How to Set Up Node/NPM?
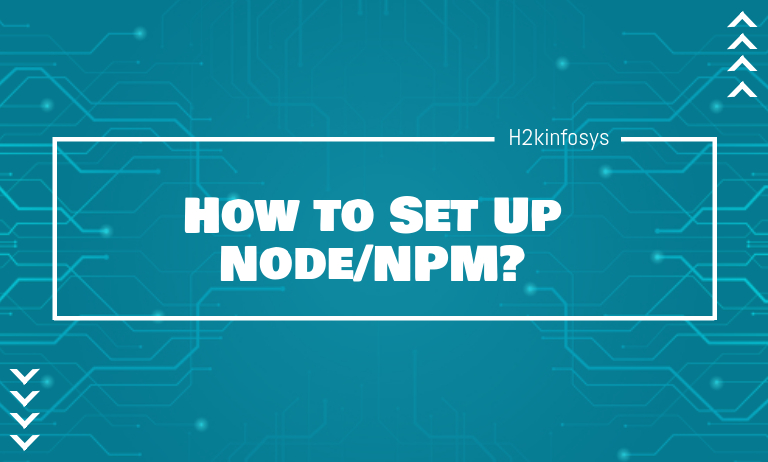
NPM package manager for the Node.js using the packages, or modules if you like. www.npmjs.com hosts thousands of free packages to download and use. NPM program should be installed on your computer when you install Node.js. Now, NPM is ready to run on your computer!
What is a Package?
A package in Node.js which contains all the files which are required for a module. Modules are the JavaScript libraries that we can include in your project.
Introduction to React:
The React is a front-end framework that is used to create a UI. JSX is a JavaScript syntax extension, which is typically used to React to describe the UI elements. A web pack is a pack of the JavaScript files which can run in a browser.
Setting up node
Step 1: Once you have the project directory, navigate to the project directory, and initialize the project using npm.
mkdir node-project
cd node-project
npm init
The above command will ask for a couple of details like name, version, git, etc. related to the Node.js project. Enter the details, and you will have the project created with a package.json file.
{ "name": "project", "version": "1.0.1", "description": "Test", "main": "home.js", "scripts": { "test": "echo \"Error: no test is specified\" && exit 1" }, "author": "", "license": "IS." }
The package1.json file will have all the information related to the Node.js project. Information related to all node package modules installed in the project can be saved to the package.json file using the –save option.
Creating Node.js Express Project
Let’s start by installing the express framework to the Node.js project. Using npm, install the express framework.
# installing express web framework
npm install express --save
The above command installs the express framework to the node-project. Now, if you check the package1.json file, you will have the express module details saved in the package1.json file as a dependency.
{ "name": "project", "version": "1.0.1", "description": "Test", "main": "home.js", "scripts": { "test": "echo \"Error: no test is specified\" && exit 1" }, "author": "", "license": "IS", "dependencies": { "express": "^4.16.4" } }
Create a file called app1.js and add the following code to it.
const express = require('express') const app = express() const port = 3000 app.get('/', (req, res) => res.send("Welcome to setting up Node.js project !')) app.listen(port, () => console.log(`Application listen
Step 2: Installing create-react-app
By using NodeJS/NPM should be installed on your machine, so you can run the command:
npm install -g create-react-app
Now, install create-react-app globally so we can use it anywhere.
Let’s Create a New React App
Use create-react-app installed, so we can create a new app by running this command:
create-react-app my-react-tutorial-app
cd my-react-tutorial-app
The above command will use to create a new directory.
my-react-tutorial-app { "name": "my-react-tutorial-app", "version": "0.1.0", "private": true, "dependencies": { "react": "^16.5.2", "react-dom": "^16.5.2", }, "devDependencies": { "react-scripts": "1.0.7" }, "scripts": { "start": "react-scripts start", "build": "react-scripts build", "test": "react-scripts test --env=jsdom", "eject": "react-scripts eject" } } { "name": "my-react-tutorial-app", "version": "0.1.0", "private": true, "dependencies": { "@babel/core": "7.1.0", "@svgr/webpack": "2.4.1", "babel-core": "7.0.0-bridge.0", "babel-eslint": "9.0.0", "babel-jest": "23.6.0", "babel-loader": "8.0.4", "babel-plugin-named-asset-import": "^0.2.2", "babel-preset-react-app": "^5.0.2", "bfj": "6.1.1", "case-sensitive-paths-webpack-plugin": "2.1.2", "chalk": "2.4.1", "css-loader": "1.0.0", "dotenv": "6.0.0", "dotenv-expand": "4.2.0", "eslint": "5.6.0", "eslint-config-react-app": "^3.0.3", "eslint-loader": "2.1.1", "eslint-plugin-flowtype": "2.50.1", "eslint-plugin-import": "2.14.0", "eslint-plugin-jsx-a11y": "6.1.1", "eslint-plugin-react": "7.11.1", "file-loader": "2.0.0", "fs-extra": "7.0.0", "html-webpack-plugin": "4.0.0-alpha.2", "identity-obj-proxy": "3.0.0", "jest": "23.6.0", "jest-pnp-resolver": "1.0.1", "jest-resolve": "23.6.0", "mini-css-extract-plugin": "0.4.3", "optimize-css-assets-webpack-plugin": "5.0.1", "pnp-webpack-plugin": "1.1.0", "postcss-flexbugs-fixes": "4.1.0", "postcss-loader": "3.0.0", "postcss-preset-env": "6.0.6", "postcss-safe-parser": "4.0.1", "react": "^16.5.2", "react-app-polyfill": "^0.1.3", "react-dev-utils": "^6.0.3", "react-dom": "^16.5.2", "resolve": "1.8.1", "sass-loader": "7.1.0", "style-loader": "0.23.0", "terser-webpack-plugin": "1.1.0", "url-loader": "1.1.1", "webpack": "4.19.1", "webpack-dev-server": "3.1.9", "webpack-manifest-plugin": "2.0.4", "workbox-webpack-plugin": "3.6.2" }, "scripts": { "start": "node scripts/start.js", "build": "node scripts/build.js", "test": "node scripts/test.js" }, "eslintConfig": { "extends": "react-app" }, "browserslist": [ ">0.2%", "not dead", "not ie <= 11", "not op_mini all" ], "jest": { "collectCoverageFrom": [ "src/**/*.{js,jsx}" ], "resolver": "jest-pnp-resolver", "setupFiles": [ "react-app-polyfill/jsdom" ], "testMatch": [ "<rootDir>/src/**/__tests__/**/*.{js,jsx}", "<rootDir>/src/**/?(*.)(spec|test).{js,jsx}" ], "testEnvironment": "jsdom", "testURL": "http://localhost", "transform": { "^.+\\.(js|jsx)$": "<rootDir>/node_modules/babel-jest", "^.+\\.css$": "<rootDir>/config/jest/cssTransform.js", "^(?!.*\\.(js|jsx|css|json)$)": "<rootDir>/config/jest/fileTransform.js" }, "transformIgnorePatterns": [ "[/\\\\]node_modules[/\\\\].+\\.(js|jsx)$", "^.+\\.module\\.(css|sass|scss)$" ], "moduleNameMapper": { "^react-native$": "react-native-web", "^.+\\.module\\.(css|sass|scss)$": "identity-obj-proxy" }, "moduleFileExtensions": [ "web.js", "js", "json", "web.jsx", "jsx", "node" ] }, "babel": { "presets": [ "react-app" ] } }
2 Comments