Refresh Page in Selenium Webdriver
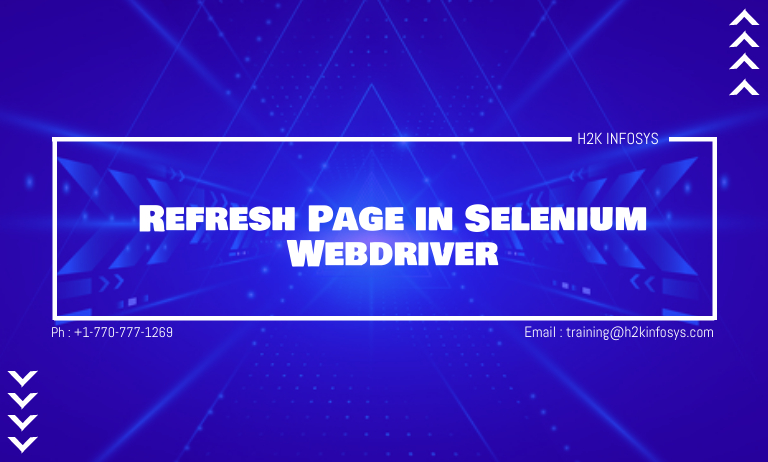
While automating a web-based application, we need to refresh the web page multiple times so that all the web elements are loaded completely. There may be some elements, which do not load during the initial page load. Therefore, we need to refresh the page for all the web elements to be loaded. In Selenium Web Driver, refreshing the web page can be achieved by using refresh command. There are different ways to refresh the web page in Selenium Web Driver:
- Driver.navigate.refresh command
- Send Keys command
- Driver.navigate.to command
1] Driver.navigate.refresh command:
Selenium Web Driver has an inbuilt method for refreshing the page and is used across test automation for performing a page refresh.
Syntax:
driver.get("https://www.google.com/");
driver.navigate().refresh();
Here navigation is an interface used to perform multiple browser operations like navigating to the previous page, navigating to the next page, page refresh, closing the browser. These navigation interface methods are accessed using the command driver.navigate, and it neither takes any arguments nor does it return any values.
Let us take an example:
import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; public class Refresh1 { public static void main(String arg[]) throws InterruptedException { System.setProperty("webdriver.chrome.driver", "E:\\chromedriver.exe"); WebDriver driver = new ChromeDriver(); driver.get("https://www.google.com/"); driver.manage().window().maximize(); driver.navigate().refresh(); driver.close(); } }
Explanation of Code:
- First, we will open the chrome browser with web page https://www.google.com/
- Once the page successfully gets loaded, we will refresh the web page using driver.navigate.refresh method
- At last, we will close the browser using the driver.close() method
When the web page does not load completely, it may result
Or sometimes it shows.
2] Get method:
This Get method is used in a recursive way to refresh a page. For this, we have to pass another method as an argument to access the get method.
Syntax:
driver.get("https://www.google.com");
driver.get(driver.getCurrentURL());
3] Navigate method:
This Navigate method uses the same concept of recursion as used in the get method. getCurrentURL() method is passed as an argument in driver.navigate.to method.
Syntax:
driver.get("https://www.google.com");
driver.navigate.to(driver.getCurrentURL());
4] Send Keys method using F5 Key:
This method takes the refresh key (F5 Key) as an argument to send the keys method. Since send keys works only on web elements and not on the browser, we first need to identify a valid web element on the web page and then use this send keys method.
Syntax:
driver.get("https://www.google.com");
driver. findElement(By.id("username")).sendKeys(Keys.F5);
5] Send Keys method using ASCII Code:
This method also uses the same refresh method concept as mentioned above; the only difference here is that instead of passing the F5 key as an argument, we send the ASCII Code instead of the refresh key as an argument.
Syntax:
driver.get("https://www.google.com");
driver. findElement(By.id("username")).sendKeys(“\uE035”);
Difference between Get Method and Navigate Method:
The main difference between the get method and navigate method is that navigate() allows you to go back and forward in the history of the browser, which is not possible through the get().
Driver.navigate().back() – Used to perform backward function of browser.
Driver.navigate().forward() – Used to perform forward function of browser.
Also, get() waits until the page loads completely before returning control to the script but navigate() does not wait for the page to load completely.