How to design a Class in Java ?
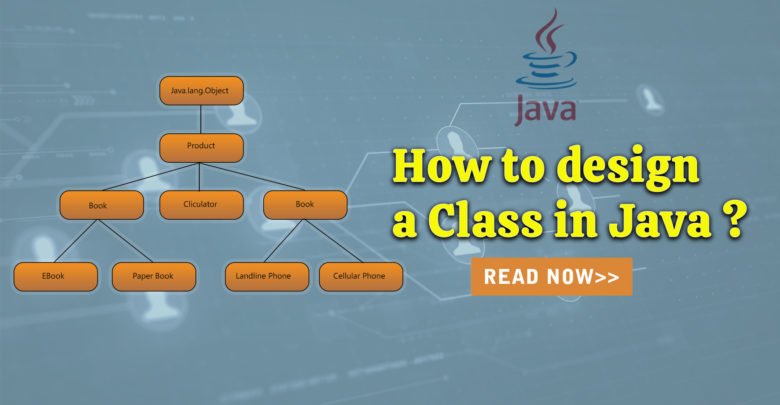
Learn how to design a class in java. Classes are the basic building blocks of Java programs. That building block has own parts and characteristics. If you want to use a class, you have to create an object. The object is a runtime instance of a class in memory. All various objects of all different classes represent a state of your program.
The class declaration includes these components, in order:
Modifiers. The Class can be public, private or default.
Class name. By convention, it should begin with a capitalized letter. You cannot use Java reserved words. Names can contain numbers.
Superclass (optionally). If your class has a parent, you need to put the name of it after the keyword extends. A class can only extend one superclass.
Interfaces (optionally). If your class implements an interface or interfaces, you need to write them like a comma-separated list after the keyword implements.
Body. The class body is surrounded by braces.
The simplest Java class you can write looks like this:
public class Animal {
}
Example of a class that has a parent:
public class Dog extends Animal {
}
Example of a class that implements an interface:
public class Dog implements Eatable {
}
The class can have a parent and implement several interfaces at once:
public class Dog extends Animal implements Eatable, Adaptable{
}
Body of Java classes has two primary elements: methods and fields. Methods are called procedures or functions in other languages. Fields are also known as variables. Methods and fields together are called the members of the class. Variables contain the states of the program, methods operate on those states. In other words, variables are responsible for properties, and methods are responsible for class behavior. That’s all classes really do.
You can find more information about fields in the chapter dedicated to Java variables and data types.
Let’s take a closer look at methods. The method is a collection of statements that has some specific goal and can return the result to the caller. Also, the method can perform some specific tasks without returning anything. For example, you just need to delete files from some specific directory.
The method declaration has six components:
-
- The modifier defines where your method can be accessed in your application. There are four types of access for methods in Java: public(accessible in all classes of your application), protected(accessible within the class in which it is defined and in subclasses), private (accessible only within the class in which it is defined), default (accessible within same class and package within which its class is defined).
- The return type is a data type of the value that method returns. It can be void if the method does not return a value.
- Method name. Rules for field names apply to method names as well, but the convention is a bit different.
- A list of parameters is a comma-separated list of input data preceded with their data type, within the enclosed parenthesis. For methods without parameters, you must use empty parentheses ().
- A list of exceptions is a comma-separated. There are all exceptions you can expect from the method.
- The method body is enclosed between braces. It’s code you need to be executed to perform your intended operations.
The method signature is included in the method declaration. It consists of the method name and parameter list (ordered parameters with their types). The return type and exceptions are not a part of the method signature.
The method signature of the above function will be sum(int x, int y).
The Java compiler can discern the difference between the methods through their method signatures. It is allowed to write methods that have the same name but accept different parameters. We can easily create one more method with name sum in the same class:
public int sum(int x, int y, int z) {
return x+y+z;
}
The method signature for this method will be sum(int x, int y, int z).
The method name is typically a lowercase single word that should be a verb or several words that preferably begins with a verb in lowercase followed by an adjective-noun. Starting from the second word first letter of each next word should be capitalized. For example, sendEmail, findMaximum, findFilesByName.
There is a special types of methods that are called constructors. Constructors are using for the creation of new objects. It is a special method that has no return type and matches the name of the class. For example:
public class Cat {
public Cat() {
System.out.println(“constructor”);
}
}
You can find more information about constructors in the dedicated paragraph.
Each Java class we usually put in its own file with extension *.java . Classes are usually public, which means they can be called by any code. There is no requirement in Java for the class to be public. The public class needs to match the name of its file. For example, public class Animal has to be in a file named Animal.java.
Two or more classes can be created in the same file. But if you do so, one of the classes in the file is allowed to be public. That means that a file Animal.java containing the following is also fine:
public class Animal {
}
class Animal2 {
}
Java has several optional specifiers that can be applied to both fields and methods of a class.
If field or method that is static can be accessed without an instance of the class. As an example of a static variable can be the field that counts of how many instances of a class have been created:
public class Rabbit {
public static int numRabbits = 0;
public Rabbit() {
numRabbits++;
}
}
Static methods do not have any access to instance fields, because instance fields store information about an instance of a class. Also, static methods do not have access to keyword this, because this refers to the current instance of a class. We use static methods for functionality that is universal to a class. Here is an example of a static method :
public class Rabbit {
public static int numRabbits() {
return 5;
}
}
We can call this method in this way:
Rabbit.numRabbits();
The field or method can be final. If you declare variables with this non-access modifier, its value can’t be modified. This also means that you must initialize a final variable. This keyword is for constants. If the final field is a reference, this means that the variable cannot be re-bound to a reference of another object. But the initial state of the object pointed by that reference can be changed. For example, if you have a final array, you can add or remove elements from it.
As it was said before, we must initialize a final variable, because the compiler will throw compile-time error. It can be initialized only one time. There are several places to initialize the final field: during declaration, inside the constructor, inside static blocks or inside the instance-initializer block. Here is an example with initialization during declaration:
public class Rabbit{
public final int MAX_WEIGHT = 5;
}
If a method is declared with the final keyword, it cannot be overridden. You are not allowed to modify a final method from a subclass. The main intention of making the final method is that the content of the method cannot be changed by any outsider.
In Java classes can be abstract, they are declared using keyword abstract. A normal class (non-abstract class) cannot have abstract methods. An abstract class can have abstract methods(methods without body) as well as regular methods with the body.
Java Objects
As was said before, if you want to use your class, you have to create a java object, because the class is just a specification for the object. When you define a class, no memory or storage is allocated, you don’t have an instance.
Let’s look at the class below:
public class FacebookUser {
private String username;
private String location;
private int age;
public FacebookUser (String username, String location, int age) {
this.username = username;
this.location = location;
this.age = age;
}
public void writeMessage(FacebookUser recipient, String message) {
recipient.readMessage(message)
}
public void readMessage(String message) {
System.out.println(username + ” received the message: ” +message);
}
}
Here we have the class FacebookUser that contains some common characteristics for Facebook users. Let’s create particular instances with particular characteristics:
FacebookUser userMary = new FacebookUser(“Mary”, “Spain”, 27);
FacebookUser userMarko = new FacebookUser(“Marko”, “Italy”, 35);
Now we have two different objects of the class FacebookUser, and they are created by the same template.
In class FacebookUser, we also have two methods, that allow for a Facebook user to read and to write messages. We can say that two objects userMary, userMarko have the same interface.
Let’s call methods of objects, pretending that Marko sends a message to Mary and she sends an answer:
userMarko.writeMessage(userMary, “Hello! You are beautiful.”);
userMary.writeMessage(userMarko. “Hello! Thanks!”);
The result of this code snippet will be:
Mary received the message: Hello! You are beautiful.
Marko received the message: Hello! Thanks!
As you can see from our example, we are calling methods from particular objects, and, as a result of our call, we got corresponding usernames in our messages.
11 Comments