WebDriver interacts with the browser directly through the browser’s API. This direct interaction makes Selenium WebDriver highly effective in performing end-to-end testing of web applications.
Benefits of Selenium WebDriver
Selenium WebDriver has gained immense popularity in the automation testing world due to its numerous benefits. Here are some of the key advantages that make Selenium WebDriver a top choice for automating web applications:
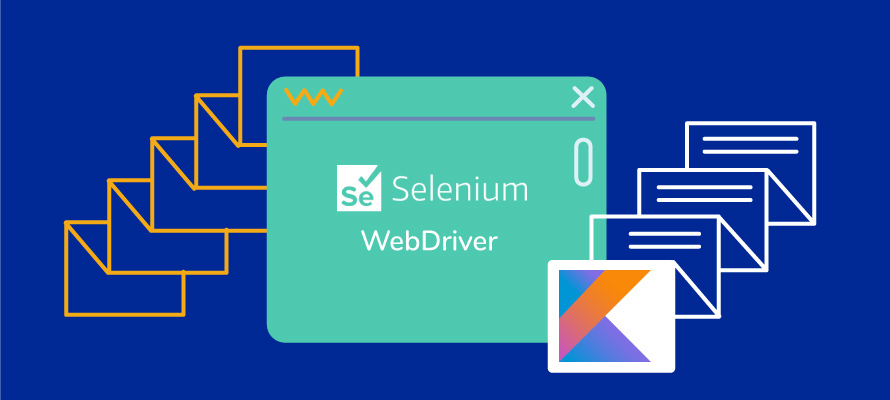
Cross-Browser Compatibility
One of the main advantages of Selenium WebDriver is its support for multiple browsers, such as Google Chrome, Mozilla Firefox, Safari, Internet Explorer, and Edge. This means testers can write a single test script and run it across different browsers to ensure the web application functions correctly on all platforms. This cross-browser compatibility helps reduce the time spent on testing and ensures a consistent user experience across various browsers.
Language Support
Selenium WebDriver allows testers to write scripts in various programming languages, such as Java, Python, C#, Ruby, JavaScript, and more. This flexibility in language choice makes it easier for testers to use their existing knowledge and integrate Selenium with their existing development environments. It also allows the development and testing teams to work more collaboratively, as they can use their preferred languages and tools.
Platform Independence
Selenium WebDriver supports all major operating systems, including Windows, macOS, and Linux. This ensures that tests can be executed across various environments, making it highly versatile for different teams working on different platforms. The ability to automate tests across multiple platforms further increases the flexibility and scalability of the testing process.
Faster Execution
Selenium WebDriver interacts directly with the browser without needing a middle layer, which improves the speed and reliability of test execution. This direct communication allows Selenium WebDriver to run faster compared to older versions of Selenium, such as Selenium RC (Remote Control), which required a server to communicate with the browser.
Why Integrate Sikuli with Selenium WebDriver?
Selenium WebDriver is a versatile tool for automating web applications, but it does have limitations when dealing with complex UI elements or visual-based actions. Sikuli can bridge this gap by integrating visual recognition capabilities into Selenium tests. Here’s why you might consider combining both tools:
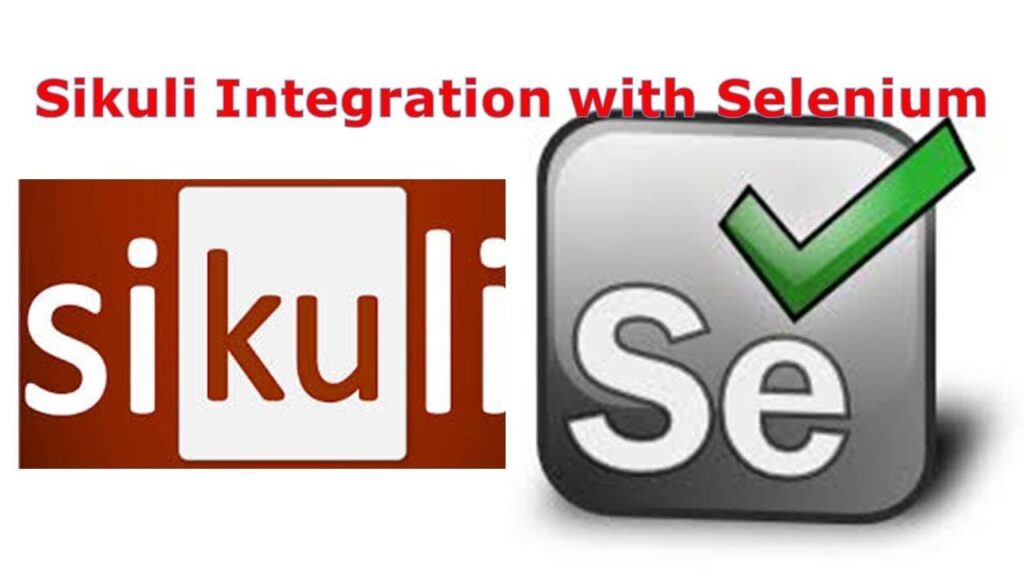
- Handling Complex or Custom UI Elements: Web applications today often feature complex or dynamic interfaces, which are difficult to automate with traditional Selenium locators. Sikuli helps overcome this limitation by allowing testers to interact with UI components based on their visual appearance.
- Combining the Power of Both Tools: While Selenium can interact with standard HTML elements, Sikuli provides an additional layer of functionality, making it easier to automate tasks such as dragging items, clicking on dynamic objects, and handling non-standard buttons.
- Improved Test Coverage: By adding Sikuli to your Selenium test suite, you can automate both functional and visual tests, improving the overall test coverage and ensuring your application works across different environments.
- Enhanced Test Flexibility: Sikuli adds flexibility to your Selenium scripts by allowing you to automate even the most intricate elements that would otherwise require additional manual intervention or workarounds.
How to Integrate Sikuli with Selenium WebDriver
Now that we understand the value of integrating Sikuli with Selenium, let’s walk through the integration process step by step. Below is a detailed guide on how to set up and use Sikuli within a Selenium WebDriver script.
Step 1: Prerequisites
Before you start, make sure you have the following tools installed:
- Java (JDK 1.8 or higher)
- Eclipse or your preferred IDE
- Selenium WebDriver (latest version)
- SikuliX API: Sikuli requires its own API, which you can download from the official SikuliX website.
Step 2: Add Dependencies to Your Project
You will need to add the following JAR files to your project:
- Selenium WebDriver JAR files: These should already be part of your Selenium project.
- Sikuli JAR files: After downloading SikuliX, add the relevant JAR files (e.g.,
sikuli-api.jar
) to your project’s classpath.
In Eclipse:
- Right-click on your project → Build Path → Configure Build Path → Add External JARs and select the Sikuli JAR files.
Step 3: Write a Basic Script for Sikuli and Selenium Integration
Let’s create a simple Selenium WebDriver script integrated with Sikuli. We will use Sikuli to find a button on the screen and click it.
javaCopyimport org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.sikuli.script.Screen;
import org.sikuli.script.Pattern;
import org.sikuli.script.FindFailed;
public class SikuliSeleniumIntegration {
public static void main(String[] args) throws FindFailed {
// Set the path for the Chrome WebDriver
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
// Initialize WebDriver
WebDriver driver = new ChromeDriver();
// Launch the web page
driver.get("https://www.example.com");
// Initialize Sikuli Screen object
Screen screen = new Screen();
// Define the image pattern to search for
Pattern buttonImage = new Pattern("path/to/button_image.png");
// Use Sikuli to click the button based on the image
screen.click(buttonImage);
// Continue with Selenium WebDriver tasks
// For example, you can verify page content, fill out forms, etc.
driver.quit();
}
}
In the script above:
- We launch a web page using Selenium WebDriver.
- Then, we use Sikuli to search for an image of a button on the screen and perform a click action based on that image.
Step 4: Troubleshooting and Best Practices
- Ensure Image Accuracy: Sikuli’s image recognition is dependent on pixel-perfect accuracy. If the screen resolution changes, the image might not be recognized. Always capture images in a consistent resolution.
- Optimize Image Search Area: Sikuli allows you to define specific regions for image search. Restricting the search area can increase performance.
- Handle Delays: Use appropriate waits in your Selenium WebDriver code to allow images to load before Sikuli attempts to click them.
Real-World Use Cases for Sikuli with Selenium
The integration of Sikuli with Selenium WebDriver is particularly useful in various real-world scenarios. Here are some examples where this combination excels:
Testing Flash and Rich Media Applications
Some web applications use Flash or rich media elements, which are not easily accessible using traditional WebDriver locators. Sikuli’s image-based automation allows you to interact with such elements by visual recognition.
Automating Complex Web Applications
Custom UI elements, such as draggable widgets or non-standard buttons, may not have straightforward locators. Sikuli helps automate actions based on images of these elements, making the test scripts more robust and reliable.
Handling Captchas
While CAPTCHA solving is not recommended in automated tests due to ethical and legal concerns, Sikuli can be used in scenarios where bypassing CAPTCHAs is acceptable (e.g., internal testing environments).
Conclusion
Integrating Sikuli with Selenium WebDriver offers a powerful solution for overcoming some of the limitations in UI automation testing. By leveraging the image recognition capabilities of Sikuli, you can test even the most complex or dynamic UI elements that are difficult to automate using standard Selenium locators. This integration enhances your testing strategy, increases your test coverage, and ensures that your web applications perform seamlessly.
For those looking to expand their knowledge in Selenium automation testing, integrating Sikuli with Selenium is an excellent skill to master. Enrolling in a Selenium certification course or a Selenium course online can equip you with the expertise needed to implement such advanced testing techniques effectively.
Key Takeaways:
- Sikuli integration allows Selenium to automate complex UI elements based on visual recognition.
- This integration is particularly useful for handling Flash, custom controls, or dynamic UI elements.
- By following the simple steps outlined in this guide, you can start using Sikuli in your own Selenium projects.
Join H2K Infosys’ Selenium certification course to gain practical skills and enhance your career in automation testing.