LinkedHashMap in Java
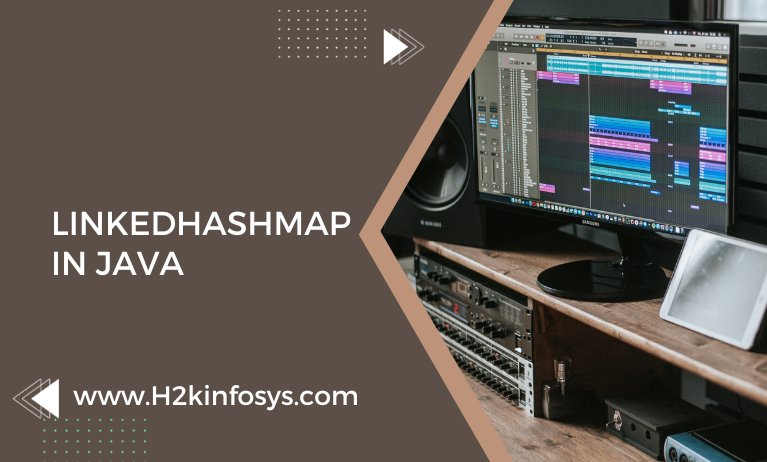
The LinkedHashMap java Class is identical to HashMap, except it also has the ability to keep track of the order in which elements are added. HashMap has the benefit of speedy insertion, search, and deletion, but it never keeps track of the order of insertion as LinkedHashMap does, allowing access to the elements in the order they were added. Check out our Java training online to learn more.
Here is a list of LinkedHashMap’s Java key characteristics:
- Based on the key, a LinkedHashMap includes values. It extends the HashMap class and implements the Map interface.
- There are just distinct components in it.
- Multiple null values and one null key are both possible.
- It lacks synchronisation.
- With the bonus of maintaining insertion order, it is identical to HashMap. For instance, when we execute the code using a HashMap, we get a different order of elements.
Declaration:
public class LinkedHashMap<K,V> extends HashMap<K,V> implements Map<K,V>
Here, K is the key Object type and V is the value Object type
K: The type of the keys in the map.
V:The type of values mapped in the map.
It implements Map<K, V> interface, and extends HashMap<K, V> class.
How LinkedHashMap Works Internally?
A LinkedHashMap implements the Map interface and is a HashMap class extension. Consequently, the class is described as:
- public class LinkedHashMap
Extends HashMap
- implements Map
Nodes are used to represent the data in this class. A doubly-linked list and the LinkedHashMap implementation are extremely similar. As a result, the LinkedHashMap’s nodes are individually represented as:
- Hash: To make searching and inserting faster, all input keys are changed into a hash, which is a condensed version of the key.
- Key: Data is saved as a key-value pair because this class extends HashMap. This parameter is hence the key to the data.
- Value: There is a value connected to every key. The value of the keys is kept in this parameter. Generics allow this value to take on any shape.
- Next: The LinkedHashMap contains the insertion order because it stores it. This contains the address to the next node of the LinkedHashMap.
- Previous: The address of the LinkedHashMap’s previous node is contained in this parameter.
Synchronised LinkedHashMap
The LinkedHashMap implementation is not synchronised. A linked hash map must be externally synchronised if multiple threads access it concurrently and at least one of them affects the map’s structure. Usually, this is done by synchronising on an object that inherently contains the map. The map should be “wrapped” using the Collections.synchronizedMap method if such an object is not present. To avoid unintentional unsynchronized access to the map, it is recommended to perform this at the time of creation:
Map m = Collections.synchronizedMap(new LinkedHashMap(…));
Constructors of LinkedHashMap Class
An object of the LinkedHashMap class must be created in order to generate a LinkedHashMap. The LinkedHashMap class has a variety of constructors that make the building of the ArrayList possible. The constructors available in this class are as follows:
- LinkedHashMap(): This is used to create a default LinkedHashMap constructor.
LinkedHashMap<K, V> lhm = new LinkedHashMap<K, V>();
- LinkedHashMap(int capacity): It is used to provide a specific LinkedHashMap with a predetermined capacity.
LinkedHashMap<K, V> lhm = new LinkedHashMap<K, V>(int capacity);
- LinkedHashMap(Map<? extends K,? extends V> map): It is used to load the contents of the provided map into a specific LinkedHashMap.
LinkedHashMap<K, V> lhm = new LinkedHashMap<K, V>(Map<? extends K,? extends V> map);
- LinkedHashMap(int capacity, float fillRatio): It is used to initialise a LinkedHashMap’s capacity and fill ratio. When to automatically expand the size of the LinkedHashMap is determined by a statistic known as the fillRatio, also known as the loadFactor. By default, this value is set to 0.75, meaning that when the map is 75% full, its size is raised.
LinkedHashMap<K, V> lhm = new LinkedHashMap<K, V>(int capacity, float fillRatio);
- LinkedHashMap(int capacity, float fillRatio, boolean Order): Additionally, a LinkedHashMap’s capacity and fill ratio, as well as whether or not to follow the insertion order, are initialised in this constructor.
LinkedHashMap<K, V> lhm = new LinkedHashMap<K, V>(int capacity, float fillRatio, boolean Order);
Here, true is passed for the last access order and false is passed for the insertion order for the Order attribute.
Various operations on the LinkedHashMap class
Let’s look at a few commonly performed operations on the instance of the LinkedHashMap class.
Operation 1: Adding Elements
The put() method can be used to add an element to the LinkedHashMap. HashMap differs from this in that the insertion order is preserved in the LinkedHashMap whereas it is not preserved in HashMap.
Operation 2: Updating or Changing Elements
If we want to update an element after adding it, we can do so by adding it once more with the put() method. Since the keys in the LinkedHashMap are used to index the elements, changing the value of a key only requires re-inserting the updated value for the key that needs to be modified.
Operation 3: Removing Element
The remove() method can be used to delete an element from the LinkedHashMap. The key value is entered into this method, which then checks to see if the key already exists and, if it does, removes the key mapping from the LinkedHashMap. In addition, if the maximum size is specified, we can also delete the first entered element from the map.
Operation 4: Iterating across the LinkedHashMap
The LinkedHashMap can be iterated over in numerous ways. The most well-known method is running a for-each loop over the selected map view (fetched using a map).the instance method entrySet(). The values of key and value can then be retrieved for each entry (set element) using the getKey() and getValue() methods.
Conclusion
To learn more about LinkedHashMaps in Java, check out the free Java course.