Alert and Popup Window Handling in Selenium WebDriver
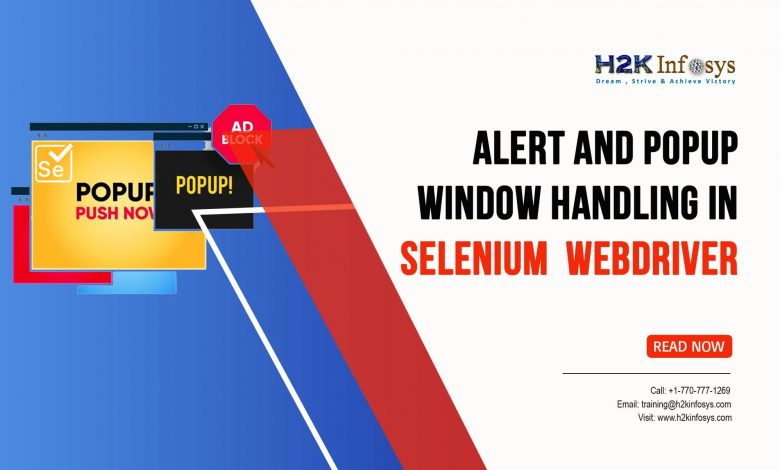
In this article, we will learn types of alerts found in the web application testing and we learn how to handle alerts in Selenium Webdriver. We will also learn how to accept and reject the alerts based upon the alert types.
In this article, you will learn
- What is an Alert?
- How to handle Alert in Selenium WebDriver
- How to handle Selenium Popup window using Selenium WebDriver
What is Alert?
Alert is a small message box that displays on-screen notification which provides the user some kind of information, If it takes User input then it’s a popup message. It also is used for warning purposes. If convey or asks for permission it’s a confirmation box. Alert is a popup window that displays on the screen. Users can perform many actions that can result in an alert on the screen. For example, when the user clicked on a button it displays a message or when you entered a form, the HTML page asks for some extra information. Usually, alerts are different from regular windows. The main difference is that alerts are blocking in nature means that it will not allow users to perform any actions on the webpage if they are present.
Types of Alerts
There are mainly three types of alerts
- Simple Alert: It displays some information and just has an OK button on them. It mainly displays some information to the user.
Syntax:
or alert(“This is an alert”);
2. Prompt Alert: In prompt alerts, you have an input box to add text to the alert box. This is used when the user is required to enter the input. We use sendKeys() method to type something in the Prompt alert box.
Syntax:
3. Confirmation Alert: This alert has an option to accept or dismiss the alert. We use Alert.accept() to accept the alert and we use Alert.dismiss() to dismiss the alert.
Syntax:
Alerts handling using Selenium WebDriver
Selenium provides an interface called Alert which is present in the org.openqa.selenium.Alert package. Alert interface has the following methods to deal with the alerts.
- accept(): To accept the alert by clicking on the ‘OK’ button of the alert.
- dismiss(): To cancel the alert by clicking on the ‘Cancel’ button.
getText(): To get the text of the alert message.
- sendKeys(): To write text to the alert
By using Selenium’s .switchTo() method we can switch to the alert from the main window.
Working with the Alert box using Selenium Webdriver:
Below is the sample code for alert box, make an HTML file and pass it to the webdriver.
The below program will show Alerts popup using above html file.
import org.openqa.selenium.Alert;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class AlertPopupHandling {
public static void main(String[] args) throws InterruptedException {
// TODO Auto-generated method stub
System.setProperty("webdriver.chrome.driver", "D:\\Drivers\\geckodriver.exe");
WebDriver driver = new ChromeDriver();
driver.manage().window().maximize();
driver.get("file:///D:/path/alertpopup.html");
Thread.sleep(2000);
driver.findElement(By.xpath("//button[@onclick='alertFunction()']")).click();
Alert alert=driver.switchTo().alert();
System.out.println(alert.getText());
alert.accept();
}
}
Working with Confirmation Popup
The below is the sample code for Confirmation Popup, make an HTML file and pass it to the webdriver.
The below program will show Confirmation Popup using above html file.
import org.openqa.selenium.Alert;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class ConfirmPopupHandling {
public static void main(String[] args) throws InterruptedException {
// TODO Auto-generated method stub
System.setProperty("webdriver.chrome.driver", "D:\\Drivers\\geckodriver.exe");
WebDriver driver = new ChromeDriver();
driver.manage().window().maximize();
driver.get("file:///D:/path/confirmation.html");
Thread.sleep(2000);
driver.findElement(By.xpath("//button[@onclick='confirmFunction()']")).click();
Alert alert=driver.switchTo().alert();
System.out.println(alert.getText());
alert.dismiss();
}
}
Working with Prompt Popups
Below is the sample code for Prompt Popup, make an HTML file and pass it to the webdriver.
The below program will show Prompt Popup using above html file.
import org.openqa.selenium.Alert;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class PromptPopupHandling {
public static void main(String[] args) throws InterruptedException {
// TODO Auto-generated method stub
System.setProperty("webdriver.chrome.driver", "D:\\Drivers\\geckodriver.exe");
WebDriver driver = new ChromeDriver();
driver.manage().window().maximize();
driver.get("file:///D:/path/prompt.html");
Thread.sleep(2000);
driver.findElement(By.xpath("//button[@onclick='promptFunction()']")).click();
Alert alert=driver.switchTo().alert();
driver.switchTo().alert().sendKeys("Helllo");
alert.accept();
System.out.println(alert.getText());
}
}
Handling Selenium Pop-up window using Webdriver
When we have multiple windows in any application, we may need to switch the control from one window to another window to complete the process. After completion of the process, it has to return to the main window i.e parent window.
Methods to handle multiple windows in selenium webdriver.
- Driver.getWindowHandles();
- Driver.getWindowHandle();
getWindowHandle(): Gets the current window handle, which is opaque to this window that uniquely identifies it within this driver instance. It returns an id pointing to the window which is currently active. The return type is of String type. We use this as a reference to return to the same window after switching multiple windows.
Syntax:
String mainWindow = driver.getWindowHandle();
getWindowHandles(): This method returns a list of all the opened windows related to the specific driver. Its return type is a Set type. Because it eliminates the duplicate instances of the window with the same id if such duplicate instances are present.
Syntax:
Set windowSet = driver.getWindowHandles();
System.out.println(“Total Windows Count: “+windowSet.size());
Programming approach to handling multiple windows
We have two approaches to handle multiple child windows.
Approach# 1: By using the For-Each loop
Approach# 2: By using While loop with Iterator
Approach# 1: By using For-Each loop
String defaultWindow = driver.getWindowHandle(); System.out.println("Default window name is- " +defaultWindow); Thread.sleep(3000); Set<String> childWindows = driver.getWindowHandles(); for(String child : childWindows){ if(!child.equalsIgnoreCase(defaultWindow)){ driver.switchTo().window(child); System.out.println("Child windows- "+child); } else { System.out.println("There are no child windows"); } }
Approach# 2: By using While loop with Iterator
String defaultWindow = driver.getWindowHandle(); System.out.println("Default window name - " +defaultWindow); Thread.sleep(3000); Set<String> childWindows = driver.getWindowHandles(); Iterator<String> iterator = childWindows.iterator(); while(iterator.hasNext()){ String child = iterator.next(); if(!child.equalsIgnoreCase(defaultWindow)){ driver.switchTo().window(child); System.out.println("Child windows- "+child); } else { System.out.println("There are no child windows"); } }
Example:
import java.util.Iterator;
import java.util.Set;
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class Popups {
public static void main(String[] args) throws InterruptedException {
// TODO Auto-generated method stub
System.setProperty("webdriver.chrome.driver"," D:\\Drivers\\geckodriver.exe ");
WebDriver driver = new ChromeDriver();
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
driver.get("https://www.naukri.com/");
driver.manage().window().maximize();
Thread.sleep(4000);
String defaultWindow = driver.getWindowHandle();
System.out.println("The Default window name - " +defaultWindow);
Thread.sleep(3000);
Set<String> allchildWindows = driver.getWindowHandles();
Iterator<String> iterator = allchildWindows.iterator();
while(iterator.hasNext()){
String child = iterator.next();
if(!child.equalsIgnoreCase(defaultWindow)){
driver.switchTo().window(child);
System.out.println("Child windows- "+child);
} else {
System.out.println("There are no child windows");
}
}
driver.close();
driver.quit();
}
}