Hibernate Program
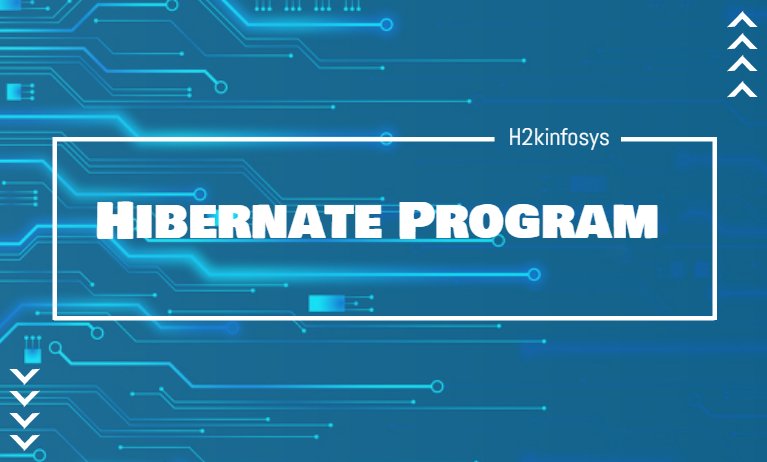
Let’s create our first Java application with Hibernate technology.
One of the simplest ways is to create a basic Maven project and to add Hibernate dependency to pom.xml. The version of the Hibernate framework can vary:
<dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-agroal</artifactId> <version>5.4.17.Final</version> <type>pom</type> </dependency>
We are going to use the MySQL database. So, you need to make sure your MySQL database already have setup. Also, we will need the JDBC driver for MySQL. As we have the maven project, we just need to add to pom.xml dependencies to the library :
<!-- https://mvnrepository.com/artifact/mysql/mysql-connector-java --> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>8.0.20</version> </dependency>
As the next preparation step, we need to create a MySQL database. For this example, we created “exampledb” and one database table Customer. Here is the SQL query that was run for the table creation:
The Java POJO(Plain Old Java Object) class is a class with fields, constructors, getters, and setters. Methods getXXX and setXXXwill make it JavaBeans compliant class.
A POJO doesn’t extend some specialized classes or implement interfaces. If you create a class that will be persisted by Hibernate, it is important to provide JavaBeans compliant and create one attribute, which will work as an ID.
Let’s create a corresponding Java POJO class in our application:
package edu.hibernate.example; public class Customer { private int id; private String firstName; private String lastName; private String email; public Customer (String firstName, String lastName, String email) { super(); this.firstName = firstName; this.lastName = lastName; this.email = email; } public Customer() { } public int getId() { return id; } public void setId(int id) { this.id = id; } public String getFirstName() { return firstName; } public void setFirstName(String firstName) { this.firstName = firstName; } public String getLastName() { return lastName; } public void setLastName(String lastName) { this.lastName = lastName; } public String getEmail() { return email; } public void setEmail(String email) { this.email = email; } }
To relate database table with java class we need to create xml-file Customer.hbh.xml in the resources folder:
<?xml version = "1.0" encoding = "utf-8"?> <!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD//EN" "http://www.hibernate.org/dtd/hibernate-mapping-3.0.dtd"> <hibernate-mapping> <class name = "edu.hibernate.example.Customer" table = "CUSTOMER"> <meta attribute = "class-description"> This class contains the customer detail. </meta> <id name = "id" type = "int" column = "id"> <generator class="native"/> </id> <property name = "firstName" column = "first_name" type = "string"/> <property name = "lastName" column = "last_name" type = "string"/> <property name = "email" column = "email" type = "string"/> </class> </hibernate-mapping>
Now we need to create a configuration file in our application with a set of settings related to MySQL database and other related parameters. Developers that work on applications that include the Hibernate technology place all such information as a standard Java properties file called hibernate.properties. Also, there is an option to supply this information as an XML file named hibernate.cfg.xml.
In our examples, we will use the way with XML formatted file hibernate.cfg.xml to specify required Hibernate properties. If some of the properties are not specified Hibernate takes the default value. Most of the properties are not required to specify them in the property file, but there are some mandatory properties. This file hibernate.cfg.xml location is in the resources folder. Let’s look at the list of the important Hibernate properties that we used in our configuration file. They are required to be configured for a database in a regular situation:
hibernate.dialect – the property is responsible to pass information to Hibernate so it will generate the appropriate SQL for the chosen database.
hibernate.connection.driver_class – this property holds the JDBC driver class.
hibernate.connection.url – property contains the JDBC URL to the database instance.
hibernate.connection.username – property to place the database username.
hibernate.connection.password – property to place the database password.
<?xml version = "1.0" encoding = "utf-8"?> <!DOCTYPE hibernate-configuration SYSTEM "http://www.hibernate.org/dtd/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <property name="hibernate.dialect"> org.hibernate.dialect.MySQLDialect </property> <property name="hibernate.connection.driver_class"> com.mysql.jdbc.Driver </property> <!-- Assume test is the database name --> <property name="hibernate.connection.url"> jdbc:mysql://localhost/exampledb?serverTimezone=UTC </property> <property name="hibernate.connection.username"> root </property> <property name="hibernate.connection.password"> root </property> <!-- List of XML mapping files --> <mapping resource="Customer.hbm.xml" /> </session-factory> </hibernate-configuration>
Finally, we are ready to create the instance of our Customer class and to interact with it. Let’s create the one, persist, update one of the properties, delete. We will list all the database instances after each of the steps. First of all, we will create a class that will be responsible for all actions on the Customer instances:
package edu.hibernate.example;
import java.util.List; import java.util.Iterator; import org.hibernate.HibernateException; import org.hibernate.Session; import org.hibernate.Transaction; import org.hibernate.SessionFactory; import org.hibernate.cfg.Configuration; public class CustomerService { private static SessionFactory factory; public CustomerService() { try { factory = new Configuration().configure().buildSessionFactory(); } catch (Throwable ex) { System.err.println("Failed to create sessionFactory object." + ex); throw new ExceptionInInitializerError(ex); } } public Integer addCustomer(String firstname, String lastname, String email) { Session session = factory.openSession(); Transaction transaction = null; Integer customerID = null; try { transaction = session.beginTransaction(); Customer customer = new Customer(firstname, lastname, email); customerID = (Integer) session.save(customer); transaction.commit(); } catch (HibernateException e) { if (transaction != null) transaction.rollback(); e.printStackTrace(); } finally { session.close(); } return customerID; } public List listCustomers() { Session session = factory.openSession(); Transaction transaction = null; List customers = null; try { transaction = session.beginTransaction(); customers = session.createQuery("FROM Customer").list(); transaction.commit(); return customers; } catch (HibernateException e) { if (transaction != null) transaction.rollback(); e.printStackTrace(); } finally { session.close(); } return customers; } public void updateCustomer(Integer CustomerID, String email) { Session session = factory.openSession(); Transaction transaction = null; try { transaction = session.beginTransaction(); Customer customer = (Customer) session.get(Customer.class, CustomerID); customer.setEmail(email); session.update(customer); transaction.commit(); } catch (HibernateException e) { if (transaction != null) transaction.rollback(); e.printStackTrace(); } finally { session.close(); } } public void deleteCustomer(Integer CustomerID) { Session session = factory.openSession(); Transaction transaction = null; try { transaction = session.beginTransaction(); Customer customer = (Customer) session.get(Customer.class, CustomerID); session.delete(customer); transaction.commit(); } catch (HibernateException e) { if (transaction != null) transaction.rollback(); e.printStackTrace(); } finally { session.close(); } } }
Finally, we got this project hierarchy:
Here is our main method of the application:
package edu.hibernate.example; import java.util.Iterator; import java.util.List; public class HibernateExample { public static void main(String[] args) { CustomerService customerService = new CustomerService(); Integer customerID1 = customerService.addCustomer("Bob", "Lie", "[email protected]"); System.out.println("List of Customers after creation of the new record:"); printListCustomers(customerService.listCustomers()); customerService.updateCustomer(customerID1, "[email protected]"); System.out.println("List of Customers after updating the record:"); printListCustomers(customerService.listCustomers()); customerService.deleteCustomer(customerID1); System.out.println("List of Customers after deleting the record:"); printListCustomers(customerService.listCustomers()); } private static void printListCustomers(List customers) { if(!customers.iterator().hasNext()) { System.out.println("The list is empty!"); } for (Iterator iterator = customers.iterator(); iterator.hasNext();) { Customer customer = (Customer) iterator.next(); System.out.print("First Name: " + customer.getFirstName()); System.out.print(" Last Name: " + customer.getLastName()); System.out.println(" Email: " + customer.getEmail()); } } }
The output of our application is:
First Name: Bob Last Name: Lie Email: [email protected]
List of Customers after updating the record:
First Name: Bob Last Name: Lie Email: [email protected]
List of Customers after deleting the record:
The list is empty!
So, we successfully created the Java project and implemented base CRUD operations (create, read, update, delete) with the help of the Hibernate framework and with the use of MySQL database.
We have used the XML mapping file for the transformation. But there is another way to do this. The Hibernate annotations are a new way to do this without the use of the XML file. You can use mixed-method: annotations and XML mapping together.
In this case, our Java POJO class will look like this:
package edu.hibernate.example; import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.Id; import javax.persistence.Table; @Entity @Table(name = "CUSTOMER") public class Customer { @Id @GeneratedValue @Column(name = "id") private int id; @Column(name = "first_name") private String firstName; @Column(name = "last_name") private String lastName; @Column(name = "email") private String email; public Customer (String firstName, String lastName, String email) { super(); this.firstName = firstName; this.lastName = lastName; this.email = email; } public Customer() { } public int getId() { return id; } public void setId(int id) { this.id = id; } public String getFirstName() { return firstName; } public void setFirstName(String firstName) { this.firstName = firstName; } public String getLastName() { return lastName; } public void setLastName(String lastName) { this.lastName = lastName; } public String getEmail() { return email; } public void setEmail(String email) { this.email = email; } }
There are more annotations. There is the way to define one-to-one, one-to-many and many-to-many relationships between classes with annotations too.
We will also need to do small changes inCustomerService class for SessionFactory builder:
public class CustomerService { private static SessionFactory factory; public CustomerService() { try { factory = new AnnotationConfiguration(). configure(). //addPackage("edu.hibernate.example") //add package if used. addAnnotatedClass(Customer.class). buildSessionFactory(); } catch (Throwable ex) { System.err.println("Failed to create sessionFactory object." + ex); throw new ExceptionInInitializerError(ex); } } ... }
Other code in our code wouldn’t be changed. All the annotations are from javax.persistence package. XML mappings have greater flexibility, but for portability to other EJB 3 compliant ORM applications, you must use annotations.