What is JAXB?
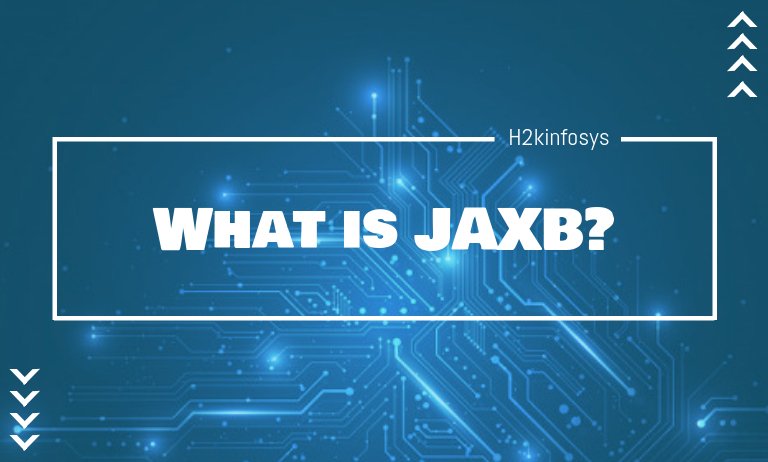
JAXB (Java Architecture of XML Binding) is a fast and convenient way to convert XML into Java objects, and Java objects to XML. It consists of an API for reading and writing Java objects to and from XML.
You can perform the following two operations using JAXB:
- Marshalling: It means converting Java objects to XML.
- UnMarshalling: It means converting XML to Java objects.
Features of JAXB:
- Annotation support: JAXB supports annotation, so less coding is required. The javax.xml.bind.annotation package consists of classes and interfaces required to develop applications.
- Support all W3C XML Schema features: It supports all the W3C schema features.
- Additional Validation Capabilities: It supports additional validation capabilities supported by JAXP validation API.
- Small Runtime Library: It requires a small runtime library.
- Reduction of generated schema-derived classes: It reduces the usage of several generated schema-derived classes.
Annotations used in JAXB:
Java Annotations are used in JAXB for augmenting the generated classes with additional information, which helps in preparing them for JAXB runtime. The below example will help to understand the annotations easily:
@XmlRootElement(name = "book") @XmlType(propOrder = { "id", "name", "date" }) public class Book { private Long id; private String name; private String author; private Date date; @XmlAttribute public void setId(Long id) { this.id = id; } @XmlElement(name = "title") public void setName(String name) { this.name = name; } @XmlTransient public void setAuthor(String author) { this.author = author; } }
- @XmlRootElement: it contains the name of the root XML element.
- @XmlType: it defines the order in which the fields are written.
- @XmlElement: it defines the actual XML element name.
- @XmlAttribute: it defines the id field that is mapped as an attribute.
- @XmlTransient: it consists of those fields, which we do not want to include in XML.
JAXB Marshalling: It means converting the object into XML. The steps that are used to convert Java objects into XML are:
- Create POJO and generate the classes.
- Create a JAXBContext object.
- Create a Marshaller object.
- Create a content tree by using the set method.
- Call the marshal method.
Question.java
import java.util.List; import javax.xml.bind.annotation.XmlAttribute; import javax.xml.bind.annotation.XmlElement; import javax.xml.bind.annotation.XmlRootElement; @XmlRootElement public class Question { private int id; private String questionname; private List<Answer> answers; public Question() {} public Question(int id, String questionname, List<Answer> answers) { super(); this.id = id; this.questionname = questionname; this.answers = answers; } @XmlAttribute public int getId() { return id; } public void setId(int id) { this.id = id; } @XmlElement public String getQuestionname() { return questionname; } public void setQuestionname(String questionname) { this.questionname = questionname; } @XmlElement public List<Answer> getAnswers() { return answers; } public void setAnswers(List<Answer> answers) { this.answers = answers; } }
Answer.java
public class Answer { private int id; private String answername; private String postedby; public Answer() {} public Answer(int id, String answername, String postedby) { super(); this.id = id; this.answername = answername; this.postedby = postedby; } public int getId() { return id; } public void setId(int id) { this.id = id; } public String getAnswername() { return answername; } public void setAnswername(String answername) { this.answername = answername; } public String getPostedby() { return postedby; } public void setPostedby(String postedby) { this.postedby = postedby; } }
ObjectToXml.java
import java.io.FileOutputStream; import java.util.ArrayList; import javax.xml.bind.JAXBContext; import javax.xml.bind.Marshaller; public class ObjectToXml { public static void main(String[] arg) throws Exception{ JAXBContext contextObj = JAXBContext.newInstance(Question.class); Marshaller marshallerObj = contextObj.createMarshaller(); marshallerObj.setProperty(Marshaller.JAXB_FORMATTED_OUTPUT, true); Answer ans1=new Answer(101,"java is a programming language","ravi"); Answer ans2=new Answer(102,"java is a platform","john"); ArrayList<Answer> list=new ArrayList<Answer>(); list.add(ans1); list.add(ans2); Question que=new Question(1,"What is java?",list); marshallerObj.marshal(que, new FileOutputStream("question.xml")); } }
Output:
<?xml version="1.0" encoding="UTF-8" standalone="yes"?> <question id="1"> <answers> <answername>java is a programming language</answername> <id>101</id> <postedby>ravi</postedby> </answers> <answers> <answername>java is a platform</answername> <id>102</id> <postedby>john</postedby> </answers> <questionname>What is java?</questionname> </question>
JAXB Unmarshalling: It means converting the XML into an object. The steps that are used to convert XML into java object are:
- Create POJO and generate the classes.
- Create a JAXBContext object.
- Create a Marshaller object.
- Call the marshal method.
- Use the getter method to access the data.
Question.xml
<?xml version="1.0" encoding="UTF-8" standalone="yes"?> <question id="1"> <answers> <answername>java is a programming language</answername> <id>101</id> <postedby>ravi</postedby> </answers> <answers> <answername>java is a platform</answername> <id>102</id> <postedby>john</postedby> </answers> <questionname>What is java?</questionname> </question>
POJO class: Question.java
import java.util.List; import javax.xml.bind.annotation.XmlAttribute; import javax.xml.bind.annotation.XmlElement; import javax.xml.bind.annotation.XmlRootElement; @XmlRootElement public class Question { private int id; private String questionname; private List<Answer> answers; public Question() {} public Question(int id, String questionname, List<Answer> answers) { super(); this.id = id; this.questionname = questionname; this.answers = answers; } @XmlAttribute public int getId() { return id; } public void setId(int id) { this.id = id; } @XmlElement public String getQuestionname() { return questionname; } public void setQuestionname(String questionname) { this.questionname = questionname; } @XmlElement public List<Answer> getAnswers() { return answers; } public void setAnswers(List<Answer> answers) { this.answers = answers; } }
Answer.java
public class Answer { private int id; private String answername; private String postedby; public Answer() {} public Answer(int id, String answername, String postedby) { super(); this.id = id; this.answername = answername; this.postedby = postedby; } public int getId() { return id; } public void setId(int id) { this.id = id; } public String getAnswername() { return answername; } public void setAnswername(String answername) { this.answername = answername; } public String getPostedby() { return postedby; } public void setPostedby(String postedby) { this.postedby = postedby; } }
XmlToObject.java
import java.io.File; import java.util.List; import javax.xml.bind.JAXBContext; import javax.xml.bind.JAXBException; import javax.xml.bind.Unmarshaller; public class XmlToObject { public static void main(String[] args) { try { File file = new File("question.xml"); JAXBContext jaxbContext = JAXBContext.newInstance(Question.class); Unmarshaller jaxbUnmarshaller = jaxbContext.createUnmarshaller(); Question que= (Question) jaxbUnmarshaller.unmarshal(file); System.out.println(que.getId()+" "+que.getQuestionname()); System.out.println("Answers:"); List<Answer> list=que.getAnswers(); for(Answer ans:list) System.out.println(ans.getId()+" "+ans.getAnswername()+" "+ans.getPostedby()); } catch (JAXBException e) { e.printStackTrace(); } } }
Output:
1 What is java?
Answers:
101 java is a programming language ravi
102 java is a platform john
Advantages of JAXB:
- It is simpler than SAX or DOM parser.
- No need to be aware of XML parsing techniques.
- No need to access XML in a tree structure.
Disadvantages of JAXB:
- It is a high layer API, so it has less control over parsing as compared to SAX or DOM.
- It is slower than SAX.