Different Types of Exception Handling in Selenium Webdriver
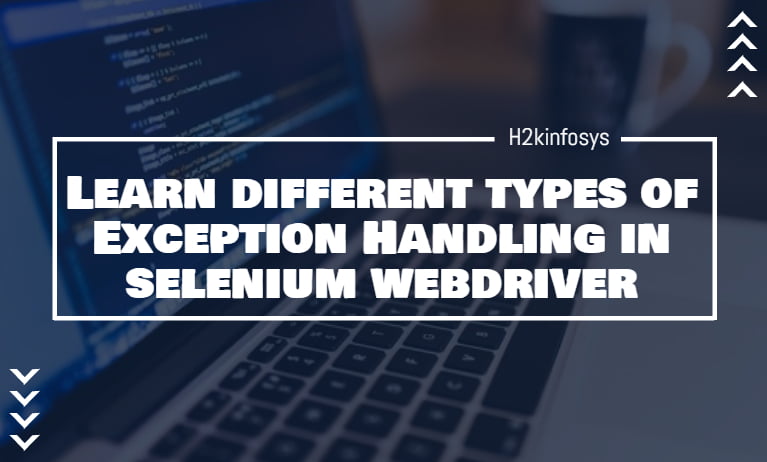
Exception handling in Selenium WebDriver is one of the most common phenomena during automation code execution. As the name suggests, the exceptions are the rare or uncommon cases that arise due to various reasons. The whole idea is to catch such bugs proactively. Therefore, Exception Handling is the process of smartly handling the rare errors before they occur. These errors might arise due to various reasons such as incorrect syntaxes, wrong parameter passing, or when certain requested support is not available.
What is an Exception in the Selenium framework?
When a test script written in Selenium framework fails due to any of the various reasons, the developer should be able to get hold of the reason behind it. They should take the necessary steps to handle the failure of the program so that it runs uninterrupted.
So, what is an exception?
An exception is essentially an uncommon error that occurs during the program execution. When an exception happens, the program flow is broken or gets interrupted without producing the desired output.
Therefore, these exceptions need to be caught and handled just so the normal flow of program execution is carried out.
How are exceptions handled?
- The piece of code that is likely to fail should be placed within the try block.
- The JVM executes the catch block after the catch statement takes the exception as the parameter and catches it.
- In case of no exception, the JVM executes just the try statement.
try{ /* Code */}catch(Exception e){ /* Catch block */}
Tip: It is recommended to avoid the common anticipated exceptions and catch the truly unavoidable exceptions.
Types of Exceptions in Selenium Automation
There are three types of exceptions in Selenium Automation:
- Checked Exception
- Unchecked Exception
- Error
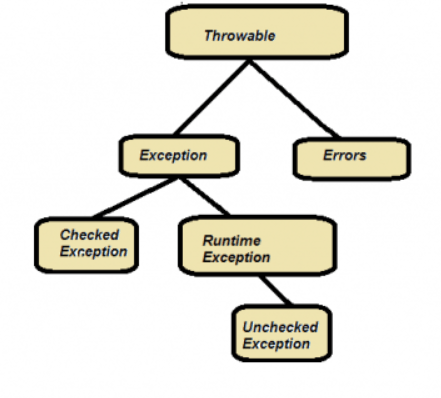
Checked Exception
The compiler handles these exceptions during the compile-time, else it shows a compilation error in case of failure to use the catch statement
Example: IOException, SQLException, ClassNotFoundException
Unchecked Exception
The compiler is not concerned about these exceptions. These go undetected during the compile-time.
Example: ArrayIndexOutOfBoundsException, ArithmeticException, ArrayStoreException
Error
The JVM throws an error when the situation is out of hands during the execution of the program. The try-catch block cannot handle the errors. Even though the developer tries to catch the errors, the escape them and eventually, it falls into the lap of JVM.
Example: OutOfMemoryError
Exception Handling in Selenium WebDriver using Java
There are 3 ways to handle exceptions:
- Try-catch block
- Using Throws keyword
- Finally block
Try-catch block:
We have already seen how this works in the earlier section.
Example:
try { int[] numbers = {1, 2, 3}; System.out.println(numbers[10]); } catch(Exception e) { System.out.println("Array really went awry:" + e);}
Note: There can be multiple catch blocks for each try block.
Using Throws keyword:
The throws keyword is used to throw an exception rather than handling it. The checked exceptions can be thrown by the methods.
Example 1:
public static void main(String[] args) throws ArrayIndexOutOfBoundsException { int[] numbers = {1, 2, 3}; System.out.println(numbers[10]); System.out.println("Array really went awry:"); }
Example 2:
static void ageCheck(int age) { if (age < 15) { throw new ArithmeticException("Stop - You must be at least 15 years old to play this game."); } else { System.out.println("Welcome!"); } } public static void main(String[] args) { ageCheck(14); // Set age to 14 (which is below 15...)}
Finally block:
The ‘finally’ block gets executed soon after the try-catch block irrespective of whether try gets executed or catch. Also, the ‘finally’ block can follow soon after the try block where the catch block can be skipped. Also, unlike catch blocks, there can be only a single finally block.
Example:
try { int[] numbers = {1, 2, 3}; System.out.println(numbers[10]); } catch(Exception e) { System.out.println("Array really went awry:" + e); finally{ System.out.println("Done with the try-catch block"); }
In the above case, the ‘finally’ block gets executed soon after the catch block.
One Comment