SQL Server is a powerful relational database management system widely used in .Net development. Whether you are an experienced developer or a beginner pursuing the Best .Net Course, knowing how to work with SQL Server is essential. One of the common tasks is determining if a specific table exists before performing operations on it. In this guide, we’ll explore step-by-step methods to check if a table exists in SQL Server, accompanied by practical examples.
For those interested in learning SQL Server and its integration with .Net, H2K Infosys offers comprehensive .Net Development Training, which covers database management in-depth. Let’s dive into the details.
Why Check for Table Existence in SQL Server?
In SQL Server, checking for a table’s existence is critical for various scenarios:
Checking for a table’s existence is a common task, particularly in dynamic or automated database operations. This skill is essential for several reasons:
1. Avoiding Runtime Errors
Attempting to query, modify, or delete a non-existent table leads to runtime errors that can disrupt application flow or result in system crashes.
2. Optimizing Performance
Preemptive checks ensure unnecessary queries or redundant operations are avoided, thereby enhancing database performance.
3. Ensuring Data Integrity
By verifying the existence of a table before creating, dropping, or altering it, developers prevent data corruption and preserve system stability.
4. Supporting Automation
Automated database operations often rely on existence checks to conditionally perform tasks, ensuring efficient workflows.
Mastering these techniques allows developers to build reliable, maintainable applications while reducing the likelihood of errors.
Methods to Check If a Table Exists in SQL Server
SQL Server provides multiple approaches to check if a table exists. Below are the most common and efficient methods:
1. Using the INFORMATION_SCHEMA.TABLES
View
The INFORMATION_SCHEMA.TABLES
view is a system view that stores metadata about tables. Here’s how you can use it:
IF EXISTS (
SELECT 1
FROM INFORMATION_SCHEMA.TABLES
WHERE TABLE_SCHEMA = 'dbo'
AND TABLE_NAME = 'YourTableName'
)
BEGIN
PRINT 'Table exists!'
END
ELSE
BEGIN
PRINT 'Table does not exist.'
END
Explanation:
TABLE_SCHEMA
specifies the schema, such asdbo
.TABLE_NAME
specifies the target table name.- Returns
1
if the table exists, otherwise returns nothing.
Best Use Case:
When dealing with databases managed across multiple schemas, this method ensures accuracy.ed.
2. Using the sys.objects
Catalog View
The sys.objects
catalog view contains information about all database objects, including tables.
IF EXISTS (
SELECT 1
FROM sys.objects
WHERE object_id = OBJECT_ID('dbo.YourTableName')
AND type = 'U'
)
BEGIN
PRINT 'Table exists!'
END
ELSE
BEGIN
PRINT 'Table does not exist.'
END
Explanation:
OBJECT_ID
retrieves the unique identifier of the object.type = 'U'
filters for user-defined tables.
Advantages:
Combines flexibility with performance.abases.
Efficient for large databases.
3. Combining sys.tables
with OBJECT_ID
For a more targeted approach, you can use the sys.tables
catalog view:
IF OBJECT_ID('dbo.YourTableName', 'U') IS NOT NULL
BEGIN
PRINT 'Table exists!'
END
ELSE
BEGIN
PRINT 'Table does not exist.'
END
Explanation:
OBJECT_ID('dbo.YourTableName', 'U')
returns the ID if the table exists, andNULL
otherwise.- This method is concise and highly efficient.
4. Using TRY-CATCH Blocks
If you prefer to handle errors gracefully, use a TRY-CATCH
block:
BEGIN TRY
SELECT TOP 1 * FROM dbo.YourTableName;
PRINT 'Table exists!'
END TRY
BEGIN CATCH
PRINT 'Table does not exist.'
END CATCH
Explanation:
- The
TRY
block queries the table. - If the table doesn’t exist, the
CATCH
block captures the error.
When to Use:
Primarily in legacy systems where other methods are unavailable. It’s less efficient due to error-handling overhead.ss efficient compared to the others and should be used sparingly.
Real-World Application: Automating Table Checks in .Net Development
In real-world .Net projects, you often need to automate database operations. Here’s a simple example using C# to check if a table exists in SQL Server:
using System;
using System.Data.SqlClient;
class Program
{
static void Main()
{
string connectionString = "YourConnectionString";
string tableName = "YourTableName";
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
string query = @"IF EXISTS (
SELECT 1
FROM INFORMATION_SCHEMA.TABLES
WHERE TABLE_SCHEMA = 'dbo'
AND TABLE_NAME = @TableName
)
SELECT 'Table exists';
ELSE
SELECT 'Table does not exist';";
using (SqlCommand command = new SqlCommand(query, connection))
{
command.Parameters.AddWithValue("@TableName", tableName);
string result = command.ExecuteScalar()?.ToString();
Console.WriteLine(result);
}
}
}
}
Features:
- Parameterized Queries: Prevents SQL injection.
- Dynamic Integration: Seamlessly integrates with .NET applications.
Benefits:
This script showcases SQL Server’s relevance in .NET development and highlights how SQL Server skills can elevate your career.
Key Points:
- Use parameterized queries to prevent SQL injection.
- This script integrates seamlessly with .Net applications, showcasing the relevance of SQL Server skills in .Net Development Training.
Best Practices for Checking Table Existence in SQL
When working with databases, it is often necessary to check whether a table exists before performing operations like creating, altering, or dropping a table. While there are multiple ways to verify table existence, following best practices ensures efficiency, maintainability, and performance. Below are some best practices to consider:
1. Use OBJECT_ID
for Simple Checks
The OBJECT_ID
function is one of the most efficient ways to check if a table exists. It is a built-in function that returns the object ID of a table (or other database objects) if it exists, otherwise, it returns NULL
.
✅ Example:
sqlIF OBJECT_ID('dbo.Employees', 'U') IS NOT NULL
PRINT 'Table exists';
ELSE
PRINT 'Table does not exist';
'U'
specifies that we are checking for a user table.- This approach is fast and straightforward for single-table existence checks.
2. Always Include the Schema in Your Checks
When checking for a table’s existence, always specify the schema (e.g., dbo
). Many databases have multiple schemas, and failing to include the schema name could result in incorrect or ambiguous results.
✅ Example:
sqlIF OBJECT_ID('HR.Employees', 'U') IS NOT NULL
PRINT 'HR schema Employees table exists';
Without specifying the schema, you might be checking for the wrong table or missing tables that exist under a different schema.
3. Consider Performance When Checking Multiple Tables
If you need to check multiple tables at once, avoid executing multiple OBJECT_ID
queries separately. Instead, use a batch query that checks all necessary tables in a single execution.
✅ Example: Checking multiple tables at once
sqlSELECT name
FROM sys.tables
WHERE name IN ('Employees', 'Departments', 'Projects');
- This query efficiently retrieves all matching table names in a single execution, reducing query overhead.
- It is much faster than running separate queries for each table.
4. Document Your Queries for Maintainability
Well-documented SQL scripts improve readability, maintainability, and collaboration among developers.
✅ Example:
sql-- Check if the Employees table exists before creating a new one
IF OBJECT_ID('dbo.Employees', 'U') IS NOT NULL
PRINT 'Employees table exists';
ELSE
CREATE TABLE dbo.Employees (ID INT, Name VARCHAR(100));
Adding comments or documentation clarifies the purpose of a check and helps future developers understand its intent.
5. Test in a Development Environment Before Deployment
Before deploying a script that checks for table existence, always test it in a development environment. Ensure it behaves as expected under various conditions, such as:
- When the table exists
- When the table does not exist
- When there are multiple schemas
- When permissions are restricted
Testing prevents unexpected errors in production.
6. Avoid Redundant Existence Checks in Loops
If you’re running a script that repeatedly checks for table existence in a loop, try to minimize unnecessary checks. Repeated existence checks can lead to performance degradation.
❌ Inefficient approach:
sqlDECLARE @i INT = 1;
WHILE @i <= 100
BEGIN
IF OBJECT_ID('dbo.TempTable', 'U') IS NOT NULL
PRINT 'Table exists';
SET @i = @i + 1;
END
- The above script checks the same table existence 100 times, which is unnecessary.
✅ Optimized approach:
sqlIF OBJECT_ID('dbo.TempTable', 'U') IS NOT NULL
BEGIN
DECLARE @i INT = 1;
WHILE @i <= 100
BEGIN
PRINT 'Processing...';
SET @i = @i + 1;
END
END
- This checks table existence once, reducing database workload.
7. Log Existence Checks for Debugging and Auditing
For debugging or auditing purposes, consider logging the results of existence checks. This can help in troubleshooting and tracking database changes.
✅ Example: Logging results
sqlINSERT INTO AuditLog (EventType, TableName, CheckTime)
SELECT 'CheckExistence', 'Employees', GETDATE()
WHERE OBJECT_ID('dbo.Employees', 'U') IS NOT NULL;
- This logs each table existence check into an audit table.
8. Use Stored Procedures for Reusability
If you frequently check for table existence, encapsulate the logic into a stored procedure. This improves code reusability and maintainability.
✅ Example: Stored Procedure
sqlCREATE PROCEDURE CheckTableExistence (@TableName NVARCHAR(128))
AS
BEGIN
IF OBJECT_ID(@TableName, 'U') IS NOT NULL
PRINT @TableName + ' exists';
ELSE
PRINT @TableName + ' does not exist';
END;
- This stored procedure can be reused across different scripts.
Common Pitfalls to Avoid
Despite having efficient methods, certain mistakes can lead to incorrect results or performance issues. Here are some common pitfalls:
1. Neglecting Schema in Table Existence Checks
❌ Incorrect approach:
sqlIF OBJECT_ID('Employees', 'U') IS NOT NULL
PRINT 'Table exists';
- If there are multiple schemas (e.g.,
HR.Employees
andSales.Employees
), this check may return incorrect results.
✅ Correct approach:
sqlIF OBJECT_ID('dbo.Employees', 'U') IS NOT NULL
PRINT 'Table exists';
- Always include the schema name.
2. Ignoring Permissions
Users without appropriate database permissions may get NULL results when querying system views like sys.objects
or INFORMATION_SCHEMA.TABLES
.
✅ Solution:
Ensure that the user has VIEW DEFINITION
or necessary privileges to check table existence:
sqlGRANT VIEW DEFINITION ON DATABASE::YourDatabase TO YourUser;
3. Hardcoding Table Names Instead of Using Variables
Hardcoding table names makes scripts inflexible and difficult to reuse.
❌ Bad practice:
sqlIF OBJECT_ID('dbo.Employees', 'U') IS NOT NULL
PRINT 'Table exists';
✅ Better approach using variables:
sqlDECLARE @TableName NVARCHAR(128) = 'dbo.Employees';
IF OBJECT_ID(@TableName, 'U') IS NOT NULL
PRINT 'Table exists';
Using variables or parameters makes scripts dynamic and reusable.
4. Overusing TRY-CATCH for Table Existence Checks
Some developers wrap existence checks in a TRY-CATCH
block, assuming it improves error handling. However, TRY-CATCH
is not needed for routine existence checks and adds unnecessary overhead.
❌ Avoid this inefficient approach:
sqlBEGIN TRY
IF OBJECT_ID('dbo.Employees', 'U') IS NOT NULL
PRINT 'Table exists';
END TRY
BEGIN CATCH
PRINT 'Error checking table existence';
END CATCH;
- This adds unnecessary processing.
✅ Instead, use direct existence checks:
sqlIF OBJECT_ID('dbo.Employees', 'U') IS NOT NULL
PRINT 'Table exists';
Handling Errors Gracefully
When working with table existence checks, it’s important to handle potential errors gracefully. For instance, if a table doesn’t exist, you might want to log this event, notify the user, or perform alternative actions.
Example Error Handling:
sqlIF OBJECT_ID(N'dbo.YourTableName', N'U') IS NULL
BEGIN
PRINT 'Table does not exist. Creating the table now...'
CREATE TABLE dbo.YourTableName (
ID INT PRIMARY KEY,
Name NVARCHAR(50)
)
END
Explanation:
- In this example, if the table doesn’t exist, the script creates it.
- This approach can be particularly useful in automated scripts where you want to ensure that a required table is always present.
SQL Server and .NET: A Perfect Match for Modern Application Development
In today’s fast-paced development environment, SQL Server and .NET form a powerful combination, enabling developers to build robust, scalable, and high-performance applications. SQL Server, Microsoft’s enterprise-grade relational database management system (RDBMS), seamlessly integrates with .NET, a versatile software framework used for developing web, desktop, and cloud-based applications. This synergy not only simplifies database management but also enhances application efficiency and security.
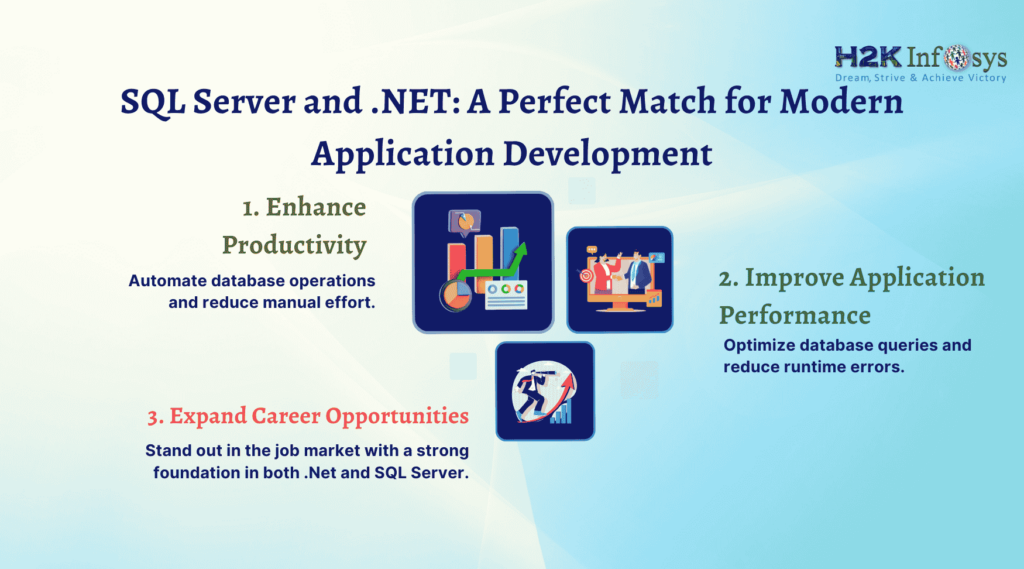
By mastering SQL Server in the context of .NET development, professionals can unlock numerous benefits, including:
1. Enhance Productivity
✅ Seamless Data Management – SQL Server’s tight integration with .NET enables smooth data handling, reducing the complexity of database operations.
✅ Automated Database Operations – Use ADO.NET, Entity Framework, and LINQ to automate data transactions, reducing manual interventions.
✅ Code Reusability – Write efficient, reusable database code through stored procedures, triggers, and .NET-based API integrations.
✅ Faster Development – Visual Studio’s built-in SQL Server tools allow direct database interaction, streamlining the development process.
2. Improve Application Performance
✅ Optimized Database Queries – Leverage indexing, query optimization techniques, and stored procedures to boost data retrieval speeds.
✅ Reduced Runtime Errors – Strong type-checking and exception handling in .NET minimize errors in SQL query execution.
✅ Efficient Data Access – Utilize frameworks like Entity Framework (EF) and Dapper for efficient data mapping and ORM-based database interaction.
✅ Scalability – SQL Server’s support for partitioning, in-memory tables, and clustering helps scale applications effortlessly.
3. Expand Career Opportunities
✅ High Demand in the Job Market – Companies seek professionals skilled in both SQL Server and .NET for enterprise-grade application development.
✅ Versatile Skill Set – Knowledge of SQL Server, C#, ASP.NET Core, and Entity Framework makes developers more adaptable across industries.
✅ Competitive Edge – Stand out from the crowd by mastering full-stack development, handling both backend database operations and frontend development.
✅ Cloud Integration – With Azure SQL Database, developers can expand their expertise to cloud-based database management and serverless architectures.
H2K Infosys’s best .Net training programs provide hands-on experience with SQL Server, empowering you to build robust, data-driven applications.
Key Takeaways
- Checking for table existence in SQL Server is a fundamental skill for .Net developers.
- Multiple methods, including
INFORMATION_SCHEMA.TABLES
andOBJECT_ID
, offer flexible options to verify table existence. - Real-world integration with .Net applications highlights the importance of SQL Server expertise.
- Following best practices ensures efficient and maintainable code.
- Avoid common pitfalls like neglecting schema or relying on inefficient methods.
Conclusion
Checking for table existence is a fundamental yet powerful skill for any SQL Server or .NET developer. By leveraging the methods discussed here, you can:
- Write efficient, error-free code.
- Enhance application performance.
- Minimize downtime and Debugging efforts.
Ready to elevate your skills?
Join H2K Infosys’s .NET Development Training for hands-on experience in SQL Server, .NET integration, and more. Build your expertise and unlock new career opportunities in software development!
2 Responses