Servlet Request and Response objects
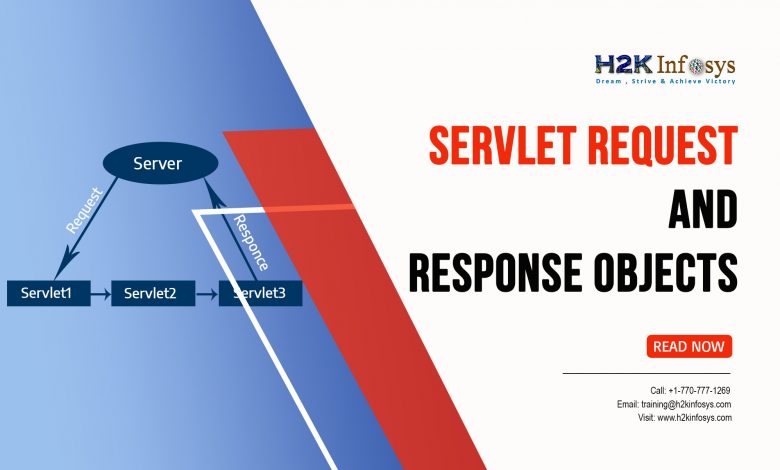
The servlet container of a web service is responsible for the creation of a ServletRequest object. The servlet container wraps all data that came from a client to a web server as a request. Also, the servlet container creates a corresponding ServletResponse object, that will be filled with data in a servlet.
The container passes the ServletRequest and the ServletResponse objects as an argument to the service() method of a corresponding servlet. The ServletRequest object contains header and body information of data that come with request.
Servlet Request object
The ServletRequest object is from package javax.servlet.
Probably, the most used method from the ServletRequest interface is the method to get parameters.
Let’s assume the situation that we have a web page with fields. A user filled some of them and pressed a button to submit changes. This triggers the get request to the server. There is a dispatcher of the servlet that sends a request to the corresponding servlet. All data are wrapped to the ServletRequest. The parameters are contained in the query string or posted form data. To get each parameter that the user filled in the web page we will use methods to get parameters. They are:
java.lang.String getParameter(java.lang.String name) – returns the value of parameter by name. The result is null if the parameter does not exist. This method is used when you are sure the parameter has only one value. If the parameter can have more than one value there is another method for it. In the case of many values, the current method will return just the first value.
java.lang.String[] getParameterValues(java.lang.String) – returns an array of values of a parameter by name. It returns all of the values the given request parameter has. If the parameter does not exist, it will return null.
java.util.Enumeration<java.lang.String> getParameterNames() – returns an Enumeration of String objects containing the names of the parameters contained in this request. It returns an empty Enumeration, if no parameters in the request.
java.util.Map<java.lang.String,java.lang.String[]> getParameterMap() – returns the parameters of this request in the form of key-value pairs( java.util.Map).
Here is simple example:
<web-app> <servlet> <servlet-name>myservlet</servlet-name> <servlet-class>edu.test.myservlets.ServletExample</servlet-class> </servlet> <servlet-mapping> <servlet-name>myservlet</servlet-name> <url-pattern>/test/myservlet</url-pattern> </servlet-mapping> </web-app>
Our servlet:
package edu.test.myservlets; import javax.servlet.http.*; import javax.servlet.*; import java.io.*; public class ServletExample extends HttpServlet{ public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException,IOException { response.setContentType("text/html"); PrintWriter printwriter=response.getWriter(); String name = request.getParameter("username"); printwriter.println("Username: " + name); printwriter.close(); } }
To call our servlet and to pass parameters we can use URL:
http://<host>:<port>/<mywebapp>/test/myservlet?username=TestUser
We will get the output:
Username: TestUser
To get all parameters we can use this method doGet:
public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { PrintWriter output = response.getWriter(); response.setContentType("text/html"); Enumeration en = request.getParameterNames(); while (en.hasMoreElements()) { Object obj = en.nextElement(); String param = (String) obj; String paramvalue = request.getParameter(param); output.print("Parameter Name: " + param + " Parameter Value: " + paramvalue); } output.close(); }
In our case, we have just one parameter, so the output will be the same as in the previous example.
There are methods in the ServletRequest interface to get HTTP Request Headers. The header is used to pass the additional information about the client to the server. There are methods:
java.lang.String getHeader(java.lang.String name) – returns single header value by key.
java.util.Enumeration getHeaderNames() – returns an enumeration of header names.
java.util.Enumeration getHeaders(java.lang.String name) – returns all the values for a specific header.
As header information can be:
- acceptable media types in response;
- acceptable character sets in response;
- an acceptable set of languages
- authorization, when client is attempting to authenticate itself with a server
- host, that indicates the internet host and port number of the resource being requested
- User-Agent, that contains the information about the client originating the request
Here is the example of getting information about servlet headers:
package edu.test.myservlets; import java.io.IOException; import java.io.PrintWriter; import java.util.Enumeration; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; public class HeaderDetailsServlet extends HttpServlet { public void doGet(HttpServletRequest request, HttpServletResponse response) throws IOException, ServletException { response.setContentType("text/html"); PrintWriter printWriter = response.getWriter(); printWriter.println("Here is the Header Information:"); Enumeration en = request.getHeaderNames(); while (en.hasMoreElements()) { String headerName = (String) en.nextElement(); String headerValue = request.getHeader(headerName); printWriter.println(headerName + " " + headerValue); } } }
There is our web.xml:
<web-app> <servlet> <servlet-name>headerservlet</servlet-name> <servlet-class>edu.test.myservlets.HeaderDetailsServlet</servlet-class> </servlet> <servlet-mapping> <servlet-name>headerservlet</servlet-name> <url-pattern>/test/myservlet</url-pattern> </servlet-mapping> </web-app>
Let’s look at more methods of ServletRequest:
java.lang.String getCharacterEncoding() – returns the name of the character encoding used in the body of this request.
java.lang.String getContentType() – returns the MIME type of the request body, or null if the type is not known.
java.lang.String getLocalAddr()– returns the IP address of the interface where the request was received.
java.util.Locale getLocale() – returns the preferred Locale that the client will accept content in. It is based on the Accept-Language header.
java.lang.String getProtocol() – returns the name and version of the protocol the request uses. For example, HTTP/1.1.
java.lang.String getScheme() – returns the name of the scheme used to make this request. E.g: http, https, ftp.
boolean isSecure() – returns true if this request was made using a secure channel. For example, HTTPS.
ServletInputStream getInputStream() – gets the request body as binary data using a ServletInputStream.
Cookie[] getCookies() – returns an array of all the Cookies that were sent by a client with this request.java.lang.String getAuthType() – returns the name of the servlet authentication type.
ServletResponse interface
ServletResponse object assists a servlet in sending a response to the client. The servlet container is responsible for the creation of a ServletResponse object. After creation, it passes ServletResponse object as an argument to the service method of the servlet.
If we need to send binary data in a MIME body response, better to use the ServletOutputStream returned by getOutputStream(). In the case of sending character data, it is better to use the PrintWriter object returned by getWriter(). If we need to send a mix of binary and text data, it is good to use a ServletOutputStream and work on the character sections manually.
You can specify charset for the MIME body response explicitly, by using the setCharacterEncoding(java.lang.String) and setContentType(java.lang.String) methods. Also, we can do this implicitly by using the setLocale(java.util.Locale) method. The ISO-8859-1 is used as default charset. The setCharacterEncoding, setContentType, setLocale method must be called before getWriter and before committing the response for the character encoding to be used.
Let’s look at the example where we will utilize the two most used methods setContentType and getWriter:
package edu.myservlets.example; import java.io.*; import javax.servlet.*; import javax.servlet.http.*; // we are extending HttpServlet class public class ServletResponseExample extends HttpServlet { public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // Set response content type response.setContentType("text/html"); PrintWriter out = response.getWriter(); out.print("<html><body><h1>"); out.print("This is our HTML response"); out.print("</h1></body></html>"); out.close(); } } Let's look at one more example that will open our response in Excel: package edu.myservlets.example; import java.io.*; import javax.servlet.*; import javax.servlet.http.*; // we are extending HttpServlet class public class ServletResponseExcelExample extends HttpServlet { public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // Set response content type response.setContentType("application/vnd.ms-excel"); PrintWriter out = response.getWriter(); out.println("Name\t Surname\t Age"); out.println("Mickey\t Mouse\t 6"); out.println("Minnie\t Mouse\t 6"); out.close(); } }
Here are more methods of the ServletResponse interface:
String getCharacterEncoding() – returns the character encoding (MIME charset) used for the response body. For example, UTF-8.
String getContentType() – returns a String specifying the content type. For example, text/html; charset=UTF-8. If content type was not specified, this method returns null.
ServletOutputStream getOutputStream() – returns ServletOutputStream object, that is suitable for writing binary data in the response. We need to call flush() on the ServletOutputStream to commit the response.
void setCharacterEncoding(String charset) – sets the character encoding of the response being sent to the client. This method overrides the character encoding that was set by different methods(setContentType(java.lang.String) or setLocale(java.util.Locale)).
void setContentLength(int length) – sets the content body length in the response for HTTP servlet. This method sets the HTTP Content-Length header. The integer value is used as input. There is the method that does the same, but get long as input data void setContentLengthLong(long length).
void setContentType(String type) – the method sets the type of the response content being sent to the client. This method can be called several times. But, the method has no effect if called after the response has been committed. Servlet Containers need to know the content type and the character encoding of the servlet response and communicate them with the client.
void setBufferSize(int size) – sets the buffer size for the response body.
A larger buffer allows more content before anything is actually sent at once. But, a smaller buffer allows the client to start receiving data more quickly. Also, it decreases the server memory load.
int getBufferSize() – method to get buffer size of the response body.
void flushBuffer() – forces any content in the buffer to be sent to the client. It automatically commits the response with the status code and headers will be written.
void reset() – clears all data that exists in the buffer. The status code, headers will be also cleared. The state of calling getWriter() or getOutputStream() is also cleared. boolean isCommitted() – returns true if the response has been commited
5 Comments