How to Use PriorityQueue in Java
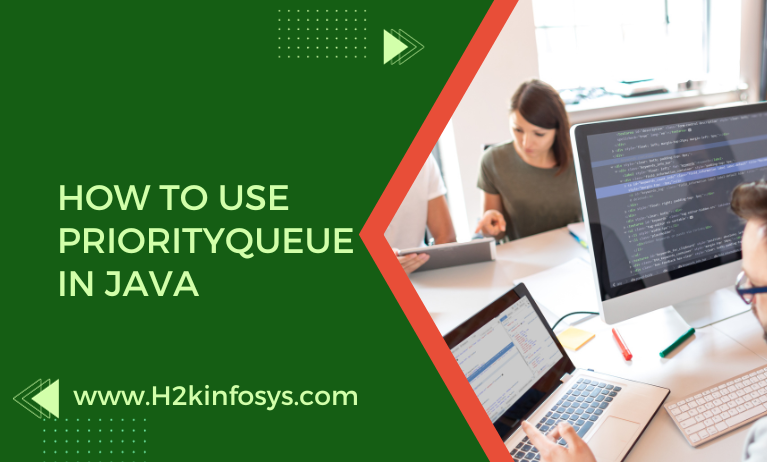
Have you ever had a job at a restaurant where you had to keep food in storage such that the products that were going bad the quickest got used first? If so, do you have experience with a priority queue?
The object known as a “PriorityQueue in java” is designed to organise and rank the items in a queue. Queues typically have a First In, First Out (FIFO) structure, similar to the previous example of the restaurant, but occasionally we may want to rearrange the items in the line to better reflect their priority. To learn more, check out the Java course online.
What does PriorityQueue do in Java?
Similar to an array, a PriorityQueue in Java keeps a group of objects together. It does, however, include methods for ranking which elements are used first. Depending on the situation and your goals, there are a lot of possible uses for this.
Say, for illustration, that you ran a website where customers could purchase goods. As the orders pour in, you observe that you have a sizable order for products that aren’t quite ready for your inventory, followed by much smaller orders for items that you can ship out right away.
If your system is configured to work on a first-in, first-out (FIFO) basis, this would essentially stop filling orders until the large order is ready, resulting in a significant backlog and probably irritating consumers. By simply determining whether the next order to be filled is indeed ready and moving it back in the queue if not, a PriorityQueue might prevent this issue.
It does not even need to be for orders placed by customers. In the event that you were hosting a web service with constrained processing capacity, you could determine which users receive priority depending on factors such as the computational complexity of their requests or if they are subscribers.
Java PriorityQueue in Action
Since this is a back-end concept, it’s challenging to determine with certainty whether a website is utilising this item. Nonetheless, it makes sense to presume that it can be occurring in the background of any website that has to give priority to the services it provides to users.
A common use case for PriorityQueue is in the context of businesses that need to divide bandwidth among customers. An organisation that assigns tasks to employees according to the task’s priority could serve as another illustration.
Even with the aforementioned “catch,” you can use this image to further reinforce your comprehension.
The process of adding an element with priority “45” between elements with priorities “40” and “50” is essentially what you’re seeing.
The element that is processed next is known as the “Front” or “Head” of the queue; elements with the lowest number in the queue are handled first. On the other hand, if the ordering remains the same, the element at the “Back” or “Tail” of the queue would be processed last.
Going back to the bandwidth management example, the queue in the above image can represent a business that does not have enough bandwidth to serve every user at once.
In order to address this problem, they might determine which customers to service first, maybe according to factors like the customer’s access level, location, or any other criterion selected by the bandwidth management firm.
How to Use PriorityQueue in Java
You will learn how to construct a new PriorityQueue object, add things to it.
- Naturally, importing the library containing the PriorityQueue class is the first step in using the PriorityQueue object.
- Declaring a variable to store the PriorityQueue object is the next step. An optional custom comparator (a means of comparing the additional objects) can be passed to the PriorityQueue constructor.
- After the creation of the object, you can start adding more objects. It will default to having a maximum of 11 elements and ranking objects according to their natural order if the constructor is called without any arguments.
- Print out the PriorityQueue.
- Use the peek() method to get the PriorityQueue’s head element.
- Use the poll() method to delete the head element.
- use the java.util library to import the PriorityQueue class
In the event that it is left empty, processing will take natural priority. This indicates that the lowest integer will be placed at the front of the queue when it comes to integers.
The PriorityQueue is a heap-based queue, thus when the entire thing is printed out, the order may appear random or unsorted. Recall that this is not a sorted array that you are working with. When you start looking at the top of the PriorityQueue rather than printing it all out, the priorities will still be determined by natural order.
The head components would actually belong in the following order, notwithstanding the above arrangement, as integers are naturally arranged from least to greatest.
The only difference between this procedure and the previous one is that the head element will be removed afterwards. Furthermore take note that if the queue is empty, this method will return null.
And that’s the fundamental idea! If you want to go one step further, though, it could be prudent to use a custom comparator.
The integers could be arranged in a fashion that goes from least to largest. Although there isn’t much of a difference between this and the natural order for integers, knowing what’s happening here will help you make your own unique comparators.
For the sake of simplicity, you are essentially comparing leftHandSide and rightHandSide, two items at a time. In the event that both sides are equal, zero is returned, preventing the items from being switched.
There won’t be any elements switched even if the left-hand side is smaller than the right-hand side.
In other words, the items would still be in the same sequence if you were to compare 10 and 20. Once more, numbers are arranged naturally from least to greatest.
The last feasible criterion is that leftHandSide must be greater than rightHandSide. In this instance, you are effectively requesting the PriorityQueue to switch these two elements by returning “1”.
The elements would still be in the order [10, 20] if leftHandSide was 20 and rightHandSide was 10. This is because the two elements would be switched.
The conditions used to decide whether or not to swap the elements can be as complex as you would like them to be. This example was a straightforward one, utilising integers. The PriorityQueue would be arranged from least to greatest to greatest to least if the -1 and +1 from the code above were switched.
Conclusion
Allow a Java PriorityQueue to handle the hard work if you want to quickly prioritise data and group them like objects. Start with coding a simple function for practice. Build PriorityQueue into the framework of your web applications from there. Check out the Java free online course to learn more.