Using the len() Function in Python with Examples
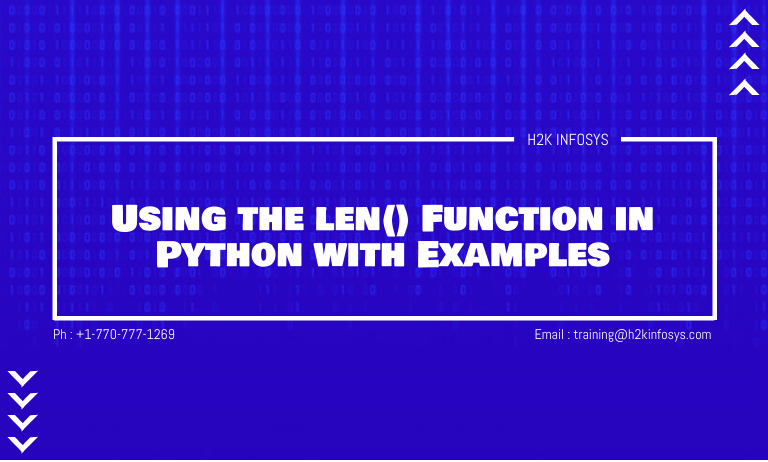
The len() function is an inbuilt python function used to return the number of characters in a string or the number of items in a collection such as a list, tuple, dictionary etc.
Using the function has a lot of use cases when writing day to day codes. You may need to check the length of an object during iterations and what have you. In this tutorial, we will take several examples on how to use the len function in different Python objects.
Let’s begin with its syntax
Syntax:
Signature: len(obj, /) Docstring: Return the number of items in a container. Type: builtin_function_or_method
Parameters: The object whose length wants to be returned. The object could be a string, list, tuple, dictionary etc.
Returns: The length of the object given
Let’s take some examples.
Finding the length of a String
The len() function returns the length of a string. It is important to note that every character in the string would be counted including whitespace, punctuations and any other type of special characters.
#check the length of a string string = "I am learning Python with H2k Infosys" print(f"The string has {len(string)} characters")
Output:
The string has 37 characters
Finding the length of a List
The len function returns the number of elements in a list. See the example below.
#check the length of a list # string = "I am learning Python with H2k Infosys" names = ['Mike', 'Josh', 'Ope', 'Toby', 'Fred', 'Krish'] print(f"The list has {len(names)} items")
Output:
The list has 6 items
Finding the length of a Tuple
A tuple is a container that stores a collection of items, like in a list. The major difference between a tuple and a list is that the element in a tuple cannot be changed. They are immutable. You can also find the number of elements in a tuple by using the len() function. See the example below.
#check the length of a list # string = "I am learning Python with H2k Infosys" names = ('Mike', 'Josh', 'Ope', 'Toby', 'Fred', 'Krish') print(f"The object is of datatype, {type(names)}") print(f"The tuple has {len(names)} items")
Output:
The object is of datatype, <class 'tuple'>
The tuple has 6 items
Finding the length of a Dictionary
The len function can be used to count the length of a dictionary. Every key-value pair is seen as one unit.
#check the length of a list # string = "I am learning Python with H2k Infosys" info = {'names': ['Mike', 'Vic', 'Ope', 'Toby', 'Mary', 'Krish'], 'age': [12, 32, 20, 21, 43, 25], 'gender': ['M', 'M', 'M', 'F', 'F', 'M']} print(f"The object is of datatype, {type(info)}") print(f"The tuple has {len(info)} items")
Output:
The object is of datatype, <class 'dict'>
Notice that the length of the dictionary is given as 3 items even though the values for each key is a list of 6 items. Again, a key-value pair is seen as one item. There were 3 key-value pairs in the dictionary, names, age and age. This is why the len function returns 3 for the dictionary.
In summary
You have seen how to use the len() function for various data types and structures in Python. It can come handy when you wish to loop over the number of items in an iterator or even in simple cases of you finding how many items the iterator contains. If you have any questions, feel free to leave them in the comment section and I’d do my best to answer them.