Control Statements in Java
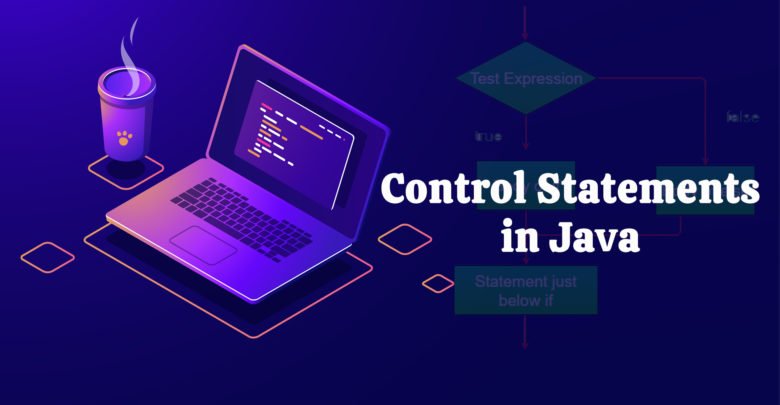
You are already familiar with the term statement, which can simply be defined as an instruction given to the computer to perform specific operations. Control Statements in Java are controlling program flow and determining whether the other statements will be executed or not. Java statement is a complete unit of execution, terminated with a semicolon.
But, imagine you want a statement or a section of code to be executed only under certain conditions that cannot be evaluated until runtime. Or you want a particular segment of code to repeat once for every item in some list.
They can break up the flow of execution by using decision making, looping, and branching, allowing the application to selectively execute particular segments of code. Control flow statements can be applied to single expressions as well as a block of Java code.
As you already know, a block of code in Java is a group of zero or more statements between balanced braces and can be used anywhere a single statement is allowed.
The if-then Statement.
Let’s suppose we only want to execute a block of code under certain circumstances. The if-then statement accomplishes this by allowing our application to execute a particular block of code if and only if during runtime a boolean expression evaluates to true:
For example, let’s print “Good Morning” if the hour of the day is less than 11:
if (hourOfDay < 11)
System.out.println(“Good Morning”);
As you can see from the example, we don’t need curly braces when there is just one statement. We can also, for example, increment some value, morningGreetingCount, every time the greeting is printed. We can easily add one more statement to the code block:
if (hourOfDay < 11) {
System.out.println(“Good Morning”);
morningGreetingCount++;
}
In this case, we added curly braces.
The if-then-else Statement.
Let’s imagine that we want to display a different message if it is 11 a.m. or later. We can use for these two if-then statements:
if(hourOfDay < 11) {
System.out.println(“Good Morning”);
}
if(hourOfDay >= 11) {
System.out.println(“Good Afternoon”);
}
We are performing an evaluation on hourOfDay twice, so this seems redundant. In some cases, the cost of the boolean expression we’re evaluating could be computationally expensive. Fortunately, Java has a more useful approach in the form of an if-then-else statement.
Let’s change our code:
if(hourOfDay < 11) {
System.out.println(“Good Morning”);
} else {
System.out.println(“Good Afternoon”);
}
Now the boolean evaluation happening only once and our code are truly branching between one of the two possible options. The else operator takes a statement or block of statements, in the same way as the if statement does. We can append additional if-then statements to an else block:
if(hourOfDay < 11) {
System.out.println(“Good Morning”);
} else if(hourOfDay < 15) {
System.out.println(“Good Afternoon”);
} else {
System.out.println(“Good Evening”);
}
The execution will continue until it finds an if-then statement that evaluates to true. If neither of the first two expressions is true, it will execute the else block. Important thing is that in the creation of complex if-then-else statements is that order is important. In some cases, we can get the unreachable code.
Ternary Operator. Java language has only one conditional ternary operator in Java. Sometimes better to replace if-then-else statement with it:
<condition> ? <expression1 >: <expression2>
The condition is an operand must be a boolean expression, expression1 and expression2 can be any expression that returns a value.
Let’s look at two snippets of code that are equal:
if-then-else statement:
int y = 10;
final int x;
if(y > 5) {
x = 2 * y;
} else {
x = 3 * y;
}
Ternary operator:
int y = 10;
int x = (y > 5) ? (2 * y) : (3 * y);
For better readability, it is better to add parentheses around the expressions in ternary operations, but it is certainly not required.
The switch Statement
A switch statement is a complex decision-making operator that evaluates a single value and flow is redirected to the first matching case statement. If no such case statement is found that matches the value, an optional default statement (if there is one) will be called. Here is syntax of switch-case:
The value for a case must have the same data type as the variable in the switch. The case values must be unique, duplications are not allowed.
The break statement is optional and it is used inside the switch to terminate a statement sequence. In another case, execution will continue on into the next case. The default can appear anywhere inside the switch block and it is optional.
From JDK7 we can use a string literal/constant in switch statement. The switch statement on Strings can be more expensive than on primitive data types. Better to use switch for strings only in cases controlling data is already in string form. You need to ensure that the expression in any switch statement is not null to prevent a NullPointerException.
The switch statement does a case sensitive comparison. Let’s look at example:
String animal = “dog”;
switch (animal) {
case “dog”:
System.out.println(“Dog is barking”);
break;
case “cat”:
System.out.println(“Cat is fluffy”);
break;
case “bear”:
System.out.println(“Bear loves honey”);
break;
case “mouse”:
System.out.println(“Mouse is small”);
break;
case “elephant”:
System.out.println(“Elephant is hude”);
break;
default:
System.out.println(“I don’t know this animal”);
}
The current output will be “Dog is barking”. If animal variable to “bear” the output will be “Bear loves honey”. If animal equals “lion” the default block will be executed and our output will be “I don’t know this animal”.
What if we miss all break operators? Answer is easy. All Strings will be printed.
The while Statement
The while statement is a repetition control structure, looping statement. This Control Statements in Java executes a part of code (one or more statements) zero or multiple times until the certain termination condition is true. The condition is a boolean expression. Let’s look at the while statement structure:
The while loop has similarities with the if-then statement. Both are composed of a condition and a block of statements. The condition is evaluated before each iteration of the loop and exits if the evaluation returns false. The statement block may never be executed, because that while loop may terminate after its first evaluation of the boolean expression. Let’s create an example
int counter = 5;
while (counter > 0) {
counter–;
System.out.println(counter + “;”);
}
This code will print numbers from 5 to 1, semicolon-separated.
Consider the following code:
int x = 2;
int y = 5;
while(x < 10)
y++;
The loop will never end! The boolean expression will never be modified and always be true. As you see, we got an infinite loop.
The do-while Statement
Java language also allows for the creation of a do-while loop. This type of loop guarantees that the statement or block of statements will be executed at least once. The conditional expression in this type of loop is after the statements. Let’s look at code snippet:
int x = 0;
do {
x++;
} while(false);
System.out.println(x);
The code statement will be executed even the conditional expression is false, and the output of this code will be “1”.
The for Statement
The simple for loop has the same sections as others that we already discussed, but there are also two new sections: an initialization block and an update statement:
For this kind of loop, firstly, the initialization statement is executed, after we check boolean expression. If the condition is true continue, else exit the loop. The body executes and just after this the block of updating the statements is executed.
Here is an example that prints the numbers 0 to 9:
for(int i = 0; i < 10; i++) {
System.out.print(i + ” “);
}
It is also possible to create an infinite loop with this kind of loop:
for( ; ; ) {
System.out.println(“Hello World”);
}
We can add multiple expressions/statements in initialization, condition, updating Control Statements in Java blocks.
The for-each Statement
The for-each loop specifically designed for iterating over arrays and Collection objects. Its declaration consists of an initialization section and an object to be iterated over. The structure of the for-each loop can be found below:
An object to be iterated over has to be a built-in Java array or an object whose class implements java.lang.Iterable. On each loop iteration, the variable on the left-hand side of the statement is assigned a new value from the array or collection on the right-hand side of the statement. For example:
List<String> listOfNumbers = List.of(“one”, “two”, “three”);
for(String number: listOfNumbers) {
System.out.print(number + ” “);
}
The output for this code will be “one two three”. This code snippet can be replaced with for loop:
List<String> listOfNumbers = List.of(“one”, “two”, “three”);
for(int i=0; i < listOfNumbers.size(); i++) {
System.out.print(listOfNumbers.get(i) + ” “);
}
As you can compare, the for-each loop looks more readable.
Nested Loops. Loops can contain other loops. The most common example of nested loop is the iteration over a two-dimensional array:
int sum = 0;
int[][] complexArray = {{1,2,3},{4,5,6},{7,8,9}};
for(int[] simpleArray : complexArray) {
for(int i=0; i < simpleArray.length; i++) {
sum += simpleArray[i];
}
}
System.out.println(sum);
As the output of this code, you will get printed the sum of all elements of the two-dimensional matrix. In this code for loop and for-each loop are intentionally mixed, it is not convenient. I just wanted to show that the same logic can be written in many ways.
7 Comments