Introduction
In the modern world of software development and data processing, one of the most common challenges is managing data in various formats. Whether you’re dealing with large volumes of XML data, transferring information between different systems, or simply trying to integrate data from various sources, an effective approach to managing these data formats is crucial.
This is where JAXB (Java Architecture for XML Binding) comes in. While JAXB is predominantly used in Java applications for binding Java objects to XML and vice versa, its principles and use cases often have a cross-industry relevance, including in fields like Data Science. Understanding JAXB can be valuable for any aspiring data professional, especially when working with data formats or processing data for Java full stack developer course or any other programming framework.
In this blog post, we will explore what JAXB is, how it works, and why it is a vital tool for managing and manipulating XML data. We will also discuss how JAXB principles can be applied across different domains, including Java, and provide insights into how integrating such technologies with the best Java certification programs can elevate your career.
What is JAXB?
JAXB (Java Architecture for XML Binding) is a Java framework that provides a convenient way to map Java objects to XML representations and vice versa. It is a part of Java’s standard API, introduced as part of the J2SE 1.6 release. The core idea behind JAXB is to facilitate the conversion between XML data and Java objects, simplifying the process of reading and writing XML content.
In essence, It eliminates the need for developers to manually parse XML data and handle its complexities. Instead, JAXB automates the process by providing annotations that can be applied to Java classes, allowing them to be automatically serialized (converted to XML) or deserialized (converted from XML) without the need for a custom parser.
JAXB (Java Architecture of XML Binding) is a fast and convenient way to convert XML into Java objects, and Java objects to XML. It consists of an API for reading and writing Java objects to and from XML.
You can perform the following two operations using JAXB:
- Marshalling: It means converting Java objects to XML.
- UnMarshalling: It means converting XML to Java objects.
Key Features and Benefits of JAXB
Automatic Conversion Between Java Objects and XML
It simplifies the process of mapping Java objects to XML elements and vice versa. It uses annotations such as @XmlRootElement
and @XmlElement
to indicate which parts of a Java class should be converted into XML tags. This automatic conversion reduces the complexity of data serialization and deserialization.
Seamless Integration
One of the key advantages of JAXB is its seamless integration with other Java technologies, such as JAX-RS for RESTful web services, and JAX-WS for SOAP-based web services. This makes it a powerful tool for developers building web services that need to handle XML payloads.
Customization and Flexibility
JAXB offers a high degree of customization through binding customizations. Developers can fine-tune how their Java objects are marshaled into XML and unmarshaled back into objects by using XML Schema and various JAXB annotations. This makes JAXB a flexible tool suited for a wide range of XML processing tasks.
Efficiency in Data Interchange
JAXB is highly efficient for processing XML data. By automating the binding process, It reduces the need for manual XML parsing, making the development process faster and more reliable. This is particularly useful in environments where XML is a primary data format, such as web services or data exchange between heterogeneous systems.
How JAXB Works: A Breakdown
To better understand JAXB, let’s look at a basic example of how it works:
- Java Class Creation: You create a Java class that represents your data model.
- Add JAXB Annotations: You annotate the Java class with JAXB annotations that define how the class should be mapped to XML elements.
- Marshalling and Unmarshalling:
- Marshalling: You can convert a Java object into an XML document using the
Marshaller
class. - Unmarshalling: You can convert an XML document back into a Java object using the
Unmarshaller
class.
- Marshalling: You can convert a Java object into an XML document using the
Here’s a simple illustration:
Java Class Example:
<pre>
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
@XmlRootElement
public class Employee {
private String name;
private int age;
// Getters and setters
@XmlElement
public String getName() {
return name;
}
@XmlElement
public int getAge() {
return age;
}
}
</pre>
Marshalling (Java Object to XML):
javaCopy codeimport javax.xml.bind.JAXBContext;
import javax.xml.bind.JAXBException;
import javax.xml.bind.Marshaller;
public class MarshallingExample {
public static void main(String[] args) throws JAXBException {
Employee employee = new Employee();
employee.setName("John Doe");
employee.setAge(30);
JAXBContext context = JAXBContext.newInstance(Employee.class);
Marshaller marshaller = context.createMarshaller();
marshaller.marshal(employee, System.out); // Output the XML representation
}
}
Unmarshalling (XML to Java Object):
javaCopy codeimport javax.xml.bind.JAXBContext;
import javax.xml.bind.JAXBException;
import javax.xml.bind.Unmarshaller;
public class UnmarshallingExample {
public static void main(String[] args) throws JAXBException {
String xml = "<employee><name>John Doe</name><age>30</age></employee>";
JAXBContext context = JAXBContext.newInstance(Employee.class);
Unmarshaller unmarshaller = context.createUnmarshaller();
Employee employee = (Employee) unmarshaller.unmarshal(new StringReader(xml));
System.out.println(employee.getName() + " - " + employee.getAge());
}
}
Practical Applications of JAXB
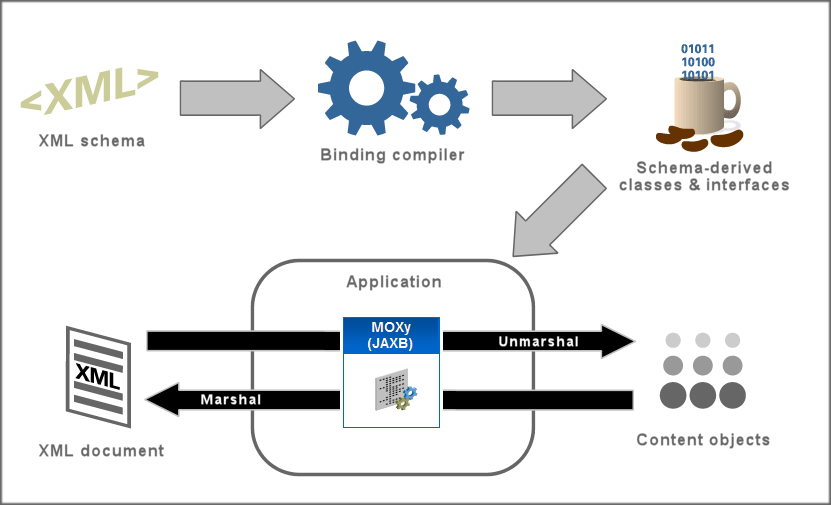
While It is primarily a Java-based tool for XML processing, its principles are highly relevant to the field of Java development. Developers often work with large datasets that come in a variety of formats, including JSON, CSV, and XML. Understanding tools like JAXB, which deal with XML data, is an important skill for Java professionals who need to handle data from external sources, APIs, or complex systems.
Data Transformation and Preprocessing:
XML data is often used in APIs, web services, and other systems. Understanding how to work with this format and converting it into a usable structure for analysis is crucial for Java developers. It enables smooth data extraction and transformation, which is necessary when preparing data for further processing or integration with other Java applications.
Handling Diverse Data Sources:
In the real world, Java developers frequently deal with data that is stored in XML format. Having a solid understanding of JAXB can help them efficiently parse and manipulate this data, whether it’s coming from legacy systems or third-party APIs.
Integration with Python:
Although It is a Java-specific tool, the knowledge of data binding principles it provides can be applied to Python-based tools like Pandas or similar data libraries. Java developers who are familiar with data binding can quickly adapt to handling JSON, CSV, and other structured formats in Java or Python, depending on the requirements of the project.
Data Interchange in Java Projects:
For larger-scale Java projects involving different systems, It facilitates the transfer of data between Java-based systems and other components. This ensures smooth communication and data flow, essential for team collaboration on large Java development projects.
How to Get Started with JAXB
Getting started with JAXB involves learning its basic components and how it integrates into Java-based applications. For developers interested in data science course online programs or those looking to enhance their programming skills, It provides a solid foundation in understanding data binding concepts.
Many online courses, including the best data science certification programs, offer modules where JAXB and similar technologies are covered, providing a comprehensive understanding of data transformation and integration. By mastering these concepts, you not only learn how to handle various data formats but also enhance your ability to work with different programming frameworks and languages.
Key Takeaways
- JAXB (Java Architecture for XML Binding) is a framework that simplifies the conversion of Java objects to XML and vice versa, reducing manual parsing and enhancing productivity.
- It’s highly beneficial in handling XML data, which is widely used in web services and APIs.
- In the realm of Data Science using Python Online Training, The principles apply when working with external datasets and transforming data for analysis.
- Understanding it is essential for data scientists working with heterogeneous systems and APIs, ensuring smooth data handling and processing.
JAXB Unmarshalling: This means converting the XML into an object. The steps that are used to convert XML into Java object are:
- Create POJO and generate the classes.
- Create a JAXBContext object.
- Create a Marshaller object.
- Call the marshal method.
- Use the getter method to access the data.
Advantages of JAXB:
- It is simpler than the SAX or DOM parser.
- No need to be aware of XML parsing techniques.
- No need to access XML in a tree structure.
Disadvantages of JAXB:
Performance Overhead:
- It can introduce performance overhead due to the conversion process between XML and Java objects. Large XML files or complex object models can significantly slow down the application.
Memory Consumption:
- It tends to consume more memory as it loads the entire XML document into memory when binding it to Java objects. This can be problematic for large XML documents, leading to memory management issues.
Limited Flexibility in XML Structure:
- It is limited when working with XML structures that do not conform to a fixed schema or have complex, highly nested elements. It works best with well-defined schemas, making it less flexible when dealing with dynamic or loosely defined XML formats.
Error Handling Complexity:
- JAXB’s error handling can be complex, especially when dealing with XML validation errors. The error messages are often not very descriptive, making it difficult to debug and fix issues during the unmarshalling process.
Dependency on Annotations:
- JAXB relies heavily on annotations to map Java classes to XML schemas. This can clutter the code, making maintenance difficult, especially when dealing with large object models.
Limited Support for JSON:
- While It is designed primarily for XML, its JSON support is not as robust or straightforward compared to other libraries specifically designed for JSON processing, like Jackson or GSON.
Requires XML Schema Definition (XSD):
- JAXB often requires an XML Schema Definition (XSD) to generate Java classes, which adds an extra step to the development process. Managing XSD files can be cumbersome and error-prone.
Static Binding:
- It uses static binding, meaning that once the Java classes are generated, changes to the XML structure require regeneration of these classes. This can be time-consuming and error-prone in agile development environments where changes are frequent.
Compatibility Issues with Complex XML Structures:
- It struggles with XML features such as mixed content, namespaces, or advanced schema constructs. Handling such complexities requires additional code and workarounds, which can complicate development.
Steep Learning Curve:
- For developers unfamiliar with binding concepts, It can have a steep learning curve, particularly when dealing with advanced features such as customizing bindings or handling complex XML schemas.
These disadvantages make it suitable primarily for scenarios where XML schemas are stable, and performance or memory constraints are not critical. For more dynamic or large-scale XML processing, alternative approaches may be more efficient.
Conclusion
As the world of Java Online Training continues to grow, professionals must be prepared to work with various data formats. While it is primarily used in Java, its principles and functionalities are relevant for anyone working with XML data in data-driven environments. By learning about JAXB, professionals can handle XML data with ease, preparing them for complex data science challenges. If you’re looking to enhance your skills in data handling and manipulation, pursuing Java full stack developer training can help you build the foundational knowledge needed for success.
To learn more and gain hands-on experience in data science, consider enrolling in H2K Infosys courses. Gain the skills you need to thrive in the world of data science and start your journey today!