Hibernate Overview
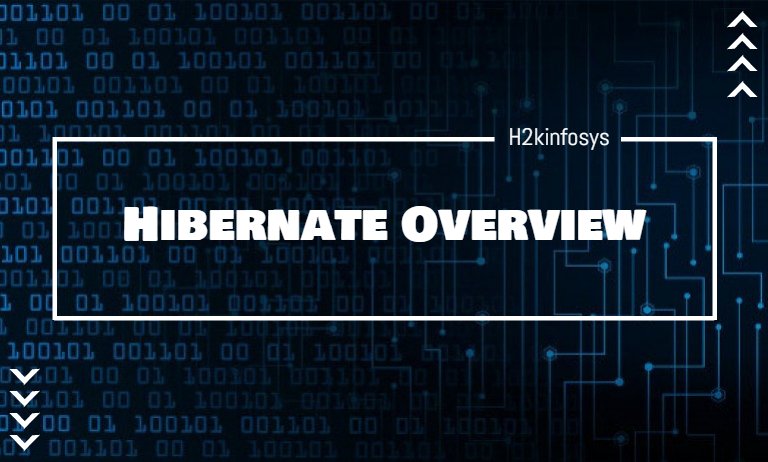
Hibernate is a widely used Object-Relational Mapping (ORM) tool in the JAVA world. It was created by Gavin King in 2001 as an open-source persistent framework. It is a powerful Object-Relational Persistence and Query service that provides high-performance mapping an object-oriented domain model to a relational database.
Object-Relational Mapping(ORM) technologies are responsible for saving/reading/editing/deleting the objects from our application to the relational database tables. They protect developers from work with SQL languages. Each relational database has its own standards of SQL language. Therefore, ORM makes our Java application more flexible.
Hibernate helps to map Java classes to database tables and converts Java data types to SQL data types. Therefore, the developer can be free from 90% of common data persistence related programming tasks.
Hibernate framework handles all the works of persisting traditional Java objects to database server based on the appropriate Object-Relational mechanisms and patterns.
The ORM technique is designed to let the business code access objects rather than DB tables. In applications that use ORM, we hide details of SQL queries from object-oriented logic. Anyway, ORMs are based on JDBC ‘under the hood.’ Developers don’t need to deal with database implementation. Entitiesapplication turns to application based on business concepts rather than the structure of the database. WIth ORM we get all the transaction management and automatic key generation. Our application development is much quicker. ORMs improve the maintainability of applications. You need to write a lot less code and do not need to deal with the database implementation. ORMs improve portability because generate database-specific SQL for you, Finally, entities are more based on business concepts rather than a database structure.
There is a bunch of persistent frameworks and ORMs for Java language: Enterprise JavaBeans Entity Beans, Java Data Objects, Castor, TopLink, Spring DAO, Hibernate, etc. We will look closer at Hibernate.
Features of Hibernate
- It minimizes the number of database accesses with smart fetching strategies
- You can save, retrieve, update, and delete your domain objects without writing any SQL queries.
- With Hibernate you can change names and columns to table.
- This technique centralizes pre-save and post-retrieve logic and also it can create complex joins for retrieving related items.
- Hibernate automatically creates schema from an object model.
- For Hibernate you don’t need any application server to operate.
- It can manipulate pretty complex associations of objects of your database.
– Hibernate has innovative solutions on caching, proxy, and lazy loading, which considerably helps to improve the performance of your Java Web application.
You can configure related objects to cascade operations from one to the other. For example, you can configure some parent object to cascade its save and/or delete operation to its child objects.
– It provides its own language called Hibernate Query Language (HQL). This language allows SQL-like queries to be written against Hibernate’s data objects. The HQL is the object-oriented version of the SQL language. Queries written in this language are database independent. Therefore, you do not need to write database-specific queries. Even more, If you change the database structure you do not require individual SQL queries to be changed as well. So, no maintenance issues in the future.
Hibernate has also Criteria Queries that are an object-oriented alternative to HQL. Criteria They are used for object modification and to provide the restriction for the objects.
Hibernate Application Architecture
Hibernate has a layered architecture that hides from the developer the underlying APIs. He does not need to learn it. Hibernate uses the database and configuration data and provides persistence services and persistent objects to the application.
Following is a high-level view of the Hibernate Application Architecture.
Hibernate has five core interfaces that are used in applications to work on persistent objects and to control transactions.
Configuration.
The first Hibernate object that you create for your Hibernate application is a Configuration object. Usually, we create just one Configuration object during application initialization. It is represented as a properties file. Hibernate requires it.
In the Configuration object, we have to describe two keys components: Database Connection and Class Mapping Setup
Database Connection is handled through one or more configuration files, such as hibernate.properties and hibernate.cfg.xml.
Class Mapping Setup creates a connection between the Java classes and database tables:
Configuration configuration = new Configuration(); configuration.configure("/hibernate.cfg.xml"); ServiceRegistry srvcReg = new StandardServiceRegistryBuilder().applySettings(configuration.getProperties()) .build();
SessionFactory
You can create a SessionFactory object with the use of the Configuration object. SessionFactory object configures Hibernate for the application using the configuration file. It is responsible for a Session object instantiation. The SessionFactory is used by all the threads of an application, it is a thread-safe object.
The SessionFactory is a heavyweight object. Therefore, it is usually created during application startup. Developers need one SessionFactory object per database, each instance needs a separate configuration file. Therefore, one database – one SessionFactory object. Here is the way to retrieve SessionFactory:
SessionFactory sessionFactory = configuration.buildSessionFactory();
Session
If you need to get a physical connection with our database, you need to create a Session object. This object is lightweight because it was designed to be instantiated each time you need interaction with the database. All work with persistent objects (saving, retrieving) is made through a Session object.
The session objects are not usually thread-safe and should be destroyed as soon as we can release them.
The Session lifecycle is between the beginning and end of a logical transaction. The main function of the Session are operations for instances of mapped entity classes.
These instances may have one of three states: transient(not associated with any Session), persistent (associated with a Session), detached(was previously persistent, but now not associated with any Session).
Hibernate implementation of Session has the following methods:
beginTransaction() – method begins a unit of work. It returns the associated Transaction object. Once our DB interactions are done, we need to invoke method commit() on the object returned by the transaction. There is a possibility to rollback operations in case of any issues. In this case call rollback() on the transaction object.
save() – method persists the given object. Before it, the method assigning a generated identifier for the object.
update()- method updates the database record.Also, it updates the persistent instance with the detached instance identifier.
saveOrUpdate() – this method can do the same as both save(Object) or update(Object) methods together. This operation cascades if the instances are mapped with cascade=”save-update”.
createQuery() – method uses a given HQL query string and creates an instance of Query.
createSQLQuery() – method uses SQL query string to create a new instance of SQLQuery.
merge() – method copies the fields of the particular object onto the persistent object that has the same identifier. In case, there is no associated with the session persistent instance, it will be loaded. The method returns the persistent instance. It can be that the given instance is unsaved. In this case, the method saves a copy of that instance and returns it as a newly persistent instance. This operation cascades if the instances are mapped with cascade=”merge”.
persist() – method persists a transient instance. This operation cascades if the instances are mapped with cascade=”persist”.
flush() – the method forces this session to flush. It is usually called before committing the transaction and closing the session, at the end of a unit of work. The flush() method synchronizes the underlying persistent store with the persistable state in memory.
delete() – the method removes an instance from the datastore. This operation cascades if the instances are mapped with cascade=”delete”.
Transaction. A transaction to database is a unit of work. In Hibernate we handle Transactions by a transaction manager.
This object is optional in Hibernate applications. Developers may choose for his Hibernate applications not to use this interface. We can instead to manage transactions in our own application code. Let’s look at code snippet to understand how to work with transaction:
Session session = null; Transaction transaction = null; try { session = sessionFactory.openSession(); transaction = session.beginTransaction(); someAction(session); transaction.commit(); } catch(RuntimeException exc) { transaction.rollback(); } finally { session.close(); }
It is a String that is using to retrieve data from the database, create objects, delete objects. The instance of a Query object is used to bind query parameters with the query, to execute the query, and to return limit the number of results by the query.
Let’s look at the list of Query methods in the Hibernate implementation:
list() – method returns the query results as a List. The results can be returned in an instance of Object[], in case the query contains multiple results.
executeUpdate() – method executes the update or delete statement and returns the number of updated or deleted entities.
setParameter() – method binds parameter with query.
uniqueResult() – method returns one instance that matches the query, or null if no instances found.
setMaxResults() – method sets the maximum number of retrieved rows.
setFirstResult() – method sets the first row to beginning retrieve from.
Criteria are used for object-oriented queries.
3 Comments