What are the Hibernate Configuration Files?
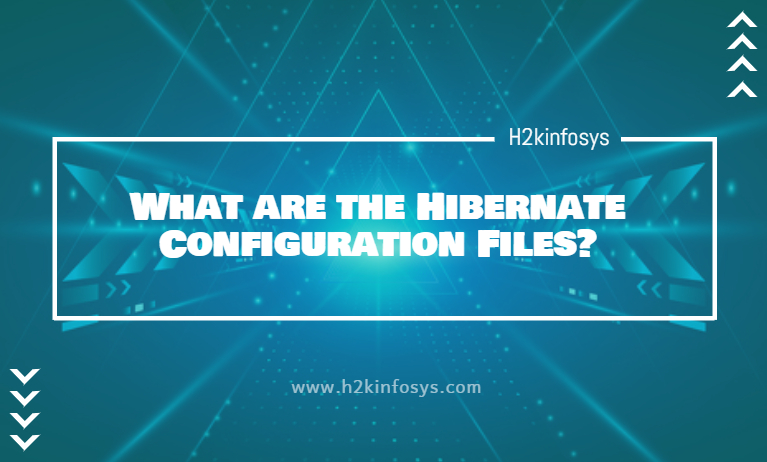
What is HBM File?
The full name of HBM is Hibernate Mapping. It is an XML file in which we define the mapping between POJO class to the database table and POJO class variables to table columns. The resource file hibernate.cfg.xml, which supports to represent the Hibernate configuration information. The connection.driver_class, connection.URL, connection.username, and connection.password property element that characterizes the JDBC connection information. The connection.pool_size is used to configure Hibernate’s built-in connection pool how many connections to the pool. The Hibernate XML mapping file which includes the mapping correlation between the Java class and the database table. It is mostly named “xx.hbm.xml” and represents in the Hibernate configuration file “hibernate.cfg.xml.”
For example, the mapping file (hbm.xml) is mention in the “mapping” tag.
<hibernate-configuration> <session-factory> <property name="hibernate.bytecode.use_reflection_optimizer">false</property> <property name="hibernate.connection.driver_class">com.mysql.jdbc.Driver</property> <property name="hibernate.connection.password">password</property> <property name="hibernate.connection.url">jdbc:mysql://localhost:3306/demo</property> <property name="hibernate.connection.username">root</property> <property name="hibernate.dialect">org.hibernate.dialect.MySQLDialect</property> <property name="show_sql">true</property> <mapping resource="com/demo/common/HiberDemo.hbm.xml"></mapping> </session-factory> </hibernate-configuration>
Add Hibernates mapping file (hbm.xml) programmatically:
- Hibernate provides a method for the developer to add mapping files technically.
- Let’s modify the default Hibernate SessionFactory class by passing your “hbm.xml” file path as an argument into the addResource() method:
SessionFactory sessionFactory = new Configuration().addResource(“com/demo/commonStock.hbm.xml”).buildSessionFactor();
HibernateUtil.java
Example of HibernateUtil.java, load the Hibernate XML mapping file demo.hbm.xml” programmatically:
import org.hibernate.SessionFactory; import org.hibernate.cfg.Configuration; public class HibernateUtil { private static final SessionFactory sessionFactory = buildSessionFactory(); private static SessionFactory buildSessionFactory() { try { SessionFactory sessionFactory = new Configuration() .configure("/com/demo/persistence/hibernate.cfg.xml") .addResource("com/demo/common/Stock.hbm.xml") .buildSessionFactory(); return sessionFactory; } catch (Throwable e) { System.err.println("Initial SessionFactory creation failed." + e); throw new ExceptionInInitializerError(e); } }
There are two property elements that declare the remaining two properties of the Event class: i.e., date and title.
The date property mapping includes the column attribute, but the title does not has. When there is an absence of a column attribute, Hibernate uses the property name as the column name. This is appropriate for the title, but since the date is a reserved keyword in most of the databases.
public static SessionFactory getSessionFactory() { return sessionFactory; } public static void shutdown() { // Close caches and connection pools getSessionFactory().close(); } }
- There is the two way of mapping in hibernate – The first one which is by using the hibernate annotation and the second one which is by using the hbm.xml.
- When we use hbm.xml, to just modify the default hibernate SessionFactory class in hibernate-cfg.xml bypassing your “hbm.xml” file path as an argument to resource method.
Example: Here, we are taking an example of the employee table.
Employee.hbm.xml file is your hbm.xml file in which table column and their types are mapped.
Employee.hbm.xml
<hibernate-mapping> <class name="net.roseindia.table.Employee" table="employee"> <id name="empId" type="int" column="emp_id"> <generator class="native" /> </id> <property name="EmpName" type="string" column="emp_name" /> <property name="salary" type="int" column="emp_salary" /> <property name="designation" type="string" column="designation" /> <property name="address" type="string" column="address" /> </class> </hibernate-mapping>
hibernate-cfg.xml is the configuration file of hibernate where you map hbm.xml
<hibernate-configuration> <session-factory> <!-- Database connection settings --> <property name="connection.driver_class">com.mysql.jdbc.Driver</property> <property name="connection.url">jdbc:mysql://localhost:3306/hibernate</property> <property name="connection.username">root</property> <property name="connection.password">root</property> <!-- JDBC connection pool (use the built-in) --> <property name="connection.pool_size">1</property> <!-- SQL dialect --> <property name="dialect">org.hibernate.dialect.MySQLDialect</property> <!-- Enable Hibernate's automatic session context management --> <property name="current_sesion_context_class">thread</property> <!-- Disable the second-level cache --> <property name="cache.provider_class">org.hibernate.cache.NoCacheProvider</property> <!-- Echo all executed SQL to stdout --> <property name="show_sql">true</property> <property name="hbm2ddl.auto">none</property> <mapping resource="Employee.hbm.xml"/> </session-factory> </hibernate-configuration>