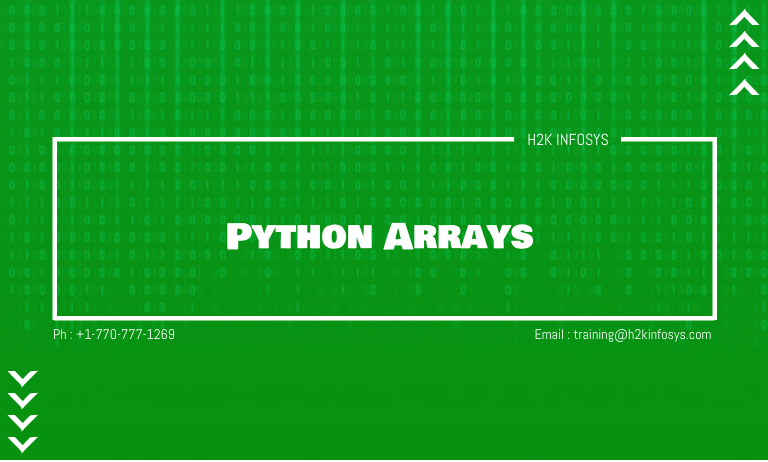
An array in Python is simply a collection of data in a particular data type. Lists in some sense can be seen as arrays, only that the elements of a list can contain more than one data type. Arrays do not support multiple data types. In Python, arrays are created using the array module which must be specifically imported.
Arrays are used for mathematical computations so their elements must be a numerical data type. In this tutorial, we will discuss how to create an array in Python and the various operations that can be done on an array. In specific terms, here’s what you will learn by the end of this tutorial.
- Are Arrays Better than List?
- Defining an Array in Python
- Accessing the Elements of an Array.
- Inserting an Element in an Array
- How to Change the Element of an Array.
- How to Join Arrays Together
- How to remove an element from an Array with the pop() method.
- Using the remove elements of an Array with the remove() method in Python
- How to Reverse the elements of an array in Python
- How to Reverse the elements of an array in Python
Let’s blast off.
Are Arrays Better than List?
We have mentioned that a list can be seen as an array only that the elements of a list are not constrained to a specific numeric datatype. In simple terms, arrays are much better than lists for numerical computations. They can do more complex mathematical operations in a faster time. Furthermore, arrays typically consume less system memory than lists.
Syntax
According to its official documentation. Here is the Docstring.
Docstring: array(typecode [, initializer]) -> array Return a new array whose items are restricted by type code, and initialized from the optional initializer value, which must be a list, string or iterable over elements of the appropriate type.
Parameters:
- Type code: The type code is the datatype of the arrays that would be defined.
- Array: This receives the list of items you wish to store as an array, following the type code you defined.
There are various single character representations for different type codes. These type codes will be shown below.
Type code | Minimum size (in bytes) | C Type |
‘b’ | 1 | Signed integer |
‘B’ | 1 | Unsigned integer |
‘u’ | Unicode character (Refer to the note) | |
‘h’ | 2 | Signed integer |
‘H’ | 2 | Unsigned integer |
‘i’ | 2 | Signed integer |
‘I’ | 2 | Unsigned integer |
‘l’ | 4 | Signed integer |
‘L’ | 4 | Unsigned integer |
‘q’ | 8 | Signed integer (Refer to note) |
‘Q’ | 8 | Unsigned integer (Refer to note) |
‘f’ | 4 | Floating point |
‘d’ | 8 | Floating point |
Note:
- The ‘u’ type code means the Unicode character in Python. It is 2 bytes on the narrow build and 4 bytes on wide builds
- Both the ‘q’ and ‘Q’ type codes are available if the C compiler upon which the Python code was written supports __int64 on Windows or long long.
If you don’t understand what a signed and unsigned data type is, an unsigned data type does not accept negative numbers. In other words, only numbers greater than 0 are permitted. A signed data type in contrast accepts negative numbers. However, the range of positive numbers it can take is reduced to accomodate the negative numbers.
Defining an Array in Python
An example of an array is shown below.
#import the necessary libraries import array #define an array array1 = array.array('i', [2, 3, 54, 4, 6, 12, -2]) print(array1)
Output:
array('i', [2, 3, 54, 4, 6, 12, -2])
Note that if the type code was changed to ‘I’, which is an unsigned data type, the array would not be successfully created because of the -2 item. See the code below.
#import the necessary libraries import array #define an array array1 = array.array('I', [2, 3, 54, 4, 6, 12, -2]) print(array1)
Output:
Traceback (most recent call last):
File "c:/Users/DELL/Desktop/pycodes/__pycache__/strings.py", line 5, in <module>
array1 = array.array('I', [2, 3, 54, 4, 6, 12, -2])
OverflowError: can't convert negative value to unsigned int
As seen, it throws an error.
Accessing the Elements of an Array through Slicing and Indexing.
Just like in lists, the element of an array can be accessed through indexing. Its syntax is as follows, array_variable[index].
Remember that the indexing in Python begins from 0. If for example, we wish to access the first element of an array, we pass index 0.
#import the necessary libraries import array #define an array array1 = array.array('i', [2, 3, 54, 4, 6, 12, -2]) print(array1[0])
Output:
2
To access a list of elements, the slicing operation is done. Again, just like in list slicing, slicing an array follows the syntax array_name[start_index:end_index]. If we wish to access the second to 6th elements in the array, we can write:
print(array1[1:5])
Output: array('i', [3, 54, 4, 6])
Inserting an Element in an Array
You can add one or more elements to an array using the insert() method. The insertion can be done at any point in the array, whether the beginning, middle, or end. The insert method follows the syntax below.
arrayName.insert(index, value)
Parameters:
- Index: This is the position where the new element will be added
- Value: The is the value of the elements added
Example:
Let’s say we wish to add a number to the beginning of an array. The index parameter would be defined as 0. The code below shows how to add 1000 to the beginning of an already defined array.
#import the necessary libraries import array #define an array array1 = array.array('i', [2, 3, 54, 4, 6, 12, -2]) #add an element to the array array1.insert(0, 1000) print(array1)
Output:
array('i', [1000, 2, 3, 54, 4, 6, 12, -2])
As seen, 1000 is now the first element in the array.
How to Change the Element of an Array.
Arrays are mutable which means you can change the elements in the array dynamically. If you wish to change the elements of an array, you simply access the element through indexing and reassign the value to a different number. See the example below.
#import the necessary libraries import array #define an array array1 = array.array('i', [2, 3, 54, 4, 6, 12, -2]) #change the first element from 2 to -10 array1[0] = -10 print(array1)
Output:
array('i', [-10, 3, 54, 4, 6, 12, -2])
As seen, the first element has been changed from 2 to -10
How to Join Arrays Together
Arrays can be concatenated using the ‘+’ sign. This operation adds the beginning of the first array to the end of the first array to return one longer array. See the example below.
#import the necessary libraries import array #define an array array1 = array.array('i', [1, 2, 3]) array2 = array.array('i', [4, 5, 6]) #join both arrays print(array1 + array2)
Output:
array('i', [1, 2, 3, 4, 5, 6])
How to remove an element from an Array with the pop() method.
The pop method removes an element from an array. The pop method receives one argument, the index of the element to be removed. By default, the index is set to -1 which means that running the pop method without defining an argument removes the last element from the array.
Example:
#import the necessary libraries import array #define an array array1 = array.array('i', [1, 2, 3]) #remove the last element array1.pop() print(array1)
Output:
array('i', [1, 2])
Using the remove elements of an Array with the remove() method in Python
While the pop method removes elements based on its index, the remove method removes elements based on their value. The remove method expects one argument, the value you wish to remove. It then removes the first occurrence of the value and rearranges the array to replace its index.
Example:
If we wish to remove the first number ‘11’ from an array defined. We can write the shown as shown below.
#import the necessary libraries import array #define an array array1 = array.array('i', [11, 20, 9, 11]) #remove the first number 11 array1.remove(11) print(array1)
Output:
array('i', [20, 9, 11])
How to Reverse the elements of an array in Python
The reverse method is used to reverse the arrangements of elements of an array from back to front. See an example below.
#import the necessary libraries import array #define an array array1 = array.array('i', [11, 20, 9, 81]) #remove the last element array1.reverse() print(array1)
Output:
array('i', [81, 9, 20, 11])
How to count the number of Occurrences of an Element
The count method is used to count the number of times an element appears in an array. It receives one argument; the element that needs to be counted.
Example:
#import the necessary libraries import array #define an array array1 = array.array('i', [11, 20, 11, 9, 81, 11]) #count the number of times 11 appears print(array1.count(11))
Output:
3
Of course, 11 appears 3 times in the array.
Wrapping up,
In this tutorial, you have learned how to create arrays in Python from the array module. You also discovered how to perform operations on an array. Operations such as accessing element(s) in an array, adding elements to an array, removing elements from an array, counting the number of occurrences of elements, etc. If you have any questions whatsoever, feel free to leave them in the comment section and I’d do my best to answer them.