TestNG @Test Priority in Selenium
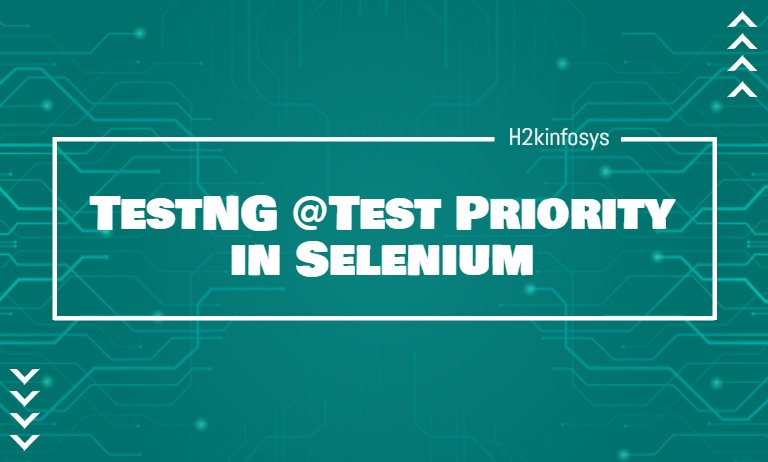
What is Test Priority?
In TestNG “Prioritization” means assigning the Execution Priority on Test Case. For example we want to execute the test cases in order when there are multiple test cases. i.e. First we need to execute “Registration” test case before login. The priority on the test case will define the Execution order of Test Case. It can be defined at Method Level. To assign the priority to a test case, you need to define the test priority at method level within the @Test annotation.
In order to set the priority, we need to add annotation as @Test (priority=?). The default value for priority will be zero. If you don’t mention the priority, it will take as “priority=0” for all the test cases and execute. Lower priorities will be executed first. If we define priority as “priority=” for multiple test cases, then these test cases will get executed only after all the test cases which don’t have any priority and set priority to 0 as the default priority.
Syntax:
//Valid Syntax @Test(priority = 1) //Invalid Syntax @Test(PRIORITY = 1)
TestNG Test Cases without Priority
Let’s consider below TestNG program where we will not define any priority with the test method.
Step 1: Launch the Eclipse
package testNGPriority; import org.testng.annotations.Test; public class NoPriority_Example { @Test public void one() { System.out.println("First Test Case"); } @Test public void two() { System.out.println("Second Test Case"); } @Test public void three() { System.out.println("Third Test Case"); } }
Step 2: Right click on Java class > Run As and then click on the TestNG Test.
Output:
In the above program, we declared a class that contains three test methods one(), two(), and three() without any priority. From the output we got TestNG executed the test methods in alphabetical order of their method names irrespective of their place of implementation in the code.
So, to execute the test methods as per our needs, we will set the priority with @Test annotation.
How to Set Priority in TestNG for Test Cases?
Let’s consider below TestNG program where we will define priority with the test methods to execute in sequential order.
Step 1: Launch the Eclipse
package testNGPriority; import org.testng.annotations.Test; public class testNGPriorityExample { @Test(priority = 1) public void one() { System.out.println("First Test Case"); } @Test(priority = 2) public void two() { System.out.println("Second Test Case"); } @Test(priority = 3) public void three() { System.out.println("Third Test Case"); } }
Step 2: Right click on Java class > Run As and then click on the TestNG Test.
Output:
After assigning the priority to each test method the output has changed. From the above output you can see the test method having lower priority is executed first i.e. execution is happened in sequential order based on priority assigned to the test method.
Thus, alphabetical order method name has not been considered to the test methods as we provide priorities and all the test cases has got executed.
Test Methods with Same Priority
Let’s consider below TestNG program where we will define the test methods with same priority and check in which order the TestNG executes.
Step 1: Launch the Eclipse
package testNGPriority; import org.testng.annotations.Test; public class SamePriorityExample { @Test(priority = 1) public void firstTest() { System.out.println("First test method"); } @Test(priority = 1) public void secondTest() { System.out.println("Second test method"); } @Test(priority = 0) public void thirdTest() { System.out.println("Third test method"); } @Test(priority = -1) public void fourthTest() { System.out.println("Fourth test method"); } @Test(priority = -2) public void fifthTest() { System.out.println("Fifth test method"); } }
Step 2: Right click on Java class > Run As and then click on the TestNG Test.
Output:
In the above example, a class contains five test methods in which two methods have the same priority. In such cases, TestNG will execute in alphabetical order of the method names which having the same priority. Out of five test methods, three methods got executed based on priority values. But the firstTest() and secondTest() methods contains the same priority value 1. So, in this case TestNG will consider the alphabetical order of ‘f’ and ‘s’ and executes them accordingly.
Combination of Prioritized and Non-prioritized methods
Let’s consider below TestNG program where we will cover two test cases i.e. methods having same priority value and more than one non-prioritized method in one TestNG class.
Step 1: Launch the Eclipse
Step 2: Create Class name as “TestNGPriorityTest”
package testNGPriority; import org.testng.annotations.Test; public class TestNGPriorityTest { @Test public void m1_c() { System.out.println("m1_c"); } @Test public void m1_b() { System.out.println("m1_b"); } @Test(priority = 1) public void m1_a() { System.out.println("m1_a"); } @Test(priority = 1) public void m1_d() { System.out.println("m1_d"); } @Test(priority = 0) public void m1_e() { System.out.println("m1_e"); } @Test(priority = 2) public void m1_f() { System.out.println("m1_f"); } }
Step 2: Right click on Java class > Run As and then click on the TestNG Test.
Output:
First preference: In the above class, m1_b() and m1_c() which are non-prioritized test methods have been executed based on alphabetical order ‘b’ and then ‘c’.
Second preference: m1_e() which is prioritized methods has been executed first because of having the highest priority (0). As the test methods m1_a() and m1_d() have the same priority. Therefore, TestNG will considered the alphabetical order of their methods names. So, between them, “m1_a” was executed first and then “m1_d”.
Third preference: m1_f() is executed last by TestNG because of having the lowest priority (2).
Let’s consider the scenario where we automate Google home page and print the title of home page by using the priority feature.
Step 1: Launch the Eclipse
Step 2: Create the Class name as “GoogleTest”
package sampletestpackage; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import org.testng.annotations.Test; public class GoogleTest { WebDriver driver; @Test(priority = 1) public void driverSetup() { System.setProperty("webdriver.chrome.driver", "D:\\Drivers\\geckodriver.exe"); driver=new ChromeDriver(); } @Test(priority = 2) public void getURL() { driver.get("https://www.google.com"); } @Test(priority = 3) public void getTitle() { String title = driver.getTitle(); System.out.println(title); } @Test(priority = 4) public void closeBrowser() { driver.close(); System.out.println("Test successfully passed"); } }
Output:
Conclusion: To run a set of test-case in specific sequence this can be done easily using Priority using testNG.
One Comment