Introduction
Python, celebrated for its simplicity and adaptability, continues to dominate as one of the most sought-after programming languages in the tech world. Its clean syntax and wide-ranging applications make it a favorite among both seasoned developers and beginners looking to establish themselves in the industry. For those aiming to excel in their careers, whether by advancing in their current roles or securing their first job, mastering Python has become increasingly vital.
This guide delves into the most pertinent Python interview questions and answers 2025, providing insights to help you navigate the job market with confidence. Additionally, we’ll explore the significance of earning a Certification on python programming, highlighting how enrolling in online classes can significantly enhance your skills and propel your career forward.
Why Python?
Python’s extensive libraries, readability, and dynamic typing make it a favorite among developers and companies alike. Its applications range from web development and data analysis to artificial intelligence and machine learning.
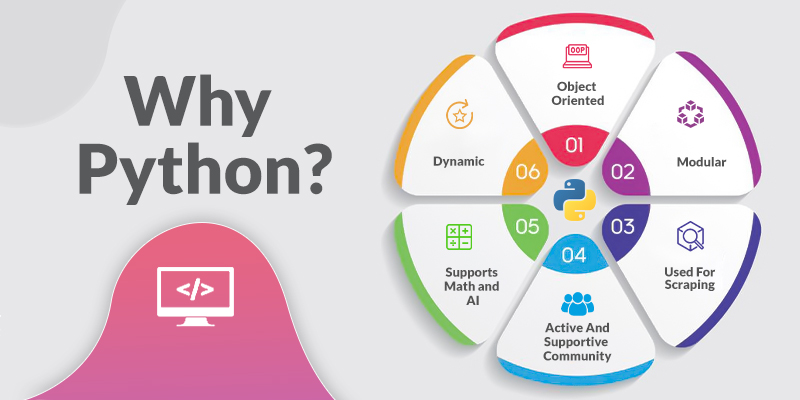
Common Python Interview Questions and Answers
What are Python’s key features?
Answer: It is an interpreted, high-level, and dynamically typed language. Key features include:
- Easy to Learn and Use: It has a simple syntax similar to English, making it beginner-friendly.
- Expressive Language: Less coding effort compared to other languages.
- Interpreted Language: The code is executed line by line, which makes debugging easier.
- Dynamic Typing: Variables are dynamically typed, meaning they don’t need explicit declaration.
- Extensive Libraries: It offers a rich standard library and numerous third-party modules.
How is memory managed in Python?
Answer: Memory management includes:
- Automatic Garbage Collection: Python has an in-built garbage collector to manage memory.
- Reference Counting: Objects are deallocated when their reference count drops to zero.
- Memory Pools: It uses private heaps and memory pools to allocate space for objects.
Explain the difference between deep copy and shallow copy.
Answer:
- Shallow Copy: Copies the reference of the object, not the object itself. Changes to the copied object reflect in the original object.
- Deep Copy: Copies the object and its nested objects. Changes in the copied object do not affect the original object.
- Example:
import copy
original_list = [[1, 2, 3], [4, 5, 6]]
shallow_copy = copy.copy(original_list)
deep_copy = copy.deepcopy(original_list)
What is the Global Interpreter Lock (GIL) in Python?
Answer: The GIL is a mutex that protects access to Python objects, preventing multiple threads from executing bytecodes simultaneously. This simplifies memory management but can be a bottleneck in multi-threaded programs.
How do you manage packages in Python?
Answer:
pip: The package installer, used to install and manage packages.
Virtualenv: A tool to create isolated environments.
Anaconda: A distribution for scientific computing, with package management and environment management.
Real-World Python Applications
Data Analysis with Python
It is a powerful tool for data analysis due to libraries like Pandas, NumPy, and Matplotlib.
- Example:
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
# Creating a simple DataFrame
data = {'Name': ['Alice', 'Bob', 'Charlie'], 'Age': [25, 30, 35]}
df = pd.DataFrame(data)
print(df)
Web Development with Python
Frameworks like Django and Flask make this an excellent choice for web development.
- Example:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello():
return "Hello, World!"
if __name__ == '__main__':
app.run(debug=True)
Machine Learning with Python
Libraries such as Scikit-learn and TensorFlow provide robust tools for Machine learning.
- Example:
from sklearn.linear_model import LinearRegression
# Sample data
X = [[1], [2], [3]]
y = [1, 2, 3]
# Create model
model = LinearRegression()
model.fit(X, y)
print(model.predict([[4]]))
Advanced Python Interview Questions
What are Python’s namespaces? Explain their types.
Answer: Namespaces in Python are a way to ensure that names are unique to avoid naming conflicts. Types include:
- Local Namespace: Created inside a function and only accessible within it.
- Global Namespace: Created at the program’s start and remains till the end. Contains global variables.
- Built-in Namespace: Contains built-in functions and exceptions.
What is Python’s pass statement?
Answer: The pass
statement is a null operation used when a statement is syntactically required but no action is needed.
- Example:
def function_that_does_nothing():
pass
Explain Python’s List Comprehension with an example.
Answer: List comprehensions provide a concise way to create lists. It consists of brackets containing an expression followed by a for
clause.
- Example:
squares = [x**2 for x in range(10)]
print(squares)
How does Python handle exceptions? Explain with an example.
Answer: It handles exceptions using try
and except
blocks. The try
block contains code that may raise an exception, and the except
block handles the exception.
- Example:
try:
result = 10 / 0
except ZeroDivisionError:
print("Cannot divide by zero!")
Explain the use of decorators in Python.
Answer: Decorators are a powerful feature that allows you to modify the behavior of a function or a class. They are often used for logging, enforcing access control, instrumentation, caching, and more.
- Example:
def decorator_function(original_function):
def wrapper_function(*args, **kwargs):
print(f"Wrapper executed this before {original_function.__name__}")
return original_function(*args, **kwargs)
return wrapper_function
@decorator_function
def display():
print("Display function ran")
display()
What is the difference between is
and ==
in Python?
Answer:
is
: Checks for object identity, i.e., whether two references point to the same object in memory.==
: Checks for value equality, i.e., whether two objects have the same value.- Example:
a = [1, 2, 3]
b = a
c = list(a)
print(a == b) # True, values are equal
print(a is b) # True, both point to the same object
print(a == c) # True, values are equal
print(a is c) # False, different objects in memory
How can you implement a singleton pattern in Python?
Answer: The singleton pattern ensures that a class has only one instance and provides a global point of access to it.
- Example:
class Singleton:
_instance = None
def __new__(cls, *args, **kwargs):
if not cls._instance:
cls._instance = super(Singleton, cls).__new__(cls, *args, **kwargs)
return cls._instance
obj1 = Singleton()
obj2 = Singleton()
print(obj1 is obj2) # True
What is the difference between deepcopy
and copy
?
Answer:
copy.copy()
: Creates a shallow copy of an object. Changes made to a copied object may affect the original object if they contain references to mutable objects.copy.deepcopy()
: Creates a deep copy of an object. Recursively copies all objects, ensuring that changes in the copied object do not affect the original.
Explain how Python’s garbage collection works.
Answer: It’s Garbage collection is a process of automatically freeing up memory by destroying unused objects. It uses reference counting and a cyclic garbage collector to clean up circular references.
What is a Python generator? How do you create one?
Answer: A generator is a function that returns an iterator and produces a sequence of values lazily, pausing between each yield.
- Example:
def simple_generator():
yield 1
yield 2
yield 3
gen = simple_generator()
print(next(gen)) # 1
print(next(gen)) # 2
print(next(gen)) # 3
What is the purpose of the with
statement in Python?
Answer: The with
statement simplifies exception handling by encapsulating common preparation and cleanup tasks in so-called context managers.
- Example:
with open('file.txt', 'r') as file:
content = file.read()
This ensures that the file is properly closed after its suite finishes, even if an exception is raised.
Explain the difference between @staticmethod
and @classmethod
.
Answer:
@staticmethod
: Defines a method that does not operate on an instance or class. It can be called on the class itself.@classmethod
: Defines a method that takes a class as its first parameter (usually namedcls
). It can modify class state that applies across all instances.- Example:
class MyClass:
@staticmethod
def static_method():
print("Static method")
@classmethod
def class_method(cls):
print("Class method", cls)
MyClass.static_method()
MyClass.class_method()
What is Python’s itertools
module, and why is it useful?
Answer: itertools
is a module in it that provides functions creating iterators for efficient looping. It is useful for creating complex iterators and performing combinatorial operations.
- Example:
import itertools
# Example of itertools.combinations
comb = itertools.combinations([1, 2, 3], 2)
for c in comb:
print(c)
How do you handle missing keys in Python dictionaries?
Answer:
- Using
get()
Method: ReturnsNone
or a default value if the key is not found. - Using
setdefault()
Method: Returns the value if the key is present; otherwise, inserts the key with a specified default value. - Example:
d = {'key1': 'value1'}
print(d.get('key2', 'default')) # default
# Using setdefault
print(d.setdefault('key2', 'default')) # default
print(d) # {'key1': 'value1', 'key2': 'default'}
These advanced questions and answers provide a deeper insight into it, preparing you for interviews and practical applications in real-world scenarios.
Python Frameworks and Libraries
Django
Django is a high-level Python web framework that encourages rapid development and clean, pragmatic design.
- Features:
- Robust security features
- Scalable and flexible
- Built-in admin panel
Flask
Flask is a lightweight WSGI web application framework. It is designed to make getting started quick and easy.
- Features:
- Minimalistic and simple
- Extensible with numerous plugins
- Flexible with minimal dependencies
Pandas
Pandas is an open-source data analysis and manipulation tool.
- Example:
import pandas as pd
data = {'Product': ['Apples', 'Oranges', 'Bananas'], 'Price': [100, 150, 120]}
df = pd.DataFrame(data)
print(df)
Preparing for Advanced Python Roles
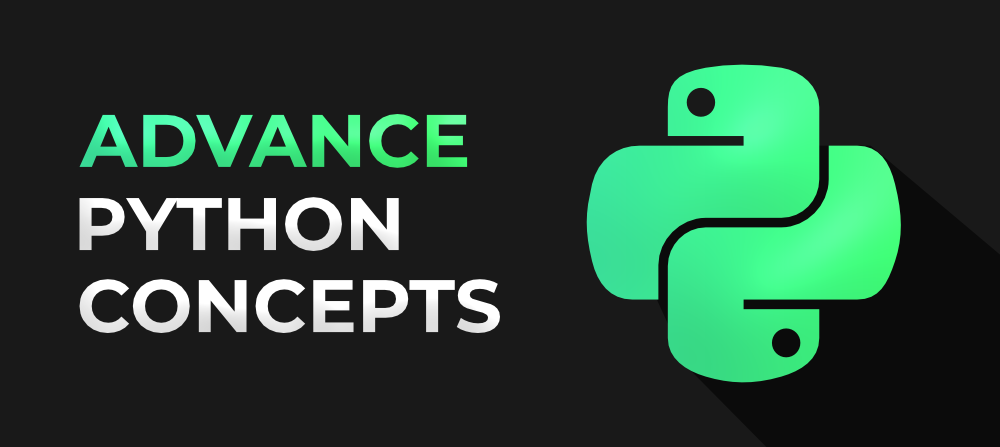
Master Data Structures and Algorithms
Understanding data structures like lists, dictionaries, sets, and tuples is crucial. Algorithms help solve problems efficiently.
Explore Asynchronous Programming
Learn about asynchronous programming with asyncio
to handle asynchronous tasks efficiently.
- Example:
import asyncio
async def main():
print("Hello")
await asyncio.sleep(1)
print("World")
asyncio.run(main())
Understand Python’s Internals
Delve it into internals, such as memory management, and understand how this executes your code.
Benefits of Online Classes
Flexibility
Online classes offer flexibility, allowing learners to balance education with other responsibilities.
Access to Resources
Students can access a plethora of resources, including recorded lectures, e-books, and forums.
Interactive Learning
Many online courses provide interactive learning experiences through quizzes, projects, and live sessions.
Networking Opportunities
Online classes connect students with peers and instructors worldwide, fostering a collaborative learning environment.
Conclusion
Preparing for interview requires a blend of theoretical knowledge and practical application. By understanding the top interview questions and answers, practicing coding problems, and obtaining relevant certifications, you can significantly enhance your chances of success. At H2K Infosys, we offer comprehensive Online classes for Python that provide hands-on learning to help you master the programming and advance your career. Enroll today to start your journey toward becoming a certified professional.
Key Takeaways
- This simplicity and versatility make it a top choice for various applications.
- Common interview questions cover topics like memory management, GIL, and package management.
- Practical experience through projects and certifications can boost your employability.