Selenium Form WebElement
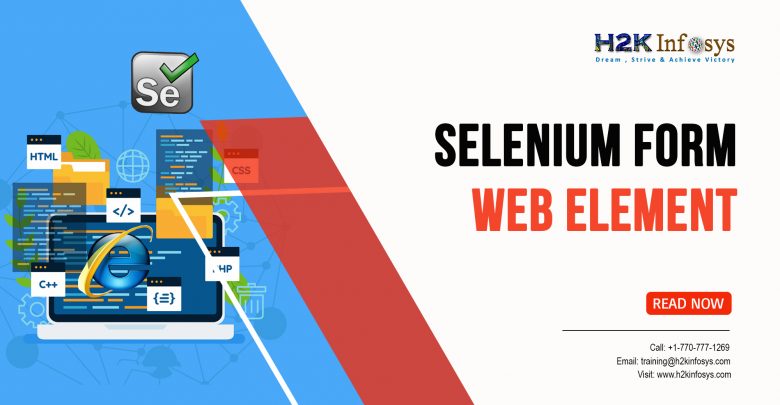
Selenium WebElement Form: TextBox, Submit button, sendkeys(), and Click()
Forms are the web elements to receive basic information from the websites. Web forms have multiple GUI elements like Checkboxes, Textboxes, Radio buttons, Dropdown fields, Input fields, etc.
In this article, we will see how to identify the form elements using Selenium WebDriver. Selenium encapsulates every element in the form as an object. We find the element using provided API and perform actions like entering text into text boxes, clicking the buttons, clicking Radio buttons, etc.
Introduction to WebElement, findElement(), findElements()
Selenium WebDriver encapsulates every element in the form as an object of WebElement.
There are different techniques through which the Selenium WebDriver identifies the WebElements which are based on different properties like Name, ID, XPath, CSS Selector, TagName, etc.
Selenium WebDriver provides two different methods to find the elements on the web page.
- findElement() – This command will return the single WebElement on the web page and returns it as a WebElement object.
- findElements() – This command will return a list of elements on the web page and finds the elements in a particular location using locators.
Let’s see the example of how to get a single element – Using the findElement() method and getting the Text Field WebElement object on a web page.
Step 1: To create objects of Web Elements we need to import the below package
import org.openqa.selenium.WebElement;
Step 2: We need to call the findElement() method and get a WebElement object which is available on the WebDriver class.
InputBox
Input boxes refer to below two types:
- Text Fields: Textboxes are used as input text from the user, and the entered text by the user will be visible as it is typed.
- Password Fields: It input text from the user, but it uses some character to obscure the text as input. The text entered in this field is not visible to the user, it displays in an encrypted format.
Locators
The findElement() method takes locator as parameter to the element. Multiple locators By.name(), By.id(), By.CSSSelector(), By.Xpath(), etc. locate the elements using properties like name, id, Xpath, etc.
We use Inspect Element to the id, name, Xpath, etc. of the elements.
Using the example https://login.yahoo.com/ given below code is the code to locate the “Email address” field using the name locator and the “Password” field using the id locator.
Step 1: Go the site https://login.yahoo.com/
Step 2: Right-click on the Email address field and select Inspect
Step 3: Click on Inspect Icon and select the Email Address field to inspect.
The “Email Address” text field name locator is name=”username”
The “Password” text field id locator is id=”login-passwd”
//WebElement corresponding to the Email Address
WebElement email = driver.findElement(By.name(“username”));
//WebElement corresponding to the Password field
WebElement password = driver.findElement(By.id(“login-passwd”));
Entering Values in Input Boxes
sendKeys() is the method to enter the text into the Text Fields and Password Fields.
Using the example https://login.yahoo.com/ given below code is the code on how to find the Text field and Password fields and enter values into them.
Scenario
- Find the “Email address” field by using the name locator
- Find the “Password” field by using the id locator
- Enter a email id into the “Email address” using the sendKeys() method.
- Enter a password into the “Password” field by using the sendKeys() method.
//WebElement corresponding to the Email Address
WebElement email = driver.findElement(By.name(“username”));
//WebElement corresponding to the Password field
WebElement password = driver.findElement(By.id(“login-passwd”));
email.sendKeys(“[email protected]”);
password.sendKeys(“******”);
Deleting Input Boxes Values
The method clear() is used to delete the given value in an input box. The code will clear the entered text from the Email address and Password fields.
Buttons
The click() method is used to access the buttons.
In the below example
Scenario
- Find the Log In button
- Click on the “Log In” button to get login to the site
WebElement facebooklogin = driver.findElement(By.id(“u_0_b”));
Submit Buttons
Submit buttons are used to submit the complete form to the server. By using either submit() method or click() method on the web element in the form or on the submit button itself.
Complete Program
Below is the complete code for above all scenarios
import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.*; public class FormTesting { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver"," D:\\Drivers\\geckodriver.exe"); WebDriver driver = new ChromeDriver(); String baseUrl = " https://www.facebook.com/ "; driver.get(baseUrl); // Get the corresponding WebElement to the Email Address WebElement email = driver.findElement(By.name(“email”)); // Get the corresponding WebElement to the Password Field WebElement password = driver.findElement(By.id(“pass”)); email.sendKeys("[email protected]"); password.sendKeys("qwerty1234"); System.out.println("Entered Text Field Set"); // Deleting the entered values in the text box email.clear(); password.clear(); System.out.println("Entered Text Field Cleared"); // Find the submit button WebElement login = driver.findElement(By.id("u_0_b ")); //Click method to submit form email.sendKeys("[email protected]"); password.sendKeys("qwerty1234"); login.click(); System.out.println("Login Done with Click Option"); //Using the submit method to submit the form. Submit used on the password field driver.get(baseUrl); driver.findElement(By.name(“email”)).sendKeys("[email protected]"); driver.findElement(By.id(“pass”)).sendKeys("qwerty1234"); driver.findElement(By.id("u_0_b")).submit(); System.out.println("Login Done with Submit Operation"); } }
Conclusion
The below table summarizes the commands to access each type of element
Element | Command | Description |
Input Box | sendKeys() | It is used to enter values into text boxes |
clear() | It is used to clear the value in the text box | |
Submit Button | submit() | It is used to submit the form |
Links | click() | It is used to click on the link to get the page load |
One Comment