Introduction to Queue Interface In Java
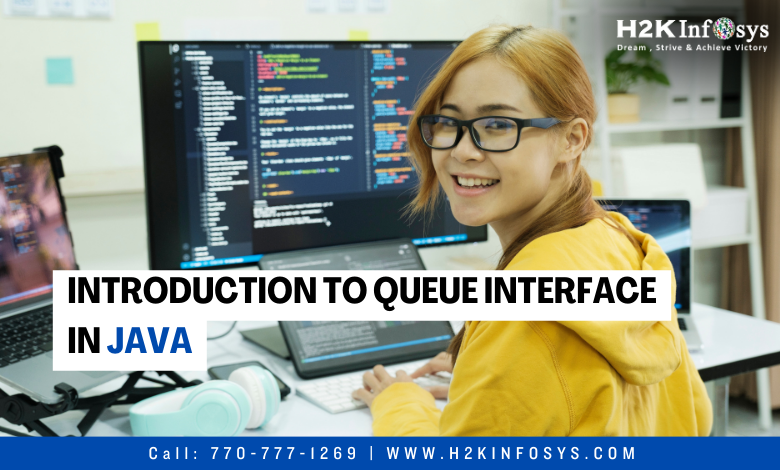
The java.util package contains the Queue interface, which extends the Collection interface and is used to store elements that are going to be processed in First In First Out (FIFO) order. It adheres to the First-In-First-Out, or FIFO, concept and is an ordered list of objects whose use is restricted to adding entries at the end and removing elements from the beginning.
Since the queue is an interface, its declaration requires a concrete class; in Java, the most popular classes are LinkedList and PriorityQueue. Be aware that there is no thread safety in either of these implementations. If a thread-safe solution is required, one possibility is PriorityBlockingQueue. Check out the Java training online to learn more.
Creating Queue Objects
It is not possible to create objects of the type queue since Queue is an interface. To build an object, we always need a class that extends this list. Additionally, you can limit the kind of object that can be kept in the Queue because Java 1.5 introduced generics. This type-safe queue can be defined as:
// Obj is the type of the object to be stored in Queue
Queue<Obj> queue = new PriorityQueue<Obj> ();
A collection of elements in a particular order is represented by the Java Queue interface, which is a subtype of the Collection interface. The elements are retrieved in the order that they were put to the queue in accordance with the first-in, first-out (FIFO) principle.
There are various ways to add, remove, and inspect queue elements using the Queue interface. The following are a few of the most popular techniques:
- add(element): Adds an element to the back of the queue using the add(element) function. It raises an exception if the queue is overflowing.
- offer(element): Moves an element to the end of the waiting list. It returns false if the queue is full.
- remove(): returns the element at the head of the queue once it has been removed. It throws an exception if the queue is empty.
- poll(): Takes out and puts back the item at the head of the queue. It yields null if the queue is empty.
- element(): Without removing the element from the queue, it returns the element at the front. It throws an exception if the queue is empty.
- peek(): Returns, without deleting, the element at the head of the queue. It yields null if the queue is empty.
Several Java classes, such as LinkedList, ArrayDeque, and PriorityQueue, implement the Queue interface. These classes offer many queue interface implementations, each with unique functionality and performance attributes.
In general, the Queue interface is a helpful instrument for managing collections of elements in a specific order, and is widely used in many different applications and industries.
Operations on Queue Interface
Let’s look at how to use the Priority Queue class to execute a few often used operations on the queue.
- Adding Elements: The add() method can be used to add an element to a queue. The PriorityQueue does not maintain the insertion order. The priority order, which is ascending by default, determines how the elements are stored.
- Removing Elements: We may use the remove() method to take an element out of a queue. The first instance of the object is eliminated if there are several of these. In addition, the poll() technique is utilised to extract the head and give it back.
- Iterating the Queue: There are various methods for looping through the Queue. The most well-known method is converting the queue to the array and traversing using the for loop. However, the queue also has an inbuilt iterator which can be used to iterate through the queue.
Characteristics of a Queue
The characteristics of the queue are as follows:
- Using the Queue, items can be added to the end of the queue and removed from the beginning. It adheres to FIFO theory.
- All Collection interface methods, such as insertion, deletion, and so on, are supported by the Java Queue.
- The most popular implementations are PriorityQueue, LinkedList, and ArrayBlockingQueue.
- NullPointerException is raised if BlockingQueues are subjected to any null operations.
- Unbounded Queues are those that are included in the java.util package.
- The Bounded Queues are the Queues that are included in the java.util.concurrent package.
- With the exception of the Deques, all queues allow additions and deletions at the head and tail of the queue, respectively. Both ends of element insertion and removal are supported by the Deques.
Classes that implement the Queue Interface:
- PriorityQueue: The collection framework’s PriorityQueue class gives us a mechanism to handle objects according to their priority. While it is common knowledge that a queue operates on the First-In-First-Out method, there are situations in which processing queue elements based on priority is required. In these situations, a PriorityQueue can be useful.
- LinkedList: The collection framework’s LinkedList class is an implementation of the linked list data structure by default. It is a linear data structure in which each element is a distinct object having a data portion and an address part, and the elements are not kept in adjacent locations. Pointers and addresses are used to connect the elements. Every component is called a node. Because of the ease and dynamicity of insertions and deletions, they are preferred over the arrays or queues.
- PriorityBlockingQueue: Note that neither the LinkedList nor the PriorityQueue implementations are thread-safe. If a thread-safe solution is required, one possibility is PriorityBlockingQueue. PriorityBlockingQueue is an unbounded blocking queue that provides blocking retrieval operations and follows the same ordering guidelines as class PriorityQueue.
Due to its unbounded nature, adding elements may occasionally fail and result in an OutOfMemoryError when resources run out.
Advantages of using the Queue interface in Java
- Order preservation: With the help of the queue interface, elements can be stored and retrieved according to the first-in, first-out (FIFO) principle.
- Flexibility: The Queue interface can be used with a wide range of data structures and algorithms, contingent on the needs of the application, as it is a subtype of the Collection interface.
- Thread-safety: Some Queue interface implementations, like the java.util.concurrent.ConcurrentLinkedQueue class, are capable of handling several threads accessing them at once without generating a conflict.
- Performance: The Queue interface is a helpful tool for managing collections of components in applications where performance is crucial since it offers effective implementations for adding, removing, and examining elements.
Disadvantages of using the Queue interface in Java
- Limited functionality: The Queue interface might not be appropriate for more complicated data structures or algorithms since it is made particularly for organising groups of elements in a particular sequence.
- Size restrictions: specific Queue interface implementations, like the ArrayDeque class, are limited in size, meaning they can’t expand to contain more elements than a specific amount.
- Memory usage: The Queue interface may use more memory than other data structures depending on how it is implemented, particularly if it needs to keep extra data regarding the elements’ order.
- Complexity: For inexperienced programmers, the Queue interface may be challenging to use and comprehend, particularly if they are unfamiliar with the fundamentals of data structures and algorithms.
Conclusion To learn more about Queue Interface in Java, check out our Java course online.