JAVA Tutorials
Introduction to Collections
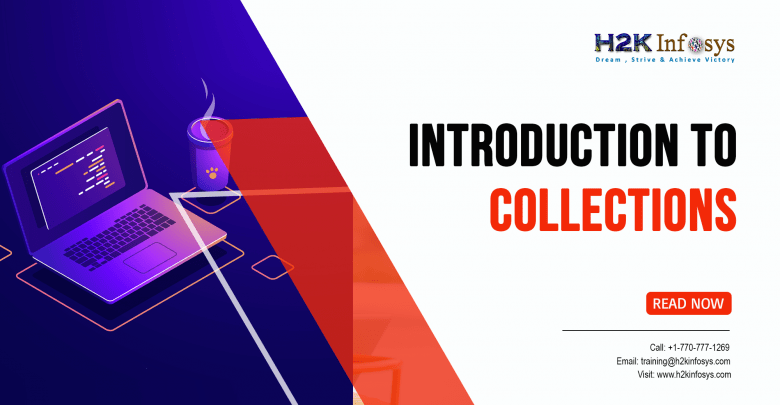
- A Collection is a classification of individual objects which represents as a single unit.
- As we say, Java also provides Collection Framework, which will define the several classes and interfaces to describes a group of the object as a single unit.
- There is two main root Collection interface of java classes are: Collection interface (java.util.Collection) and the Map interface (java.util.Map).
- Collections in java are also provided an architecture to store and manipulate the group of objects.
- It can also achieve all the operations that you perform on the data like searching, sorting, insertion, manipulation, and deletion.
It provides many interfaces (Set, List, Queue, Deque) and classes (ArrayList, Vector, LinkedList, PriorityQueue, HashSet, LinkedHashSet, TreeSet).
“java.util” package, which contains all the classes and interfaces for the Collection framework.
Program: 1 for Collection:
import java.io.*; import java.util.*; class TestCollection { public static void main (String[] args) { int arr[] = new int[] {1, 2, 3}; Vector<Integer> v = new Vector(); Hashtable<Integer, String> h = new Hashtable(); v.addElement(1); v.addElement(2); h.put(1,"colllections1"); h.put(2,"colllections2"); System.out.println(arr[0]); System.out.println(v.elementAt(0)); System.out.println(h.get(1)); } } Output: 1 1 colllections2
Program: 2 for Collection using ArrayList:
import java.util.*; class JavaArrayList{ public static void main(String args[]){ ArrayList<String> alist=new ArrayList<String>(); alist.add("Steve"); alist.add("Tim"); alist.add("Lucy"); alist.add("Pat"); alist.add("Angela"); alist.add("Tom"); //displaying elements System.out.println(alist); //Adding "Steve" at the fourth position alist.add(3, "Steve"); //displaying elements System.out.println(alist); } } Output: [Steve, Tim, Lucy, Pat, Angela, Tom] [Steve, Tim, Lucy, Steve, Pat, Angela, Tom]
Program: 3 for Collection using ArrayList to Remove Elements:
import java.util.*; class JavaRemoveArrayList{ public static void main(String args[]){ ArrayList<String> ralist=new ArrayList<String>(); ralist.add("Steve"); ralist.add("Tim"); ralist.add("Lucy"); ralist.add("Pat"); ralist.add("Angela"); ralist.add("Tom"); System.out.println(ralist); //Removing "Steve" and "Angela" ralist.remove("Steve"); ralist.remove("Angela"); //displaying elements System.out.println(ralist); //Removing 3rd element ralist.remove(2); //displaying elements System.out.println(ralist); } } Output: [Steve, Tim, Lucy, Pat, Angela, Tom] [Tim, Lucy, Pat, Tom] [Tim, Lucy, Tom]
Program: 4 for Collection using Queue
public class QueueDemo { public static void main(String[] args) { Queue<String> que = new LinkedList<>(); que .add("one"); que .add("two"); que .add("three"); que .add("four"); System.out.println(que ); que .remove("three"); System.out.println(que ); System.out.println("Queue Size: " + que .size()); System.out.println("Queue Contains element 'two' or not? : " + que .contains("two")); // To empty the queue que .clear(); } } Output:- [one, two, three, four] [one, two, four] Queue Size: 3 Queue Contains element 'two' or not? : true
Advantages of Collection Framework:
- Consistent API: The API has a generic set of interfaces such as Collection, Set, List, or Map. All classes (ArrayList, LinkedList, Vector, etc.) which implement these interfaces have some standard set of methods.
- Reduces programming effort: Programmer no need to worry about the design of Collection, programmer should focus on its best use in his program.
- Increases the program speed and quality: Increases performance by providing high- performance implementations of useful data structures and algorithms.
- The collections framework is designed to meet all the several goals, such as –
- The framework should be high-performance.
- The implementations done for the fundamental collections (dynamic arrays, linked lists, trees, and hashtables) were to be highly structured.
- The framework is to allow different types of groups to work in a similar manner and with a high degree of interoperability.
- The framework had to extend and adapt a collection easily.
- The collections framework is an architecture to represents and manipulate the collections.
- Here are all the collections frameworks which contain the following: −
- Implementations like Classes − These are the concrete implementations of the collection interfaces. In essence, they are reusable data structures. ·
- Interfaces − It allows collections to be manipulated independently of the details of their representation.
- Algorithms − It is mostly useful for computations, like searching and sorting, on objects that implement collection interfaces.
In addition to collections, the framework defines several map interfaces and classes. Maps store key/value pairs. Although maps are not collections in the proper use of the term, they are fully integrated with collections.
Facebook Comments
One Comment