Constructors in Java
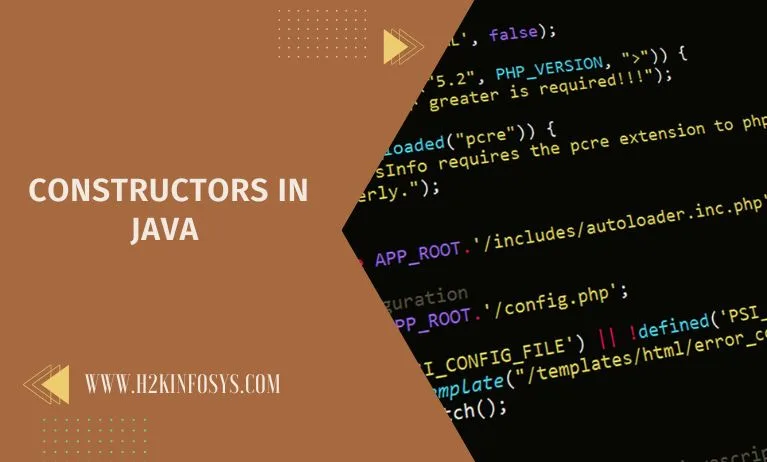
In Java programming, a constructor is a section of code that initialises (constructs) the state and value when an object is created. The Java compiler calls default constructors in Java even if you don’t provide one in the code. Every time an object is created using a new () keyword, it is called. There is no return type for this special member function of a class with the same name as the class. When a class’s new keyword is used to create an object, the class’s constructor is called.
The object is given default states and values, such as 0, null, etc., through the usage of the default constructor. A constructor’s general syntax is as follows:
class ClassName{
ClassName(){ //creating a constructor
}
}
A constructor in Java can do more than just initialise; it can also call methods, create objects, and launch threads. To obtain a constructor’s internal data, Java additionally provides a distinct Constructor class.
Although a constructor and a method are structurally identical, they differ in a number of ways. First of all, a constructor does not have an explicit return type, even though it returns the current class object. Second, unlike a method, it is implicitly invoked. However, there are a few guidelines for writing a constructor in Java, much like for writing a method.
What Are the Rules for Creating Constructors in Java?
There are a total of three rules defined for creating a constructor.
- The constructor’s and class’s names must be identical
- You cannot define an explicit value to a constructor
- A constructor cannot be any of these: static, synchronised, abstract, or final
One thing to note here is that you can have a public, private, or protected constructor in Java by using access modifiers that control object creation.
There are good platforms where you can learn Java online, so it is advisable to check out one of them.
Features of Constructors in Java
Constructors in Java have the following characteristics:
- The constructor name is the same as the class name.
- Explanation: Constructors are distinguished from other member functions of the class by the compiler using this character.
- A constructor cannot have any sort of return. Nothing at all.
- It automatically calls and is frequently employed for initialising values, as explained.
- When an object is created for the class, the constructor is automatically called.
- Contrary to C++ constructors, Java prohibits the definition of a constructor outside of a class.
- Typically, constructors are used to initialise all of the class’s data members (variables).
- Additionally, a class may define many constructors with various parameters. It is referred to as “Constructor Overloading.”
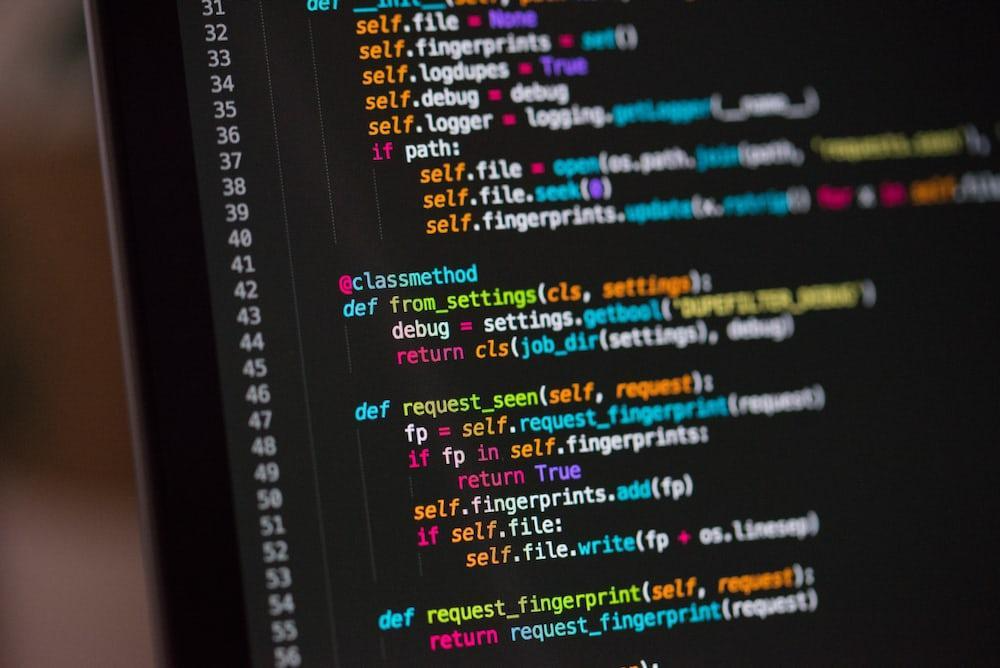
What Are the Different Types of Java Constructors?
Depending on the provided parameters, Java constructors can be of two types, which are:
- No-arg constructors
- Parameterized constructors
1.No-arg Constructors
No-arg constructors, as their name suggests, have no arguments (parameters). The values of every object you initialise using a no-arg constructor will be the same. The Java syntax for a no-arg constructor is:
ClassName(){}
Because it lacks any parameters, the default constructor that the Java compiler calls is also a sort of no-arg constructor.
2.Parameterized Constructors
Parameterized constructors can accept parameters as opposed to no-arg constructors. The constructor can take as many parameters as you like. When creating an object, you may use this kind of constructor to provide it with a variety of values. Here is an illustration of how a parameterized constructor in Java works.
What Does Java’s Constructor Overload Mean?
Java’s constructors resemble methods in many ways. It can therefore get overloaded like the latter. You can create numerous constructors with various argument lists by using constructor overloading. Overloading is the best option if you wish to specify many constructors and have them carry out distinct functions. The parameter lists’ numbers, order, and data types are used by the Java compiler to distinguish between distinct constructors.
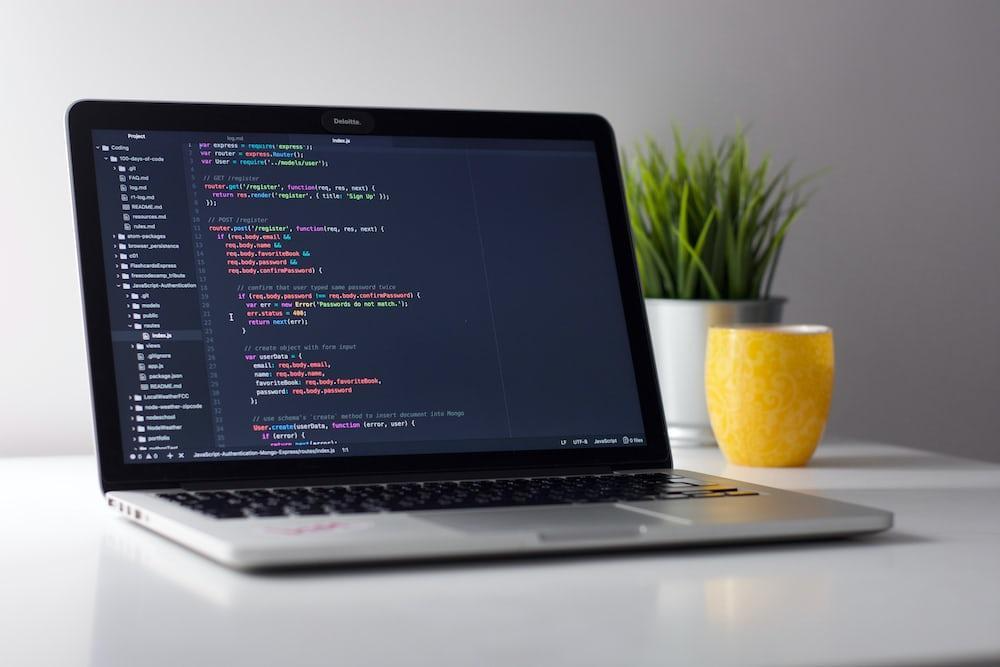
How to Copy Values Without Constructors in Java?
Java doesn’t have a copy constructor. Nevertheless, you may still use a constructor to replicate values from one object to another.
There are a few additional ways to copy values in Java without using a constructor, but one common way is by transferring values between different objects.
Conclusion
One of the most well-liked and sought-after programming languages worldwide is Java. It is crucial to study and comprehend ideas like Java constructors if you want to pursue a profession in software development. A good Java course will explain everything about Constructors in Java, so it is advisable to check out any one of them.