Introduction
In this comprehensive guide, we’ll cover the top interview questions and answers related to HTML CSS JavaScript to help you ace your next interview. HTML, CSS, and JavaScript are the foundational technologies of web development. Whether you’re aiming for a front-end developer role or looking to strengthen your knowledge, preparing for interviews in these areas is essential.
HTML Interview Questions & Answers
1. What is HTML and why is it important?
Answer: HTML (HyperText Markup Language) is the standard language used to create web pages. It structures the content of web pages using elements such as headings, paragraphs, links, images, and more. HTML is crucial because it forms the backbone of all web content, allowing browsers to display text, images, and multimedia in a structured manner.
2. Explain the difference between HTML4 and HTML5.
Answer: HTML5 is an updated version of HTML4 and includes several enhancements:
- New Semantic Elements: HTML5 introduced elements like
<header>
,<footer>
,<article>
, and<section>
, which gives meaning to the structure of the webpage. - Multimedia Support: HTML5 natively supports multimedia elements like
<audio>
and<video>
, eliminating the need for external plugins. - APIs and Interactive Features: HTML5 includes APIs such as the Geolocation API, Web Storage API, and Canvas for drawing graphics.
- Improved Forms: HTML5 offers new input types such as
email
,date
, andrange
, which provides better form validation and user experience.
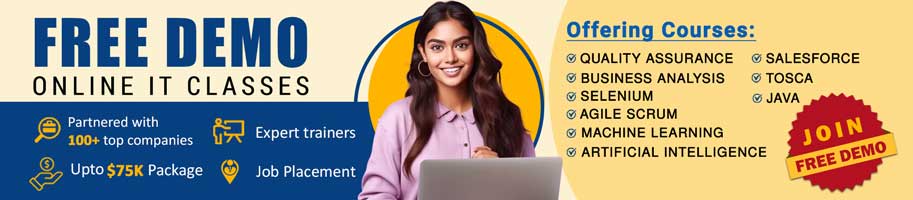
3. What are meta tags in HTML?
Answer : Meta tags in HTML are elements that provide metadata about a webpage, such as its description, keywords, author, and viewport settings. They are placed within the <head>
section of an HTML document and are used by search engines, browsers, and other services to understand and process the page content. Example: <meta name="description" content="This is a sample webpage.">
.
4. What is the role of the alt
attribute in the <img>
tag?
Answer: The alt
attribute provides alternative text for an image. This text is displayed if the image cannot be loaded and is also read by screen readers for accessibility purposes. Additionally, the alt
attribute helps search engines understand the content of the image, contributing to SEO.
5. Describe the difference between block-level and inline elements.
Answer:
- Block-Level Elements: Block-level elements take up the full width available and always start on a new line. Examples include
<div>
,<p>
, and<h1>
. - Inline Elements: Inline elements only take up as much width as necessary and do not start on a new line. Examples include
<span>
,<a>
, and<img>
.
6. What are semantic HTML elements and why are they important?
Answer: Semantic HTML elements clearly describe their meaning in a human- and machine-readable way. Examples include <article>
, <aside>
, <details>
, <figcaption>
, <figure>
, <footer>
, <header>
, and <section>
. These elements improve the accessibility and SEO of a webpage, as search engines and screen readers can better understand the structure and content of the page.
7. What is the difference between HTML and XHTML?
Answer: XHTML is stricter, derived from XML, and requires all tags to be properly closed and case-sensitive (lowercase).
HTML is less strict with syntax and does not enforce well-formed documents.
8. What is the purpose of the data-*
attribute?
Answer:
The data-*
attribute is used to store custom data within HTML elements that can be accessed using JavaScript.
Example:
htmlCopy code<div data-id="12345">Item</div>
CSS Interview Questions & Answers
1. What is CSS and why is it used?
Answer: CSS (Cascading Style Sheets) is a stylesheet language used to control the presentation of HTML documents. It allows developers to style elements, control layout, and apply visual effects to create visually appealing web pages. CSS can be applied inline, internally within the HTML document, or externally through linked CSS files.
2. How do you center a div
element in CSS?
Answer: One common way to center a div
horizontally is by using the following CSS:
css .div {
width: 50%;
margin: 0 auto;
}
For both horizontal and vertical centering, Flexbox can be used:
css.container {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
3. Explain the CSS box model.
Answer: The CSS box model describes how elements are structured on a web page. It consists of:
- Content: The actual content inside the element, such as text or images.
- Padding: The space between the content and the border of the element.
- Border: The edge around the padding and content.
- Margin: The space outside the border, separating the element from other elements.
4. What is the difference between id
and class
selectors in CSS?
Answer:
id
Selector: Theid
selector targets a unique element on a webpage. It is denoted by#
followed by the ID value (e.g.,#header
). IDs must be unique within a document.class
Selector: Theclass
selector targets one or more elements that share the same class. It is denoted by.
followed by the class name (e.g.,.button
). Multiple elements can have the same class.
5. How does Flexbox work in CSS?
Answer: Flexbox is a CSS layout module designed to distribute space along a container’s main axis and predictably align-items. It is handy for creating responsive layouts. Flexbox properties include:
display: flex
: Sets the container to use Flexbox.justify-content
: Aligns items horizontally (main axis).align-items
: Aligns items vertically (cross-axis).flex-direction
: Defines the direction of the main axis (row or column).flex-wrap
: Controls whether the items should wrap onto multiple lines.
6. What are pseudo-classes and pseudo-elements in CSS?
Answer:
- Pseudo-Classes: Pseudo-classes are keywords added to selectors that specify a special state of the selected elements. Examples include
:hover
,:focus
, and:nth-child
. - Pseudo-Elements: Pseudo-elements allow you to style specific parts of an element. They are denoted by double colons
::
. Examples include::before
,::after
, and::first-line
.
7. What is the difference between relative
, absolute
, fixed
, and sticky
positioning in CSS?
Answer:
relative
: Positions the element relative to its normal position. It does not remove the element from the document flow.absolute
: Positions the element relative to its nearest positioned ancestor. The element is removed from the document flow.fixed
: Positions the element relative to the viewport, and it remains fixed in place even when scrolling.sticky
: A hybrid ofrelative
andfixed
. The element toggles between the two based on the user’s scroll position.
8. What is the difference between inline, internal, and external CSS?
Answer:
- Inline CSS: Styles applied directly on the element using the
style
attribute. - Internal CSS: Styles defined within a
<style>
tag in the HTML document’s<head>
. - External CSS: Styles are defined in a separate
.css
file and linked using<link>
.
9. What is the box model in CSS?
Answer:
The box model includes the following components:
- Content: The actual text or image.
- Padding: Space around content.
- Border: Surrounds the padding.
- Margin: Space outside the border.
JavaScript Interview Questions & Answers
1. What is JavaScript and how is it used in web development?
Answer: JavaScript is a high-level, interpreted programming language that enables interactive web pages. It is used to manipulate the Document Object Model handle events, perform asynchronous operations, and create dynamic content. JavaScript runs on the client side (in the browser) but can also be used on the server side with environments like Node.js.
2. What are JavaScript data types?
Answer: JavaScript data types include:
- Primitive Types:
string
,number
,boolean
,null
,undefined
,symbol
, andbigint
. - Object Types: Objects, arrays, and functions are considered complex data types in JavaScript.
3. Explain the difference between ==
and ===
.
Answer:
==
(Loose Equality): Compares two values for equality after performing type conversion if necessary. For example,5 == '5'
returnstrue
.===
(Strict Equality): Compares two values for equality without type conversion. Both value and type must be the same. For example,5 === '5'
returnsfalse
.
4. What is a closure in JavaScript?
Answer: A closure is a function that retains access to its lexical scope even when the function is executed outside of that scope. Closures are commonly used to create private variables or functions within a function.
Example:
javascriptfunction outerFunction() {
let count = 0;
return function innerFunction() {
count++;
return count;
}
}
const increment = outerFunction();
console.log(increment()); // Output: 1
console.log(increment()); // Output: 2
5. What is event delegation in JavaScript?
Answer: Event delegation is a technique where a single event listener is added to a parent element to manage events on its child elements. Instead of adding multiple event listeners to individual child elements, the event listener on the parent handles events that bubble up from the children.
Example:
javascriptdocument.querySelector("#parentElement").addEventListener("click", function(event) {
if (event.target.matches(".childElement")) {
console.log("Child element clicked:", event.target);
}
});
6. What is the this
keyword in JavaScript?
Answer: The this
keyword in JavaScript refers to the object that is currently executing the code. The value this
depends on the context in which it is used:
- Global Context: In the global scope,
this
refers to the global object (e.g.,window
in browsers). - Function Context: In a function,
this
refers to the object that is called the function. In strict mode,this
isundefined
in a standalone function. - Object Context: In an object method,
this
refers to the object itself. - Constructor Function Context: In a constructor function,
this
refers to the newly created object.
7. Explain the difference between var
, let
, and const
.
Answer:
var
:var
is function-scoped and can be redeclared. It is hoisted to the top of its scope but may lead to unexpected behavior due to its global scope or function scope.let
:let
is block-scoped and cannot be redeclared within the same scope. It is also hoisted but not initialized until execution.const
:const
is also block-scoped and is used to declare constants. Variables declaredconst
cannot be reassigned. However, if the constant is an object or array, its properties or elements can still be modified.
8. What is the DOM and how does JavaScript interact with it?
Answer: The DOM (Document Object Model) is a programming interface for HTML and XML documents. It represents the structure of a document as a tree of nodes, where each node corresponds to an element, attribute, or piece of text. JavaScript can manipulate the DOM to dynamically update the content, style, and structure of a webpage by adding, removing, or modifying nodes.
Example:
javascriptdocument.getElementById("myElement").textContent = "New Text";
document.querySelector(".myClass").style.color = "blue";
9. What is asynchronous programming in JavaScript?
Answer: Asynchronous programming in JavaScript allows for non-blocking operations, meaning the program can continue executing while waiting for an operation (like a network request) to complete. Common approaches to asynchronous programming include:
- Callbacks: Functions passed as arguments to be executed once an asynchronous operation is complete.
- Promises: Objects representing the eventual completion (or failure) of an asynchronous operation, with
then
andcatch
methods to handle the result. - Async/Await: Syntactic sugar built on Promises that allow asynchronous code to be written in a more synchronous style.
Example:
javascript async function fetchData() {
try {
let response = await fetch('https://api.example.com/data');
let data = await response.json();
console.log(data);
} catch (error) {
console.error('Error:', error);
}
}
fetchData();
10. How do you handle errors in JavaScript?
Answer: Errors in JavaScript can be handled using try...catch
blocks. This structure allows you to attempt to run a block of code (try
) and catch any errors that occur (catch
).
Example:
javascript try {
let result = riskyOperation();
console.log(result);
} catch (error) {
console.error('An error occurred:', error.message);
}
Additionally, promises provide .catch()
to handle errors in asynchronous code.
11. What are JavaScript modules and how do you use them?
Answer: JavaScript modules are reusable pieces of code that can be exported from one file and imported into another. This promotes code organization and reuse. ES6 introduced native support for modules in JavaScript.
Example:
javascript // math.js
export function add(a, b) {
return a + b;
}
// app.js
import { add } from './math.js';
console.log(add(2, 3)); // Output: 5
In older environments, modules could be managed using CommonJS (module.exports
and require()
) or AMD (Asynchronous Module Definition).
12. What is the event loop in JavaScript?
Answer: The event loop is a mechanism in JavaScript that allows the language to handle asynchronous operations. The event loop continuously checks the call stack and the task queue. If the call stack is empty, the event loop pushes the first task in the queue to the stack, allowing it to execute.
This process enables JavaScript to perform non-blocking operations, such as handling I/O, timers, and promises.
13. What is the difference between let
, var
, and const
?
Answer:
var
: Function-scoped, can be redeclared.let
: Block-scoped, cannot be redeclared.const
: Block-scoped, value cannot be reassigned.
14. What is async/await in JavaScript?
Answer:async/await
is syntactic sugar for handling promises.
Example:
javascript async function fetchData() {
const data = await fetch("https://api.example.com/data");
const json = await data.json();
console.log(json);
}
Conclusion
Understanding the core concepts of HTML, CSS, and JavaScript is essential for any web developer. The questions and answers provided in this guide cover a wide range of topics, from basic principles to more advanced concepts, ensuring you’re well-prepared for your next interview. Whether you’re just starting or looking to advance your career, mastering these fundamentals will help you build a strong foundation in web development. To further enhance your skills, H2K Infosys offers an in-depth Java course designed by industry experts. This course will equip you with the knowledge and hands-on experience needed to excel in both web development and backend programming. By familiarizing yourself with common HTML, CSS, and JavaScript interview questions, you will have a better understanding of what employers are looking for. These skills will not only help you ace interviews but also empower you to build and maintain dynamic, interactive websites.
One Response