Python readline() Method: How to read files in Python
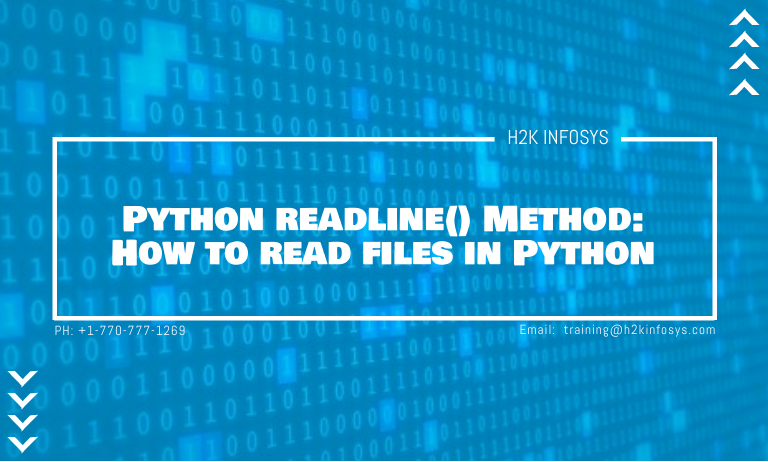
The Python readline() method is an inbuilt method in Python used to read a line of text in Python. While reading a file, the realine() sees that point as the end of a line as \n and prints all preceding characters.
You can also tweak the number of characters to print in a given line using the size parameter of the readline() method. By default, it is set to -1, which means the readline() method prints all the line characters.
This tutorial will discuss how to read files in Python using the readline() method. In specific terms, you will learn the following by the end of this tutorial.
Working with Files in Python
Before reading files in Python, you must first open the file. Thus, it is critical to discuss how to do basic input/output file operations in Python. The open() function is used to open a file in Python. The function takes two parameters, the file path, and the mode.
Syntax
open("filepath", "mode")
The file path is a string of where the file you wish to open is stored on your machine. The mode on the other hand defines the format with which the file should be opened. The mode argument is also a string. Different mode parameters can be specified when opening a file. They are:
- ‘r’ – This allows you to read the characters in the file. The readline() or readlines() method can be called afterward
- ‘w’ – This mode allows you to write to a file. After using this mode to open the file, you can use the write(). Note that this overwrites any content found in the file or creates a new file if it does not exist.
- ‘r+’ – This is used for both reading and writing to a file.
- ‘x’ – This creates a file and opens the file for writing.
- ‘a’ – This is used to append more content at the end of the file. Note that this mode does not overwrite the contents of the file but rather adds to it from the end.
- ‘rb’ – This is used for reading the file as a binary object.
- ‘wb’ – This is used for writing to a file as a binary object.
Now we understand how to open a file, let’s get on to how to read a file using the readline() method.
Using the readline() method
Syntax.
file.readline(size)
Parameters:
size: This is an optional parameter. It defines the number of characters that should be opened. By default, it is set to -1, which means all the characters in the line would be printed.
Returns:
The readline() method returns the characters in the line of a file based on the size defined.
Let’s see an example. We will create a new file called test.txt that contains the following content.
This is the first line
This is the second line
This is the third line
This is the fourth line
This is the fifth line
The test.txt file is saved in the same file directory as my Python interpreter. This way, the file path can simply be passed as test.txt.
Step 1:
The first step is to open the file with the read mode. We will save it as a variable called the_file.
the_file = open('test.txt', 'r')
Step 2:
Call the readline() method on the opened file object and print the result.
print(the_file.readline())
Output:
This is the first line
Step 3:
Close the file. It is a good practice to close a file after performing all the operations intended. Not closing the file can lead to unforeseen errors in the file. So we need to close the file. You close a file in Python using the close() method.
the_file.close()
The entire code is shown below.
the_file = open('test.txt', 'r') print(the_file.readline()) the_file.close()
Output:
This is the first line
Notice that it prints only the first file of the string. The question then is, how do you read multiple lines in a file.
Reading Multiple Lines in a File using the readlines() method
You can read multiple lines in a file using the readlines() method rather than the readline() method. This method returns a list of the lines in the text. See an example below.
the_file = open('test.txt', 'r') print(the_file.readlines()) the_file.close()
Output:
['This is the first line\n', 'This is the second line\n', 'This is the third line\n', 'This is the fourth line\n', 'This is the fifth line']
Alternatively, you can loop over the opened file object to read all the lines in a text. This method does not require you to call the readline or readlines() method. In the example below, we loop over the opened file object with a for loop.
the_file = open('test.txt', 'r') for each_line in the_file: print(each_line) the_file.close()
Output:
This is the first line
This is the second line
This is the third line
This is the fourth line
This is the fifth line
We can append the result to an empty list and print the result to produce the same output as the readlines method. See the example below.
lines_list = [] the_file = open('test.txt', 'r') for each_line in the_file: lines_list.append(each_line) print(lines_list) the_file.close()
Output:
['This is the first line\n', 'This is the second line\n', 'This is the third line\n', 'This is the fourth line\n', 'This is the fifth line']
Reading Files in Python using Context Managers.
Using context managers is another way of opening and working with files in Python. One of the key advantages of using context managers is that you do not need to close the file at the end of the code.
The with statement is used to indicate the use of a context manager. So rather than typing
the_file = open('test.txt', 'r')
Type,
with open('test.txt', 'r') as the_file:
Both codes mean and do the same thing. But recall that with context managers, you do not need to close the file as it is done automatically. Let’s read the context in a file using a context manager.
lines_list = [] with open('test.txt', 'r') as the_file: for each_line in the_file: lines_list.append(each_line) print(lines_list)
Output:
['This is the first line\n', 'This is the second line\n', 'This is the third line\n', 'This is the fourth line\n', 'This is the fifth line']
Most programmers prefer to use context managers rather than the traditional way because of its compactness and the proper memory allocation. It is therefore recommended to use context managers when dealing with files I/O in Python.
In summary
You have seen how to work with files in Python. Specifically, you learned how to read files using the readline() and readline() methods. Remember that before reading a file, the file must be opened in a read mode. Lastly, you learned a cleaner way of reading files in Python – using context managers. If you have any questions, feel free to leave them in the comment section and I’d do my best to answer them.