HashMap in Java
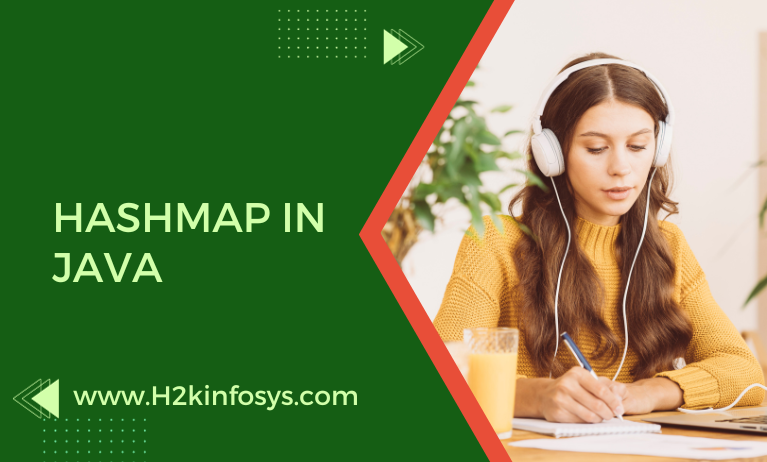
HashMap, also known as HashMapKey, Value> or HashMapK, V>, is a Map-based collection class that is used to store Key & value pairs. With the exception of the fact that it is unsynchronized and allows nulls (null values and null keys), Java’sHashMap in Java class is similar to the Hashtable class.
It does not return the keys and values in the same order in which they were placed into the HashMap because it is not an ordered collection. The stored keys and values are not sorted. To utilise the HashMap class and methods, you must import java.util.HashMap or its superclass. You can learn more about HashMap in Java by checking the Java training online.
Let’s now get into the specifics of HashMap.
- The key in key-value pairs needs to be distinct.
- It lacks synchronisation. You can synchronise it in any case.
- Does not preserve the sequence of insertion.
- Does not sort the elements
- Null keys and values are supported. However, there can be only one null key. The number of null values is unlimited.
How to declare HashMap in Java?
HashMap<K, V> = new HashMap<K, V>();
Where K stands for the key’s type in a key-value pair.
V stands for the value’s type in a key-value pair.
For instance: It is possible to declare a hashmap with integer keys and string values as follows:
HashMap<Integer, String> hmap = new HashMap<Integer, String>();
HashMap in Java Examples
- Adding elements to HashMap
To add new key-value pairs to the HashMap, utilise the put() function of the HashMap class. The entrySet() method is being used to iterate the hashmap. An analogous Set is returned by this method. This is how you provide the Map of the key-value pairs.HashMap key-value pairs can be printed using the methods getKey() and getValue() found in the entry. Key-value pairs are written as the elements of the hash map are explored using a for loop.
- Checking duplicate key insertion in HashMap
We are attempting to add an element with a duplicate key in this case. The hash map already has the key value 111, which is used in the new element (111,”Karate”). HashMap modified the value of the already existing element with key “111” rather than adding this new element.
- HashMap remove() method
- HashMap replace() method
Internal working of HashMap in Java
Hashing is a method that is used by HashMap internally to create indexes for keys. This indexing facilitates quicker record searches. As a result, activities like search, update, and deletion can respond more quickly.
When we execute the following statement, we call the HashMap put() method. It uses the hash function to determine the key’s hash code, which in this case is 101. The hashing method is used to determine this hash code. This hash code is given an index. This key-value pair is identified exclusively by this index.
Hash Collision occurs when a duplicate key is added to the hash map. HashMap responds to this by replacing the previous value with the present one.
HashMap Class Methods
Here is a list of the HashMap class’s available methods. After reading this article, you can also check out additional covered examples employing these techniques.
- void clear(): This function clears the supplied Map’s key-value pairs.
- Using the object clone() function, all of a map’s mappings can be copied and pasted into another map.
- containsKey(Object key) is a boolean function that determines whether the specified key is present in the map by returning true or false.
- boolean containsValue(Object Value): This method is similar to containsKey() in that it searches for the supplied value rather than the specified key.
- Value get(Object key): It returns the value associated with the given key.
- The boolean function isEmpty() determines if the map is empty. This function returns true if there are no key-value mappings in the map; otherwise, it returns false.
- KeySet(): This function retrieves the Set of keys that were fetched from the map.
- key value mapping is added to the map using value put(Key k, Value v). used in the example up top.
- Returns the number of key-value mappings and the size of the map as an integer.
- Collection values(): This function returns a group of map values.
- Removes the key-value pair for the given key when using the value remove(Object key). used in the example up top.
- void putAll(Map m): Copies all of a map’s elements to the other map that is supplied.
Conclusion To learn more about HashMap in Java, check out the free Java course online.