Using the find() Method in Python with Examples
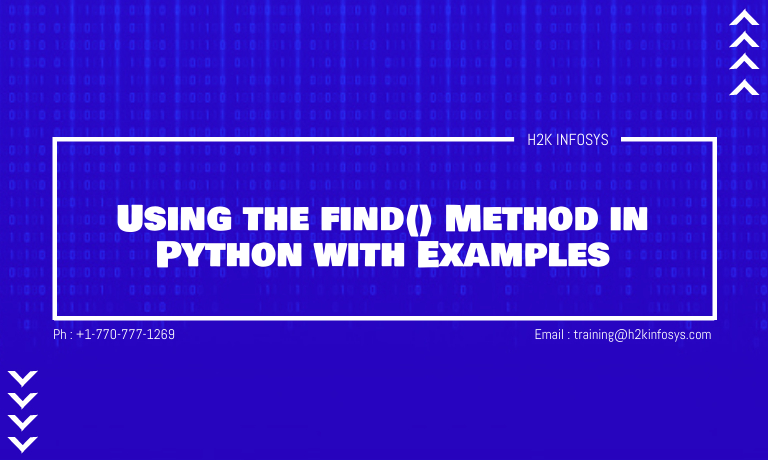
In Python, you can find the position/index of a character or index using the find() method. The find() method is a built-in method in Python that returns the index of the first occurrence of a substring. Where the substring is not found in the string, the find() method returns -1. In this tutorial, we will focus on how to use the find method, and how to play around with its arguments to suit your needs. By the end of this tutorial, you will learn:
- Using the find() method
- Using the find() method with the start Argument Defined.
- Using the find() Method with the start and end Arguments defined.
- Using the find() method for Substrings not Present
- Using the rfind() method.
- Using the index() method in Python.
- How to calculate the total number of times a Substring Exists
We will begin with its syntax.
Syntax:
find(sub: Text, start: Optional[int]=..., end: Optional[int]=..., /) -> int
Parameters: There are 3 parameters to be defined. Just 1 is required, the other two are optional.
- Sub (required): This is the substring you wish to find.
- Start (optional): You can tweak where you want the Python interpreter to begin its search. By default, it is set to 0 which means that the search begins from the first index of the string.
- end (optional): This allows you to change the index where the Python interpreter begins its search and stops. By default, it is set to the length of the string.
Using the find() method
We will begin by simply using the find() method by defining only the substring to be found. This implies that the default values for the start and end parameters will be taken. Let’s say we wish to find the index of the first ‘s’ in H2k Infosys, we can run the code below.
#check the first existence of 's' in the string defined string = 'H2k Infosys' print(f"The first 's' exists at index {string.find('s')}")
Output:
The first 's' exists at index 8
In the same vein, you can find substrings as words. When substrings are in the form of words, the index of the first character of the word is returned. Again, if the substring occurs more than once, the Python interpreter returns the index of the first occurrence. Let’s see an example.
#check the first existence of 's' in the string defined string = 'Learning Python with H2k Infosys has been fun. I will keep on learning Python' print(f"The first 'Python' exists at index {string.find('Python')}")
Output:
The first 'Python' exists at index 9
Using the find() method with the start Argument Defined.
When you specify the start argument, the interpreter begins its search from that index. This means that even if the substring to be found exists in an earlier index, it will not be chosen. One important thing to point out is that although the interpreter begins its search from the integer passed, the count begins at the first index nevertheless.
In the last example, there exists another ‘Python’ word after the 9th index, we can search for it and begin choosing a start index greater than 9. Let’s pick 10.
#check the first existence of 's' in the string defined string = 'Learning Python with H2k Infosys has been fun. I will keep on learning Python' print(f"The first 'Python' exists at index {string.find('Python', 10)}")
Output:
The first 'Python' exists at index 71
Using the find() Method with the start and end Arguments defined.
Defining the start and end argument gives you more flexibility when using the find() method. It limits not only where the interpreter begins its search but where it ends. Let’s take an example
#check the first existence of 's' in the string defined string = 'Learning Python with H2k Infosys has been fun. I will keep on learning Python' print(f"The first 'Python' exists at index {string.find('Python', 0, 15)}")
Output:
The first 'Python' exists at index 9
Note that this only happens when the start argument has been defined. The find() method does not accept keywords arguments but rather positional arguments so you’d need to define a start argument before the end argument can work.
Using the find() method for Substrings not Present
When the substring is not found. The Python interpreter returns -1. This can be useful in codes where you want to ensure the string is not present before an action is done.
Let’s take an example.
#check the first existence of 's' in the string defined string = 'Learning Python with H2k Infosys has been fun. I will keep on learning Python' print(f"The first 'Java' exists at index {string.find('Java')}")
Output:
The first 'Java' exists at index -1
As seen it returns -1. This is because Java does not appear in the string defined.
Using the rfind() method.
You may be wondering, what about if you require the index of the last string occurrence. Well, there is a method for it and it is called the rfind() method. The rfind() method works like the find method but returns the highest index of the substring. This implies that the index of the last substring occurrence is returned. If, for instance, we call the rfind() function on the string, ‘Python’, the index of ‘P’ in the last occurrence is returned, rather than the first occurrence in find(). Let’s see a coding example.
#check the first existence of 's' in the string defined string = 'Learning Python with H2k Infosys has been fun. I will keep on learning Python' print(f"The first 'Python' exists at index {string.find('Python')}") print(f"The last index 'Python is at index {string.rfind('Python')}")
Output:
The first 'Python' exists at index 9
The last index 'Python is at index 71
Using the index() method in Python.
The index() method works exactly like the find method in that it returns the index of the first occurrence of a substring. Let’s give it a shot link in previous examples with the find() method.
#check the first existence of 's' in the string defined string = 'Learning Python with H2k Infosys has been fun. I will keep on learning Python' print(f"The first 'Python' exists at index {string.index('Python')}")
Output:
The first 'Python' exists at index 9
The only difference between the index() method and the find() method is that the index() method throws an error when the substring is not found. Remember its find() method counterpart returns -1. Let’s run the two functions on the same string.
#check the first existence of 's' in the string defined string = 'Learning Python with H2k Infosys has been fun. I will keep on learning Python' print(f"The first 'Java' exists at index {string.find('Java')}") print(f"The first 'Java' exists at index {string.index('Java')}")
Output:
The first 'Java' exists at index -1
Traceback (most recent call last):
File "c:/Users/DELL/Desktop/pycodes/__pycache__/strings.py", line 5, in <module>
print(f"The first 'Java' exists at index {string.index('Java')}")
ValueError: substring not found
As seen, the find() returns -1 while the index() method throws a ValueError.
How to calculate the total number of times a Substring Exists
One of the fun things to do with the find() method is to calculate the total number of times a python substring occurs in a string. In this example, we will loop over the total length of the string and call the find() method. Where the string is found, the method returns 1, which is added to a counter initialized as 0. The index of the counter is then reassigned as the index + 1, where the find() method returned a 1.
This explanation is done in Python as shown below.
#check the first existence of 's' in the string defined string = 'Learning Python with H2k Infosys has been fun. I will keep on learning Python' #define a string to count find_string = 'Python' start_index = 0 counter = 0 #check if the string exist, add one to the index and reassign counter for x, _ in enumerate(string): if string.find(find_string, start_index) != -1: counter += 1 #reassign the index (+ 1) so that the earlier substring will not be recounted start_index = string.find(find_string, start_index) + 1 #print the result print(f"{find_string} exists {counter} times in the string")
Output:
Python exists 2 times in the string
As seen, Python appears twice in the string. Let’s assume we want to search for H2k, we simply change the find_string variable to H2k. See the code below.
#check the first existence of 's' in the string defined string = 'Learning Python with H2k Infosys has been fun. I will keep on learning Python' #define a string to count find_string = 'H2k' start_index = 0 counter = 0 #check if the string exist, add one to the index and reassign counter for x, _ in enumerate(string): if string.find(find_string, start_index) != -1: counter += 1 #reassign the index (+ 1) so that the earlier substring will not be recounted start_index = string.find(find_string, start_index) + 1 #print the result print(f"{find_string} exists {counter} time(s) in the string")
Output:
H2k exists 1 time(s) in the string
To conclude,
You have seen how to use the find() method to check the index of a substring in a list. You also learn that the rfind() rather returns the index of the last element. Going forward, you learned that the index method behaves like the find method but throws an error in the event that the substring is not found. Finally, you discovered how to count the number of elements in a string by using the find() method.
If you’ve got any questions, feel free to leave them in the comment section and I’d do my best to answer them.