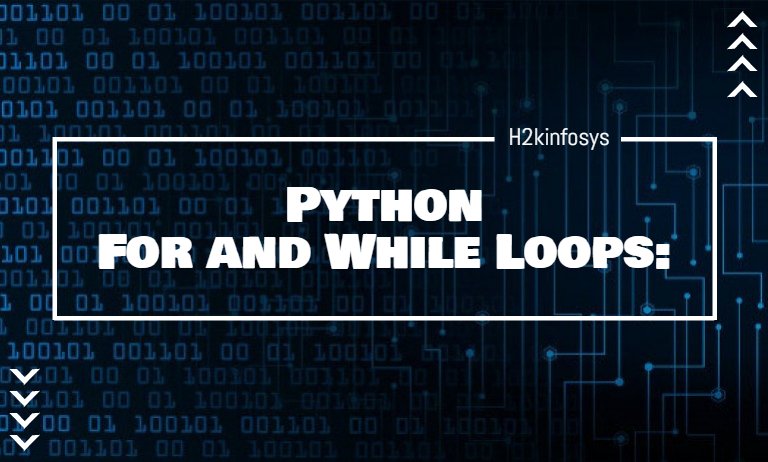
If you need to print “Hello World” for 5 times or repeat a specific snippet of code for 5 times then one way is to write that print statement or code snippet 5 times.
There is a famous quote in programmers.
“If you have to write the same code twice then you are doing it wrong”
Loops are the solution to the above problem.
There are majorly two types of loop
- For loop
- While loop
For Loop
For loop is used when you exactly know how many times you have to print a statement or how many time you want to repeat a specific code snippet.
The syntax of for loop is as follows.
for variable_name in range(5): print("Hello World")
The range() function returns an object like a list of number to specific input given. The output of the above code will be
Let’s take another example in which we will use the variable_name too. In this example, we will generate a table of 2.
for i in range(11): print("2 x ",i, " = ", i*2)
The following will be the output.
For loop over List
For loops can be used to iterate over lists too. If you have a list of fruits and you want to get them one by one then for loop will be used.
Let’s take an example.
fruits = ['apple', 'banana', 'mango', 'orange'] for fruit in fruits: print(fruit)
The following will be the output.
Break statement in For loop
The break statement is used to stop the loop. For example, you are searching for “mango” in the list and you want to stop print the fruit names if “mango” comes. Let’s do this by writing a program.
fruits = ['apple', 'banana', 'mango', 'orange'] for fruit in fruits: if fruit == "mango": break print(fruit)
Continue statement in For loop
In the above example, we stopped the loop when the “mango” was found on the list. What if you just want to skip the “mango”? For skipping the “continue” statement is used. Let’s apply “continue” in the above example.
fruits = ['apple', 'banana', 'mango', 'orange'] for fruit in fruits: if fruit == "mango": continue print(fruit)
The following will be the output.
Enumerate in For loop
Enumerate is used to count the number of iterations. For example, you want to see what number “mango” comes in the list. Let’s use enumerate in the above example.
fruits = ['apple', 'banana', 'mango', 'orange'] for fruit in enumerate(fruits): print(fruit)
While loop
“While loop” is used when you don’t know when to stop the loop but you have a conditional statement to stop the loop. Let’s take a simple example, you are adding plus 1 to a variable and you want to stop adding when the number reaches 5. Let’s see how to do this task.
x = 0 while x != 5: print(x) x = x + 1
The above code keeps on incrementing the ‘x’ until it becomes 5 then it stopped incrementing.
One Comment