JAVA Tutorials
What are Servlet Filters?
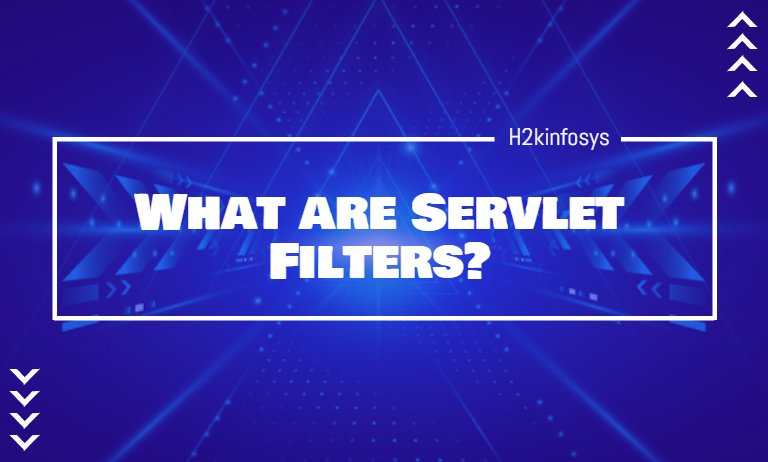
A Servlet Filters is an object that is used to perform filtering tasks such as conversion, encryption, decryption, etc. The servlet filter entry is done in a file named web.xml. If the entry is removed from this file, then we do not need to change the servlet, and the filter will be automatically removed. This implies that the maintenance cost is low. The servlet filters is also used for logging the IP address of the system, which requests the server.
How does Servlet Filter work?
- When a request is made, it reaches the Web Container and checks if the filter contains any URL pattern, which is similar to the pattern of the URL requested.
- The Web Container locates the very first filter which matches the request URL, and then that filter code is executed.
- If more than one pattern is found, then all the matching filter codes are executed.
- When all the matching filter codes are executed successfully and no error is found, then the request is passed to the Servlet.
- The Servlet then returns the response to the caller, and then this response will be passed to the client passing through the Web Container.
Methods in Servlet Filter
- public void init(FilterConfig config): This method is used to initialize the filter, and this method is invoked only once in the lifecycle of Servlet.
- public void doFilter(HttpServletRequest request, HttpServletResponse response, FilterChain chain): This function is used to perform filtering tasks and is invoked whenever a request is made. It is also used to call the next filter available in the chain.
- public void destroy(): This method is also invoked only once when the service of the filter is complete, and we want to close all the resources used by the Servlet Filters.
How to declare a Servlet Filter in web.xml file:
<filter> <filter-name>RequestLoggingFilter</filter-name> <!-- mandatory --> <filter-class>com.journaldev.servlet.filters.RequestLoggingFilter</filter-class> <!-- mandatory --> <init-param> <!-- optional --> <param-name>test</param-name> <param-value>testValue</param-value> </init-param> </filter>
How to map a Filter to the Servlet Classes:
<filter-mapping> <filter-name>RequestLoggingFilter</filter-name> <!-- mandatory --> <url-pattern>/*</url-pattern> <!-- either url-pattern or servlet-name is mandatory --> <servlet-name>LoginServlet</servlet-name> <dispatcher>REQUEST</dispatcher> </filter-mapping>
Given Below is the example of Servlet Filter implementation:
import javax.servlet.*; import java.io.IOException; public class SimpleServletFilter implements Filter { public void init(FilterConfig filterConfig) throws ServletException { } public void doFilter(ServletRequest request, ServletResponse response, FilterChain filterChain) throws IOException, ServletException { } public void destroy() { } }
Example of Servlet Filter:
import java.io.*; import javax.servlet.*; import javax.servlet.http.*; import java.util.*; public class LogFilter implements Filter { public void init(FilterConfig config) throws ServletException { String testParam = config.getInitParameter("test-param"); System.out.println("Test Param: " + testParam); } public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain) throws java.io.IOException, ServletException { String ipAddress = request.getRemoteAddr(); System.out.println("IP "+ ipAddress + ", Time " + new Date().toString()); chain.doFilter(request,response); } public void destroy( ) { } }
Using Multiple Filters:
<filter> <filter-name>LogFilter</filter-name> <filter-class>LogFilter</filter-class> <init-param> <param-name>test-param</param-name> <param-value>Initialization Paramter</param-value> </init-param> </filter> <filter> <filter-name>AuthenFilter</filter-name> <filter-class>AuthenFilter</filter-class> <init-param> <param-name>test-param</param-name> <param-value>Initialization Paramter</param-value> </init-param> </filter> <filter-mapping> <filter-name>LogFilter</filter-name> <url-pattern>/*</url-pattern> </filter-mapping> <filter-mapping> <filter-name>AuthenFilter</filter-name> <url-pattern>/*</url-pattern> </filter-mapping>
Facebook Comments