Object Oriented Programming Concepts
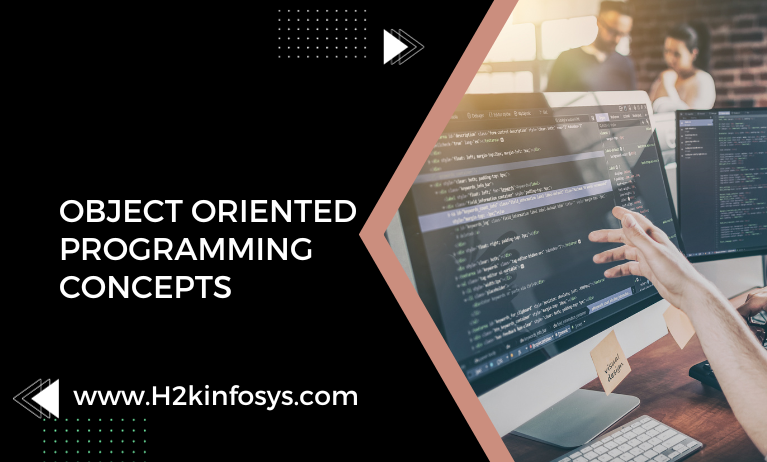
Introduction to OOPS?
The full form of OOPs is “Object-Oriented Programming Concepts.” It is a programming paradigm that works based on abstraction, encapsulation, inheritance, and polymorphism. OOP allows users to create objects and define methods to manipulate and interact with those objects. The fundamental concept of Object-Oriented Programming is to create and model real-world objects in a program, reuse them throughout the program, and manipulate these objects to achieve desired results.

The OOPS concepts are:
1. Class:
- A class is a blueprint for creating objects.
- It represents a group of similar entities.
- It defines the properties (attributes) and behaviors (methods) that objects of that class will have.
- For example, the class “Animals” can include objects like ‘Dog,’ ‘Cat,’ and ‘Snake’ with properties such as color and breed and methods like age, name, and food.
2. Object:
- An object is an instance of a class.
- It encapsulates both data and methods to operate on that data.
- Multiple instances of a class can exist in a program.
- For example, if the class is “Fruit,” then objects could be ‘Apple,’ ‘Banana,’ ‘Kiwi,’ and ‘Strawberry.’
3. Inheritance:
- Inheritance allows one class to inherit properties and behaviors from another class.
- It establishes a parent-child relationship between classes.
- It promotes code reusability and structure.
4. Polymorphism:
- Polymorphism enables objects to perform the same action in different ways.
- It can be achieved through Method Overloading and Method Overriding.
- It allows a variable, object, or function to take on multiple forms.
5. Abstraction:
- Abstraction focuses on representing essential features without exposing underlying details.
- It simplifies the complex reality by creating new data types for specific applications.
- It’s like driving a car without needing to understand its internal workings.
6. Encapsulation:
- Encapsulation involves wrapping data and code into a single unit.
- It hides class variables from external access, ensuring data integrity.
- Access to data is allowed only through class methods.
7. Association:
- Association represents connections between two objects.
- It defines relationships and interactions between objects.
- For example, students can be associated with teachers for different subjects.
8. Aggregation:
- Aggregation is a relationship where objects have separate lifecycles, and there is ownership.
- Child objects do not belong to another parent object.
- For example, a teacher can belong to a department, but the teacher’s existence is independent of the department’s.
9. Composition:
- Composition is a more specific form of aggregation.
- Child objects have no independent lifecycle; they depend on the parent object.
- If the parent object is deleted, child objects are also deleted.
Pros of OOP:
- OOP offers a modular structure, making programs easier to understand.
- Code and objects can be reused, reducing development costs.
- It enhances program modularity, as objects exist independently.
- Design and development teams can create more robust software with fewer flaws.
Comparison with Other Programming Styles:
- OOP stands apart from unstructured and structured programming.
- Unstructured programming relies on repetition and has a sequential flow.
- Structured programming uses functions for reusability and non-sequential flow.
- OOP combines data and action into objects for improved organization and code reusability.
Example:
- Imagine creating a banking software.
- In unstructured programming, you’d use variables for account numbers and balances.
- For deposits and withdrawals, code would be repeated, making it less modular.
- In OOP, you’d create classes for accounts, with methods for depositing, withdrawing, and checking balances, promoting code reuse and modularity.
In conclusion, Object-Oriented Programming offers a structured and efficient way to design and develop software, making it easier to manage and maintain complex systems.
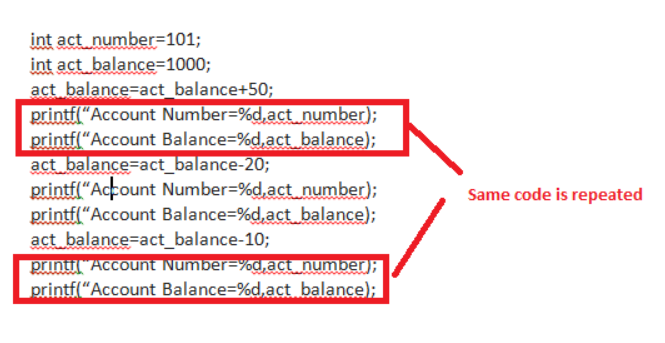
Structured Programming
In structured programming, the code is organized into functions or methods. Repeated lines of code are encapsulated within these functions, which are called when needed. This approach simplifies code maintenance and promotes reusability through modularization.
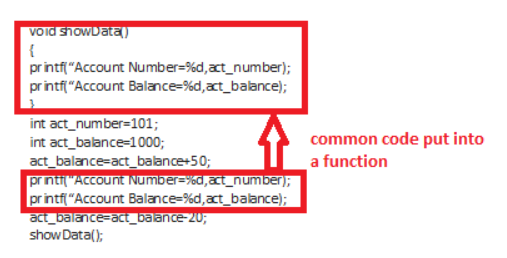
Object-Oriented Programming (OOP) Concepts: OOP is an approach that combines data and operations into objects, providing a more organized and efficient way to manage and manipulate data. It introduces key concepts like classes and objects for encapsulating data and behavior into reusable units.
Code Summary: Below is a code snippet that demonstrates the basic structure of an OOP class in the context of an “Account” class:
Object-Oriented Programming
OOP is an approach that combines data and operations into objects, providing a more organized and efficient way to manage and manipulate data. It introduces key concepts like classes and objects for encapsulating data and behavior into reusable units.
Class Account{
int act_number;
int act_balance;
public void showdata(){
system.out.println(“Account Number”+act_number)
system.outprintln(“Account Balance”+ act_balance)
}
}
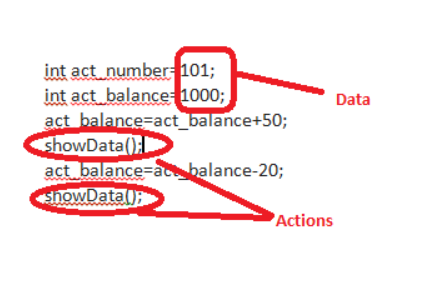